Jump to a key chapter
Understanding jQuery Fundamentals
jQuery Tutorial for Beginners
jQuery is a fast, small, and feature-rich JavaScript library. It simplifies HTML document traversal and manipulation, event handling, and animation, making it easier for you to work with web pages.To get started with jQuery, it's essential to include it in your HTML. Typically, this is done by linking to a CDN or downloading the library directly. Here's an example of how to include it:
With jQuery loaded, you can start using it in your scripts. Common tasks that jQuery can handle include:- Document handling
- Event handling
- AJAX requests
- Animation and effects
Key jQuery Selectors Explained
Selectors are fundamental in jQuery as they allow you to target and work with elements on the page.The most common types of selectors include:
- Element Selector: Selects elements by their tag name (e.g., $('p') selects all
elements).
- ID Selector: Selects an element with a specific ID (e.g., $('#elementID') selects the element with ID 'elementID').
- Class Selector: Selects elements with a specific class (e.g., $('.className') selects all elements with class 'className').
$(document).ready(function() { $('p').css('color', 'blue'); $('#example').css('font-weight', 'bold'); $('.highlight').css('background-color', 'yellow');});In this example, jQuery is used to change the style of various elements based on their selectors.
Mastering jQuery AJAX
How to Use jQuery AJAX
AJAX (Asynchronous JavaScript and XML) allows web pages to send and receive data from a server asynchronously without interfering with the display and behavior of the existing page.With jQuery, making an AJAX call is very straightforward. The basic syntax for an AJAX request using jQuery is as follows:
$.ajax({ url: 'your-url-here', type: 'GET', // or POST success: function(data) { // Code to handle successful response }, error: function(error) { // Code to handle an error }});Here’s an example that demonstrates a simple GET request using jQuery AJAX:
$.ajax({ url: 'https://api.example.com/data', type: 'GET', success: function(data) { console.log(data); }, error: function(error) { console.error('An error occurred:', error); }});
jQuery AJAX Best Practices
When using jQuery AJAX, following best practices is essential to ensure that your application performs efficiently and remains user-friendly.Here are some key best practices to consider:
- Use the right HTTP method: Choose between GET and POST based on the nature of the data being sent or received.
- Handle errors gracefully: Always implement error handling in your AJAX calls to give users feedback and help with debugging.
- Keep requests efficient: Minimize the data sent over the network by only requesting what is necessary.
- Use caching: Leverage caching mechanisms to reduce unnecessary server load and improve performance.
- Simplify your code: Use jQuery's shorthand methods like $.get() and $.post() for cleaner and more readable code.
Always test your AJAX calls in different scenarios to ensure they handle various responses correctly.
The power of AJAX lies in its ability to create dynamic user experiences. By loading data asynchronously, you can update parts of a webpage without requiring a full reload, which leads to better performance and user satisfaction. Here are some important concepts to understand when working with jQuery AJAX:
- JSON: Often, the data exchanged between the client and server is formatted in JSON (JavaScript Object Notation), which is easy to parse and use in JavaScript.
- Cross-Origin Requests: Be aware of CORS (Cross-Origin Resource Sharing) when making AJAX requests to different domains. Some servers may require specific headers to allow requests.
- Deferreds and Promises: jQuery AJAX methods return a Promise, which represents the eventual completion (or failure) of the asynchronous operation, enabling further processing once the request is complete.
Exploring jQuery Selectors
Types of jQuery Selectors
In jQuery, selectors are used to find and manipulate HTML elements. They are the backbone of jQuery’s ability to interact with elements on a web page. Below are some common types of jQuery selectors that you should be familiar with:
- Universal Selector: Selects all elements in the document. You can use it as
*
. - Tag Selector: Selects all elements of a specific type. For example,
$('div')
selects all elements. - ID Selector: Targets an element with a specific ID. For example,
$('#myID')
selects the element with the ID 'myID'. - Class Selector: Selects all elements with a specific class. You can write it as
$('.myClass')
. - Attribute Selector: Targets elements based on their attributes like
$('[type=
jQuery Selectors Examples
Here are some practical examples that demonstrate how to use various jQuery selectors in real-world scenarios:1. Selecting All Paragraphs:
$(document).ready(function() { $('p').css('color', 'red');});
This code selects allelements on the page and changes their text color to red.2. Selecting by ID:
$(document).ready(function() { $('#uniqueElement').addClass('highlight');});
This example selects the element with the ID 'uniqueElement' and adds the class 'highlight' to it.3. Selecting by Class:$(document).ready(function() { $('.active').fadeOut();});
This code selects all elements with the class 'active' and fades them out.4. Attribute Selector:$(document).ready(function() { $('a[target]').css('font-weight', 'bold');});
This snippet selects all elements that have a 'target' attribute and changes their font weight to bold.To chain multiple selectors, separate them with a comma, like
$('.class1, .class2')
.Understanding jQuery selectors is crucial for manipulating DOM elements. Each selector type serves a specific purpose, making it easier to target elements you want to work with. The performance of your jQuery code can also depend on how efficiently you use selectors.For instance, using tag selectors is generally faster than using class or ID selectors, as they are less specific. Here’s a breakdown of selector performance:
Selecting the right kind of elements can significantly improve the efficiency of your web applications. Always consider the scope and necessity of your selectors for optimal results.Selector Type Performance Universal Selector Slowest Tag Selector Fast ID Selector Fast Class Selector Moderate Attribute Selector Moderate Implementing jQuery Each
jQuery Each Function Explained
The jQuery each() function is an essential method that allows you to iterate over a collection of elements. It assigns a function to each element in the selected set of elements, enabling bulk manipulation.Here’s how the syntax looks:
$(selector).each(function(index, element) { // Code to run for each element});
This method can be particularly useful when you need to apply the same function to multiple elements. It supports both index (the position of the element in the set) and element (the actual DOM element) as parameters.Here’s a basic example using the each() function to alert the text of each li element in a list:
$('li').each(function(index, element) { alert($(element).text());});
This code will loop through every list item and show an alert with the text of each item.Practical Use Cases for jQuery Each
The each() function is versatile and can be applied in multiple scenarios. Here are some practical use cases:
- Applying Styles: You can change the appearance of multiple elements at once. For example, to change the background color of all paragraphs:
$('p').each(function() { $(this).css('background-color', 'yellow');});
- Aggregating Data: You can sum up values from a collection of elements, such as getting the total price of items in a cart:
let total = 0;$('span.price').each(function() { total += parseFloat($(this).text());});console.log('Total Price:', total);
- Manipulating Element Attributes: You can modify or retrieve attributes for multiple elements. For example, to add a custom attribute to all images:
$('img').each(function() { $(this).attr('data-custom', 'myValue');});
Use
this
within theeach()
function to directly reference the current element.Understanding the jQuery each() function is critical for efficient DOM manipulation. This function does not create a new jQuery object, which means you are working with the actual elements in the DOM as you manipulate them. Here’s an in-depth look at its benefits and considerations:
- Performance: Iterating using each() is generally faster than using a traditional for loop, especially with larger sets of elements.
- Contextual Access: Within the each() function,
this
refers to the current element being iterated. This allows for more readable and maintainable code. - Callback Function: The callback function receives two arguments: the index of the element and the element itself, providing flexibility for various operations.
jQuery - Key takeaways
- jQuery Overview: jQuery is a powerful JavaScript library that simplifies HTML document manipulation, event handling, and AJAX requests, making web development more efficient.
- jQuery Selectors: Selectors, such as element, ID, and class selectors, are fundamental jQuery concepts that allow targeting and manipulation of specific HTML elements.
- jQuery AJAX: AJAX in jQuery enables asynchronous data requests without disrupting the user experience, facilitating dynamic content updates on web pages.
- Best Practices in jQuery AJAX: Key best practices include using appropriate HTTP methods, implementing error handling, and minimizing request data for optimal application performance.
- jQuery Each Function: The jQuery each() function allows iteration over a collection of elements, enabling bulk manipulation and access to individual elements within a set.
- Performance of Selectors: The efficiency of jQuery code can be improved by understanding the performance characteristics of different selector types, with tag selectors being generally faster than ID or class selectors.
- Applying Styles: You can change the appearance of multiple elements at once. For example, to change the background color of all paragraphs:
Learn faster with the 27 flashcards about jQuery
Sign up for free to gain access to all our flashcards.
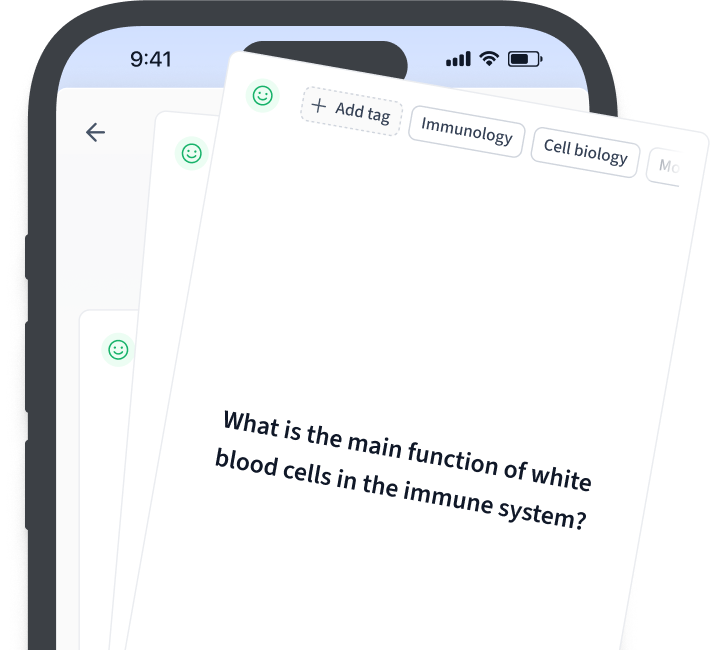