Jump to a key chapter
Understanding the Basics of Garbage Collection in Computer Science
Garbage collection, in this context, is not the physical waste you dispense weekly; rather, it's a critical function in computer programming.In computer science, garbage collection refers to the automated process of identifying and reclaiming memory that is no longer in use by a program.
The Fundamentals of Garbage Collection
Garbage Collection (GC) is primarily an automatic memory management system. It is the process through which programs try to free up space previously allocated and currently not in use by the program.Allocated Memory | The storage set aside for a running program to utilize at any given time. |
Reusable Memory | The portion of the allocated memory that is currently unused or no longer needed by the program. |
Heap memory is a region of a computer's RAM used for dynamic memory allocation. Stack memory is faster, but variables created in this region only exist for the duration of the function frame.
Types of Garbage Collection Techniques
There are numerous techniques for executing garbage collection, each with its own set of trade-offs. Here are some of the most common:- Mark and Sweep: This process involves marking active objects and then sweeping away inactive ones.
- Copy Collection: This involves copying all active objects to a separate space in memory.
- Reference Counting: This strategy involves keeping a count of the number of references to each object.
Detailed Breakdown of the Garbage Collection Process
In some languages like Java, garbage collectors are part of standard library features. Others, like C++, place the burden of memory management fully in the hands of the programmer.
- Mark: The garbage collector marks all memory segments currently in use.
- Sweep: It then sweeps through the memory, freeing segments not marked in use.
- Compact: Now, the garbage collector compacts the remaining used memory segments to create one big chunk of free memory.
totalMemory = 10 inUseMemory = [1, 2, 5, 7, 8] Mark phase: for slot in totalMemory: if slot in inUseMemory: mark slot as in-use Sweep phase: for slot in totalMemory: if slot not marked as in-use: free slot
Delving into the Concept of Garbage Collection in Java
Garbage collection in Java is an automatic process which aims to reclaim the 'garbage', or memory space taken up by objects that are no longer used by the program. Without garbage collection, unused but allocated memory could potentially rack up, leading to inefficiency in memory use, or in extreme cases, a memory leak. Freeing memory that is no longer needed allows for an optimised use of resources.Basics of Garbage Collection in Java
In the Java programming language, memory management is largely automated by the Java Virtual Machine (JVM). The JVM employs garbage collection to deal with the challenge of automatically freeing memory by deleting objects that are no longer accessible to the program. With garbage collection, programmers are unburdened from tracking every new object, allowing them to focus more on core application development. The garbage collection process consists of three basic activities: Marking, Sweeping, and Compacting. Marking is the first step where the garbage collector identifies which pieces of memory are in use and which are not. Sweeping is the phase where the garbage collector eliminates unreferenced objects and reclaims memory occupied by garbage objects. And finally, Compacting is the third phase that aims to eliminate the fragmentation created in the sweep phase by moving the objects left after sweeping phase to one end of heap memory, thereby making a contiguous free space block at the end.How Java Manages Garbage Collection Process
Java manages garbage collection via an aspect of JVM known as the garbage collector (GC). When a program creates an object, the JVM automatically allocates memory space for that object from the heap. When the object is no longer in use, that memory becomes unallocated or 'garbage.' To determine which objects are no longer in use, the Java GC uses a process known as 'mark and sweep'. Java employs multiple GC algorithms like Serial Collector, Parallel Collector, CMS Collector and G1 Collector each with various strengths designed for specific use cases, but they all adhere to the key principle of mark, sweep and compact. Garbage Collection can be manually requested in Java by callingSystem.gc(), however, the execution of this is not guaranteed as the decision is ultimately made by the JVM.
Garbage Collection Algorithm in Java
Java's garbage collection mainly employs four algorithms: Serial Collector, Parallel Collector, CMS Collector, and G1 Collector. Serial Collector is the simplest GC algorithm. It uses a single threaded model for both minor and major garbage collection, meaning only one processor is used for GC operations and all other tasks are stopped during its operation. It is useful for client-machines such as our personal computers, or for applications with smaller data sets (up to approximately 100MB). Parallel Collector, also known as the throughput collector, is aimed at multiprocessor machines. Its objective is to maximize the throughput by minimizing the CPU time required for garbage collection. Next is the CMS Collector, or the Concurrent Mark Sweep Collector. It reduces garbage collection pause times for applications that require low latency. And finally, the G1 Collector, also known as the Garbage-First collector, is intended for applications running on multi-processor servers with large amounts of memory. It attempts to meet GC pause time goals with high probability while achieving high throughput. Each algorithm has its unique functionalities and trade-offs. Java developers can choose the best garbage collection algorithm for their application based on specific needs and workloads.Exploring the Mechanism of Python Garbage Collection
Python is one of the high-level programming languages that automatically provides memory management, including garbage collection. This robust feature of the language enables developers to create applications without focusing on lower-level details such as manual memory allocation and deallocation.Introduction to Python Garbage Collection
In Python, memory management is done in a two-fold manner: through reference counting as the primary method and garbage collection as a secondary mechanism. Garbage collection in Python incorporates algorithms to detect and collect circular, or self-referential, groups of waste that reference counting can't handle. Essentially, the Python garbage collector ensures efficient management of dynamically allocated memory for Python objects. That is, it automatically reclaims memory that the data objects aren't using, and returns it to the operating system or designates it for reuse within the program. The main reasons for employing garbage collection are: to prevent software bugs resulting from poor memory handling, to mitigate system crashes due to memory exhaustion, and to reduce the time and complexity that comes with manual memory management.How Python’s Garbage Collection Process Works
The process of garbage collection in Python kicks in when objects are no longer of use. So, how does Python determine whether an object is being used or not? Python automatically employs a system of reference counting to keep track. Each object contains a counter, which is incremented whenever a reference to the object is stored somewhere, and decremented when a reference to it is deleted. In essence, this counter keeps track of the number of references to the object. When an object's reference count hits zero — meaning there are no references to the object — it becomes 'orphaned'. These orphaned objects are considered garbage since they're no longer reachable by the program and hence, can't be used again. However, reference counting alone isn't enough. Python's garbage collection also deals with 'circular references'.A circular reference occurs when a group of objects become unreachable from the rest of the application but still reference each other. In this case, even though they’re collectively unreachable, their reference counts never fall to zero.
Python Garbage Collection Techniques
Since circular references can't be handled efficiently by basic reference counting, Python uses a more complex garbage collection process specifically targeted at detecting and cleaning up these reference loops.
import gc gc.set_debug(gc.DEBUG_STATS) lst1 = ['Python', 'Garbage', 'Collection'] lst2 = lst1 lst1.append(lst1)
Conventional Garbage Collection Algorithms in Computer Science
The management of data object memory, especially their creation and disposal, is a critical aspect of programming. This management is essential to avoid wasted memory and the possible failure of programs due to lack of memory. Therefore, garbage collection algorithms are indispensable in any computing environment.An Overview of Garbage Collection Algorithm
In computer science, a garbage collection algorithm is an automatic memory management scheme that reclaims heap-allocated memory that is not being used by the program. This 'garbage' encompasses objects that are no longer used or have become unreachable in the memory. To be more specific, when a program no longer has any references to a data object, that object is considered dead and its memory is termed 'garbage'. If this garbage isn't collected, memory resources can gradually dwindle, leading to slower performance or, in severe cases, failure due to memory exhaustion. Garbage collection involves two significant processes: identifying garbage objects and reusing or deallocating the memory allocated to those objects. The way these two processes are carried out depends on the specific garbage collection algorithm in use. With respect to unearthing garbage, the garbage collector must accurately determine objects that are no longer useful. A common approach is to view objects which are unreachable as garbage. The garbage collector deems all objects reachable from global variables or the program stack as live and those in the heap that can't be reached as dead. In the context of reusing or deallocating memory, after the garbage collector establishes which objects are garbage, it needs to recycle the object's memory. The process of recycling the memory varies across different garbage collection algorithms and depends on the resources available.Popular Garbage Collection Algorithms
Several garbage collection algorithms have evolved over the years given the varying demands of different systems. The popular ones include Reference Counting, Mark-Sweep, Mark-Compact, and Copying garbage collection algorithms. Reference Counting: This garbage collection algorithm handles each object with a reference count. When a reference is added or removed, the count is updated accordingly. If an object's reference count drops to zero, the object is viewed as garbage. Mark-Sweep: In the Mark-Sweep garbage collection algorithm, the garbage collector 'marks' all live objects throughout the system and 'sweeps' through the heap space, erasing unmarked objects and freeing up memory. However, this technique might lead to problems of fragmentation. Mark-Compact: To rectify fragmentation issues, the Mark-Compact garbage collection algorithm shifts live objects so that they are adjacent in memory, making the remaining memory one large block. Copying: This technique divides the heap into two halves, with all allocations happening in one half. Once that half is full, the algorithm copies over live objects to the other half, abandoning the remaining objects as it goes. Each garbage collection algorithm has advantages and disadvantages, and different applications may favour different techniques based on their requirements.Algorithm Advantages and Pitfalls in Garbage Collection
While garbage collection algorithms provide automatic memory management, they come with their strengths and weaknesses, affecting the performance of a system. Advantages of garbage collection algorithms:- Eliminate 'dangling pointers' by ensuring that an object is not mistakenly accessed after it has been deleted.
- Handle critical tasks of memory management, removing some burdens from the programmer.
- Protect against memory leaks by limiting the build-up of unused objects.
- Decrease fragmentation via certain methods like compacting.
- Can cause possible pauses during execution as garbage collection may require halting other processes.
- May lead to memory overhead since objects remain in memory until the garbage collector disposes them.
- Dealing with circular references might be complex for certain algorithms.
- Different languages might not equally support or optimise for all types of garbage collection.
Exploring Advanced Garbage Collection Techniques
Garbage Collection (GC), as an aspect of automatic memory management, has seen substantial development over the decades, with multiple techniques emerging to address the trade-offs inherent in GC. In high-level languages like Java or Python, memory management and, implicitly, garbage collection, is an essential background process. Advanced garbage collection techniques aim to optimise this process, enhancing program performance and mitigating associated downsides.Different Techniques in Garbage Collection
There are numerous advanced techniques applied today in garbage collection, each addressing the fundamentals of the process in unique ways. The right choice of technique often depends on the specific requirements of the application in question. Incremental Collection: This technique aims to reduce the interruptions caused by garbage collection. Instead of processing the entire heap all at once - which can lead to pauses - it processes a part of the heap in each garbage collection cycle, distributing the workload over time. Generational Collection: Generational GC is based on the empirical observation, also known as the Generational Hypothesis, that most objects die young. Here, memory is divided into generations. New objects are placed in a generation for young objects (also known as the nursery), and objects that survive several garbage collections are moved to an older generation. This technique optimises GC by concentrating on collecting 'young' objects. Parallel Collection: In a multi-processor or multi-core environment, GC can be executed in parallel across multiple threads. This technique aims to speed up garbage collection by taking advantage of the multiple cores available. Concurrent Collection: Concurrent GC is an extension of incremental GC where some parts of a collection cycle are executed concurrently with the application. This technique further reduces GC pauses in the application, making it suitable where low latency is needed. Real-Time Collection: In applications where meeting real-time constraints is necessary, the real-time garbage collection technique comes in handy. Real-time GC guarantees a maximum pause time, reducing the total time spent in garbage collection.Latest Developments in Garbage Collection Techniques
As computing applications continue to expand in complexity and size, the need for more efficient and effective garbage collection techniques grows. Algorithmic advancements and ever-evolving hardware capabilities bring forth novel approaches to garbage collection.
Common Problems and Solutions in Garbage Collection Techniques
Garbage collection techniques face notable challenges. However, with thoughtful design and architecture decisions, these can be mitigated effectively. Problem - Performance Overhead: GC comes with computational costs that can impact application performance. A frequently running garbage collector can degrade system performance and responsiveness. The solution could lie in utilising advanced techniques like incremental, concurrent, or parallel garbage collection, which help balance garbage collection workload and application performance. Problem - Memory Overhead: Mismanaged garbage collection can introduce memory overhead. Live objects might occupy only a small fraction of the heap, leaving large parts of it unused. Incorporating generational or region-based garbage collection could be a practical solution to this issue. Problem - Stop-the-world Pauses: Certain phases in garbage collection may necessitate a pause in application execution, thereby causing performance issues. Employing concurrent garbage collectors that run concurrently with the application helps in mitigating such pause times. Problem - Fragmentation: After several cycles of allocation and deallocation, memory can become fragmented, with free memory blocks scattered throughout the heap. Techniques such as Mark-Compact or Copying collectors can compact memory, reducing fragmentation.The selection and application of garbage collection techniques is a trade-off scenario. The best approach depends on the specific needs and context of the application. A thorough understanding of the underlying principles of GC and a clear definition of performance metrics pave the way towards choosing the optimally efficient garbage collection technique.
Garbage Collection - Key takeaways
- Garbage Collection is the process of automatically freeing memory by deleting objects that are no longer in use.
- In Java, garbage collection is managed by the JVM (Java Virtual Machine), and involves three processes: Marking (identifying which pieces of memory are in use), Sweeping (eliminating unreferenced objects and reclaiming memory), and Compacting (eliminating fragmentation created in the sweep phase).
- Java's garbage collection can employ various algorithms. These are: Serial Collector (useful for applications with smaller data sets), Parallel Collector (minimizes the CPU time required for garbage collection), CMS Collector (reduces garbage collection pause times), and G1 Collector (meets GC pause time goals with high probability while achieving high throughput).
- Python garbage collection is done through reference counting and a garbage collector to handle circular references (objects that become unreachable from the rest of the application but still reference each other). It groups objects into 3 generations to make the process more efficient.
- Garbage Collection algorithms in Computer Science include: Reference Counting (handles each object with a reference count), Mark-Sweep (marks all live objects and sweeps through the heap space, erasing unmarked objects), Mark-Compact (shifts live objects so that they are adjacent in memory), and Copying (divides the heap into two halves, moving live objects to the other half).
Learn faster with the 39 flashcards about Garbage Collection
Sign up for free to gain access to all our flashcards.
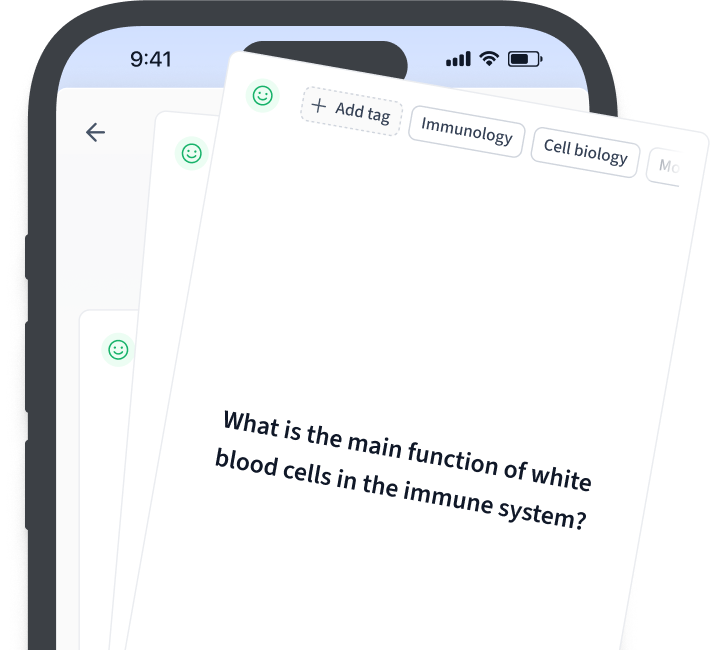
Frequently Asked Questions about Garbage Collection
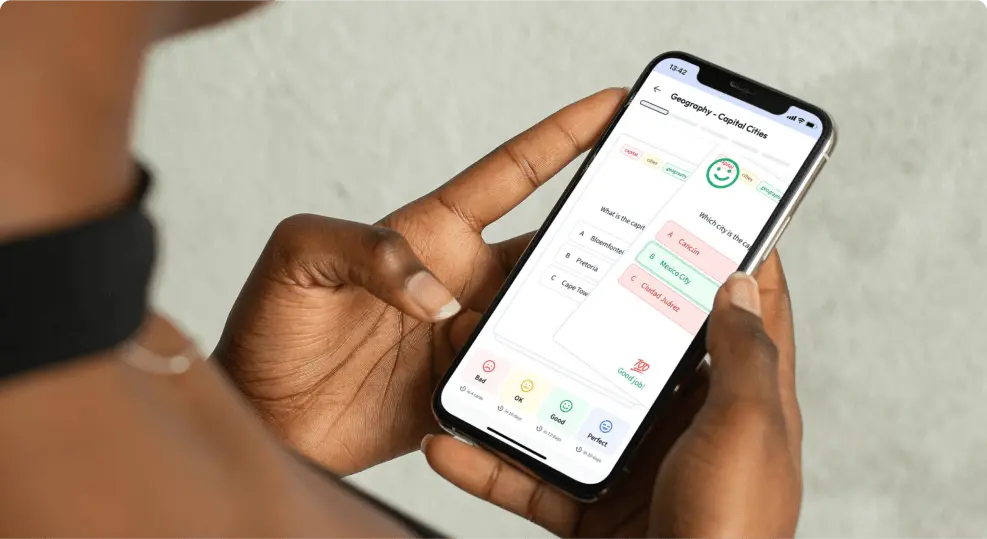
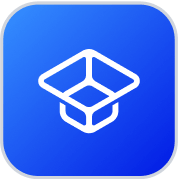
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more