Jump to a key chapter
Understanding the Accumulator in Computer Science
When diving into the world of computer science, you'll encounter various terms and concepts that are key to understanding how computers operate and perform tasks. One such term is an "accumulator".
Definition of Accumulator
In computer science, an accumulator generally refers to a type of register in a computer's central processing unit (CPU) that temporarily stores the results of computations.
Specifically, an accumulator is a register wherein intermediate arithmetic and logic results are stored. Without an accumulator, it would be necessary to write the result of each calculation (addition, multiplication, shift, etc.) to main memory, and only then could they be used in other calculations. Accumulators significantly increase the speed of the CPU and thereby improve the system's overall performance.
Key Features of An Accumulator
- The accumulator actively participates in computations and data manipulation.
- Its primary purpose is to hold and transfer data.
- Accumulators can operate in conjunction with other registers to manage data flow within the CPU.
For instance, when performing a task such as adding multiple numbers together, the accumulator will store the initial value (usually starting at zero), then as each digit is calculated, the value is added to the accumulator's current value, thereby "accumulating" the total sum.
Role of the Accumulator in Computer Science
As you venture deeper into computer science, you'll discover that accumulators have a broad range of applications. They serve a crucial role in the operation of a CPU, functioning as a bridge between computations and the memory unit. Let's explore this further:
Accumulators are fundamental components of the arithmetic and logic unit (ALU), the part of the CPU that performs arithmetic and logic operations. The ALU uses the accumulator as a kind of "working space" to facilitate these computations. As computations take place, the accumulator collects the partial results, stowing them away securely until they’re needed for upcoming calculations. It then holds onto the final result until it can be moved to a more permanent storage location, like a memory location or another register.
Operation | Description |
Addition/Subtraction | The accumulator adds or subtracts quantities and keeps the resultant value. |
Multiplication/Division | In multiplication or division, the accumulator stores the product or quotient. |
Data Transfer | The accumulator temporarily holds data that is being moved around within the CPU. |
Importance of Accumulator for Computation
If you're wondering about the significance of accumulators in the grand scheme of computations, you're asking the right questions. Here's why accumulators matter:
- Speed: Accumulators enhance the speed of calculations. Temporary storage of intermediate results minimizes the need to frequently access the slower main memory.
- Efficiency: By acting as a portal between the ALU and the memory unit, accumulators make the computational process more efficient.
- Performance: Ultimately, the use of accumulators improves the overall performance of the CPU.
Say you're using your computer to edit a large image file. This task requires a lot of pixel computations. Your CPU doesn’t need to go to the main memory for each of these computations – instead, the accumulator provides a much faster alternative, storing and providing the intermediate values required, resulting in a smoother, faster editing process.
Establishing Accumulator Examples
In computer programming, accumulators are utilised in a variety of practical ways. By understanding their function and utility, you can gain a more nuanced perspective of their influence on everyday computing.
Practical Examples of Accumulator Use
There are many practical examples of how accumulators are used in computing. To understand the concept better, let's go through a few examples where accumulators play a crucial role.
Let’s take the popular high-level programming language, C++. In this language, the accumulator pattern is often used to sum up arrays. Here's a small code snippet for the sum of an array:
int array[] = {1, 2, 3, 4, 5}; int sum = 0; // accumulator initialised at 0 for (int n=0; n<5; n++) { sum += array[n]; // accumulate the sum }
In the above example, the sum is the accumulator. It stores temporary results of the computations and keeps adding each new array element to the existing total.
In another example, let's consider a scenario where you're doing data analytics. You might be using a tool like Apache Spark, which provides an Accumulator API for these precise needs. Here, an accumulator could be used to count events in a very large dataset in a parallel and distributed way.
Influence of Accumulators on Everyday Computing
The impact of accumulators on everyday computing is quite significant. While their operation lies buried deep within the hardware or hidden in the lines of a software code, their effects ripple out, shaping various aspects of your computing experience.
- Speed: Similar to the example of image processing mentioned earlier, imagine watching a high-definition video, playing a graphics-intensive game, or running a complex simulation. These tasks involve numerous operations that rely on the swift functioning of accumulators.
- Multi-tasking: Whenever you multitask on your computer - opening multiple applications or browser tabs, editing a document while running a scan, and so on - accumulators make this possible by efficiently handling intermediate results of various operations.
- Data Analysis: In the field of data analysis, accumulators play a pivotal role. They help handle large amounts of data effectively and make the process of extracting useful information much faster and more efficient.
If you've ever used a spreadsheet application like Microsoft Excel, you've certainly relied on accumulators. Functions like SUM, where you add a whole range of numbers, utilise an accumulator to store the ongoing total. Every time a new value is added to the sum, it's added to the value in the accumulator, and the result becomes the new accumulated value. So every time you use such a function, you're using an accumulator!
The efficiency of accumulators, their speed in handling computations, and their ability to multitask make them crucial components of modern computing devices. Their behind-the-scenes work allows you to experience seamless use of technology in your daily operations.
Exploring the Accumulator Technique
As you deepen your understanding of computer science, it's important to fully grasp the functioning and impact of the accumulator. The very essence of the accumulator technique revolves around its ability to temporarily hold and manipulate data for quick and efficient computations.
How Does the Accumulator Technique Function?
Let's delve deeper into the functioning of the accumulator technique. Devised to store intermediate results of computations, the process centres around the arithmetic and logic unit (ALU). Upon completion of each computation, the ALU uses the accumulator as a storage space for these interim results.
But how is such a process beneficial? Let's unfold its working with a step-by-step breakdown:
- The initial stage of the process involves the ALU performing an arithmetic or logic operation.
- Immediately following this operation, the outcome, known as the intermediate result, needs temporary storage. This storage space is provided by the accumulator.
- These intermediate results can then be utilised for further calculations without having to tap into the slower main memory – a feature that remarkably speeds up computational tasks.
- The accumulator persists in maintaining these outcomes until they can be transferred to another register or a permanent storage location, such as a memory location.
It should be noted that while the process varies slightly depending on the specific CPU architecture and the operation being executed, the basic idea remains the same: the accumulator collects and holds results for future use, thereby speeding up the computation process.
Impact of Accumulator Technique on Computer Performance
By now, you've become familiar with the workings of the accumulator technique. But to what extent does it influence computer performance? In essence, the accumulator significantly optimises the performance by minimising the reliance on the main memory for each calculation, resulting in a faster and more efficient operation. Let's breakdown its impact into three key areas:
- Versatility: Accumulators cater to a broad spectrum of arithmetic and logical operations, making them incredibly versatile with diverse applications.
- Speed and Efficiency: Since the accumulator technique facilitates storing intermediate results close to the ALU, the necessity for slower memory access is considerably reduced. This element results in faster computation, enhancing overall efficiency.
- Optimisation of Data Flow: The accumulator acts as a portal connecting the ALU and the memory unit, thereby facilitating a smoother and more optimised data flow.
It's evident that the accumulator technique has substantial benefits and its contribution towards improving computer performance is vital.
For instance, in parallel computing – a form of computation where several calculations are performed simultaneously – accumulators are indispensable. Consider a program that's executing on a multi-core processor, utilising multiple threads to perform the same operation on different pieces of data. If this operation involves accumulating a result (like adding up all these pieces), you'll need a separate accumulator for each thread to avoid data collisions. Here, the accumulator technique's efficient handling of temporary data enables each core to perform its tasks independently and swiftly.
Despite being an intrinsic part of the processor’s functionality, the accumulator and its technique can often go unnoticed given their internal role in computation. However, through this deep exploration into their functioning and impact on performance, their fundamental importance in the realm of computer science is distinctly highlighted.
Accumulator Vs Register: A Detailed Comparison
Delving into the diverse components that define the composition of a computer, the terms "accumulator" and "register" often surface, sparking debates regarding their roles, purposes, and differences. Despite both being storage units within the processor, their functionalities differ, warranting a detailed comparison.
Key Differences Between Accumulator and Register
Centred around processor operations, accumulators and registers are both storage units within a CPU (Central Processing Unit). The heart of their contrast lies in their operating protocols and responsibilities.
The accumulator, as previously discussed, is a special kind of register that temporarily stores and manipulates data for quick and efficient arithmetic and logical computations. It's responsible for holding the intermediate results of calculations and acts as a portal connecting the ALU (Arithmetic Logic Unit) and memory unit. Its role is crucial in optimising data flow, reducing the need for slower memory access, and hence enhancing overall computational efficiency.
On the other hand, a register is a small storage entity that directly resides within the CPU. Registers hold instructions, storage addresses, and other data relevant during execution. There are many types of registers based on their usage such as General Purpose Registers, Instruction Registers, and Stack Registers. Unlike the accumulator, registers are not limited to holding and manipulating arithmetic and logic data. They are also responsible for tracking program execution and multi-tasking within the processor.
Aligning these differences to better understand their roles within the processor, we can create a comparison table:
Difference Aspect | Accumulator | Register |
Data Storage | Stores intermediate computations | Holds instructions, storage addresses, etc |
Functionality | Focused on arithmetic and logic calculations | Broad functionality including program tracking & multi-tasking |
Relation with ALU | Directly linked with ALU's operation | Not directly linked with ALU's operation |
Understanding When to Use Accumulator and When to Use Register
While both the accumulator and register are imperative components of a processor, discerning when to use each can further streamline your computing tasks. As previously mentioned, accumulators are the go-to units for tasks involving arithmetic and logic computations. Products of such computations are held in the accumulator for future use, fostering smoother data flow and improved computational speed. Consequently, if your task involves lots of additions, subtractions, and logical operations, the accumulator becomes essential.
Registers, in comparison, offer a broader spectrum of functionalities. If your task involves tracking program execution, maintaining instruction sequences, or multi-tasking, registers are naturally more suitable. They facilitate simultaneous execution of multiple operations, driving the system towards efficiency. Furthermore, registers also play an important role in memory management – they hold memory addresses and frequently used data to decrease access time.
- Accumulator:
Use when your computing task involves significant mathematics and logical operations.
For instance, if you are implementing a machine learning algorithm that involves a lot of mathematical calculations, having a strong accumulator is paramount.
- Register:
Use when tasks require tracking sequences or multi-tasking.
An example here is managing an operating system. In this case, registers are required to track tasks, manage memory, and provide a smooth user experience.
To sum up, selecting between an accumulator and a register largely depends on the nature of your computing task. An understanding of their key differences helps you optimise your system's performance by making the appropriate choice based on the task's requirements.
Examining Accumulator Principles
Diving deeper into the realm of computer science, the principles governing the functioning of an accumulator play a pivotal role in fostering computational efficiency. These principles are intrinsic to computer organisation and directly influence the architecture of a system, thus shaping its capabilities and potential.
Basic Principles of Accumulator in Computer Organisation
Within the discipline of computer organisation, the accumulation principle forms a cornerstone. This principle, embodying the essence of an accumulator, is hinged on the concept of using interim storage for conducting arithmetic and logical operations, subsequently expediting the computation process.
Outlined as follows are a few key principles surrounding an accumulator's functionality:
- Temporary Storage: Accumulator's primary function is to serve as temporary storage for intermediate results during calculations. This principle negates excessive memory access, thereby increasing speed and efficiency.
- Arithmetic and Logic Operations: Accumulators are directly linked to the ALU, performing a plethora of arithmetic and logical operations. This principle facilitates computation at high speed, as the results are stored within the accumulator itself for further use.
- Data Flow Optimisation: One of the less discussed yet critical principles of accumulator functioning is its influence on data flow. By acting as a portal connecting the ALU and memory units, accumulators streamline data transfer, thereby optimising the flow within the CPU.
- Decreased Proficiency for Large Data Sets: Accumulators work best for small or medium datasets. Since accumulators have a limited storage capacity, their proficiency decreases in case of larger data sets needing computation – an important principle that determines accumulator usage based on task requirements.
The Last-In-First-Out (LIFO) Principle: Accumulators often operate on the Last-In-First-Out (LIFO) principle. This means the most recent data fed into the accumulator will be the first to be retrieved for further calculations or data transfer. This operation is also observed in controlling data flow in stack memory.
For instance, if you're manipulating an array of integers in Java, you might use an accumulator principle to sum the numbers. The code might appear similar to this:
int sum = 0; // Accumulator starts at 0 for (int i = 0; i < arr.length; i++) { sum += arr[i]; // The sum accumulator adds the value of arr[i] } // After the loop, 'sum' would contain the total sum of the array elements.
How Accumulator Principles Affect System Architecture
The principles guiding the operation of an accumulator are crucial to moulding the architecture of a system. By reducing the processor's dependence on the main memory for every computation, accumulators alter the system architecture towards improved efficiency and operational speed.
In single-accumulator architecture, also known as a 'one address computer', the accumulator plays a central role. Here, the accumulator handles one of the operands for all computational instruction. This architecture fosters simpler operation and easy-to-understand coding, though the code might be lengthier, with limited computational capability.
For example, consider a small system like an 8-bit microcontroller (e.g., PIC18). This class of device features single-accumulator architecture, evident from the following assembly code snippet:
movlw 5 // Loads the constant value 5 into W addwf val // Adds W to 'val' and stores the result into W
On the contrary, complex systems like an x86 processor utilise multiple registers alongside an accumulator, constituting a 'two address computer' architecture. In such systems, either operand for a computation can come from any register, introducing flexibility and allowing for more complex and efficient code.
Let's consider an x86 assembly code example:
mov eax, 5 // Loads the constant value 5 into EAX add eax, val // Adds EAX to 'val' and stores the result into EAX
System architecture decisions, therefore, are largely influenced by the principles of accumulator functioning. These principles shape the operation protocols within a CPU, dictate the system's handling of computations, and ultimately, define the efficiency and performance aspect of the entire system.
Accumulator - Key takeaways
- The significance of accumulators in computations comes from their ability to enhance calculation speed, improve computational efficiency, and boost overall CPU performance.
- The accumulator in programming languages like C++ is often used in utilising accumulator pattern to sum up arrays, acting as a temporary storage for intermediate results of computations.
- The accumulator technique functions by storing intermediate results from the ALU, which facilitates quick computations without having to access the slower main memory, leading to overall enhancement in computer performance.
- Understanding the key differences between an accumulator and a register is important: while both are storage units within the CPU, the accumulator is used specifically for arithmetic and logical computations, while registers have a more diverse range of functionalities, such as tracking program execution and multi-tasking.
- Accumulator principles in computer organisation revolve around the concept of using temporary storage for conducting operations, thus enabling faster and more efficient computations.
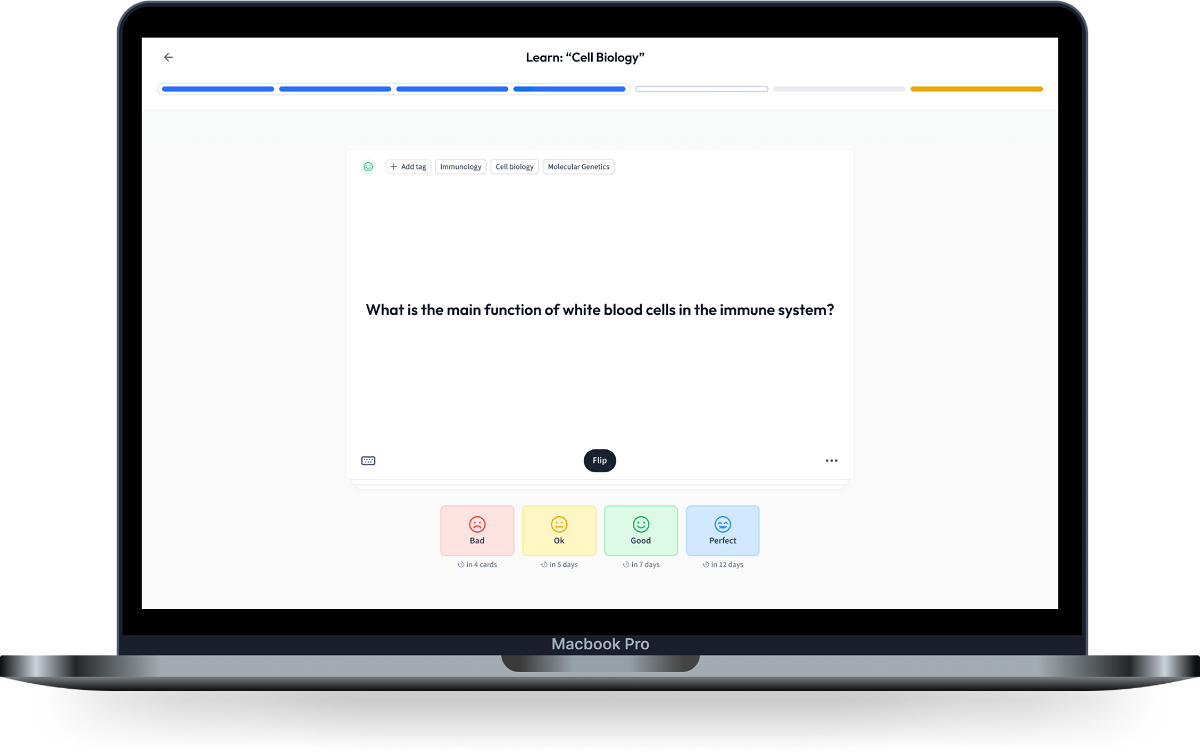
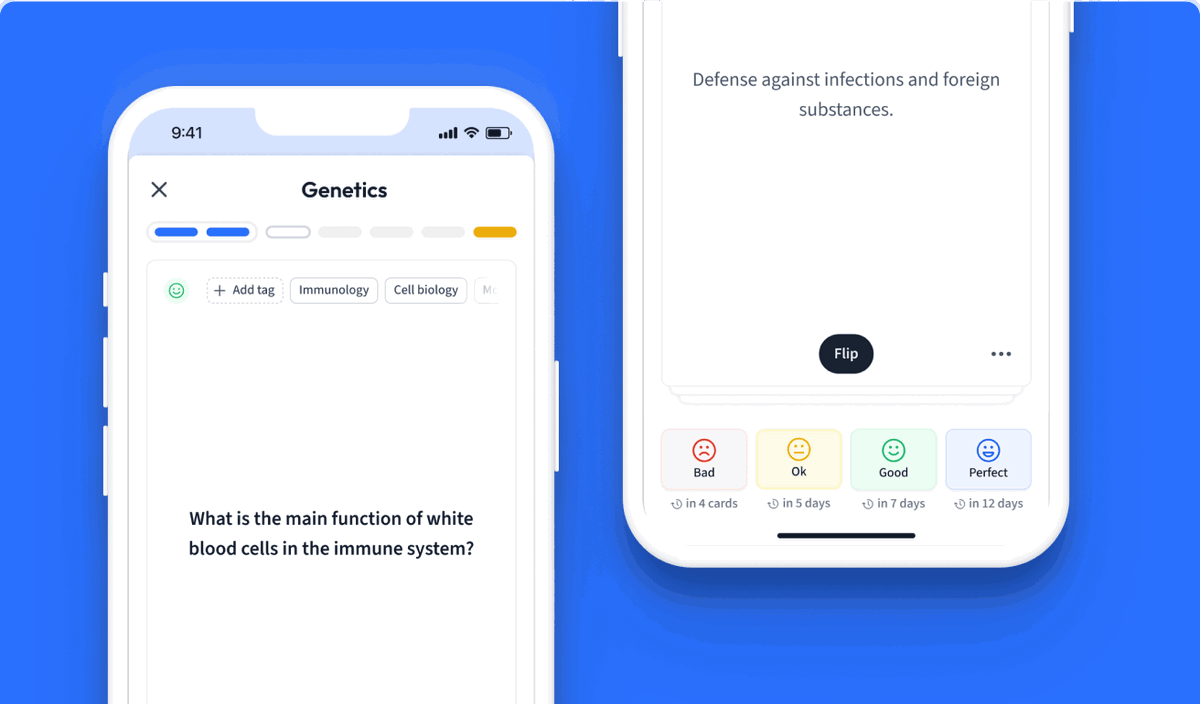
Learn with 15 Accumulator flashcards in the free StudySmarter app
Already have an account? Log in
Frequently Asked Questions about Accumulator
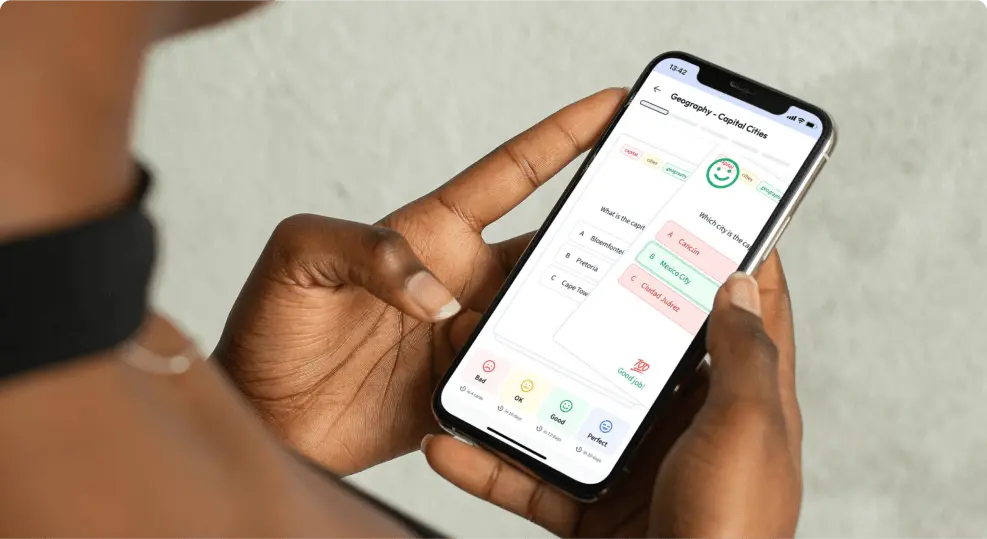
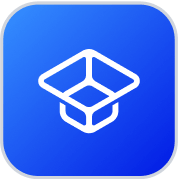
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more