Jump to a key chapter
Front-End Development Explained
Front-end development involves creating the user-facing part of a website or application. It's essential for crafting a seamless and interactive user experience. This area of design is critical for student developers to understand as it bridges creativity with technology.
What is Front-End Development?
Front-end development is the practice of implementing graphical interfaces through code, allowing users to interact with websites. It's the combination of HTML, CSS, and JavaScript to build everything you see and use on the web. Key roles include ensuring that a site is visually appealing, interactive, accessible, and efficient.
The front-end interacts directly with users and is responsible for how a website looks and behaves. Developers often use HTML for structure, CSS for styling, and JavaScript for dynamic content. Together, these technologies build web pages with responsive designs and interactive elements.
Front-end development is not just about code, but also involves understanding user behavior and accessibility. Developers must create sites that work well across various devices and browsers while keeping load times manageable.
HTML (HyperText Markup Language) is the standard language for creating web pages. It describes the structure of a website using elements such as headings, paragraphs, links, and images.
Here's a basic example of HTML code structure for a webpage:
My Web PageWelcome to My Website
This is a paragraph of content.
Key Front-End Development Principles
Front-end development requires adherence to several core principles to ensure a consistent and effective user experience. These key principles include:
- Responsive Design: Ensuring your design adapts fluidly to different screen sizes and orientations for mobile, desktop, or tablet viewing.
- Performance Optimization: Keeping load times short and interactions snappy, improving both user experience and SEO.
- Clean Code: Writing semantic, organized, and readable code for easy maintenance and collaboration.
- Accessibility: Designing interfaces accessible to users with disabilities, including using Aria labels and ensuring keyboard navigation support.
Each principle plays a vital role in delivering an intuitive and engaging experience for users while also considering the technological and accessibility standards necessary for modern web development.
While responsive design is a well-known aspect of front-end development, the concept goes beyond merely scaling a site's layout. It involves fluid grids, flexible images, and CSS media queries. Responsive design allows for a single codebase to serve users on a variety of devices, dynamically adjusting to different resolutions and orientations to maintain usability and design integrity across all platforms.
Continuously testing your web pages on different devices and browsers is important to ensure consistent user experiences and diagnose any issues with responsiveness or performance.
Importance of Front-End Development Techniques
Mastering front-end development techniques brings numerous benefits to web development. These techniques not only enhance your ability to deliver appealing and usable web applications but also improve your site's performance and expand your audience reach.
- User Engagement: Visually appealing interfaces and interactive elements keep users engaged, reducing bounce rates and increasing site usage.
- Brand Representation: The way a site looks and feels is integral to conveying a brand's identity and values, requiring careful design and attention to detail.
- SEO Optimization: Front-end practices like structured data and optimized load speeds improve search engine ranking.
- Future-Proofing: By adopting modern techniques, developers can prepare their sites for future technological advancements and standards.
These front-end techniques support the continuous growth and maintenance of websites, aligning with both business goals and user expectations.
Elements of Front-End Design
In front-end development, focusing on key design elements is crucial for creating interactive and functional web experiences. By mastering these elements, you can enhance both usability and aesthetics, providing an optimal experience for users.
Core Elements of Front-End Design
Core elements in front-end design include effective use of HTML, CSS, and JavaScript to structure and style web pages. These technologies work harmoniously to deliver easy-to-use browser experiences.
HTML provides the skeleton or structure of web content, defining its various components. This includes headings, paragraphs, forms, and tables. HTML uses tags to describe the elements on a page, ensuring that all content is organized and accessible.
CSS (Cascading Style Sheets) is used for styling web pages. It determines the look and feel of elements on a webpage, including layouts, colors, fonts, and overall visual appearance.
Here's an example of how CSS can be used to change the background color of a webpage:
body { background-color: lightblue;}
JavaScript adds interactivity to a webpage. It's used to create dynamic content updatable in real-time. JavaScript can be used for functions such as responding to user events (clicks, keyboard inputs), creating animations, or even storing data in the user’s web browser with local storage.
Effective front-end design also involves considering user interface (UI) and user experience (UX) design principles. This includes organizing elements logically and designing intuitive navigation. Implementing responsive design ensures web pages are functional across a variety of devices and screen sizes.
Use consistent CSS naming conventions and organization to keep your style sheets manageable and maintainable over time.
Balancing Aesthetics and Usability in Front-End Design
Balancing aesthetics and usability is important in front-end design, as it involves creating a visually appealing design without compromising user experience. This balance is achieved by focusing on elements such as color schemes, typography, and layout while ensuring ease of navigation and accessibility.
The aesthetic elements of front-end design often involve the use of contrast, balance, and whitespace to enhance the visual appeal of a site. A well-chosen color scheme can create a mood or connect emotionally with users, while typography affects readability and helps convey the site's message.
For usability, it's essential to focus on navigational structure, intuitive UI elements, and responsive design. Ensuring that interactive elements such as buttons and links are clearly labeled and follow accessibility standards (e.g., using Alt text for images) can enhance the overall user experience.
In practice, achieving a balance between aesthetics and usability involves the careful consideration of form and function. Gestalt principles of design can be applied to front-end development to improve user perception and interaction with web content. This includes concepts such as proximity, similarity, and continuity, which help users process information quickly and efficiently. These principles support the delivery of a cohesive, intuitive design that aesthetically pleases while simultaneously meeting functional needs.
Front-End Development Techniques
Diving into front-end development requires understanding a variety of techniques that enhance a website's functionality and user experience. This field is constantly evolving, with new tools and methodologies improving how developers create interactive and efficient web pages.
Modern Front-End Development Techniques
Modern front-end development leverages technologies that streamline and enhance the process of creating web applications. With these techniques, complex interactions and vibrant designs become accessible.
Notably, frameworks such as React, Vue.js, and Angular enable developers to build complex single-page applications. These frameworks offer reusable components, state management, and efficient UI updates.
Another important technique involves Progressive Web Apps (PWAs). PWAs bridge the gap between web and native apps, providing offline access, push notifications, and home-screen icons. They offer a better user experience, particularly on mobile devices.
Using CSS Preprocessors like SASS or LESS allows developers to write more maintainable and scalable CSS. Features such as variables, nesting, and mixins reduce repetition and improve organization.
Here's an example of using SASS to define a variable for color:
$primary-color: #333;body { background-color: $primary-color;}
Leverage browser DevTools for in-depth debugging and optimization of your front-end code.
Tools Essential for Front-End Development
Front-end development relies on a range of tools that simplify coding, improve workflow, and enhance productivity. These tools cater to different aspects of development, from coding and collaboration to design and testing.
Code editors like Visual Studio Code, Sublime Text, and Atom, provide syntax highlighting, code completion, and extensions tailored for web development.
Version control systems, such as Git, allow developers to track changes and collaborate with teams. GitHub and GitLab offer cloud-based repositories to manage and share code.
Design tools such as Sketch, Figma, and Adobe XD are crucial for creating prototypes and UI mockups. They help translate design concepts into visually consistent front-end elements.
Task Runners and Module Bundlers, like Gulp and Webpack, automate repetitive tasks like minification, compilation, and file concatenation.
Webpack, as a module bundler, is incredibly powerful in handling complex build processes. It offers features such as code splitting, allowing scripts to be loaded as needed rather than in bulk. This reduces initial load times and enhances the performance of large applications. Additionally, Webpack's hot module replacement (HMR) offers rapid development feedback by injecting updates into the browser without a full page reload, promoting a more efficient development environment.
Responsive Design Techniques in Front-End Development
Responsive design is a cornerstone of front-end web development, ensuring that web pages adapt to the array of devices and screen sizes used today. It focuses on delivering an optimal viewing experience with minimal resizing or scrolling.
A fundamental technique in responsive design is the use of media queries in CSS. Media queries allow for specific styles based on device conditions like width, height, orientation, and display type.
Flexbox and CSS Grid are critical layout modules for creating complex grid systems that adjust based on screen size. These tools simplify the process of developing responsive sites by providing fluidity and flexibility in design.
Example of a simple media query to adjust a page's layout:
@media (max-width: 768px) { .container { flex-direction: column; }}
Remember to use viewport units (vw, vh) for responsive typography and element sizing, ensuring consistent scaling across devices.
Front-End Design Aesthetics
The aesthetics of front-end design play a vital role in crafting sites that are not only functional but also visually appealing. Effective use of design aesthetics can transform the user's interaction by creating an engaging and inviting environment.
Understanding Front-End Design Aesthetics
Front-end design aesthetics involve the art of integrating visually compelling elements within the structure of a website. It's about balancing the beauty with functionality to make a site intuitive and pleasing to the eye.
Aesthetics in design consider various factors such as color schemes, typography, imagery, and layout. Each element contributes to the overall sense of style and impacts how users perceive and interact with the site.
An effective aesthetic design leads to a positive emotional response, helping establish brand identity and user trust. It's not just about looking good; it's about fostering a connection with the user.
Typography refers to the visual style and appearance of the text on a website. It includes font choices, sizes, colors, and the spacing between lines and letters.
Consider using
font-family: 'Roboto', sans-serif;to ensure a clean and modern look throughout your website.
Consistent use of color and typography can improve a brand's recognition across different platforms.
Enhancing User Experience through Design Aesthetics
Design aesthetics enhance user experience by making websites not only visually appealing but also easier to navigate and understand. Effective aesthetic design encourages users to engage with content comfortably and intuitively.
Using whitespace effectively to separate content can improve readability. Strategic use of color can direct users' attention and create an intuitive way to interact with navigational elements.
Consistency is critical in aesthetic design. Ensuring a consistent color palette, typography, and element style across pages helps users familiarize themselves with your layout, reducing the learning curve and improving their overall experience.
Understanding color psycho
psychology is crucial to leveraging aesthetics effectively. Different colors can evoke different emotions and behaviors in users. For example, blue tends to be associated with trust and dependability, often used by financial institutions. Red can incite urgency and passion, frequently seen in call-to-action buttons. By harnessing these psychological effects, designers can subtly influence user engagement and conversion rates, aligning emotional design with strategic business goals.
Visual Hierarchy and Design Aesthetics
Visual hierarchy is a primary principle in design aesthetics that determines the order in which users perceive information. By organizing elements based on importance, designers guide users naturally through content in a logical sequence.
A successful visual hierarchy uses size, color, contrast, and positioning to highlight essential elements. Larger or bolder elements draw more attention, whereas the use of color contrast can make key elements stand out against a backdrop.
Utilizing a grid system can aid in maintaining consistency and alignment, providing a clear path for users to follow. This organized structure helps users process information efficiently without feeling overwhelmed.
An example of a simple grid system setup using CSS Grid:
.container { display: grid; grid-template-columns: repeat(3, 1fr); grid-gap: 15px;}
Remember, aligning key visual elements with user goals can improve navigation and accessibility, fostering a more engaging user experience.
front-end development - Key takeaways
- Front-end development explained: Creating the user-facing part of websites using techniques for a seamless and interactive experience, bridging creativity and technology.
- Front-end development techniques: Use of HTML, CSS, and JavaScript to build visually appealing, interactive, and accessible websites.
- Front-end development principles: Responsive design, performance optimization, clean code, and accessibility ensure effective user experiences.
- Front-end design aesthetics: Balancing aesthetics and usability through color, typography, and layout to create visually appealing and functional designs.
- Elements of front-end design: Effective use of HTML, CSS, and JavaScript for structuring and styling web pages, focusing on usability and responsiveness.
- Modern front-end techniques explained: Incorporating frameworks like React and Vue.js, PWAs, and CSS preprocessors to enhance website functionality and user experience.
Learn faster with the 12 flashcards about front-end development
Sign up for free to gain access to all our flashcards.
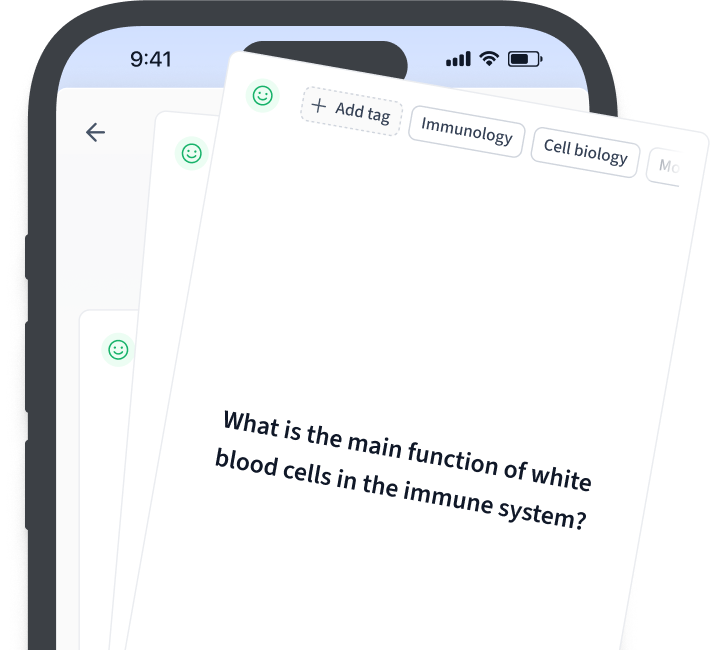
Frequently Asked Questions about front-end development
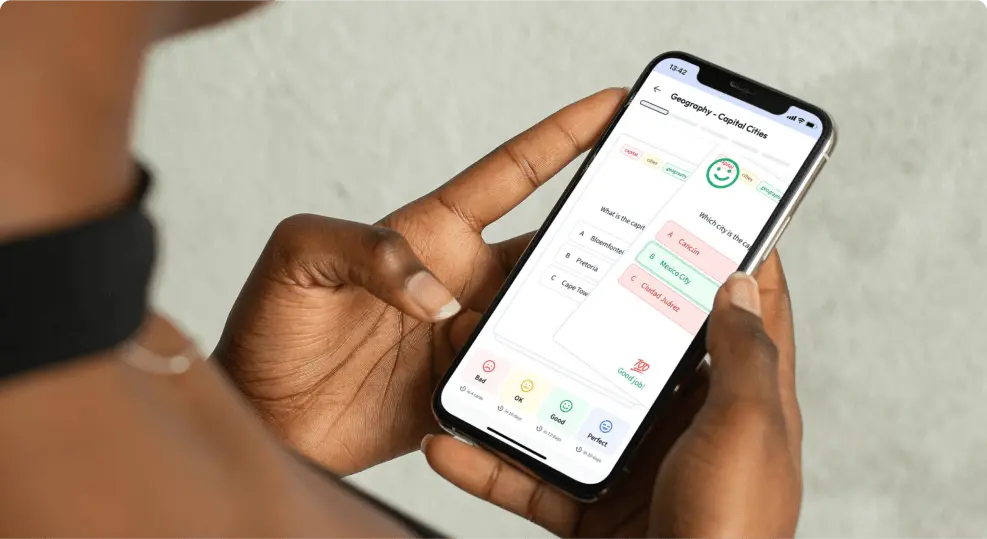
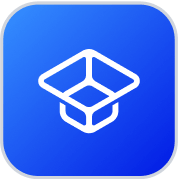
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more