Jump to a key chapter
Understanding Linear Search in Computer Science
Linear Search, or Sequential search, is a simple, yet powerful algorithm commonly adopted in Computer Science. This method is employed to locate a specific value within an array by sequentially checking each component until the target value is located, or all components have been checked.Linear Search is an algorithm that iteratively checks each element in an array, starting from the first element, in a linear or sequential manner until it finds a match with the target value. If the algorithm does not find a match, it denotes that the target value is not present in the array.
Definition of Linear Search Algorithm
The Linear Search algorithm operates by comparing each element in the array with the target value. Starting from the first element, it sequentially moves through the array until it either finds a match or exhausts all possible elements. The pseudocode can be visualised as follows:Start from the leftmost element of arr[] and one by one compare the target with each element of arr[] If the target matches arr[i], then return the index 'i' If the target doesn’t match with any of the elements, return -1The time complexity of the Linear Search algorithm is \(O(n)\) where \(n\) is the number of elements in the array.
Linear Search is usually preferred when the array has a small number of elements or when performing a single search in an unsorted array. For larger or sorted arrays, more efficient algorithms like Binary Search or Hashing should be considered.
The Process Behind Linear Search in Computer Science
The Linear Search process is simple to understand. Imagine the values in the array as a line of cards laid out on a table. The search process involves looking at each card, one by one, until the target card is found or all cards have been checked. This process can be broken down into the following steps:- Start from the first element in the array.
- Compare the target value with the current array element.
- If they match, return the current index.
- If they do not, move to the next element and repeat the process.
- If the end of the array is reached without finding a match, the search has failed and -1 is returned.
Practical Linear Search Example
Now, let's consider a practical example of a Linear Search. Suppose there is an array, and the goal is to find if the number '5' exists in the array and record its index. We have an array: Array: [2, 3, 4, 5, 6] Now, we'll run the linear search algorithm:Starting from the first index, the algorithm checks if '2' equals '5'. As they do not match, we move to the next element. The process is repeated for '3' and '4'. Upon reaching '5', as '5' equals '5', the algorithm stops, and the current index is returned.
Advantages of Linear Search
The simplicity of the Linear Search algorithm is what often deems it the go-to choice in numerous scenarios of array exploration. Its primary advantages can be encapsulated in three important elements:- Implementation ease - Linear Search is straightforward to understand and code.
- Space efficiency - Unlike other searching algorithms, Linear Search does not require any extra space since it works on the existing data structure.
- Unordered data - It is effective on both sorted and unsorted arrays, irrespective of the order of the elements.
Efficiency in the Application of Linear Search Algorithm
The Linear Search algorithm is undoubtedly a beneficial player when it comes to array searching activities in Computer Science. Its relative efficiency to alternative search algorithms depends a lot on the nature and structure of the data set employed. The time complexity of the Linear Search algorithm is \(O(n)\), and is thus correlated directly to the size of the array. This essentially signifies that the total time required for execution increases with the increase in the number of elements in the array.Time complexity is a computational complexity that describes the amount of computational time taken by an algorithm to run, as a function of the size of the input to the program. The time complexity of algorithms is most commonly expressed using Big O notation.
A Binary Search algorithm operates by dividing the data set into two halves and then continuously checking the middle element of the current half until the desired element is found or all elements have been checked. The necessity for the data set to be sorted prior to carrying out a Binary Search poses a constraint to its implementation, particularly if the data set is frequently updated or modified.
Scenarios Where Linear Search Holds an Advantage
Despite its simplicity, Linear Search lends itself exceptionally well to certain situations. Considering its nature, the Linear Search algorithm turns out to be the preferred choice in the following circumstances:- Small Datasets: In an environment where the data sizes are small, Linear Search shines due to its simplicity. It eliminates the overhead of more complicated algorithms.
- Unsorted Datasets: When dealing with unsorted data arrays, Linear Search is often the most practical solution. More refined algorithms such as a Binary Search are not functional with unsorted arrays unless a sorting technique is implemented at the outset.
- Sequential Memory: Linear Search works seamlessly with an array or list where data is stored sequentially in memory. This algorithm naturally maps to how this data is structured, improving its accessibility.
- Searching for multiple instances: Linear Search does not cease its operation at the identification of the first instance of the target, allowing the algorithm to locate and return multiple occurrence indices of the target value, if desired.
Analysing Linear and Binary Search
In the realm of Computer Science, Linear Search and Binary Search hold prominent positions as practical and common searching algorithms. While a Linear Search works optimally with small and unsorted data sets, Binary Search demonstrates its effectiveness with larger, sorted arrays. Understanding the intricacies of each algorithm and its application can help in making the most of these powerful searching tools.Distinctions Between Linear and Binary Search
The key dissimilarities between Linear Search and Binary Search stem from their underlying workings, efficiency, and prerequisites.- Working Principle: While Linear Search inspects every element in sequence, starting from the beginning of the list until the target value is found or the list is exhausted, Binary Search adopts a divide-and-conquer methodology. Binary Search begins at the median value and repeatedly halves the search space until the target is located.
- Efficiency: The time complexity of Linear Search is \(O(n)\) owing to its linear progression. On the other hand, Binary Search sports a time complexity of \(O(\log n)\) as it efficiently reduces the search space by half after every comparison.
- Prerequisites: Linear Search works without any prerequisites concerning the order of data. Conversely, Binary Search requires the data set to be pre-sorted to function correctly.
Deciding Between Linear Search and Binary Search
The selection between Linear and Binary Search comes down to understanding the data structure in question and the context of the problem.- Data Size: For smaller data sets, a Linear Search is often sufficient and might even be faster than a Binary Search, considering the latter's initialization overhead.
- Data Order: If the data is unsorted and sorting it is not efficient (due to time constraints or frequent updates), a Linear Search becomes the feasible option. Binary Search mandates a sorted list.
- Number of Searches: If the list is large but has to be searched frequently, the overhead cost of sorting to allow Binary Search might be worth it in the long run because of its high search speed for subsequent searches.
- Memory Constraints: Binary Search might require more memory than a Linear Search due to the recursive nature of its algorithm.
Linear and Binary Search: Practical Examples
Let's envisage the practical usage of both Linear and Binary Search using a simple scenario where we have a sorted array of 10 elements, and the aim is to find the number '8'.Linear Search
The Linear Search algorithm begins at the start of the array and checks each element until it finds the number '8' or traverses the whole array. Even though the array is sorted, the Linear Search algorithm does not take this into account. In the worst-case scenario, with our target being '8', it would take 8 comparisons to find the element.
Binary Search
The Binary Search algorithm, on the other hand, takes the median value in the array and compares it with the target value. If the target is equal to the median, the search is successful. If the target is less or more, the array is virtually halved, and the search is resumed within the relevant section. In the worst-case scenario, the target '8' would be found in 4 comparisons, thereby establishing the greater efficiency of Binary Search for sorted, larger datasets.
Implementing Linear Search in Computer Programming
The implementation of Linear Search operates on a relatively straightforward principle: check each element in the dataset until a match is found or all elements have been examined. This simple structure allows for its adaptable implementation across multiple programming languages, including widely-used ones like Python, JavaScript, Java, C++, and more. Despite the syntax differences between these languages, the underlying methodology remains consistent.Building a Linear Search Algorithm: Step-by-Step
Let's delve into the step-by-step process of creating a linear search algorithm. For illustrative purposes, we'll create a Python function.
1. Start by defining a function, let's name it `linear_search`, that takes in two arguments: a list (which we'll call `data`) and a target value (`target`).
2. Loop over the list by index. In Python, you can use the built-in `enumerate()` function for this. The `enumerate()` function adds a counter to an iterable and returns an enumerated object containing pairs of index and elements.
3. Inside the loop, compare the current item with the target. If they match, return the current index to indicate the position where the target was found.
4. If the loop completes without returning, then the target must not be in the list. In this case, return `-1` or some indication that the search was unsuccessful.
Here is what that looks like in Python code:
def linear_search(data, target): for i, item in enumerate(data): if item == target: return i return -1
This function will search through `data` until it finds `target`; it will then return the index of `target`. If `target` is not in `data`, it will return `-1`.
Ideal Situations for the Use of Linear Search in Programming
Linear Search finds its optimal implementation in specific situations, chiefly dictated by the nature of the dataset and the problem at hand. The simplicity and ease-of-use of Linear Search make it a favourable choice in the following scenarios: 1. Small Datasets: With fewer elements to search, Linear Search is both efficient and quick to implement. The overhead complexity that comes with more advanced algorithms is usually not worth the marginally improved performance for small datasets. 2. Unknown Data Length: This technique is an excellent fit when the length of the dataset is unknown since it doesn't require any setup steps and can begin searching immediately. 3. Unsorted Data Arrays: As Linear Search does not require any order in the dataset, it proves an advantage when handling unsorted data. Algorithms like Binary Search, which need sorted data, are not practical in such cases unless we sort the dataset beforehand, incurring additional overhead. 4. Sequential Memory Access: Datasets with sequential memory layout like arrays and lists are naturally suited for Linear Search, as it directly maps to this data structure. 5. Multiple Matches: Linear Search is ideal if we need to find all matches of a target value in a dataset. It effortlessly traverses the entire array, finding and noting all occurrences. While these situations underpin the areas where Linear Search takes precedence, it's vital to understand that the choice of algorithm will always depend on the specific needs and constraints of your programming task. It's crucial to have a toolbox of algorithms and to understand when and where to apply each.Linear Search - Key takeaways
Linear Search is a simplistic algorithm used in computer science to locate a specific value within an array by sequentially checking each component.
The process of Linear Search involves starting from the first element of an array and checking each element in a sequential manner until a match with the target value is found. If no match is found, it implies the target value is not present in the array.
The pseudocode for a Linear Search algorithm typically involves starting from the leftmost element, comparing the target value with each element, and either returning the index of a matching value or -1 if no match is found.
The time complexity of the Linear Search algorithm is \(O(n)\), where \(n\) is the number of elements in the array.
Linear Search is most effective when dealing with small datasets or unsorted arrays. In large or sorted arrays, more efficient algorithms like Binary Search should be considered.
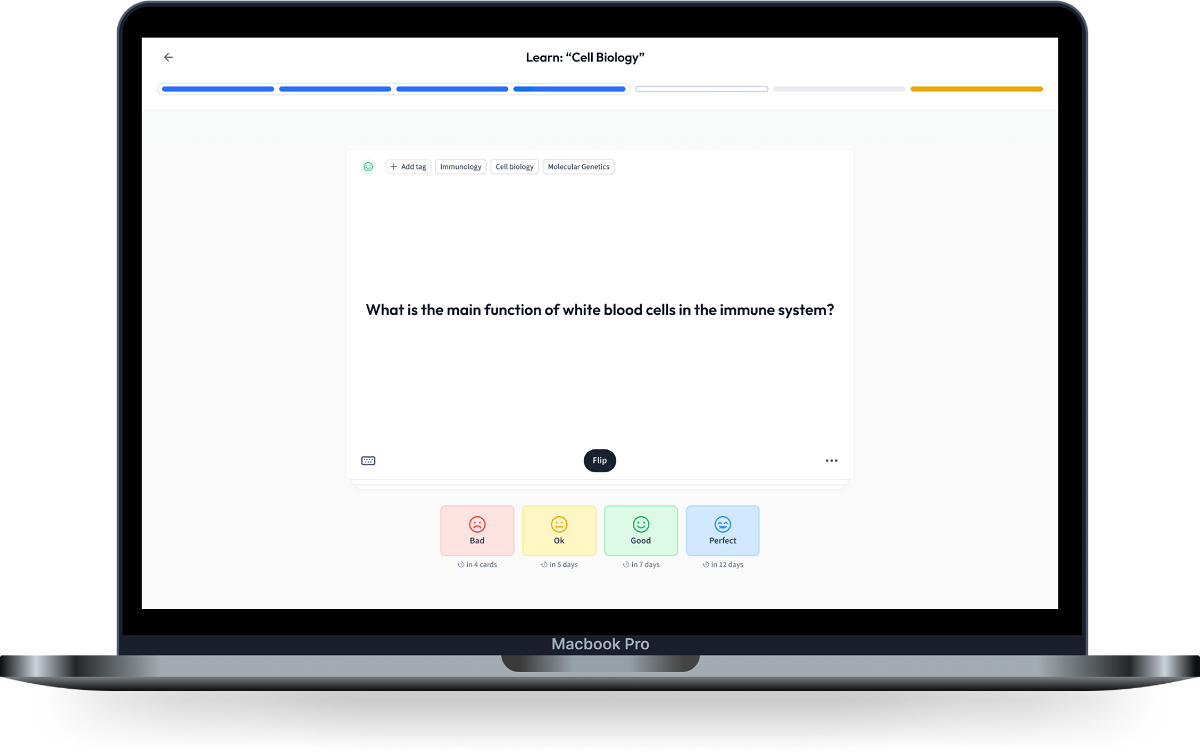
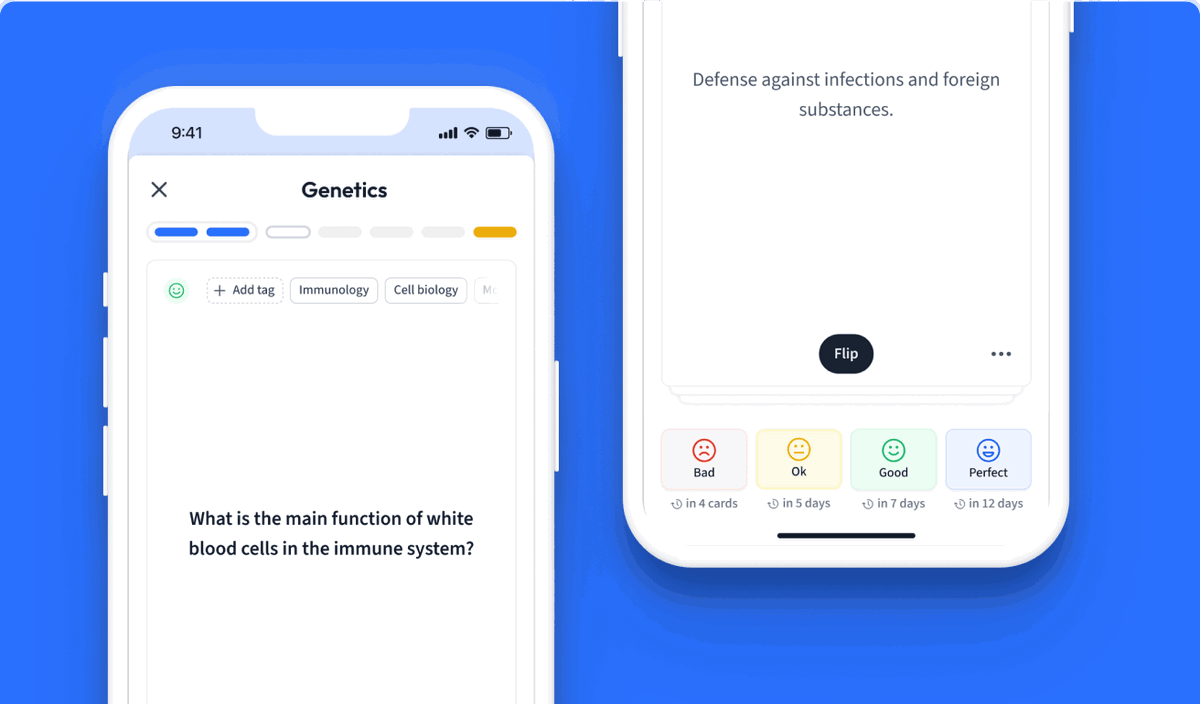
Learn with 16 Linear Search flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Linear Search
What is linear search?
Linear search is a simple method used to search for a particular value in a list or array. It works by starting at the first item in the list and sequentially going through each item until the desired value is found or until it has looked at all elements. It is straightforward and practical when searching small data sets. However, it may not be the most efficient method for large data sets because it looks at each item one-by-one in order.
How does a linear search work?
A linear search works by sequentially checking each element in a list until it finds the target value. It starts searching from the beginning of the list and moves towards the end, comparing each element with the target value. If the target value matches with an element, the search ends. If the end of the list is reached without finding the target, it indicates that the target is not in the list.
How does a linear search algorithm work?
A linear search algorithm works by sequentially checking each element of the array from start to end until the desired element is found or all elements have been checked. It starts at the first element of an array and moves element to element in a linear fashion. If the desired element is found, the search ends and returns the index of the element. If the element is not found after checking the entire array, the algorithm returns a message indicating that the element is not in the array.
How to do a linear search?
To perform a linear search, start at one end of the array or list and scan through each element one by one. Compare each element with the target value you're looking for. Continue this process until you either find a match or reach the end of the array or list. If you reach the end without finding a match, the item is not in the array or list.
What is a linear search algorithm?
A linear search algorithm is a basic search algorithm that checks each element of a list sequentially until a match is found or the whole list has been searched. It's the simplest form of search algorithm, suitable for small data sets, but can be inefficient for larger ones. It doesn't require the list to be sorted and operates by comparing each element to the target value until the desired element is found or all elements have been checked.
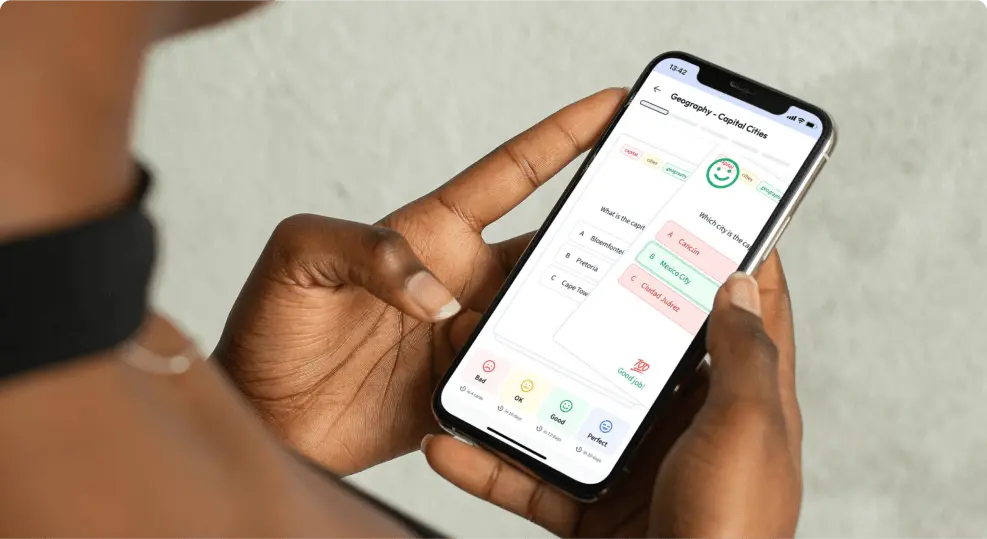
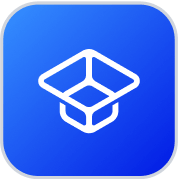
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more