Jump to a key chapter
Understanding Quick Sort
Deep dive into the realm of sorting algorithms through the lens of Quick Sort. Known for its efficiency and speed, Quick Sort is one of the most widely employed algorithms in computing and programming.
Basics of Quick Sort Algorithm
Quick Sort, also referred to as partition-exchange sort, is a popular sorting algorithm that employs a divide-and-conquer strategy, streamlining computation by breaking down the task into simpler, smaller tasks that are solved recursively.
A recursive algorithm is a method that breaks down complex problems into simpler tasks, and solves the original problem by recursively solving the simpler tasks.
- Quick Sort begins with selecting a 'pivot' from the array to be sorted.
- The pivot serves as the reference point and sorts all elements in the array; those less than or equal to the pivot go to its left and those greater to its right.
- This process effectively divides the array into two parts, each of which is then sorted by recursively applying the same algorithm.
Intrinsically, the efficiency of Quick Sort is attributed to its ability to perform well for larger lists and data sets. Moreover, it is faster than other sorting algorithms, such as Bubble Sort and Insertion Sort, particularly for extensive data sets.
Origin and Functions of Quick Sort
Quick Sort was developed by British computer scientist, Sir Charles Antony Richard Hoare, often known as Tony Hoare, in 1959. The algorithm soon became prominent in the domain of computer science for its ability to sort large data sets efficiently.
- Quick Sort is widely used in many fields, including search engines and databases where sorting large amounts of data is a common task.
- The algorithm is also used in scientific computing, specifically in tasks like simulation and modeling, genomics data processing, and much more.
Analysing Quick Sort Example
Let's examine an example of Quick Sort to better grasp its operation. Consider a simple array of five integers: {20, 5, 7, 25, 15}.
Let's choose the first element, 20, as the pivot. After partitioning, the elements remain in their original places since all elements are less than the pivot. The array now appears as {20, 5, 7, 25, 15}. The elements on the left and right sub-array of the pivot are then sorted recursively. The final sorted array is {5, 7, 15, 20, 25}.
The Time Complexity of the Quick Sort algorithm is best explained through formulae. The best and average case time complexity is \(O(n \log n)\), while the worst case is \(O(n^{2})\), where 'n' represents the number of items being sorted.
Time complexity refers to the computational complexity that measures or estimates the time taken by an algorithm to run as a function of the size of the input data.
Emphasising the relevance of time complexity in the Quick Sort algorithm accentuates the efficiency of this approach for sorting elements in an array. It aids in determining the qualitative measure of the running time of the algorithm.
Factors Influencing Quick Sort Time Complexity
Several factors inherently influence the time complexity of the Quick Sort algorithm, shaping its performance and efficiency in various scenarios.
- Choosing the Pivot: The choice of the pivot greatly affects the time complexity. If the pivot divides the array in a balanced manner, it results in optimal time complexity. In contrast, if the partitioning is unbalanced, the time complexity increases.
- Size of the Array: The size of the array is directly proportional to the time complexity. Larger arrays require more computations, increasing the time complexity.
- State of the Array: The initial state of the array (already sorted, reverse sorted, or randomly organised) can impact the time complexity. For an array that is already sorted or reverse sorted, Quick Sort operates at worst-case time complexity.
To optimise the performance of Quick Sort, it is often desirable to utilise a hybrid approach. For instance, when the data set level reduces to a certain level during partitioning, it may be beneficial to use simpler sorting algorithms such as insertion sort, which can outperform Quick Sort for smaller lists.
Best, Worst and Average Case Scenarios
Scenario | Time Complexity | Remarks |
---|---|---|
Best Case | \(O(n \log n)\) | The scenario occurs when the pivot divides the array into two nearly equal halves, leading to well-balanced partitioning. |
Average Case | \(O(n \log n)\) | This scenario considers all possible cases and calculates the average time complexity. Moreover, it's assumed that all permutations of array element orders are equally likely. |
Worst Case | \(O(n^{2})\) | The worst-case scenario occurs when the smallest or largest element is chosen as a pivot. In this case, the partitioning is heavily unbalanced, leading to significantly higher time complexity. |
Here, 'n' is the total number of elements in the array to be sorted. The time complexity expressions used above, \( O(n \log n) \) and \( O(n^{2}) \), are mathematical notations that describe the limiting behaviour of a function when the argument (here 'n') tends towards a particular value or infinity. 'O' is a part of Big O notation, which is the most widely known notation used for analysing algorithm's efficiency.
For instance, suppose an array of size 10,000 is to be sorted using Quick Sort. If due to an unfortunate pivot selection, every partition chooses the worst-case scenario (heavily unbalanced partitioning), the complexity will be \(O(n^{2})\), resulting in around 100 million operations! This example lays bare how algorithm efficiency can drastically vary due to the influence of both input data and pivot selection.
Quick Sort vs Merge Sort
When delving deeper into sorting algorithms, two prominent names that pop up are Quick Sort and Merge Sort. Though they both apply the divide-and-conquer methodology, there are inherent differences in how they segment and sort the arrays. Understanding how each operates and their relative efficiencies are pivotal in choosing the right algorithm for a specific task.
Core Differences between Quick Sort and Merge Sort
While both Quick Sort and Merge Sort are comparative sorting algorithms based on the divide-and-conquer technique, they do differ significantly in terms of partitioning strategy, stability, and worst-case time complexity.
- Partitioning Strategy: Quick Sort partitions the array into two parts such that elements of the left partition are less than those of the right partition. Merge Sort, on the other hand, divides the array into two halves, sorts them separately and merges them.
- Stability: Stability is a factor where the original order of equal elements is preserved after sorting. Merge Sort is a stable sorting algorithm, whereas Quick Sort is not. This can be crucial when dealing with equal sort keys with distinctive data.
- Worst-case Time Complexity: Quick Sort has a worst-case time complexity of \(O(n^{2})\) compared to Merge Sort's \(O(n \log n)\), making Merge Sort more consistent in its performance.
Sorting Algorithm | Partitioning Strategy | Stability | Worst-case Time Complexity |
---|---|---|---|
Quick Sort | Partitions array such that elements of left partition are less than those of the right partition. | Not Stable | \(O(n^{2})\) |
Merge Sort | Divides array into two halves, sorts them separately and merges them. | Stable | \(O(n \log n)\) |
Efficiency of Quick Sort versus Merge Sort
The efficiency of a sorting algorithm is typically assessed based on its time complexity and space requirements. While Quick Sort is generally faster on average, both algorithms have their strengths and weaknesses in different scenarios.
- Average-Case Time Complexity: Both Quick Sort and Merge Sort have an average case time complexity of \(O(n \log n)\), but the constant factors in Quick Sort are smaller than Merge Sort, making it faster for large arrays, especially when optimization techniques are applied.
- Space Complexity: Merge Sort requires additional space equivalent to the array size during the merge process (\(O(n)\) in the worst-case scenario), while Quick Sort operates in-place and requires only a small extra space (\(O(\log n)\) in the worst-case scenario).
- Worst-Case Scenario: The worst-case running time for Quick Sort (when the input is already sorted or reverse sorted) is \(O(n^{2})\). However, this can be mostly avoided by using randomized Quick Sort. Merge Sort guarantees a consistent \(O(n \log n)\) running time regardless of the input.
Let's take an example of having an array with 100,000 records. If all values are distinct and random, Quick Sort, with an optimized choice of pivot, tends to outperform Merge Sort, thanks to its lower coefficient in time complexity. However, if the array contains many duplicates or it's already sorted, Quick Sort will downgrade to \(O(n^{2})\) if a bad pivot is chosen. Meanwhile, Merge Sort still ensures a good performance with \(O(n \log n)\) running time, offering more predictability.
This comparison highlights how the efficiency of either sort depends heavily upon the nature of the input dataset and specific task requirements. It underscores the importance of understanding your dataset and the nuances of different algorithms before choosing the right one. There's no one-size-fits-all in sorting algorithms, and the efficiency of one algorithm over another is greatly governed by the specific nature and volume of data being dealt with.
Evaluating Quick Sort
Understanding the effectiveness and efficiency of an algorithm is crucial to determine whether it's the right choice for a given task. This section focuses on evaluating the Quick Sort algorithm, examining its advantages and disadvantages in depth.Advantages of Quick Sort
Quick Sort, like other sorting techniques, has its own set of benefits that can impact computational tasks. Here are some key advantages of Quick Sort.- Speed: On average, Quick Sort is one of the fastest sorting algorithms for large data sets, with a time complexity of \(O(n \log n)\), which makes it more efficient than many other algorithms like Bubble Sort or Insertion Sort.
- In-Place Sort: Quick Sort is an in-place sorting algorithm. This indicates that it hasn't to create any additional arrays while sorting, thus saving memory. It only needs a small stack space of \(O(\log n)\) to manage recursion calls.
- Cache Performance: Due to being an in-place kind of algorithm, Quick Sort deals nicely with modern computer architectures, where memory access times are not constant and can greatly affect the speed of an algorithm.
- Universally Applicable: Quick Sort works effectively on both arrays and linked lists. It can sort any kind of data including numbers, characters, strings, or custom data types.
- Scalability: Quick Sort, with its efficient time complexity, is conducive to sorting larger lists or data sets, making it beneficial for operations on large-scale data.
Why You Should Use Quick Sort
In a control system or large data operation, if you're looking for a sorting algorithm that offers optimal time complexity, saves space by handling sorting in-place, and handles cache better, then Quick Sort is the optimal choice for you. For example, imagine having a list of several million entries that you need to sort. Quick Sort fits nicely into this scenario, because of its efficiency and speed. Furthermore, its ability to handle a variety of data types increases its applicability horizons. You can use this versatile sorting method to sort numbers, text characters, or even custom data types, enhancing its adaptability in numerous scenarios.Disadvantages of Quick Sort
However, like any algorithm, Quick Sort likewise exhibits a few drawbacks that are important to consider while deciding on its application for a specific task.- Worst-Case Time Complexity: In the worst-case scenario, which occurs when the smallest or largest element is chosen as the pivot, Quick Sort shows a time complexity of \(O(n^{2})\), which is undesirable for larger arrays.
- Pivot Selection: The efficiency of Quick Sort heavily depends on the selection of the pivot element. Poor choice of pivot can lead to imbalanced partitions causing the algorithm to degrade to quadratic performance. However, this can be mitigated by using techniques like randomized Quick Sort.
- Not Stable: Quick Sort is not a stable sort. In case of equal elements, the original order might not be maintained. This is especially crucial when dealing with equal sort keys with different data.
Potential Hurdles with Quick Sort
The main hurdle with Quick Sort is its worst-case time complexity. Sorting an already sorted list or a list that is in reverse order may lead the algorithm down its worst-case scenario path, reducing the overall efficiency of the algorithm. Moreover, the instability of Quick Sort can be a significant factor to consider, especially when working with data sets where the relative order of the same key values matters. Even the pivot point selection issue is of concern, as an unfortunate selection of the pivot element can lead to degrading the performance of Quick Sort. The choice of pivot has a huge effect on the efficiency of the algorithm. For instance, if the last element is chosen as pivot and the list is already sorted in increasing or decreasing order, the partition process leads to unbalanced sub-arrays, causing an increase in time complexity. To mitigate these potential hurdles, hybrid versions of Quick Sort or optimised pivot selection techniques - such as Median of Medians or choosing a random pivot could be used to optimise performance and tackle these challenges effectively. Remember, optimal performance is rarely achieved by chance, but it’s born out of knowledge of the algorithm's strengths and weaknesses, careful planning and smart implementation.Quick Sort - Key takeaways
Quick Sort is a popular sorting algorithm known for its efficiency and speed, using a divide-and-conquer strategy or 'partition-exchange'.
A recursive algorithm breaks down complex problems into simpler tasks and solves the original problem by recursively solving simpler tasks.
Key aspects of Quick Sort include selecting a 'pivot' and sorting all elements against it, dividing the array into two parts and sorting each recursively.
The Quick Sort algorithm was developed by British computer scientist, Sir Charles Antony Richard Hoare in 1959, and is used in multiple fields such as search engines, databases, and scientific computing.
Time complexity measures the time taken by an algorithm to run as a function of the size of the input data. In Quick Sort, the best and average case time complexity is \(O(n \log n)\), and the worst case is \(O(n^{2})\).
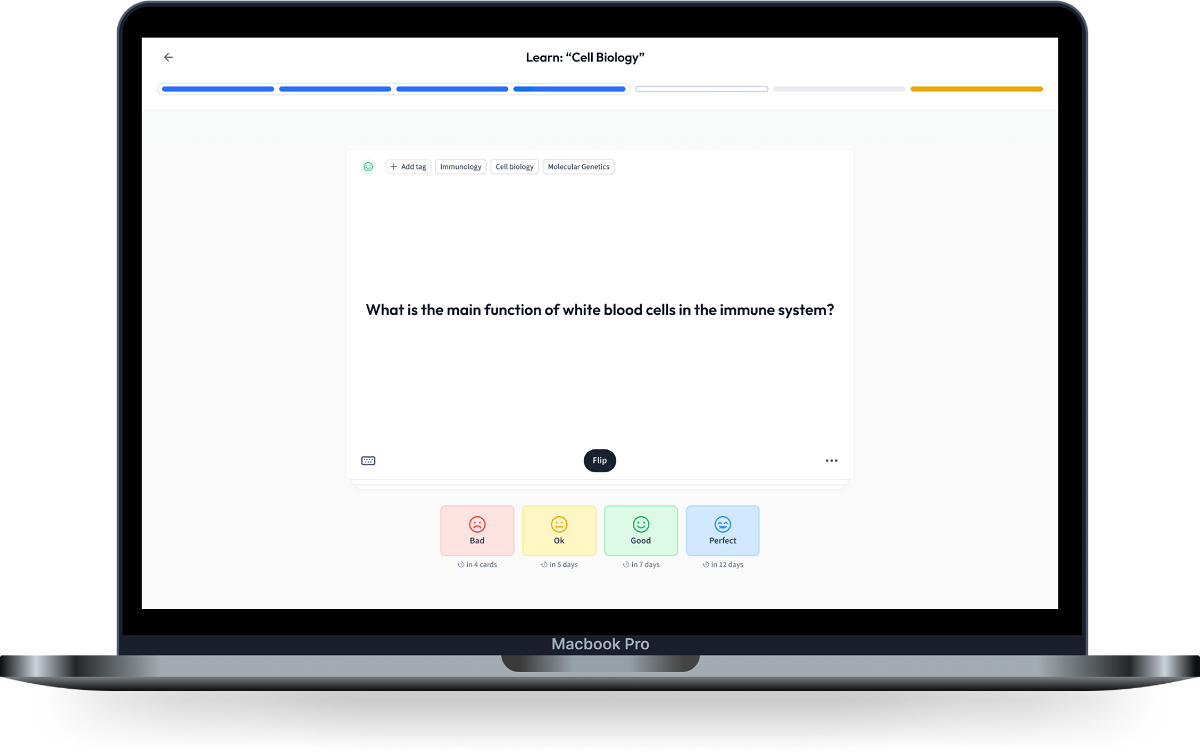
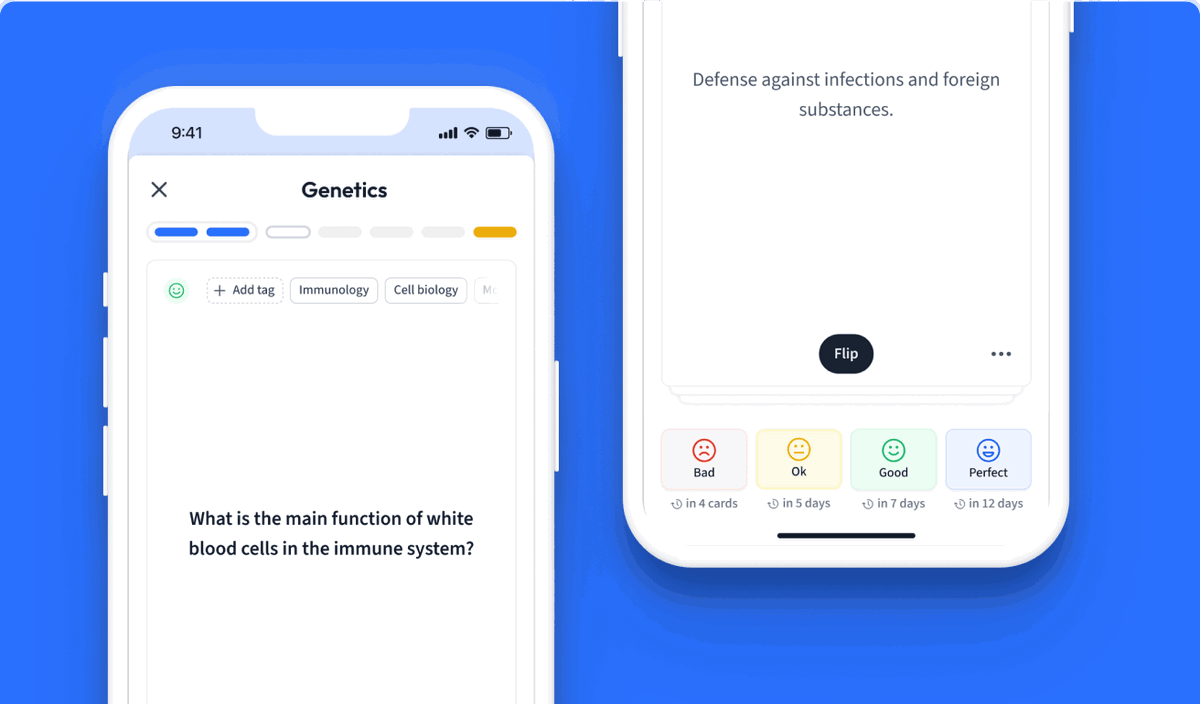
Learn with 16 Quick Sort flashcards in the free StudySmarter app
Already have an account? Log in
Frequently Asked Questions about Quick Sort
How does quick sort work?
Quick sort operates by selecting a 'pivot' element from the array and partitioning the other elements into two sub-arrays, according to whether they are less than or greater than the pivot. The sub-arrays are then recursively sorted. This process repeats until the entire array is sorted. Thus, Quick Sort separates the data into smaller arrays and sort them individually to achieve a completely sorted array.
What is a quick sort?
Quick sort is a highly efficient sorting algorithm often used to order large datasets. It operates on the divide and conquer principle by selecting a 'pivot' element from the array and partitioning the other elements into two sub-arrays, according to whether they are less than or greater than the pivot. The algorithm then recursively sorts the sub-arrays. Despite its worst-case time complexity being O(n^2), it's generally considered faster due to its in-place memory usage and average time complexity of O(n log n).
Is quick sort faster than merge sort?
In general, quick sort and merge sort have the same average and worst-case time complexity which is O(n log n). However, quick sort tends to be faster in practice as it has smaller hidden constants. It also has better locality of reference and requires less space than merge sort. However, quicksort's performance can heavily depend on the choice of pivot.
How to implement quick sort?
To implement quicksort, first choose a "pivot" element from the array and partition the other elements into two sub-arrays, according to whether they are less than or greater than the pivot. The pivot element is then in its final position. Next, recursively apply the same steps to the sub-arrays. Each recursion ends when the sub-array has just one element, at which point it is sorted.
What is quick sort algorithm?
The quick sort algorithm is a highly efficient sorting method used in computer science. It works by selecting a 'pivot' element from an array and partitioning the other elements into two sub-arrays, according to whether they are less than or greater than the pivot. The algorithm repeats this process on the sub-arrays until it can no longer be divided. Hence, a collection is sorted by a system of continuous division.
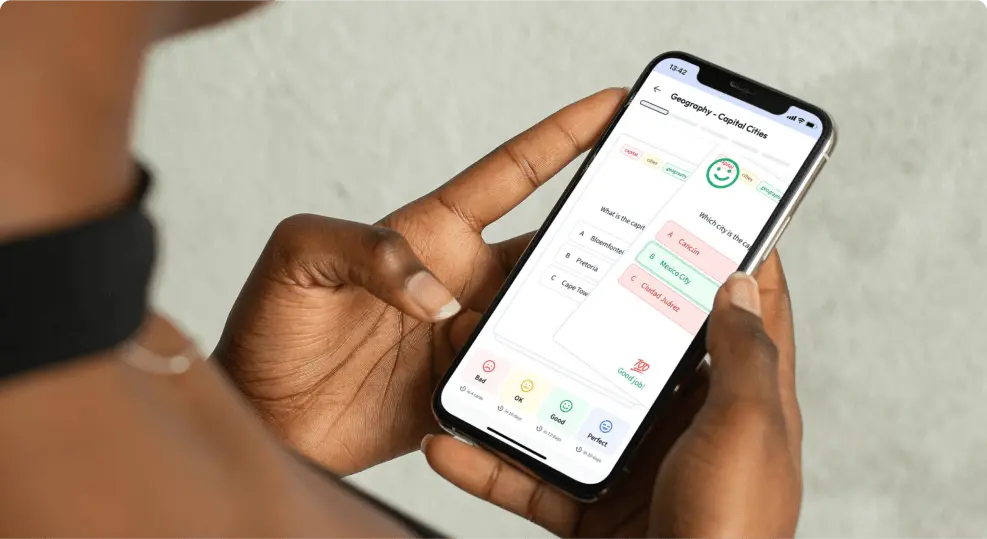
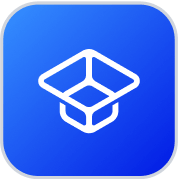
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more