Jump to a key chapter
Overview of Radix Sort in Computer Science
Radix sort is an intriguing algorithm in Computer Science that belongs to the bucket sort family of algorithms. It's unique and highly effective in certain scenarios because it doesn't involve comparison of values, unlike many of the most frequently used sorting methods. Instead, Radix Sort, often used for sorting large integers or strings of letters, utilises the mathematical concept of radix or base.What's a radix? In mathematics, the radix or base is the number of unique digits, including zero, used to represent numbers in a positional numeral system. For example, for the decimal system the radix is 10, because it uses 10 digits from 0 to 9.
Understand the Radix Sort Algorithm
The Radix Sort algorithm operates under a straightforward principle: sort numbers or letters sequentially from the least significant digit to the most significant. Remember this concept? A number like 123 has 3 as its least significant digit and 1 as its most significant. Letters in words can also be considered significant in an alphabetic order.Radix sort has been around since the invention of punch-card machines and has been utilised in early electronic computers like the IBM 701. It's an age-old algorithm with impressive staying power!
- For each digit position, starting from least significant digit and moving to most: - Distribute all values amongst buckets according to their digit value. - Recombine the values, maintaining their order within each bucket.It's essential to note that Radix sort can only handle positive integers and needs to be modified to sort negative integers or floating-point numbers.
How Does Radix Sort Work in Practice?
In practice, the Radix Sort algorithm works in two main modes: Least Significant Digit (LSD) and Most Significant Digit (MSD). The LSD starts scanning from the least significant digit towards the more significant, while the MSD algorithm works the other way around. While these methods may seem similar, their real-life applications can be quite different. For instance: - The LSD method is used when the length of the key values in the dataset is small. - The MSD method is useful in cases where the more significant part of the key is more likely to affect the sorted order, like in string sorting.Example of Radix Sort in Action
Radix Sort in action can invariably be best illustrated through an example. Consider sorting the following sequence of 3-digit numbers: [170, 45, 75, 90, 802, 24, 2, 66].
First Pass (Sort by least significant digit): - Bucket 0: { 170, 90, 802 } - Bucket 1: {} - Bucket 2: { 2 } - Bucket 3: {} - Bucket 4: { 24 } - Bucket 5: { 45 } - Bucket 6: { 75, 66 } The array after the first pass: { 170, 90, 802, 2, 24, 45, 75, 66 } Second Pass (Sort by second digit): - Bucket 0: { 802, 2 } - Bucket 1: {} - Bucket 2: {} - Bucket 3: {} - Bucket 4: { 24 } - Bucket 5: { 75 } - Bucket 6: { 66 } - Bucket 7: { 170 } - Bucket 9: { 90 } The array after the second pass: { 802, 2, 24, 75, 66, 170, 90 } Third Pass (Sort by most significant digit): - Bucket 0: { 2 } - Bucket 2: { 24 } - Bucket 6: { 66 } - Bucket 7: { 75 } - Bucket 8: { 802 } - Bucket 1: { 170 } - Bucket 9: { 90 } Final Sorted Array: { 2, 24, 66, 75, 90, 170, 802 }
The Technicalities behind Radix Sort
The strength and uniqueness of the Radix Sort algorithm lie in its underlying principle, which adopts a non-comparative approach to sorting. Indeed, the nitty-gritty of its application revolves around send-ahead computations, distribution and collection of elements, and the mathematical construct of radix itself. To grasp the entirety of this sorting technique, it's crucial to delve into its time complexity and identify its stability in sorting operations.Exploring Radix Sort Time Complexity
The time complexity of an algorithm quantifies the amount of time taken by an algorithm to run as a function of the length of the input. In the case of the Radix sort, you'll find that its time complexity is an interesting subject for exploration. Radix sort isn't compared based; unlike quick sort or merge sort. Instead, they fall into the category of integer sorting algorithms. As such, their time complexity doesn't follow the typical \(O(n \log n)\) that many common comparison-based sorting algorithms do. With careful observation, you'll realise that the time complexity of Radix Sort is \(O(nk)\), where: - \(n\) is the number of elements in the input array - \(k\) is the digit length of the number In terms of space complexity, Radix sort requires additional space for the output array and the counting array. As such, it has a space complexity of \(O(n+k)\). However, it is important to note that Radix Sort's efficiency drastically reduces when the range of the input data is significantly greater than the number of data points.Is Radix Sort Stable or Unstable?
Another factor that you'll need to consider when learning about sorting algorithms is whether they're stable or unstable. Stability in sorting algorithms is the preservation of the relative order of equal sort keys in the sorted output. This property can be essential in certain applications. Let's make this clearer with an example. If you've got consistent equal items A and B in an array, where A appears before B, a stable sort will always ensure A remains before B in the sorted array as well. Luckily, Radix sort falls into the category of stable sorting algorithms. This stability is guaranteed because Radix sort examines each digit from right to left (or least significant to most significant), and during each stage, elements with the same significant place value are kept in their original order. Therefore, elements with the same value remain in their initial occurrence order throughout the sorting process. The stability of the Radix sort brings about a unique benefit where you can sort by one attribute, and then sort by another attribute while keeping the relative order of the first attribute. It's amazingly useful in certain data handling implementations.Overall, in your route to becoming a Computer Science savant, understanding the technicalities of each algorithm, such as Radix Sort, will equip you with the necessary insight to implement the right algorithms in relevant scenarios. And remember, knowing the stability and time complexity of algorithms is an integral part of this overall comprehension.
Radix Sort Vs. Quick Sort
When it comes to sorting algorithms, Radix Sort and Quick Sort stand out as two significant methods applied in a range of computational scenarios. However, they possess contrasting characteristics and varying levels of efficiency based on the nature of the data being sorted. The comparison becomes imperative to understand these sorting algorithms' operational integrity, efficiency, and their pros and cons.Comparing the Pros and Cons of Radix Sort and Quick Sort
Radix Sort and Quick Sort exhibit distinct advantages and disadvantages that can influence their implementation in different scenarios. Here's a detailed look at some of their pros and cons: Radix Sort:- Pros:
- It has a linear time complexity, which means it's faster than comparison-based methods for longer lists when the range isn't massive.
- This algorithm is stable, maintaining the relative order of equal sort keys.
- It can sort items with multiple key types, i.e., not just integers.
- Cons:
- Radix Sort becomes less efficient when the range of the input data exceeds the number of data points.
- It requires more space compared to in-place sort algorithms like Quick Sort; hence, it's not as space-efficient.
- Modifications are necessary for it to handle floating-point numbers, negative integers, and strings.
- Pros:
- Quick Sort is universally versatile and can handle any types of data.
- It can be easily implemented and used in various programming languages.
- It can be optimised to sort elements in-place, which makes it space-efficient.
- Cons:
- The worst-case performance of Quick Sort (\(O(n^2)\)) can be quite poor, particularly for already sorted or nearly sorted input data.
- It is an unstable sort, the original order of equal keys is not preserved.
- A mediocre pivot selection can drastically reduce its efficiency.
Operational Differences between Radix Sort and Quick Sort
Operationally, Radix Sort and Quick Sort stand at opposite ends of the sorting spectrum. The differentiation in their operational approaches is fundamental to understand their intricacy and application. Radix Sort: As previously explained, Radix Sort operates by sorting integers digit by digit from least significant digit to the most. The sorted result is then placed into buckets, corresponding to each radix (base). Each pass of the sorting process corresponds to a digit position, starting from the least significant digit. It's a non-comparative sorting algorithm, which means that comparison of key elements isn't part of its operational process. Quick Sort: On the other hand, Quick Sort is a divide-and-conquer algorithm that applies a different operational mechanism. First, it picks an element from the array to serve as a pivot. It then partitions the rest of the array into two sections - elements less than or equal to the pivot and elements greater than the pivot. This process is recursively applied to each subsection until the entire array is sorted. It's worth noting that Quick Sort is a comparative sorting algorithm, which fundamentally separates its operational mechanics from Radix Sort.Example of Quick Sort operation: Consider an unsorted array: [9, 7, 5, 11, 12, 2, 14, 3, 10, 6] 1. Take the first element as pivot: 9 2. Partition around the pivot: [7, 5, 2, 3, 6, 9, 11, 12, 14, 10] - Left of pivot: [7, 5, 2, 3, 6] - Right of pivot: [11, 12, 14, 10] 3. Apply Quick sort recursively to both partitions Final Sorted Array: [2, 3, 5, 6, 7, 9, 10, 11, 12, 14]These clear operational differences between Radix Sort and Quick Sort demonstrate how vastly different sorting techniques can be utilised to achieve the same end goal - sorting a list in a particular order. Depending on the sorting tasks' specifics and requirements, software engineers might prefer one sorting algorithm over the other.
Radix Sort Explained Through Practical Examples
Learning new concepts often gets easier with some practical illustration. Let's thus follow some step-by-step examples to boost your understanding of the Radix Sort algorithm.Step-by-Step Radix Sort Example
Surely, not many things could teach you better about Radix Sort than a detailed, step-by-step example. To make this as straightforward as possible, let's start with a simple array: [121, 432, 564, 23, 1, 45, 788]. We'll use the LSD (Least Significant Digit) method, starting with sorting the least significant digits first. The process to sort the above array using Radix Sort is as follows: First Pass (Sort by the least significant digit):- Bucket 0: { } - Bucket 1: { 121, 1 } - Bucket 2: { 432 } - Bucket 3: { 23 } - Bucket 4: { 564 } - Bucket 5: { 45 } - Bucket 6: { } - Bucket 7: { } - Bucket 8: { 788 } - Bucket 9: { } The array after the first pass: { 121, 1, 432, 23, 564, 45, 788 }Second Pass (Sort by the second digit):
- Bucket 0: { 1 } - Bucket 1: { } - Bucket 2: { 121, 23 } - Bucket 3: { 432, 564 } - Bucket 4: { 45 } - Bucket 5: { } - Bucket 6: { } - Bucket 7: { 788 } - Bucket 8: { } - Bucket 9: { } The array after the second pass: { 1, 121, 23, 432, 564, 45, 788 }Third pass (Sort by the most significant digit):
- Bucket 0: { 1, 23, 45 } - Bucket 1: { 121 } - Bucket 2: { } - Bucket 3: { } - Bucket 4: { 432 } - Bucket 5: { 564 } - Bucket 6: { } - Bucket 7: { 788 } - Bucket 8: { } - Bucket 9: { } The final sorted array: { 1, 23, 45, 121, 432, 564, 788 }Each pass of the radix sort algorithm distributes the items into buckets based on the digit being considered and then collects the items preserving the order of the buckets.
Another Example: Radix Sort in Real-World Cases
In the real world, Radix Sort isn't restricted to only numerical values. Its capability extends to sorting strings as well, especially those with the same length. As a quick illustration, let's sort characters using the Radix Sort algorithm. Consider the following array of three-letter words: ['car', 'dog', 'cat', 'ant', 'cow', 'cut', 'arc'].Initial Array: { 'car', 'dog', 'cat', 'ant', 'cow', 'cut', 'arc' } The following steps summarise the Radix Sort process: First Pass (Sort by the First Letter): - Bucket 'a': { 'ant', 'arc' } - Bucket 'c': { 'car', 'cat', 'cow', 'cut' } - Bucket 'd': { 'dog' } - Bucket 'b' to 'z': { } The array after the first pass: { 'ant', 'arc', 'car', 'cat', 'cow', 'cut', 'dog' } Second Pass (Sort by the Second Letter): - Bucket 'a': { 'car', 'cat' } - Bucket 'n': { 'ant' } - Bucket 'o': { 'dog', 'cow' } - Bucket 'r': { 'arc' } - Bucket 'u': { 'cut' } - Bucket 'b' to 'z': { } The array after the second pass: { 'car', 'cat', 'ant', 'dog', 'cow', 'arc', 'cut' } Third Pass (Sort by the Third Letter): - Bucket 'a': { 'car', 'cat' } - Bucket 'g': { 'dog' } - Bucket 'n': { 'ant' } - Bucket 'r': { 'car' } - Bucket 't': { 'cat', 'cut' } - Bucket 'w': { 'cow' } - Bucket 'b' to 'z': { } Final Sorted Array: { 'ant', 'arc', 'car', 'cat', 'cow', 'cut', 'dog' }Sorting strings using Radix Sort follows the same principle of distributing and collecting items based on the significant digit, but in this case, the significant digit is a character. It's why Radix Sort, while sounding mathematical with its name, also functions brilliantly with alphanumeric values, showcasing its flexibility in sorting implementations.
Advantages and Disadvantages of Using Radix Sort
Every sorting algorithm inherently presents a unique symphony of trade-offs – a blend of advantages and disadvantages. Radix Sort is a perfect illustration of this symphonic symphony. Effectively judging when to use Radix Sort and when not to will invariably depend on the detailed understanding of these trade-offs, and how they align with the specific use case at hand. This section presents an informative discourse highlighting the significant advantages and potential disadvantages pertaining to the Radix Sort algorithm.Significant Advantages of Radix Sort
Radix Sort, despite being one of the less common sorting algorithms, delivers a number of noteworthy advantages that can make it an ideal choice in the right circumstances. Linear Time Complexity: Radix Sort boasts of a linear time complexity \(O(nk)\), where \(n\) is the number of elements and \(k\) is the number of digits in the largest number. This is notably more efficient than comparison-based sorting algorithms which have an average and worst-case time complexity of \(O(n \log n)\). This means that in situations where \(k\) is relatively small, Radix Sort can perform exceptionally well. Stability: Radix Sort is a stable sort. Stability in sorting algorithms implies that equal keys stay in their original order, which can significantly impact certain applications where consistency of similar elements is crucial. Non-comparative: Radix Sort is a non-comparative sorting algorithm. While comparative sorting algorithms compare elements to determine their order, Radix Sort instead groups elements based on their individual digits or letters position. This makes Radix Sort a convenient choice when dealing with various data types and formats, as comparisons can often be computational expensive or not feasible.Potential Disadvantages of Radix Sort
Despite its significant advantages, Radix Sort is not a Swiss army knife of sorting that is ideal for all scenarios. It presents a few drawbacks, which, under certain conditions, may lead to inefficiencies or complicacies. Restricted Input: Radix Sort algorithm is specifically tailored to handle integers or strings. It does not natively support other data types and requires modification to accommodate floating-point numbers, negative integers, or strings of variable lengths. Moreover, if the range of the input data significantly exceeds the size of the input, Radix Sort loses its linchpin advantage of linear time complexity. Space Inefficiency: Radix Sort requires additional space to perform its operations. It isn't an in-place sorting algorithm as it requires extra space for output, making it less space-efficient than in-place sorting algorithms like Quick Sort or Insertion Sort. Sequential Processing: Unlike some other sorting algorithms, Radix Sort's operations are harder to parallelise due to its sequential processing. In environments where parallel processing is beneficial or resources are abundant, this could be considered a disadvantage. Understanding these advantages and corresponding disadvantages is key to utilising Radix Sort astutely. It isn't ideally suited for all circumstances but can serve as a powerful tool in the right settings, guiding you to make informed decisions while handling large data and complex programming applications.Radix Sort - Key takeaways
- Radix Sort is a non-comparative algorithm that uses a unique method of sorting where the values are distributed into buckets and collected in order of significance, going from the least significant digit towards the more significant one.
- The time complexity of Radix sort is \(O(nk)\), where \(n\) represents the number of elements in the input array and \(k\) is the digit length of the number, making it a potential candidate for efficient sorting with the right datasets.
- Radix Sort is identified as a stable sorting algorithm as it preserves the initial occurrence order of elements with the same value throughout the sorting process.
- An example of Radix sort in action is demonstrated, which involves sorting of the sequence [170, 45, 75, 90, 802, 24, 2, 66] via multiple passes, each corresponding to a digit position starting from the least significant digit.
- Both Radix Sort and Quick Sort offer various advantages but also possess distinctive disadvantages, including the fact that while Radix Sort can maintain the relative order of equal sort keys, it becomes less efficient if the range of input data is greater than the number of data points.
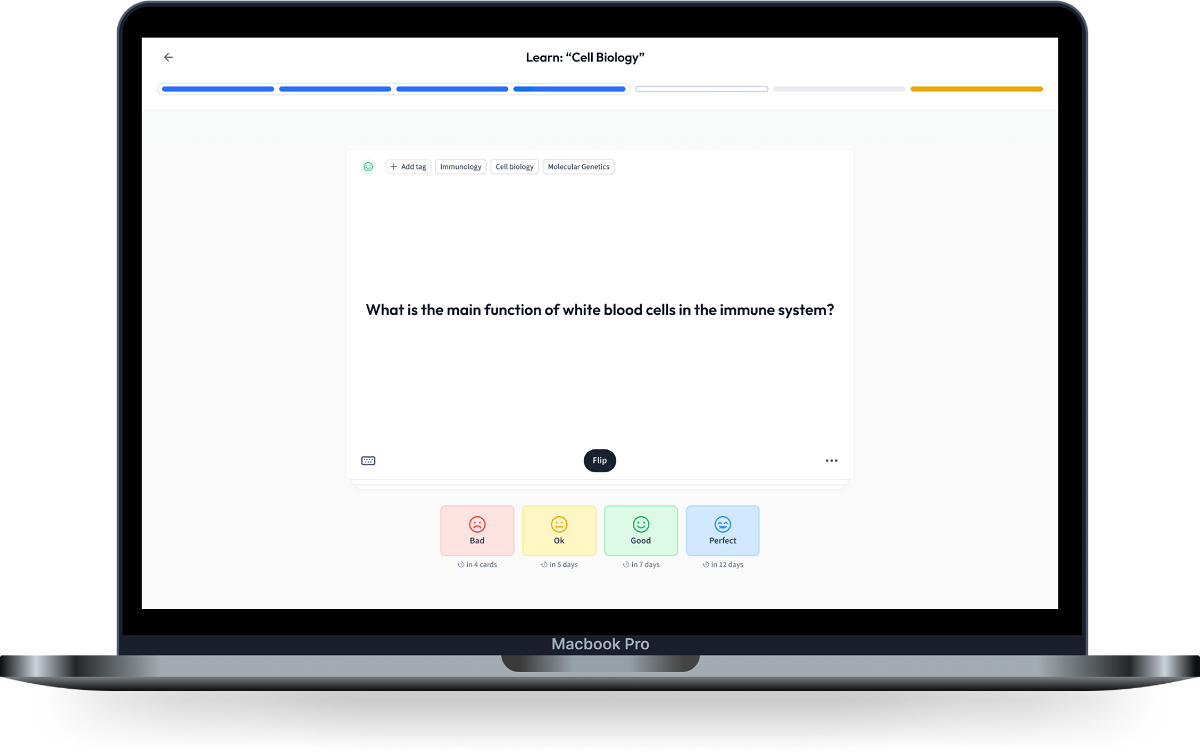
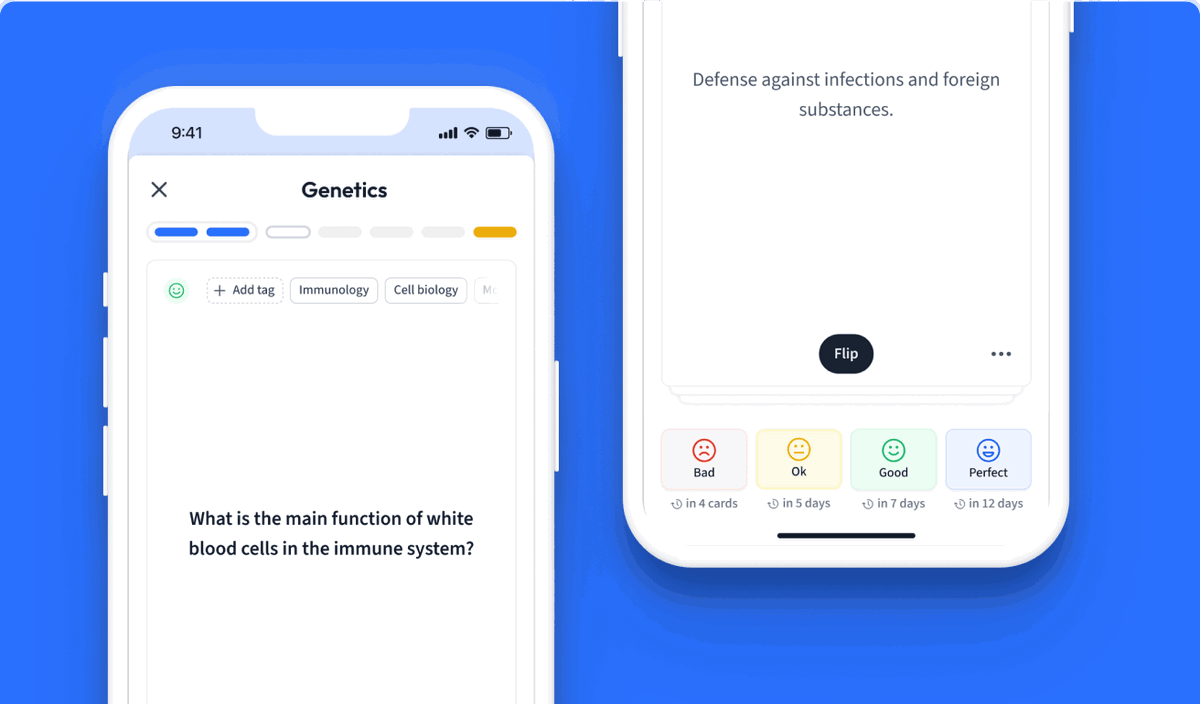
Learn with 15 Radix Sort flashcards in the free StudySmarter app
Already have an account? Log in
Frequently Asked Questions about Radix Sort
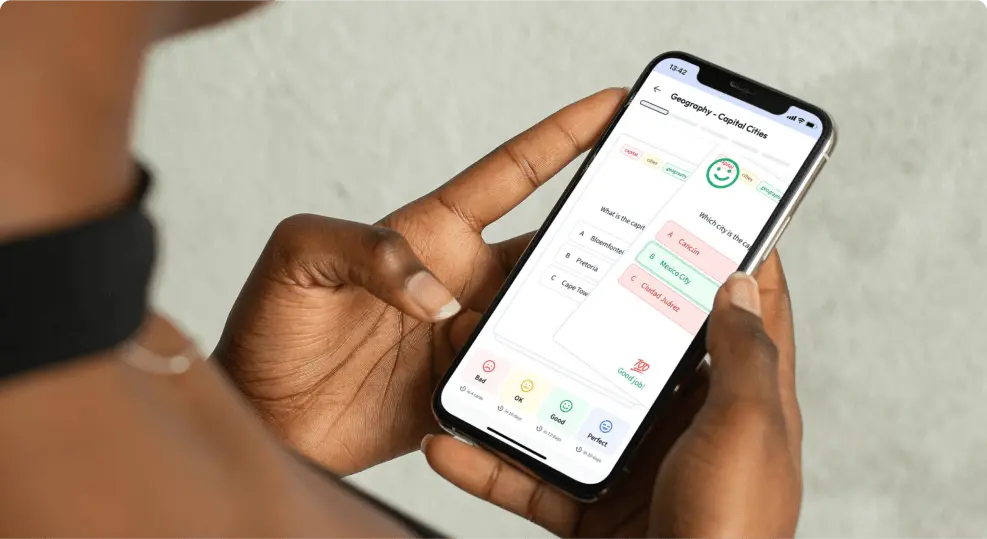
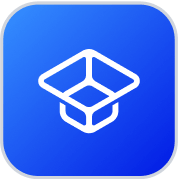
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more