Jump to a key chapter
Understanding Merge Sort
Before jumping into the intricacies of Merge Sort, it's essential to understand its fundamental principle. You're likely to stumble upon this powerful and efficient algorithm when dealing with data sorting in Computer Science.
Merge Sort is an efficient, stable, comparison-based sorting algorithm, highly appreciated for its worst-case and average time complexity of \(O(n \log n)\), where \(n\) represents the length of the array. This algorithm follows the divide-and-conquer programming approach, which essentially breaks down a problem into sub-problems until they become simple enough to solve.
The term 'stable' in the context of sorting algorithms indicates that equal elements retain their relative order after sorting. This characteristic, combined with the algorithm's efficiency makes it a popular choice for numerous applications, especially when working with large datasets.
Definition of Merge Sort Algorithm
In the simplest of terms, the Merge Sort algorithm divides an unsorted list into \(n\) sub-lists with each containing one element, then repeatedly merges sub-lists to produce newly sorted sub-lists until there is only one sub-list remaining. This pattern of divide, conquer, and combine gives a solution to the problem at hand.
Consider an unsorted array \([2, 5, 1, 3]\). The Merge Sort algorithm starts by dividing this array into sub-arrays until each contains only one element: \([2]\), \([5]\), \([1]\), and \([3]\). It then merges the sub-arrays in a manner that they're sorted, resulting in the sorted array \([1, 2, 3, 5]\).
The primary two operations within this algorithm are the 'Divide' and the 'Conquer' steps. 'Divide' is the step where the array is divided into two halves, whereas the 'Conquer' step involves resolving the two halves that have been sorted individually.
The Process of Merge Sorting
The process of Merge Sorting is a little intricate as different activities happen simultaneously. It all starts with the division of the initial unsorted array, and as the sorting progresses, smaller sorted lists are merged to form a larger sorted list until finally one sorted array is formed.
Basic Steps in Merge Sorting
Merge sorting comprises a series of steps. Here are the ones that merit your keen attention:
- Step 1: Divide the unsorted list into \(n\) sub-lists, each containing one element. This is achieved by breaking down the list in half until only individual elements are left.
- Step 2: Repeatedly merge sub-lists to create a new sorted sub-list until only a single sorted sub-list is left. This can also be considered a 'conquer' phase.
Practical Merge Sort Example
To illustrate how Merge Sort operates, let's take a look at a practical example. Consider an array of numbers: 14, 33, 27, 10, 35, 19, 48, and 44.
Before applying Merge Sort, the array looks like this:
14 | 33 | 27 | 10 | 35 | 19 | 48 | 44 |
---|
After applying the Merge Sort algorithm, the final sorted array becomes:
10 | 14 | 19 | 27 | 33 | 35 | 44 | 48 |
---|
Time Complexity for Merge Sort
Understanding the time complexity for Merge Sort is critical as it provides insights into the algorithm's efficiency. Time complexity essentially refers to the computational complexity that evaluates the amount of computational time taken by an algorithm to run, as a contributing factor is the size of the input.
Time Complexity Explanation
In computer science, the concept of time complexity is pivotal when it comes to analysing algorithms. Time complexity provides a measure of the time an algorithm requires to execute in relation to the size of the input data. It's indicated using Big O notation, which describes the upper limit of time complexity in the worst-case scenario.
In more simplified terms, time complexity represents how scalable an algorithm is. The less the time complexity, the more efficient the algorithm, especially when dealing with larger datasets.
For Merge Sort, time complexity is calculated in terms of comparisons made while sorting the elements.
It's important to note that Merge Sort is among the most efficient sorting algorithms due its linear-logarithmic time complexity. Considering its ability to manage large amounts of data, it's frequently employed in scenarios where stability of data is required, and time efficiency is of essence.
function mergeSort(array){
// Base case or terminating scenario
if(array.length <= 1){
return array;
}
// Find the middle point with integer division
var middle = Math.floor(array.length / 2);
// Call mergeSort for first half:
var left = mergeSort(array.slice(0, middle));
// Call mergeSort for second half:
var right = mergeSort(array.slice(middle));
// Combine both halves:
return merge(left, right);
}
Best Case Scenario in Time Complexity for Merge Sort
In the context of time complexity, the best case scenario happens when the input data to be sorted using Merge Sort is already in order, either fully or partially.
Let's say you have an array like \([1, 2, 3, 4, 5]\). In the best-case scenario, no extra comparisons are needed because the array is already sorted. So, the best-case time complexity for Merge Sort is still \(O(n \log n)\).
This means for Merge Sort, the best-case scenario is as efficient as merging one sorted list of \(n\) elements, which gives it a complexity of \(O(n \log n)\), the same as the worst-case scenario. This is one of the reasons why Merge Sort is reliable while dealing with large data sets.
Worst Case Scenario in Time Complexity for Merge Sort
It's also important to consider the worst-case scenario in time complexity, which for Merge Sort happens when the input data is in reverse order or when all elements are identical.
So, if you have to sort an array like \([5, 4, 3, 2, 1]\) or \([4, 4, 4, 4, 4]\), the Merge Sort algorithm will go through the entire process of dividing and merging, resulting in \(O(n \log n)\) operations.
Given that Merge Sort's algorithm splits the input data into two equal halves recursively, the computation for every element will be done \(\log n\) times. Therefore, in total, Merge Sort performs \(n \log n\) calculations in the worst-case scenario, providing it a worst-case time complexity of \(O(n \log n)\). The central feature here is that the time complexity remains consistent, regardless of the initial order of data in the input list.
Advantages of Merge Sort
Like all computer science algorithms, Merge Sort comes with its own unique advantages that make it a go-to solution in certain situations. Particularly, it shines in aspects such as efficiency and stability, among others.
Efficiency of the Merge Sort Algorithm
When it comes to sorting data, efficiency is always a key consideration. In computer science jargon, this typically means the algorithm's ability to manage resources like time and space effectively. Merge Sort, in this case, is recognised for its impressively high efficiency.
Time efficiency is of utmost importance in algorithms because the shorter the time an algorithm takes to execute, the more data points it can handle in a given period. Merge Sort, with its time complexity of \(O(n \log n)\), offers reliable efficiency, making it an excellent choice for large datasets.
However, it's crucial to note that Merge Sort is not necessarily the most space-efficient algorithm. It uses additional space proportional to the size of the input data, giving it a space complexity of \(O(n)\). This is because, during the sorting process, the algorithm creates additional arrays for storing the temporarily divided data. While this could be a concern in space-restricted cases, contemporary systems with ample memory often overshadow this downside with the benefit of time efficiency.
Stability of Merge Sort
Stability typically suggests that an algorithm maintains the relative order of equal elements - Merge Sort excels at this. This stability comes in handy in scenarios where the original order holds significance and needs to be maintained post-sorting.
In sorting algorithms, stability refers to the algorithm's capacity to maintain the relative order of identical inputs. In simple terms, if two equal elements appear in the same order in the sorted output as they were in the input, the algorithm is deemed 'stable'.
The stability property of Merge Sort algorithm bolsters its applicability in various real-world sorting problems where the preservation of relative order is a substantial requirement. For instance, in applications like sorting a list of documents by date and then sorting the same list by author, stability ensures that the original sort order is maintained within the second sort order.
Application Examples of Merge Sort
Merge Sort is a versatile algorithm with potential applications in numerous scenarios, owing to its dependable efficiency and stability.
An example of where Merge Sort shines is in processing large datasets where the data is stored in external storage such as disk drives or databases. Given that these data repositories cannot support other efficient, in-memory sorting algorithms due to their limit on simultaneous memory holding, Merge Sort becomes the default choice with its ability to handle disk-loaded (or external) data.
Another classic example is its usefulness in sorting linked lists. Since Merge Sort does not require random access to elements (like arrays do), it can sort linked lists with \(O(1)\) extra space, making it an efficient and practical solution.
- E-commerce Catalogues: Merge Sort can help arrange a store's inventory in an orderly manner, particularly when dealing with numerous product items.
- Database Management: Merge Sort is applicable in sorting large databases efficiently, such as those in hospitals, schools, government agencies, and corporations.
- Sorting Mail: Postal departments can greatly benefit from Merge Sort, arranging mail by postal code, ensuring quick and efficient delivery.
Real-world applications of Merge Sort extend to managing sundry data types like strings and floating-point numbers. It delivers an excellent sorting solution when dealing with data that has complex comparison operations or needs to preserve relative element order.
Working with Merge Sort Algorithm
Walking through the workings of the Merge Sort algorithm offers valuable insights into its operations. This computational mechanism is central to understanding and employing the algorithm effectively in practical scenarios.
Detailed Workflow with Merge Sort
Working with the Merge Sort algorithm entails a series of steps revolving around the core principle of 'divide and conquer'. Whether you’re dealing with a small array or a large dataset, each operation remains almost identical. The entire workflow can be summarised into three distinct phases: Division, Sorting, and Merging.
function mergeSort(array){
// Base case or terminating scenario
if(array.length <= 1){
return array;
}
// Find the middle point with integer division
var middle = Math.floor(array.length / 2);
// Call mergeSort for first half:
var left = mergeSort(array.slice(0, middle));
// Call mergeSort for second half:
var right = mergeSort(array.slice(middle));
// Combine both halves:
return merge(left, right);
}
When two halves are merged, the elements of each half are compared and arranged in order, forming a sorted list. This merging operation is performed iteratively until there is only one sorted array left.
Guidelines in Implementing Merge Sort Algorithm
When implementing Merge Sort, there are several guidelines to bear in mind. The right approach not only makes the task easier but also ensures efficient sorting.
Here’s a step-by-step guide to implement Merge Sort:
- Step 1: Identification of Base Case: Identify the base case to be when the array length is less than or equal to 1. If this is the case, return the array as it's already sorted.
- Step 2: Division into Halves: Find the middle of the array and divide it into two halves. The first half includes elements from the beginning of the array to the middle, while the second half consists of elements from the middle to the end.
- Step 3: Recurrence on Sub-arrays: Apply Merge Sort on both halves recursively. This brings us back to our base case (step 1), except now, it's applied on the divided halves of the original array. This recursive operation continues to divide the array until every sub-array contains only a single element.
- Step 4: Merging Sorted Sub-arrays: Merge the two halves that have been sorted separately. Comparison of elements is done on each half and they're arranged in order. This merging operation is repeated for all divided parts of the original array until one sorted array is obtained.
Let's look at a four-element array: \([5, 2, 4, 1]\). According to the Merge Sort guidelines:
- The base case is for an array with one element or fewer, which does not apply initially as the array has four elements. Hence, we proceed to the next step.
- We divide the data into two halves: the first half is \([5, 2]\) and the second half is \([4, 1]\).
- We recursively apply Merge Sort on both halves. The first half ([5, 2]) is divided into \([5]\) and \([2]\), and the second half ([4, 1]) into \([4]\) and \([1]\).
- Finally, having reached our base case, we start merging. We first merge [5] and [2] to get \([2, 5]\), and then [4] and [1] to obtain \([1, 4]\). Lastly, we merge the two halves \([1, 4]\) and \([2, 5]\) to get the fully sorted array \([1, 2, 4, 5]\).
Proper usage of Merge Sort requires understanding exactly how it divides and combines arrays to sort your data. Consequently, knowing the guidelines will allow you to effectively harness the power of this algorithm to handle complex sorting problems.
Merge Sort and Other Sorting Algorithms
Indeed, Merge Sort is renowned for its commendable performance in sorting large datasets. However, it's always insightful to understand where it stands compared to other popular sorting algorithms. In computer science, there exist several sorting algorithms, and each has its unique traits, advantages, and disadvantages. They include Bubble Sort, Insertion Sort, Selection Sort, Quick Sort, and Heap Sort, among many others.
Comparing Merge Sort to Other Algorithms
While Merge Sort upholds impressive performance, especially with large datasets, there's merit in comparing it with other sorting algorithms. Each algorithm carries distinct attributes, and hence, deducing the most suitable one heavily relies on the particular use-case.
- Insertion Sort: An intuitive algorithm that sorts an array by building a sorted array one item at a time. It works similarly to how you might sort playing cards in your hand. Although simple, Insertion Sort is quite inefficient for large datasets, with its worst-case time complexity of \(O(n^{2})\).
- Bubble Sort: Known for its simplicity but also its inefficiency, Bubble Sort repeatedly swaps adjacent elements if they are in the wrong order, resulting in larger elements 'bubbling' to the end of the list. It's not practical for large data due to a time complexity of \(O(n^{2})\).
- Quick Sort: An efficient, divide-and-conquer algorithm like Merge Sort, but it divides the array differently. Quick Sort selects a 'pivot' and partition the array around the pivot, then recursively sorts the partitions. While faster in practice, its worst-case time complexity can be \(O(n^{2})\), unlike Merge Sort's consistent \(O(n \log n)\).
- Heap Sort: Works by visualising the data structure as a binary heap. It starts by building a max heap and then swapping the root with the end node. Heap Sort restructures the heap and repeats the swapping process until the array is sorted. It shares the same time complexity as Merge Sort, \(O(n \log n)\), but is typically slower in practice.
Here's a comparative summary of these algorithms:
Algorithm | Best Case | Average Case | Worst Case | Stable |
---|---|---|---|---|
Merge Sort | \(O(n \log n)\) | \(O(n \log n)\) | \(O(n \log n)\) | Yes |
Insertion Sort | \(O(n)\) | \(O(n^{2})\) | \(O(n^{2})\) | Yes |
Bubble Sort | \(O(n)\) | \(O(n^{2})\) | \(O(n^{2})\) | Yes |
Quick Sort | \(O(n \log n)\) | \(O(n \log n)\) | \(O(n^{2})\) | No |
Heap Sort | \(O(n \log n)\) | \(O(n \log n)\) | \(O(n \log n)\) | No |
Ultimately, each sorting algorithm comes with its pros and cons. They differ in terms of performance, stability, space complexity, and usage simplicity. Hence, the selection of sorting algorithms largely relies on the nature of the problem, data type, size of data, and any pre-defined constraints.
Choosing the Right Sorting Algorithm
The choice of a sorting algorithm in any use-case depends on several factors like the size of the dataset, availability of system memory, and the need for stability in sorted output.
While some algorithms are tailor-made for specific data structures and volumes, others are more general-purpose, offering decent performance on a broader range of datasets. Here are some tips that may help in choosing the right sorting algorithm:
- Size of Data: For smaller datasets, simpler algorithms like Insertion Sort or Bubble Sort could suffice despite being inefficient for larger data. For extensive datasets, however, algorithms that exploit efficiency like Merge Sort or Quick Sort are significantly preferred.
- Nature of Data: When data are nearly sorted already, 'adaptive' algorithms like Insertion Sort can perform better. However, for completely random or worst-case scenarios, merge-based algorithms like Merge Sort prove remarkably resilient and efficient.
- Memory Restrictions: When memory is tight, it's advisable to opt for in-place algorithms which sort the data within the dataset itself, thus minimising additional space requirements. Heap Sort and Quick Sort are such examples. Merge Sort, conversely, is not space-efficient as it requires extra space to hold the divided data during the sorting process.
- Stability Requirement: If you need to maintain relative order in equal elements (stability), go for a stable algorithm like Merge Sort. Always keep in mind, not all sorting algorithms are stable.
Mindful consideration of the available sorting algorithms in accordance with the specific problems can result in sound and optimised decisions. After all, efficient sorting is a fundamental necessity which can heavily reflect on the performance of an entire system or application.
Practical Learning with Merge Sort
Learning about Merge Sort isn't just about understanding the theory behind it. It also requires a practical hands-on approach to fully grasp how this algorithm works. Taking a more interactive approach - working with examples, overcoming challenges, and trying different scenarios - strengthens your familiarity with the algorithm, making the learning experience both informative and enjoyable.
Interactive Learning: Step by Step Merge Sort Example
A practical and interactive approach to understanding Merge Sort starts with straightforward examples. It’s from these simple step-by-step examples that you can build on more complex scenarios. Let's walk through the sorting of a simple unsorted array using the Merge Sort algorithm.
For this example, consider the array \([38, 27, 43, 3, 9, 82, 10]\).
Consider the array above. With Merge Sort, the array is first divided consecutively into sub-arrays. The first level of division gives us two sub-arrays: \([38, 27, 43]\) and \([3, 9, 82, 10]\). At the second level of division, the first sub-array is divided into \([38]\) and \([27, 43]\), while the second sub-array splits into \([3, 9]\) and \([82, 10]\). The process continues until each sub-array contains only one element.
Once we've divided the array down to individual elements, we start merging them back up. It might seem like the array is back to square one, but that isn't the case! As sub-arrays are merged, their elements are compared and placed in increasing order. This is the essential step that sorts the array.
In the first level of merging, the sub-array \([38]\) merges with \([27, 43]\) to form \([27, 38, 43]\), and the sub-array \([3, 9]\) merges with \([82, 10]\) to form \([3, 9, 10, 82]\). In the second level of merging, these sorted sub-arrays are then merged to form a fully sorted array of \([3, 9, 10, 27, 38, 43, 82]\). With this, the Merge Sort process is complete!
Challenges faced in Implementing Merge Sort
Though Merge Sort is renowned for its efficiency, particularly with large data sets, it doesn't come without its share of challenges, especially when it comes to its implementation.
- Memory Usage: Since Merge Sort creates additional sub-arrays during the sorting process, it requires extra memory. This can be a significant drawback, especially in memory-restricted environments.
- Complex Algorithm: The divide and conquer approach, though efficient, is complex compared to basic algorithms like Bubble Sort and Insertion Sort. It requires understanding recursion and how sub-problems combine to solve the overall problem.
- Stability: While it's an advantage that Merge Sort is a stable algorithm, maintaining this stability requires careful programming. Not adhering to stability protocols can lead to instability in some circumstances.
Consider the challenge of the complex algorithm and recursion in Merge Sort. Understanding recursion, the idea of a function calling itself, could be quite challenging to beginners. Take the array \([38, 27, 43, 3, 9, 82, 10]\) from the previous example. The process of breaking down the array into sub-arrays, sorting them, and merging them is done recursively. So, having a sound understanding of recursion is crucial in understanding and implementing Merge Sort.
Thus, while implementing Merge Sort, it’s essential to be familiar with these challenges and ways to navigate them effectively. Despite these issues, once you get the hang of it, Merge Sort proves to be a powerful and reliable sorting algorithm!
Merge Sort - Key takeaways
Merge Sort is a comparison-based sorting algorithm known for its worst-case and average time complexity of O(n log n), where n is the length of the array. Applying the divide-and-conquer approach, it divides unsorted lists into simplest sub-problems to solve.
The process of Merge Sorting starts with dividing the initial unsorted array and further proceeds with merging smaller sorted lists into a larger sorted list until only one sorted array remains.
Time Complexity for Merge Sort: This refers to computational complexity that evaluates the computational time taken as a contributing factor to the size of the input. For Merge Sort, its worst-case time complexity is O(n log n), making it one of the most time-efficient sorting algorithms, especially for large datasets.
Best and Worst Case Scenarios: The best-case time complexity for Merge Sort is O(n log n), occurring when the input data is already sorted. The worst-case time complexity is also O(n log n), happening when the input data is in reverse order or when all elements are identical.
Advantages of Merge Sort: It is appreciated for its stability (maintaining the relative order of equal elements after sorting) and its reliable efficiency, especially when dealing with large datasets. However, its drawback is that it is not space-efficient as it requires additional space proportional to the size of the input data.
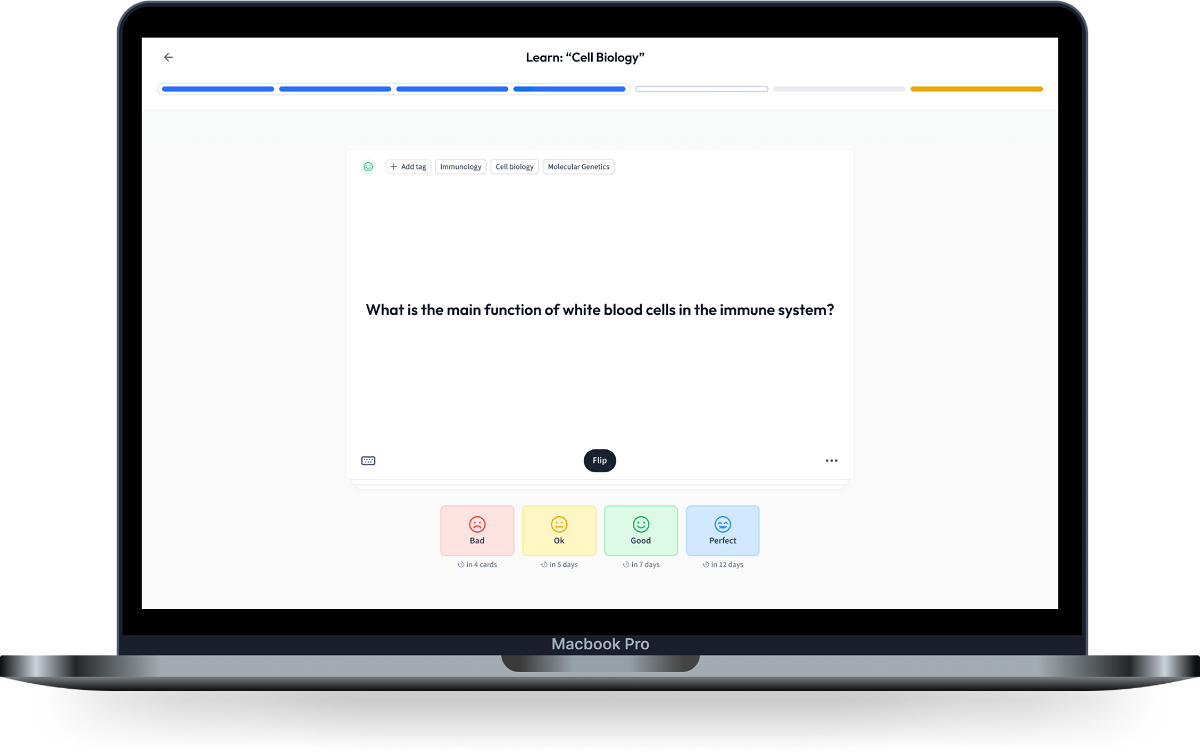
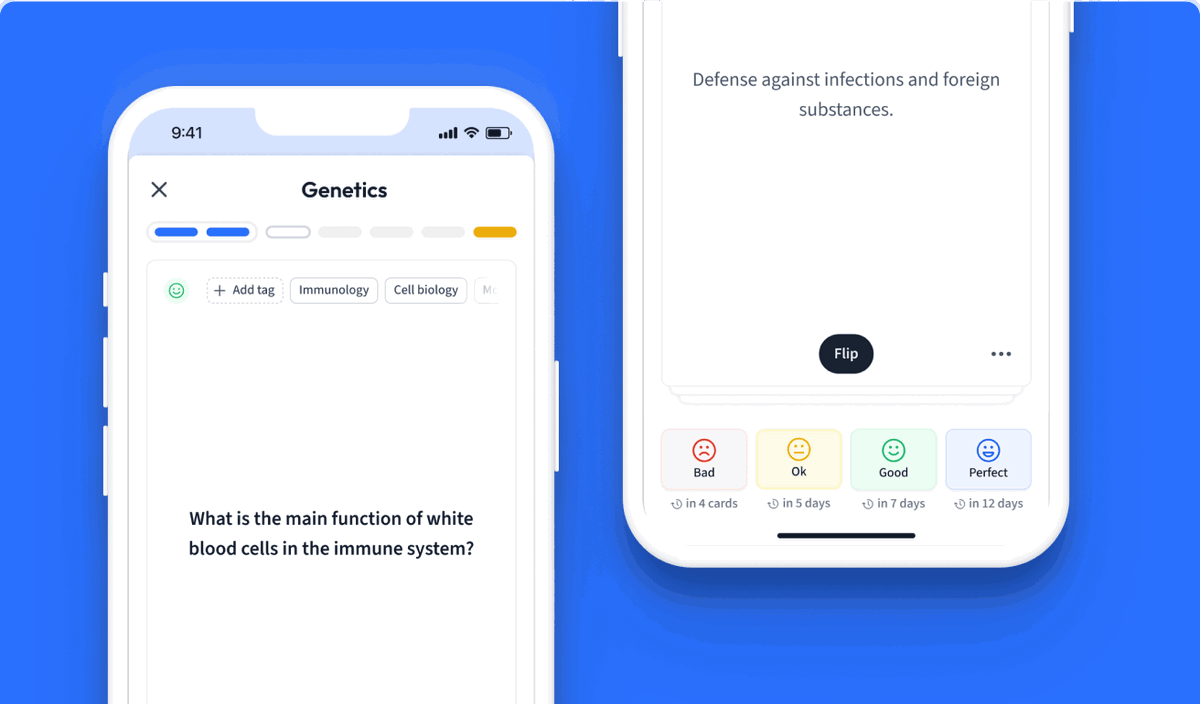
Learn with 18 Merge Sort flashcards in the free StudySmarter app
Already have an account? Log in
Frequently Asked Questions about Merge Sort
Is merge sort recursive?
Yes, merge sort is a recursive algorithm. It continually splits a list in half until it has subdivided the list down to its single elements. These elements are then merged back together in order, thus sorting the list. This splitting and merging process repeats recursively until the entire list is sorted.
What is a merge sort?
Merge sort is a type of sorting algorithm in computer science that follows the divide and conquer strategy. It works by recursively dividing the unsorted list into n sublists, each containing one element (which is considered sorted), and then repeatedly merging the sublists to produce new sorted sublists until there is only one sublist left. This final sublist is the sorted list. Merge sort is efficient for large datasets and provides stable sorting.
How does merge sort work?
Merge sort works by repeatedly dividing a list into two halves until it's broken down into individual elements. These individual elements are then repeatedly combined (or merged), while at the same time sorting them in a specified order. This combining and sorting process continues until all elements are merged back into a single sorted list. It's a divide and conquer algorithm that utilises recursion.
Is quick sort faster than merge sort?
In most cases, Quick Sort is faster than Merge Sort. Both have an average time complexity of O(n log n), but the constants in Quick Sort are typically smaller, leading to faster execution in practice. However, Quick Sort's worst-case performance can be far worse than Merge Sort's, which always guarantees a time complexity of O(n log n). Therefore, if worst-case scenarios are a concern, Merge Sort might be preferred.
How to do a merge sort?
Merge sort involves the following steps: 1) Dividing the unsorted list into n divisions, each subdivision having one element (a list of one element is considered sorted). 2) Repeatedly merge subdivisions to produce new sorted subdivisions until there is only one subdivision remaining. The final subdivision will result in a sorted list. This is implemented recursively in programming.
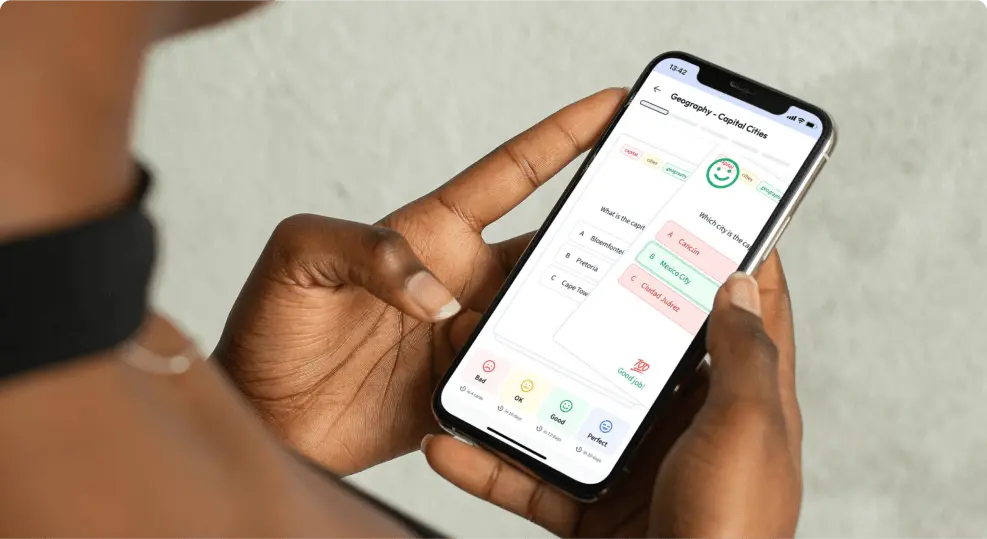
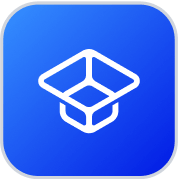
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more