Jump to a key chapter
Understanding Memory Leaks
When it comes to programming and computer science, you will come across a myriad of concepts and principles that are crucial to creating efficient and effective software. One of these concepts is 'memory leak' which is a key issue in memory management.Definition of a Memory Leak for Students
A memory leak, in essence, occurs when a computer program incorrectly manages memory allocations in a way that memory which is no longer needed is not released. In object-oriented programming languages, this typically happens when an object is stored in memory but cannot be accessed by the running code.
- Causes: In general, memory leaks result from a program failing to free memory that it no longer needs. This can happen for a variety of reasons, including software defects and programming errors.
- Implications: Over time, memory leaks can impact system performance, leading to slower response times, system crashes, and in severe cases, a complete system failure.
What is a Memory Leak: Simplified Explanation for Learners
Imagine if you're a student studying in a library. You start by picking a book from the shelf and reading it at a table. Once you're done with the book, you leave it on the table instead of putting it back on the shelf. Soon, you pick up another book, read it, and leave it on the table. If you continue doing this, eventually you'll run out of space to read or even move! This situation is quite similar to how a memory leak occurs in a computer.In computer terms, the table represents the computer’s memory, books are pieces of data or applications, and you’re the program that’s using and mismanaging memory. Just like books pile up on the table, data piles up in memory. If not properly managed, this will lead to the memory (or table space) running out, causing the system to slow down or crash.
An Example of a Memory Leak in Computer Science
For a practical illustration, let's consider a simple program coded in the Java language. Here's how you might generate a memory leak:public class MemoryLeak { public static void main(String[] args) { ListIn this code, an infinite while loop keeps adding random integers to the list without ever removing them when they are no longer needed. This continuous addition of integers without removal will eventually fill up all available memory, causing a memory leak. The uncontrolled growth of the list leads to an OutOfMemoryError and will ultimately crash the program.list = new ArrayList<>(); while (true) { list.add(new Random().nextInt()); } } }
Causes of Memory Leaks
Understanding memory leaks begins with identifying their main causes. Remember, memory leaks stem from programming errors that result in chunks of memory going uninhabited or unreturned to the operating system. These errors manifest in different forms and can occur within various parts of computer architecture, making it essential to treat each instance with the right approach.Common Causes of Memory Leaks in Computer Architecture
A common cause of memory leaks involves how memory is allocated and deallocated in your machine. For instance, in C++, when you create an object using the 'new' keyword, it is your responsibility to delete it when you're done. However, if you forget to do so, a memory leak will ensue.Memory allocation in computer systems refers to the assignment of unused memory blocks to software programs that request additional memory. Deallocation, on the other hand, involves returning these memory blocks to the system for other uses once they are no longer needed.
- Unwanted retain cycles: These are situations where two or more objects reference one another in a loop. If this occurs, the memory will not be freed, triggering a memory leak.
- Lost pointers: Here, a pointer pointing to a memory location is overwritten before the memory is released, thus causing a memory leak.
- Thread locks and unused caches: In multithreaded applications, threads failing to release locks can lead to memory leaks. Similarly, caches that are no longer needed still occupying memory can also create leaks.
Tackling the Issue: How Costs of Memory Leaks Impact System Architecture
Memory leaks can have a significant bearing on your system's architecture. They can lead to slower run-times due to memory overflow, unexpected system crashes, and generally unstable operation of applications. Hence, it is crucial to address memory leaks to ensure the efficiency of your system. System architecture can be designed to combat memory leaks. For instance, let's consider a comparison table that focuses on the allocation and deallocation of memory:Java | Relies on an automatic garbage collector to manage memory allocation and deallocation, thereby reducing the chances of memory leaks. |
C++ | Requires manual allocation and deallocation, leading to a higher risk of memory leaks if not carefully managed. |
Memory Leak in Java: An In-depth Study
Java, as you might already know, is a high-level programming language that manages memory allocation for you through a component called 'Garbage Collector'. But don't be fooled into believing that you are immune from memory leaks just because you are using Java.Analysing the Causes of Memory Leak in Java
Even in a language like Java that boasts of automatic memory management, memory leaks can occur. The main reason for this is a misconception about how Java handles memory. It's easy for programmers to assume that once a memory is allocated in Java, the garbage collector will automatically deallocate it when no longer needed. But that is not entirely correct.Garbage Collection (GC) in Java is a dynamic memory management technique where unused memory blocks are identified and freed for reuse. GC operation is triggered when there is not enough space in the heap to allocate memory or according to Java's internal algorithms.
- Extended use of Static variables: Static variables or enhancements are linked with a class level instead of instance level. Thus, unless the class loader that loads this class is accessible, these static variables will neither be dismissed nor have their memory freed.
- Improper use of Listeners and other callbacks: Another common cause for Java memory leaks is the incorrect use of callbacks and listeners. If you add a listener to a class and forget to remove it, the memory taken by it won't be reclaimed leading to a memory leak.
Memory Leak in Java: Real-life Examples
Now that you've learned about the common causes of memory leaks in Java, it's time to look at some real-life examples to help reinforce your understanding. Example 1: Consider this simple program in Java:class MemoryExhaust { public static void main(String[] arguments) { try { List items = new ArrayList<>(); while(true) { items.add(new Object()); } } catch (OutOfMemoryError error) { error.printStackTrace(); } } }In this program, an ArrayList named 'items' is created. Inside the 'while' loop, new objects are continuously added to the list. However, none of the objects are removed from the list or made eligible for garbage collection, leading to an 'OutOfMemoryError'. This is a classic example of a memory leak. Example 2: Misuse of static variables.
class Example { private static HashMap map = new HashMap(); public void add(String key, String value) { map.put(key, value); } }This Java code continuously adds elements to a HashMap that's static (class level). Even when the instance of 'Example' class is no longer in use, the elements added to the map remain in memory causing a memory leak. These examples serve as a reminder that caution and best practices are required when dealing with memory usage in Java, even with its garbage collector taking care of much of it. Effective memory management in programming largely sells on diligent cleanup and understanding of how exactly the language handles memory allocation and deallocation. So, keep these insights handy to avoid memory leaks in your Java applications.
How to Prevent Memory Leaks
Memory leaks, as any seasoned programmer will tell you, can transform a high-functioning software system into a slow, inefficient, and unstable mess. Therefore, preventing these system-draining issues from occurring in the first place is incredibly important in software development. Below, you'll find detailed information on the best practices in computer architecture that help avert memory leaks and practical tips on how to sidestep them in the realm of computer science.Preventing Memory Leaks: Best Practices in Computer Architecture
Preventing memory leaks begins with an understanding of your computer's architecture and how memory is managed within it. Here are the best practices to put into action: 1. Proper Memory Allocation and Deallocation: Ensure that every block of memory you allocate in your system is properly deallocated when no longer needed. Languages like C and C++ place the burden of manual memory management squarely on the programmer's shoulders. Practice tight control over your memory allocation and deallocation to prevent leaks. 2. Avoid Retain Cycles: In Object-Oriented Programming languages, retain cycles can be responsible for more considerable memory leak issues. A retain cycle occurs when two or more objects inter-reference each other, creating a loop that prevents memory s from being freed. 3. Effective use of Caches: Cache memory, if not managed well, can also lead to memory leaks. For instance, if an application uses cache memory for speed but fails to free or refresh it appropriately when not needed, this leads to memory leaks—make sure to review and manage your caching strategy effectively. 4. Memory Profiling: A memory profiler is a tool used in software development to understand the memory usage of a program. Regularly use memory profiling tools to detect and fix memory leak issues. 5. Isolation of Processes: Design your system architecture so that processes run in isolation, each utilising a specified amount of memory. This approach reduces the impact of a memory leak on the overall system, confining it to a smaller area where it can be more easily detected and addressed.Practical Tips on How to Avoid Memory Leaks in Computer Science
Moving beyond system architecture, here are some practical programming tips to help you prevent memory leaks in your software systems. 1. Always Unsubscribe: When you subscribe to an event, always make sure to unsubscribe when the event is no longer needed. Failing to do so can result in the object not being garbage collected, leading to a memory leak. 2. Null your References: When you're done with an object, and you're confident it's not needed anymore, null it. By doing this, you make the object eligible for garbage collection, thereby freeing up the memory. 3. Be Aware of Static Variables: Static variables in a class can potentially lead to memory leaks. The static variables hold a reference to the class they are in and prevent them from being collected by the garbage collector, hence causing a memory leak. 4. Thorough Testing: Test your software under various scenarios and with different inputs to ensure it performs well under all conditions. This kind of rigorous testing will allow you to catch memory leaks before they can cause major damage. 5. Use Tools: Finally, use tools and libraries specifically designed to help find and fix memory leaks. Employing these tools can help you identify and patch up leaks more efficiently, leading to better overall system performance. By keeping these points in mind while coding, and incorporating them regularly into your programming practices, you can significantly lower your chances of introducing memory leaks into your software system. Remember, the key to avoiding memory leaks is a combination of effective system design, disciplined coding practices, and routine testing.How to Fix a Memory Leak
Memory leaks can significantly degrade the performance of your programs, leading to sluggish operation and even system failure. If you've detected a memory leak in your software system, it's essential to understand how to stride ahead to fix it. The process can seem daunting, but, armed with the right knowledge and tools, it's entirely manageable. Let's delve deeper into a step-by-step guide on fixing memory leaks and some proven fixes specifically for memory leaks in computer architecture.Step by Step Guide to Fix a Memory Leak
Fixing a memory leak starts with the correct identification of the leak. Once you have accurately detected the leak, you can proceed to resolve the situation. Here is a step-by-step guide: 1. Detecting the Memory Leak: Use system monitoring tools and application logs to track memory usage. Tools like Valgrind, LeakDiag (for .Net applications), and Java’s built-in JVM tools can assist in identifying memory leaks. 2. Isolating the Leak: Once the leak is discovered, isolate the specific areas of code where memory is not being deallocated correctly. For C++ applications, this could be any place where 'new' is used without a corresponding 'delete'. In Java applications, this typically involves objects that are no longer in use not being garbage collected because there are still references to them. 3. Repairing the Leak: After identifying the problematic areas, you can take steps to fix the memory leak. This generally involves correctly deallocating (freeing up) memory that is no longer in use. In languages with garbage collectors, you might be able to fix the problem simply by removing unnecessary references to objects. 4. Testing: Finally, after you’ve fixed the memory leak, you need to rigorously test your software again to ensure your fix worked. You can also run the same tools you used to detect the leak in the first place to verify that the leak is gone. Fixing a memory leak can sometimes require a good deal of detective work. But the relief and system performance improvement resulting from a successful repair are well worth the effort.Proven Fixes for Memory Leaks in Computer Architecture
Here are some proven fixes specifically designed for addressing memory leaks within computer architecture. 1. Manual Garbage Collection: In languages that support manual garbage collection technique, where the onus of freeing up memory primarily rests with you, ensure you liberally use the 'free' method (in C) or 'delete' (in C++) whenever you're done with an allocated block of memory. 2. Sure-fire Remediation for Retain Cycles: To break a retain cycle, which can cause significant memory leaks, try to redefine one of the relationships to be a 'weak' reference instead of a 'strong' one. 3. Controlling Overuse of Memory: In some cases, the issue is not exactly a 'leak' but rather a case of simply overusing memory, such as by repeatedly opening large files without closing them. Always try to minimise the memory footprint of your program by only using as much memory as you truly need. 4. Profit from Smart Pointers: In more advanced languages such as C++, you can often avoid many potential memory leaks by using smart pointers. These are special types of pointers that automatically deallocate the memory they point to when they go out of scope, freeing you from the burden of having to remember to free memory manually. 5. Profiling Tools: Use profiling tools to routinely monitor memory usage. These tools can provide invaluable insights into how your code is using memory and, honestly, they can sometimes spot a potential leak before it even becomes a serious problem. In conclusion, the process of fixing memory leaks requires a keen eye and a profound understanding of how your program interacts with memory. It's a critical skill for any serious programmer, and the ability to efficiently tackle memory leaks will significantly enhance the quality of your software and your credibility as a developer.Memory Leaks - Key takeaways
- Definition of a Memory Leak: A memory leak is a type of programming error resulting in chunks of memory going uninhabited or unreturned to the operating system. This may cause the system to slow down or crash.
- Memory Allocation and Deallocation: In computer systems, the assignment of unused memory blocks to software programs and returning these memory blocks back to the system for other uses once they are no longer needed. Incorrect allocation and deallocation of memory are common causes of memory leaks.
- Preventing Memory Leaks: Some best practices in computer architecture to prevent memory leaks include proper memory allocation and deallocation, avoiding retain cycles, effective use of caches, memory profiling, and isolation of processes.
- Memory Leak in Java: Despite automatic memory management through the Garbage Collector (GC), memory leaks can occur in Java. This is often due to misunderstandings about how Java handles memory and improper usage of static variables and callbacks.
- Fixing Memory Leaks: This involves steps such as detecting the memory leak using certain tools, isolating the leak, and utilizing safe coding practices to ensure the correct deallocation of memory.
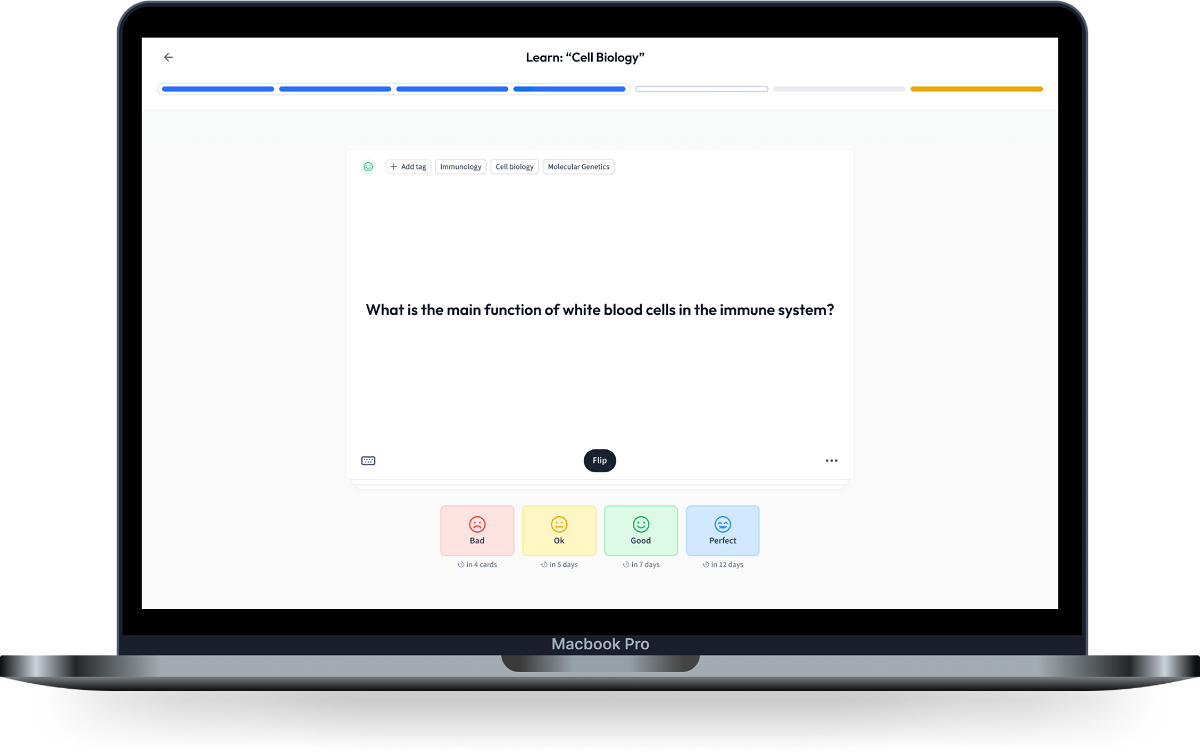
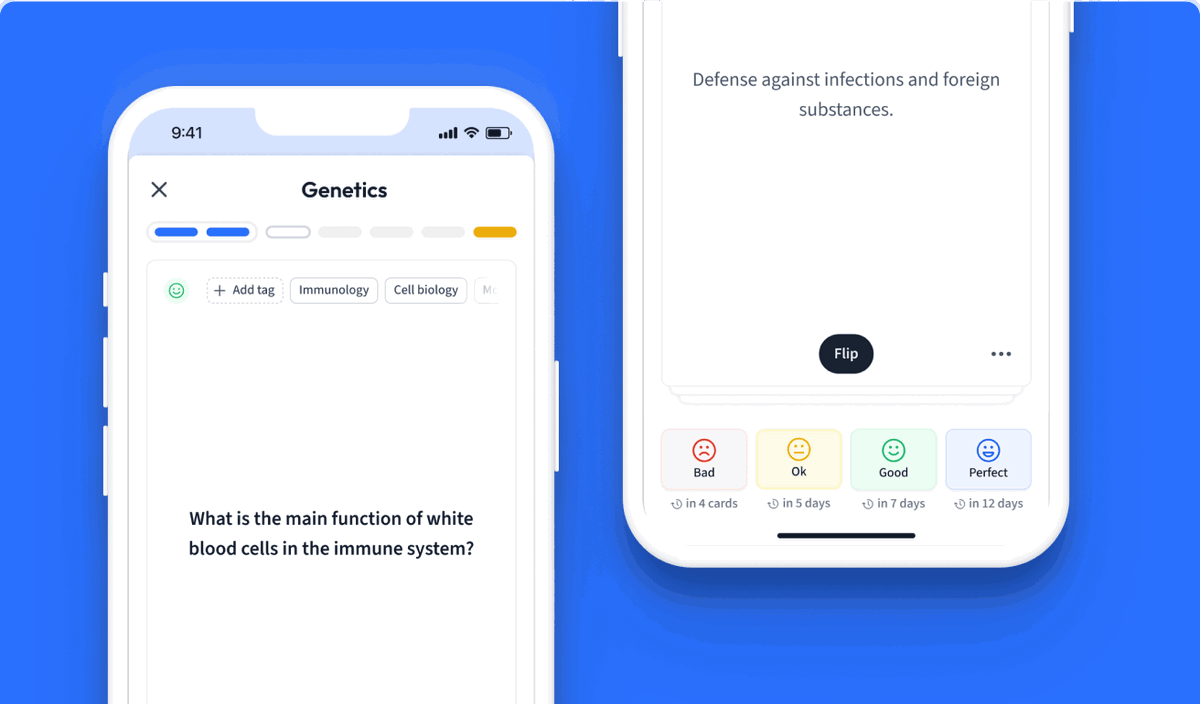
Learn with 15 Memory Leaks flashcards in the free StudySmarter app
Already have an account? Log in
Frequently Asked Questions about Memory Leaks
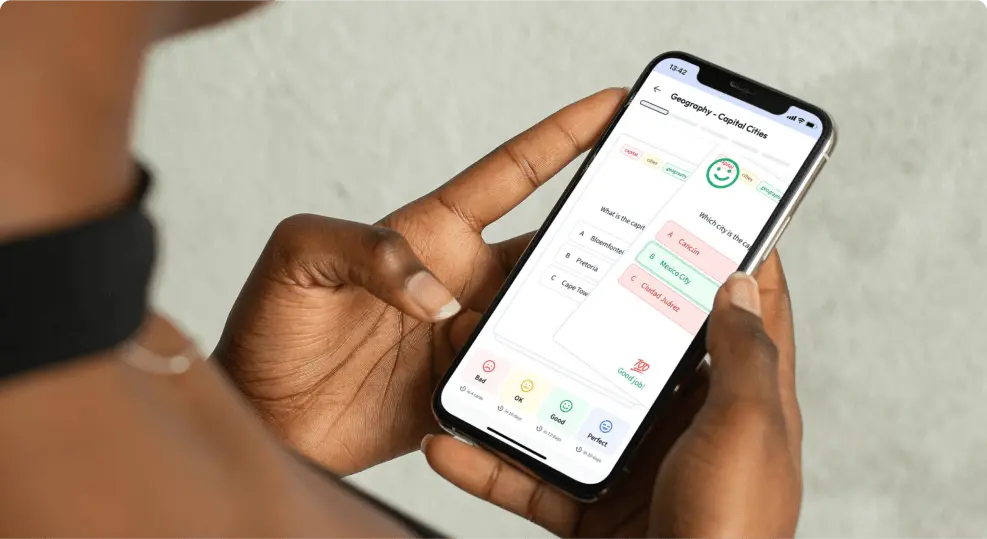
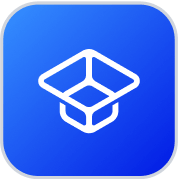
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more