Jump to a key chapter
Introduction to Access Modifiers in Computer Programming
In the world of computer programming, access modifiers play an important role in determining the visibility and accessibility of class members. By providing various levels of access control, they help developers create well-structured and secure code. In this article, you will learn about the importance of access modifiers and how they can help you achieve proper encapsulation and control over your code.
Understanding the Importance of Access Modifiers
Access modifiers are essential in any programming language as they ensure the security, flexibility, and maintainability of the code. There are several reasons why access modifiers are crucial in computer programming, including:
- They restrict the visibility of class members, ensuring that only the required components are available to other parts of the code.
- They provide encapsulation, hiding the implementation details of the code from other classes and improving the overall organization.
- They enhance code reusability and maintainability by allowing developers to make changes without affecting other parts of the system.
Now let's delve deeper into these aspects and understand how access modifiers control access to class members and achieve encapsulation.
Controlling Access to Class Members
In an object-oriented programming language, access modifiers are used to set specific rules for accessing class members such as variables, methods, and inner classes. These rules help protect the integrity of the data and prevent unauthorized manipulation of the code.
There are typically four types of access modifiers in most programming languages:
Public | Accessible from any part of the code, both within and outside the class. |
Protected | Accessible only within the class and its subclasses, but not from other unrelated classes. |
Private | Accessible only within the class; not accessible outside the class or in its subclasses. |
Default/Package-private | Accessible within the same package or namespace; not accessible outside the package. |
By using these access modifiers strategically, you can create a solid architecture for your code and prevent unauthorized access to sensitive data.
Access Modifier: A keyword used in the declaration of a class or its members to specify the visibility and accessibility level of the class or its members.
Achieving Encapsulation
Encapsulation is one of the core principles of object-oriented programming, which refers to the bundling of data with the methods that operate on that data. It allows developers to hide the implementation details of a class from other classes, making the code more modular and easier to maintain.
Access modifiers are used to achieve encapsulation by restricting access to the class members. By keeping class members private or protected, you can prevent external access and manipulation of the data and force the usage of designated methods (getter and setter methods) to interact with the data.
Here's an example of encapsulation using access modifiers:
class Employee {
private String name;
private int salary;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getSalary() {
return salary;
}
public void setSalary(int salary) {
this.salary = salary;
}
}
In the above example, the Employee class has two private fields, name and salary, which cannot be accessed directly by other classes. Instead, getter and setter methods are provided with public access, allowing other classes to interact with the data in a controlled manner.
Remember that encapsulation not only helps in controlling access to class members but also contributes to code reusability, maintainability, and adaptability. It allows developers to change the implementation details of a class without affecting other parts of the system, as long as the public interface remains the same.
Types of Access Modifiers in TypeScript and Their Use
In TypeScript, access modifiers help manage the accessibility of class members and create a clear distinction between the public and private interface of a class. TypeScript supports three types of access modifiers: public, private, and protected. Let's dive deeper into the private and public access modifiers and learn about their syntax and usage.
Private Access Modifier in TypeScript
The private access modifier is used to restrict access to class members within the class itself, making the member invisible and inaccessible from outside the class, including subclasses and instances of the class. The purpose of the private access modifier is to encapsulate specific details and allow for better separation of concerns in your code.
Syntax and Usage
To use the private access modifier in TypeScript, simply precede the declaration of the class member with the keyword "private". The following example demonstrates the syntax and usage of the private access modifier in TypeScript:
class Employee {
private name: string;
constructor(name: string) {
this.name = name;
}
public getName(): string {
return this.name;
}
}
In the example above, the "name" variable is declared as private, meaning it can only be accessed within the Employee class. The public accessor method "getName()" provides a way to access the value of the "name" variable from outside the class.
Private Access Modifier: An access modifier in TypeScript that restricts access to class members within the class definition only.
It's worth noting that if an access modifier is not explicitly specified for a class member, it is assumed to be public by default. Nonetheless, it is still considered good practice to explicitly specify the public access modifier for clarity.
Public Access Modifier in TypeScript
The public access modifier in TypeScript is used to make class members accessible from any part of the code, both inside and outside the class. Public members act as a part of the public interface of a class, enabling other classes and modules to interact with the data and functionality provided by the class.
Syntax and Usage
To use the public access modifier in TypeScript, precede the declaration of the class member with the keyword "public". However, if no access modifier is specified, the class member is considered public by default. The following example demonstrates the syntax and usage of the public access modifier in TypeScript:
class Employee {
public name: string;
constructor(name: string) {
this.name = name;
}
public setName(newName: string): void {
this.name = newName;
}
}
In the example above, the "name" variable is declared public, making it accessible from any part of the code. The public method "setName()" is also provided for updating the value of the "name" variable from outside the class.
Public Access Modifier: An access modifier in TypeScript that makes class members accessible from any part of the code, both inside and outside the class.
By using the public and private access modifiers in TypeScript, you can create a well-structured and organized codebase that separates the roles and responsibilities of different components, improving code maintainability, encapsulation, and flexibility.
Although TypeScript enforces access modifiers at compile-time, it's important to note that during runtime (in JavaScript), these access restrictions are lost, as JavaScript does not natively support the concept of private or protected members. However, using access modifiers in TypeScript still provides value from a code design and maintainability standpoint.
Understanding Class Access Modifiers in Computer Programming
Class access modifiers are fundamental elements in programming languages that help determine the visibility and accessibility of class members. They are used to control access to variables, methods, and inner classes, contributing to the security, organization, and maintainability of code in object-oriented programming languages.
Default Access Modifier and its Role
The default access modifier is the access level assigned to a class member when no specific modifier is specified. Its role in programming is to provide a baseline level of access control, defining the minimum restrictions applied to class members, making code organization easier and more maintainable. It is also known as package-private in some programming languages, such as Java.
- Restricts access to class members within the same package or namespace
- Offers a balance between security and flexibility
- Encourages modular programming and code organization
Working with Default Access in Classes
In programming languages with a default access modifier, class members without an explicit modifier are automatically given the default access level. Here's an example in Java:
package com.example;
class Employee {
String name; // default access, only accessible within the same package
...
}
Though not explicitly marked with an access modifier, the "name" member in the Employee class is only accessible to other classes within the "com.example" package.
In Python, every class member is public by default, and there is no built-in default access modifier. However, you can use a single leading underscore (e.g., "_variable") as a convention to indicate that a class member is meant to be private or protected.
Internal Access Modifier and its Benefits
The internal access modifier is utilized to restrict the visibility of class members to the current assembly or module. Primarily used in languages like C# and Swift, the internal modifier serves several benefits:
- Provides a higher level of encapsulation than default/package-private access
- Helps maintain separation of concerns, improving code organization and maintainability
- Allows you to expose functionality within an assembly while hiding implementation details from external components
Applying Internal Access in Programming
The following example demonstrates the use of the internal access modifier in C#:
internal class Employee {
internal string name;
...
}
In this example, the Employee class and the "name" member both carry the internal modifier, which means that they are accessible only within the same assembly.
In languages that lack an internal access modifier, you can achieve similar functionality using public classes and interfaces but with components separated into distinct packages or namespaces to limit accessibility.
Protected Access Modifier and its Functionality
The protected access modifier is used to restrict access to class members within the class and its subclasses, promoting encapsulation and inheritance while restricting access to unrelated classes. Using the protected modifier offers the following functionality:
- Allows subclasses to access and override base class members, enhancing code reusability
- Prevents external access, protecting sensitive data and implementation details
- Supports modularity, helping to maintain a structured and organized codebase
Implementing Protected Access in Class Members
Here's an example in Java that demonstrates the use of the protected access modifier:
class Employee {
protected String name;
protected void setName(String newName) {
name = newName;
}
}
class Manager extends Employee {
void changeName(String newName) {
setName(newName); // accessing the protected method of the base class
}
}
In this example, the setName() method and the "name" variable are both marked as protected, meaning they can only be accessed within the Employee and Manager classes. The protected access allows the Manager subclass to override the setName() method, demonstrating the value of protected access in inheritance and code reusability.
Access Modifiers Best Practices in Programming
Using access modifiers effectively is crucial in designing well-structured, secure, and maintainable code in modern programming languages. Following best practices with respect to access modifiers can help improve the overall quality and organization of your code and ensure proper encapsulation and inheritance behaviours.
Achieving Proper Encapsulation
Encapsulation is a fundamental principle in object-oriented programming that emphasizes hiding implementation details and exposing a clean interface to other classes. Access modifiers play a vital role in achieving this functionality. Using access modifiers strategically can help ensure proper encapsulation and strike a balance between security, flexibility and modularity.
Balancing Security and Flexibility
Striking the right balance between security and flexibility is crucial when using access modifiers to achieve proper encapsulation. You must consider the following key points:
- Opt for the least restrictive access level that meets the requirements of your design, limiting access to sensitive data and implementation details, without hindering functionality.
- Use private access for state data and internal helper methods, as they should be inaccessible from outside the class.
- Employ protected access for members intended for use in subclasses or when necessary to facilitate inheritance.
- Utilize public access for members intended to form part of the class's external interface, ensuring ease of interaction from other parts of the code.
- Consider the default access modifier (package-private or internal) when exposing members within the same package or assembly, without allowing external access.
By adopting these principles, you can achieve proper encapsulation and create a well-organized and maintainable code structure while allowing for secure and flexible interactions between components.
Applying Access Modifiers in Inheritance
Inheritance is another core concept of object-oriented programming that involves creating new classes by extending existing ones. Access modifiers play an important role in controlling the access rights of derived classes to members of their base class, contributing to the encapsulation and abstraction of your code.
Preserving Abstraction in Derived Classes
To maintain the integrity of the base class's abstraction while designing derived classes, consider the following aspects:
- Restrict access to private members in the base class to ensure they are only accessible within that class. This prevents potential misuse or unintentional modifications from derived classes, while preserving encapsulation.
- Use protected access for members that are intended to be reused or overridden in subclasses, allowing them to access and manipulate these members while still limiting their visibility from unrelated classes.
- Use public access for members that are meant to be exposed as part of the base class's interface, allowing derived classes to inherit and extend the functionality easily while keeping a consistent public interface.
- Avoid exposing unnecessary implementation details of the base class through public or protected access, as it can lead to tight coupling and hinder maintainability and modularity.
By following these guidelines, you can maintain the abstraction in derived classes and ensure proper encapsulation, flexibility, and maintainability in your code.
Access Modifiers - Key takeaways
Access Modifiers: keywords that control access to class members, aiding in encapsulation and code organization.
Access Modifiers in TypeScript: three types - private, public, and protected access modifiers, used to control visibility and accessibility of class members.
Class Access Modifiers: default, internal, and protected access modifiers, used to manage class member visibility and accessibility in different programming languages.
Default Access Modifier: assigned to class members when no specific modifier is specified, restricting access within the same package or namespace.
Encapsulation and Inheritance: achieved through strategic use of access modifiers, promoting modularity, security, and maintainability of code.
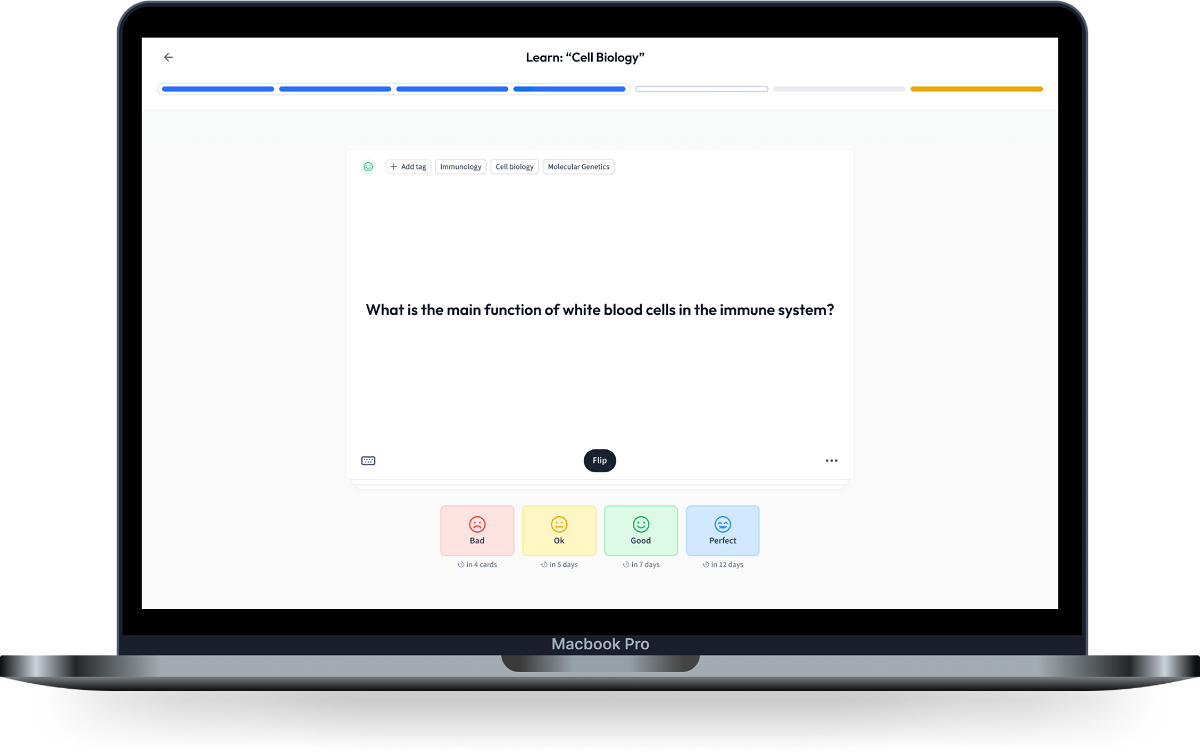
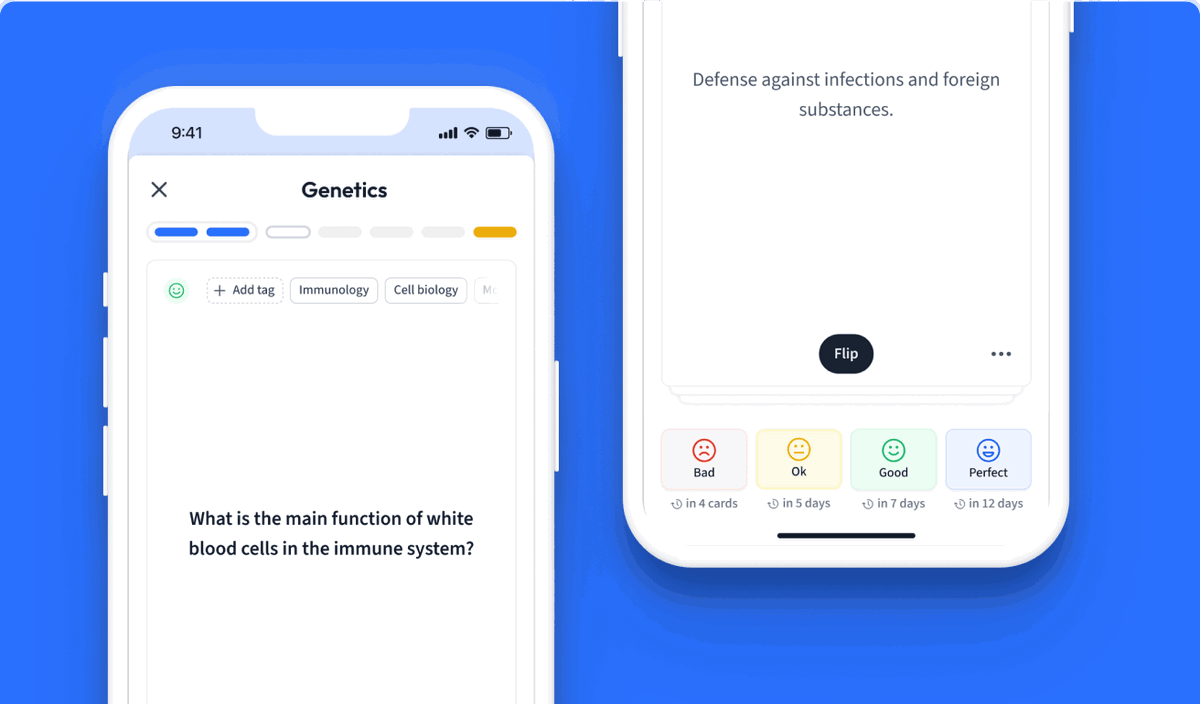
Learn with 16 Access Modifiers flashcards in the free StudySmarter app
Already have an account? Log in
Frequently Asked Questions about Access Modifiers
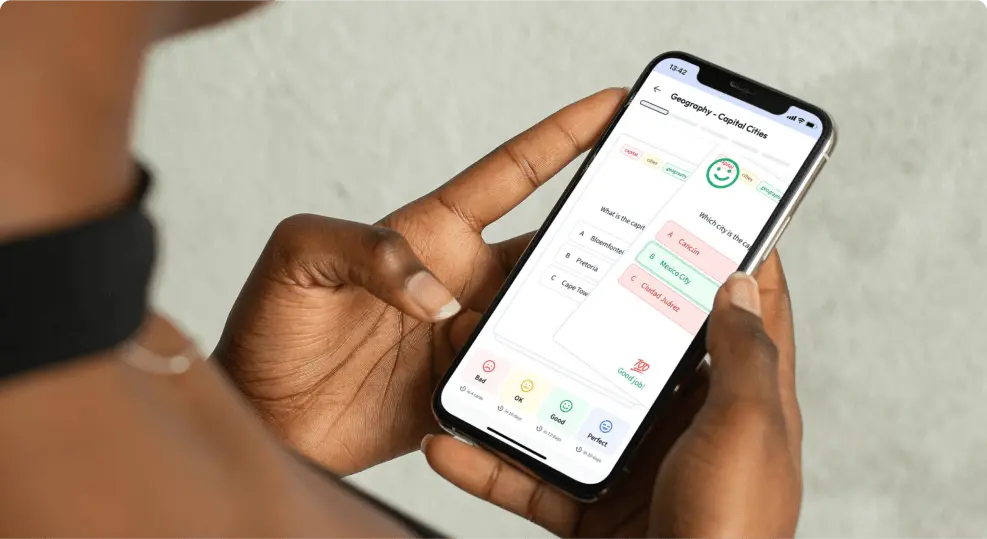
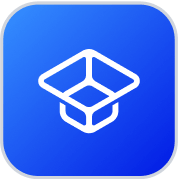
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more