Jump to a key chapter
Big O Notation Definition and Basics
Before diving into complex algorithms and data structures, it's essential to understand Big O Notation. This mathematical notation provides a way to describe the performance or complexity of an algorithm as its input size grows. It’s crucial for analyzing how the execution time or the space consumed by an algorithm scales with respect to its input size.
Understanding Big O Notation
Big O Notation helps you predict the behavior of an algorithm. It represents an upper bound on the time or space complexity of an algorithm. Understanding various Big O expressions enables you to make informed decisions when choosing the most appropriate algorithm for a specific problem.You can express Big O as several forms, depending on the algorithm's behavior:
- O(1): Constant Time - The execution time remains constant regardless of input size. Example: Accessing an array element.
- O(log n): Logarithmic Time - The execution time scales logarithmically as the input size increases. Example: Binary search.
- O(n): Linear Time - The execution time scales linearly with the input size. Example: A single loop through an array.
- O(n log n): Linearithmic Time - The execution time grows in a manner proportional to n log n. Example: Merge sort.
- O(n^2): Quadratic Time - The execution time is proportional to the square of the input size. Example: A nested loop over an array.
Big O Notation is a mathematical representation used to describe the upper bound of an algorithm’s time or space complexity in terms of its input size, denoted as n.
Consider a program that checks whether a number is prime by trying to divide it by every number less than itself. Its time complexity can be expressed as O(n), where n is the number because it potentially checks each number less than itself.
For efficiency, always prefer algorithms with lower Big O Notation when handling large data sets. Choosing O(log n) over O(n^2) can make a big difference in performance.
What is Big O Notation in Algorithms?
In the realm of algorithms, understanding their efficiency is crucial, and this is where Big O Notation comes into play. It's a method to describe the performance in both time and space that an algorithm requires as the input size increases. By providing an upper bound, it allows you to predict how well an algorithm will perform when scaling up. Big O Notation is a fundamental concept that helps you choose the most efficient algorithm for your needs.
Different Big O Complexity Classes
Big O Notation is expressed in different forms, each suited to specific algorithm behaviors. Understanding these forms can guide you in assessing different algorithms correctly:
- O(1): Constant Time ComplexityThis form denotes that the algorithm's execution time is constant and does not change with the size of the input data. Example: Accessing a specific element in an array.
- O(log n): Logarithmic Time Complexity Here, the execution time grows logarithmically, implying that doubling the input size produces a marginal increase in time. Example: Binary search algorithm.
- O(n): Linear Time Complexity The time scales linearly with the input size, meaning the time increases directly as the input increases. Example: Iterating over all elements in a list to find the largest number.
- O(n^2): Quadratic Time ComplexityThe execution time is proportional to the square of the input size. This often occurs with algorithms having nested loops. Example: Bubble sort algorithm.
The Big O Notation provides a high-level understanding of the algorithm performance limits, helping you estimate how algorithms will behave in certain conditions.
Let's say you write a program that checks if a number is prime by confirming no number less than the square root of the given number divides it without a remainder. The time complexity might appear as follows: O(\(\sqrt{n}\)), where \(n\) is the number in question. This is because you only need to check up to the square root of \(n\), not all numbers below \(n\).
In real-world scenarios, Big O Notation serves as more than just a theoretical concept. It's a tool that guides software engineers in optimizing program performance. By comparing different algorithms under similar conditions, engineers can choose the best method for the problem at hand. Despite its importance, remember that Big O Notation simplifies actual performance measurement because it doesn't account for constant factors or lower-order terms.For example, both O(n^2) and O(n^2 + n) simplify to O(n^2). However, in practice, the latter might run slightly faster with smaller values of \(n\) because of the additional term. In larger scales, however, these terms become negligible.Moreover, some algorithms might perform well with a smaller input size but degrade as the input size grows. Thus, while Big O Notation is a crucial starting point, it is equally important to perform empirical analysis and testing to ensure optimal performance across different scenarios.
A crucial aspect of using Big O Notation effectively is to consider not just the time complexity but also the space complexity, especially in memory-constrained environments like embedded systems.
Understanding Big O Notation Through Examples
In computer science, learning how to measure the efficiency of algorithms is vital, and Big O Notation plays a pivotal role in this process. It succinctly describes an algorithm's performance concerning time and space as the input size increases. This understanding helps in choosing the most efficient algorithm, especially when dealing with substantial datasets. Using examples can significantly improve comprehension of this influential concept.
Practical Examples of Big O Notation
To grasp Big O Notation effectively, it's beneficial to look at a few examples:
- O(1) - Constant Time ComplexityAccessing a specific element in an array regardless of its size. For instance,
element = array[0];
No matter how large the array is, the time taken to access an element is constant. - O(log n) - Logarithmic Time ComplexityBinary search on a sorted dataset exemplifies this. The algorithm divides the data into halves, effectively reducing the data to search in logarithmic steps.
- O(n) - Linear Time ComplexityConsider finding a maximum number in an unsorted list by iterating through each element. The time complexity grows linearly as the number of elements increase.
Consider a scenario where you must check if a list contains a particular value by examining each element. The time complexity is O(n). If n is the number of elements in the list, the worst-case scenario is examining every element.Suppose you implement this in Python:
def contains_value(lst, value): for item in lst: if item == value: return True return FalseThis function iterates over each element, making the time complexity O(n).
Binary search is efficient with O(log n) but requires sorted data to work correctly. Utilizing unsorted data results in O(n) with linear search.
Beyond understanding Big O Notation, it's crucial to consider other complexities like space complexity where an algorithm’s memory usage is analyzed. For instance, a recursive Fibonacci sequence calculator has a space complexity of O(n) due to the call stack:
def fibonacci(n): if n <= 1: return n return fibonacci(n - 1) + fibonacci(n - 2)Despite recursion providing simplicity, it poses memory overhead as each function call adds to the stack. Thus, considering both time and space complexities is vital when evaluating algorithm efficiency.An optimized approach can be memoization, where previously computed values are stored to eliminate repeated calculations, thereby reducing redundant processes.For instance, modifying the Fibonacci function with memoization reduces time complexity from O(2^n) to O(n) because it stores intermediate results:
def fibonacci_memo(n, memo={}): if n in memo: return memo[n] if n <= 1: return n memo[n] = fibonacci_memo(n - 1, memo) + fibonacci_memo(n - 2, memo) return memo[n]This adjustment considers both complexities, optimizing performance efficiently.
Big O Notation Applications in Computer Science
In computer science, Big O Notation has pervasive applications due to its ability to describe the efficiency of algorithms. Understanding these uses can significantly aid in analyzing and improving algorithm performance across various domains. It allows you to conceptualize how an algorithm handles increasingly large data efficiently.
Algorithm Efficiency in Data Structures
Big O Notation is extensively used to assess algorithm efficiency in data structures. Its purpose is to compare the performance of different operations like insertion, deletion, and searching across various data structures.Here's a quick overview of common data structures and their operations with respect to Big O Notation:
Data Structure | Insertion | Deletion | Search |
Array | O(1) | O(n) | O(n) |
Linked List | O(1)* | O(n) | O(n) |
Binary Search Tree | O(log n) | O(log n) | O(log n) |
For instance, consider a task where you need to store and find elements quickly. Using a hash table, you get average-case time complexities of O(1) for insertion and search. This marks a significant difference compared to an array, where the average search operation is O(n).Suppose you implement a basic hash table in Python:
class HashTable: def __init__(self): self.table = [None] * 10 def insert(self, key, value): index = hash(key) % len(self.table) self.table[index] = value def search(self, key): index = hash(key) % len(self.table) return self.table[index]This table allows for average-case O(1) operations for both insert and search when hashed keys don't collide.
When analyzing an algorithm, consider both worst-case and average-case complexities. Big O primarily provides worst-case insights.
Besides static data structure operations, Big O Notation extends to dynamic problems like sorting algorithms. Different sorting methods exhibit distinct time complexities, influencing the choice of algorithm based on the data set size and characteristics.Here's a table illustrating common sorting algorithms and their time complexities:
Algorithm | Best Case | Average Case | Worst Case |
Bubble Sort | O(n) | O(n^2) | O(n^2) |
Merge Sort | O(n log n) | O(n log n) | O(n log n) |
Quick Sort | O(n log n) | O(n log n) | O(n^2) |
Big O Notation - Key takeaways
- Big O Notation is a mathematical representation used to describe the upper bound of an algorithm's time or space complexity as input size grows.
- It serves as a tool to understand and predict algorithm efficiency, focusing on both time and space complexity.
- Several common Big O forms include: O(1) - constant time, O(log n) - logarithmic time, O(n) - linear time, O(n log n) - linearithmic time, and O(n^2) - quadratic time.
- Understanding Big O Notation allows for informed decisions in algorithm selection, especially when scaling data.
- The notation is widely applied across different computer science domains for algorithm comparison and improvement of performance under varying conditions.
- In practical applications, Big O Notation is important for both static and dynamic problems, such as data structure operations and sorting algorithms.
Learn faster with the 27 flashcards about Big O Notation
Sign up for free to gain access to all our flashcards.
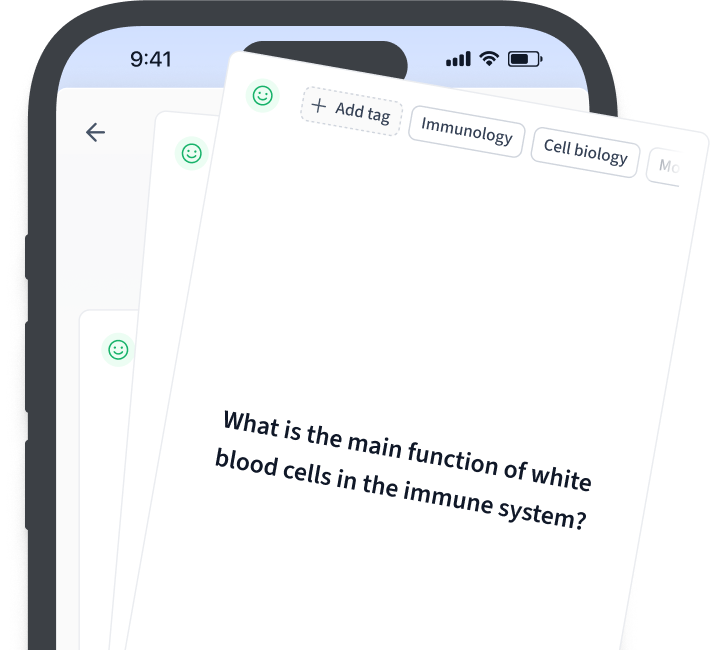
Frequently Asked Questions about Big O Notation
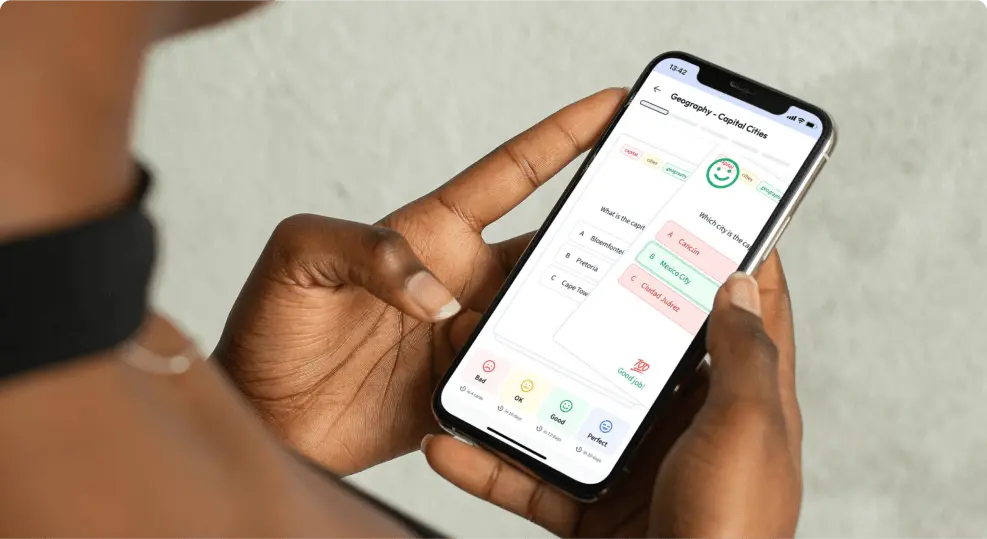
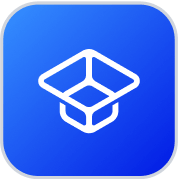
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more