Jump to a key chapter
Definition of Graph Traversal
Graph Traversal is a fundamental concept in computer science that involves visiting all the nodes, also known as vertices, in a graph. The goal is to ensure that every node is processed at least once, using a specific method to systematically go through the graph's vertices and edges.
Basic Concepts in Graph Traversal
In understanding graph traversal, it's important to be familiar with some foundational concepts:
- Graph: A collection of nodes (vertices) connected by edges.
- Node/Vertex: A fundamental unit of a graph representing an entity.
- Edge: A connection between two nodes in a graph.
Graph traversal can be utilized in various applications such as pathfinding, searching through databases, and solving puzzles. Different methods and algorithms can be employed based on the type of graph, whether it is directed or undirected, cyclic or acyclic, etc.
The primary objective of graph traversal is to visit and process each node in a graph. This can be achieved using different traversal strategies like Breadth-First Search (BFS) and Depth-First Search (DFS).
Breadth-First Search (BFS)
BFS is a strategy where the traversal starts from a selected node and moves across its neighbors before moving to the next level of nodes (i.e., nodes that are two edges away from the starting node). BFS is executed using a queue data structure.
For example, consider the following graph:
`A---B---C---D`
If you start BFS from Node A, the nodes visited would be A, B, C, D
Depth-First Search (DFS)
In DFS, the traversal proceeds by moving as far ahead as possible along each branch before backing up. This is typically implemented using a stack or recursion.
Using the same graph:
`A---B---C---D`
Starting DFS from Node A, one possible sequence is A, B, C, D. This continues deepening down each path until all nodes are visited.
Both BFS and DFS have time complexities of O(V + E), where V is vertices and E is edges.
Applications of Graph Traversal
Graph traversal is widely used in various fields:
- Networking: Finding the shortest path between devices.
- Web Crawlers: Traversing through web pages.
- Artificial Intelligence: Navigating through search spaces.
Techniques of Graph Traversal
Graph traversal is essential for navigating and understanding the relationships between nodes in a graph. Two primary algorithms, Breadth-First Search (BFS) and Depth-First Search (DFS), offer systematic approaches to explore graphs effectively.
Graph Traversal Algorithms Overview
The selection of a graph traversal algorithm depends on the intended use and the nature of the graph. Both BFS and DFS algorithms are fundamental, each having distinct approaches and use cases:
- BFS is ideal for finding the shortest path in unweighted graphs and exploring all nodes by level.
- DFS is effective for pathfinding or discovering connected components.
Understanding the data structures employed is crucial:
- BFS utilizes a queue ensuring nodes are processed in the order they are discovered.
- DFS makes use of a stack, often implemented recursively.
A graph traversal algorithm is a method used to visit or check vertices in a graph. The traversal ensures systematic and comprehensive examination of vertices and edges.
Choosing between these algorithms relies on specific applications:
- BFS is often used in scenarios like peer-to-peer network communication, shortest path finding in navigation systems, and social networking sites.
- DFS finds extensive application in puzzles (solving mazes), topological sorting in compiler design, and detecting cycles in graphs.
Both algorithms fundamentally operate with a time complexity of \(\text{O}(V + E)\), where \(V\) is the number of vertices and \(E\) the number of edges.
BFS Graph Traversal
Breadth-First Search (BFS) is an algorithm to traverse or search graph data structures. It begins at the root node and explores all neighboring nodes; once all neighbors are explored, it moves to the next level.
The process of BFS can be described as:
- Initiate at the source node and enqueue it.
- Dequeue a vertex, process it, and enqueue its adjacent nodes.
- Repeat until every vertex is processed.
Consider the following adjacency list representation:
{ 'A': ['B', 'C'], 'B': ['A', 'D', 'E'], 'C': ['A', 'F'], 'D': ['B'], 'E': ['B', 'F'], 'F': ['C', 'E']}
Running BFS from Node A, the output would look like A, B, C, D, E, F.
Don't forget that BFS is perfect for unweighted graphs when you need to find the least number of edges from start to target.
Depth First Traversal Graph
Depth-First Search (DFS) explores as far as possible along each branch before backtracking. DFS can be implemented using a recursive approach or an iterative one using a stack.
The DFS algorithm steps are as follows:
- Visit the starting node and push it onto the stack.
- Explore each unvisited neighbor, push to stack, and recurse.
- Backtracking through previously visited nodes as needed.
Take this adjacency list for instance:
{ 'A': ['B', 'C'], 'B': ['A', 'D', 'E'], 'C': ['A', 'F'], 'D': ['B'], 'E': ['B', 'F'], 'F': ['C', 'E']}
Executing DFS starting at Node A could result in traversal such as A, B, D, E, F, C.
DFS excels in tasks involving exhaustive searches over all paths or nodes.
Graph Traversal Examples for Students
Graph traversal is a key technique in computer science, helping with the exploration of nodes and connecting edges. Through examples and explanations, you'll grasp how these traversal methods come to life in various applications, learning to harness their power for problem-solving.
Illustrative Examples of Breadth-First Search
Breadth-First Search (BFS) is often employed in scenarios where you need to explore all possible options level by level. In a simple graph, the process is demonstrated as follows:
- Start at the root node.
- Visit and enqueue each neighbor.
- Continue visiting, queuing, and processing subsequent levels.
Imagine a typical social network where nodes represent people and edges are friendships:
Consider a graph of friend connections:
{ 'You': ['Alice', 'Bob'], 'Alice': ['You', 'Cora'], 'Bob': ['You', 'David'], 'Cora': ['Alice'], 'David': ['Bob']}
BFS starting from 'You' might follow this order: You, Alice, Bob, Cora, David.
BFS can have variations such as finding the shortest path during traversal. Because it explores all nodes at the current level before moving deeper, BFS can effectively determine the quickest way to link any two nodes, which is particularly useful when nodes represent unweighted paths.
Explorative Examples of Depth-First Search
Depth-First Search (DFS) takes a different approach by diving as deep into a network as possible before backtracking. This method is particularly useful for tasks like analyzing structures or networks deeply connected to one another.
For a simpler path exploration, consider:
{ '1': ['2', '3'], '2': ['4'], '3': ['1', '5'], '4': ['2', '6'], '5': ['3'], '6': ['4']}
Using DFS starting from node '1', you could traverse: 1, 2, 4, 6, 3, 5.
DFS efficiently resolves problems like cycle detection and pathfinding where the path is weighted or involves backtracking.
Applications of Graph Traversal Algorithms
Graph traversal algorithms are vital in a wide variety of applications across multiple domains. From computer networks to artificial intelligence, the ability to explore nodes efficiently offers solutions to complex problems.
Networking and Data Transmission
Breadth-First Search (BFS) and Depth-First Search (DFS) are used in networking to determine optimal routing paths, ensuring efficient data transmission. With BFS guiding unweighted shortest paths, network protocols often employ it to minimize travel distances between nodes.
In packet routing, graphs are created with devices as nodes and connections as edges, making traversal algorithms key in maintaining quick communication channels.
Spanning Tree Protocol (STP) is an advanced application of graph traversal in networking. This protocol, involving tree-like structures, ensures loop-free network reconfigurations. By leveraging tree structures formed via DFS, STP efficiently manages redundant paths.
Artificial Intelligence and Games
Graph traversal is fundamental in AI for pathfinding and decision making. In game development, algorithms such as A* use graph traversal for navigating characters within virtual environments.
Consider a playing field modeled as a graph:
- Nodes represent game states.
- Edges denote possible moves between states.
Traversal aids in orienting AI-driven characters, finding optimal strategies in games like chess or Go.
Queue and stack management in traversal algorithms simulate decision trees, aiding AI in real-time decision-making.
Social Network Analysis
In social networks, graph traversal algorithms explore relationships between users, efficiently analyzing user connectivity. BFS pinpoints friend recommendations by assessing immediate contacts, while DFS dives deeper into analyzing user influence or network subgroups.
Social media platforms rely heavily on these algorithms for:
- Friend suggestions.
- Trend analysis.
- Influence measurement.
Imagine a platform where users form nodes:
{ 'User1': ['User2', 'User3'], 'User2': ['User1', 'User4'], 'User3': ['User1'], 'User4': ['User2']}
Graph traversal finds paths between influencers and followers, maximizing engagement studies.
Biological and Chemical Research
In biological sciences, graphs model molecular structures and interactions. Graph traversal helps in identifying pathways in metabolic networks or visualizing molecular interactions.
For example, DFS can trace pathways in biochemical networks:
- Understand enzyme reaction sequences.
- Identify potential drug targets by examining protein-ligand interactions.
Understanding metabolic pathways through traversal illuminates interconnections in intricate biological systems.
Graph Traversal - Key takeaways
- Definition of Graph Traversal: The process of visiting all the nodes (vertices) in a graph to process each at least once through a systematic method involving the graph's vertices and edges.
- Graph Traversal Algorithms: Key methods include Breadth-First Search (BFS) and Depth-First Search (DFS), utilized to explore graph structures efficiently.
- BFS Graph Traversal: An algorithm that explores the neighbor nodes level by level starting from a selected node using a queue.
- Depth First Traversal Graph: An approach where the exploration dives deep into each branch before backtracking, utilizing a stack or recursion.
- Techniques of Graph Traversal: Systematic approaches (BFS and DFS) to explore graph structures, each with distinct use cases and applications.
- Graph Traversal Examples for Students: Illustrative examples of BFS and DFS demonstrating traversal on graph structures for practical understanding.
Learn faster with the 27 flashcards about Graph Traversal
Sign up for free to gain access to all our flashcards.
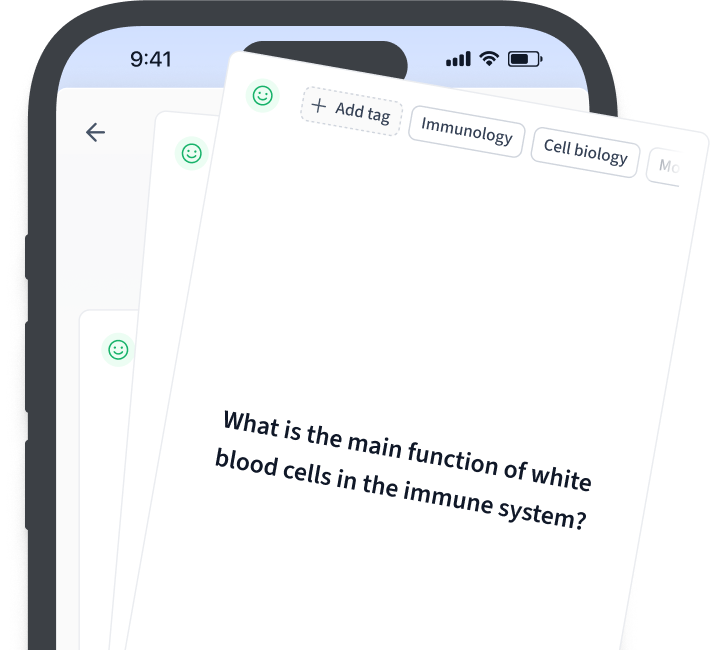
Frequently Asked Questions about Graph Traversal
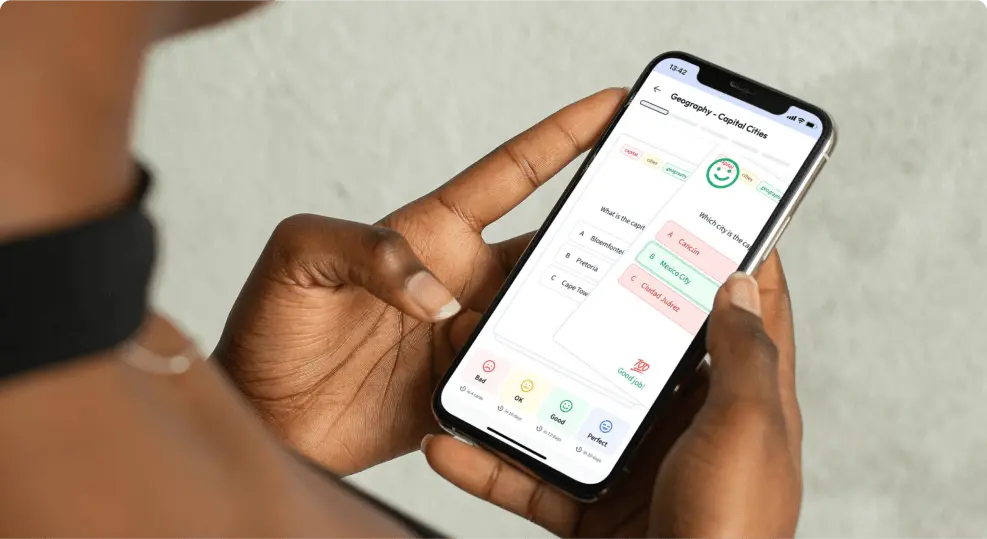
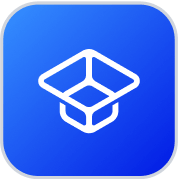
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more