Jump to a key chapter
Basic Concept behind the AND Operator in C
The AND Operator in C is a fundamental concept in the realm of computer programming. It comes under the category of logical operators and is widely employed for performing comparisons between two variables or values. By following the rules of binary logic, the AND Operator compares the bits of two given operands and returns a true or false value as a result. The basic principle of the AND Operator can be represented as: if both input values (bits) are true (1), then the output is true (1); otherwise, the output is false (0).
AND Operator: A logical operator in programming languages like C that compares two operands bit by bit, following the rules of binary logic to produce a true (1) or false (0) value.
How the AND Operator in C works with operands
In the context of the C programming language, the AND Operator is symbolized by the ampersand character (&). To better understand how the AND Operator in C works with operands, let's dive deeper into a step-by-step explanation:
- Firstly, the operands' values are converted into their respective binary forms.
- Each operand's binary representation is then aligned bit by bit.
- For each set of aligned bits, an AND operation is performed, which follows the aforementioned logic (1 if both bits are 1, and 0 otherwise).
- At last, the result obtained after performing the AND operation on all the bits is converted back into its decimal equivalent.
Example: Let's take the operands 10 and 5. When applying the AND Operator in C, the following steps occur:
- Convert the values to binary: 10 (in Decimal) = 1010 (in Binary), 5 (Decimal) = 0101 (Binary).
- Align the binary representations: 1010 and 0101.
- Perform the AND operation: 0000.
- Convert the result back to decimal: 0000 (Binary) = 0 (Decimal).
So, in this example, 10 & 5 returns 0 as the result.
Differentiating the AND Operator from other Logical Operators in C
Apart from the AND Operator, there are other logical operators in C, such as the OR Operator and the NOT Operator. Each operator serves a specific purpose and follows a distinct logic. To gain a comprehensive understanding of their differences, let's explore each operator and their respective behaviours.
Contrast between AND Operator and OR Operator in C
The primary distinction between the AND Operator and the OR Operator in C is the way they operate on the given operands' bits. The OR Operator (|) compares the bits of two operands and returns 1 if any of the two bits is 1; otherwise, it returns 0. This behaviour is more inclusive than the AND Operator, which requires both bits to be 1 for the output to be 1.
OR Operator: A logical operator in programming languages like C that compares two operands bit by bit and returns 1 if at least one of the bits is 1, or 0 if both bits are 0.
Example: Let's take the same operands as before, 10 and 5. When applying the OR Operator in C, the following steps occur:
- Convert the values to binary: 10 (in Decimal) = 1010 (in Binary), 5 (Decimal) = 0101 (Binary).
- Align the binary representations: 1010 and 0101.
- Perform the OR operation: 1111.
- Convert the result back to decimal: 1111 (Binary) = 15 (Decimal).
So, in this example, 10 | 5 returns 15 as the result.
Note: Another crucial logical operator in C is the NOT Operator (~), which inverts the bits of single operand. The NOT Operator is a unary operator, meaning it only operates on one operand, unlike the AND and OR Operators, which require two operands for their operations.
Implementing the AND Operator in C
To properly implement the AND Operator in your C programs, it's crucial to follow the correct syntax. The AND Operator is defined by the ampersand symbol (&) and is placed between the two operands being compared. Here's a rundown of how the syntax for the AND Operator works:
- Specify the variable or constant for the first operand.
- Insert the AND Operator (&) symbol.
- Provide the variable or constant for the second operand.
- Enclose the expression in a suitable statement or condition, such as an if statement.
It is also possible to place the result of the AND operation in a variable by incorporating an assignment statement. Keep in mind that variable types must be compatible to store the result of the AND operation.
Example: Here is an example demonstrating the syntax for using the AND Operator in C:
int result, a = 12, b = 7; result = a & b;
In this example, 'a' (12) and 'b' (7) are the operands, '&' is the AND Operator, and 'result' stores the result of the AND operation between 'a' and 'b'. After executing the code, the 'result' variable will hold the value 4, which is the outcome of the AND operation (12 & 7).
Writing a Sample AND Operator Program
To illustrate how the AND Operator is put into practice in a C program, let's walk through a sample program. This program will acquire two integer inputs from the user and perform an AND operation on these inputs using the AND Operator:
#includeint main() { int num1, num2, result; printf("Enter the first number: "); scanf("%d", &num1); printf("Enter the second number: "); scanf("%d", &num2); result = num1 & num2; printf("The result of the AND operation is: %d\n", result); return 0; }
In this program, we use the 'printf()' function to prompt the user for two integer values, which are then stored in the 'num1' and 'num2' variables. The AND Operator (&) is then employed to perform the AND operation on these two numbers, and the result is stored in the 'result' variable. Finally, the 'printf()' function prints the outcome of the AND operation. After executing the code, if the user enters 12 and 7 as the input values, the result will be 4, since 12 & 7 = 4 in binary logic.
Practical Scenarios for Applying the AND Operator in C
The AND Operator can be employed in a variety of contexts within a C program. Some practical scenarios for using the AND Operator in C include, but are not limited to:
- Bit masking and bitwise manipulation
- Testing specific bits within a numerical value
- Control registers for peripheral devices
- Encoding and decoding functions
- Creating custom puzzles or game logic
- Implementing efficient algorithms in cryptography or data compression
In each scenario, the AND Operator allows the programmer to access, examine, manipulate and control individual bits within the chosen operands. By doing so, it enables efficient and precise control over the execution of various tasks and operations within a C program.
Examples of Using the AND Operator in Real-Life Contexts
Let's explore some real-life examples that demonstrate the importance and applicability of the AND Operator in C:
Example 1: Bit Masking for Extracting Specific Bits
#includeint main() { int num = 0b11001100; int mask = 0b00001111; int extracted_bits; extracted_bits = num & mask; printf("The extracted bits are: %d\n", extracted_bits); return 0; }
In this example, we first define a number 'num' in binary (11001100) and a mask 'mask' (00001111). We then use the AND Operator to extract the last 4 bits from 'num'. The result of the AND operation (11001100 & 00001111) will be 00001100 (12 in decimal), which is then stored in the 'extracted_bits' variable.
Example 2: Testing a Specific Bit in a Number
#includeint main() { int test_bit = 5; int num = 42; // 0b00101010 if (num & (1 << test_bit)) { printf("Bit %d is set.\n", test_bit); } else { printf("Bit %d is not set.\n", test_bit); } return 0; }
In this example, we want to test whether the 5th bit is set in the given number (42 in decimal, 0b00101010 in binary). We first create a mask by left-shifting the number 1 by 'test_bit' positions (1 << 5). The AND Operator is then employed to check if this bit is set or not. If the result of the AND operation is nonzero, it means that the bit is set; otherwise, it is not set. In this case, the 5th bit of 42 is set (since 0b00101010 & 0b00100000 = 0b00100000), and therefore the output will be "Bit 5 is set".
Examples of Using the AND Operator in C
Let's look at a straightforward example of using the AND Operator in C programming. This example compares two integer numbers, illustrates how the operator is used, and provides an insight into how the output is derived. Analyzing such simple examples aids in understanding the basics of the AND Operator, making it easier to comprehend its application in more complex settings.
Consider the following C program that takes two integers, A and B, and performs an AND operation on them using the AND Operator (&):
#includeint main() { int A = 15; // Binary: 0b001111 int B = 9; // Binary: 0b01001 int result; result = A & B; printf("The result of the AND operation is: %d\n", result); return 0; }
The output of this program will be 9, as the AND operation on the binary values of A (0b001111) and B (0b01001) returns 0b01001, which is equivalent to 9 in decimal.
Demonstrating How to Apply the AND Operator in Various Conditions
In C programming, you can use the AND Operator in numerous circumstances to perform complex logical operations. It's essential to comprehend how the AND Operator is applied to different conditions and scenarios. Examples discussed below will help you better understand the application of the AND Operator in C.
Example 1: AND Operator with a conditional statement
#includeint main() { int num1 = 12; int num2 = 5; if (num1 & num2) { printf("The condition evaluates to true.\n"); } else { printf("The condition evaluates to false.\n"); } return 0; }
In this program, the AND Operator is used to compare the binary bits of num1 (12) and num2 (5). If the resulting output of the AND operation is nonzero, the if condition evaluates to true and the statement within the if block is executed. Else, the condition evaluates to false and the statement within the else block is executed. In this case, since 12 & 5 = 4 (not equal to 0), the output will be "The condition evaluates to true."
Example 2: Combining AND Operator with other bitwise operators in C
#includeint main() { int A = 15; // Binary: 0b001111 int B = 9; // Binary: 0b01001 int AND_result = A & B; int OR_result = A | B; int XOR_result = A ^ B; printf("AND Result: %d\n", AND_result); printf("OR Result: %d\n", OR_result); printf("XOR Result: %d\n", XOR_result); return 0; }
This program demonstrates how the AND Operator can be combined with other bitwise operators, like OR (|) and XOR (^), to perform multiple bitwise operations on a pair of operands. The output of this program will be:
AND Result: 9 OR Result: 15 XOR Result: 6
AND Operator Involving Bit Manipulation in C
In C programming, the AND Operator plays a crucial role in various bitwise manipulation techniques. Understanding how the AND Operator works with bit manipulation can help unlock a wide range of applications, from efficient integer manipulation and data extraction to error detection and correction in communication systems.
Use of AND Operator in C for Altering Binary Values
When it comes to altering binary values in C, the AND Operator offers great precision and flexibility. By combining the AND Operator with other bitwise techniques, programmers can achieve desired outcomes with minimal efforts. Let's consider some examples to showcase the use of the AND Operator in altering binary values.
Example 1: Clearing specific bits using the AND Operator
#includeint main() { int num = 175; // Binary: 0b10101111 int clear_mask = 0xF3; // Binary: 0b11110011 int cleared_bits = num & clear_mask; printf("The cleared bits result: %d\n", cleared_bits); return 0; }
In this example, the AND Operator is used to clear (unset) specific bits in a number by creating a mask with the desired bits set to 0. The output of this program will be 147, as the AND operation on the binary values of num (0b10101111) and clear_mask (0b11110011) returns 0b10010011, which is equivalent to 147 in decimal.
Example 2: Flipping a specific bit using the AND Operator and XOR Operator
#includeint main() { int num = 175; // Binary: 0b10101111 int flip_bit = 2; int flipped_num = num ^ (1 << flip_bit); printf("The number after flipping bit %d: %d\n", flip_bit, flipped_num); return 0; }
In this example, we first create a mask by left-shifting the number 1 by 'flip_bit' positions (1 << 2). We then use the AND Operator in conjunction with the XOR operator to flip the specific bit in the number. The output of this program will be 179, as flipping the second bit of num (175) results in the binary number 0b10110011, which is equivalent to 179 in decimal.
These examples underline the versatility of the AND Operator in C programming when it comes to bit manipulation. By understanding the AND Operator's use cases in different scenarios, you can further expand your knowledge and apply this crucial tool to a wide range of programming challenges.
Logical Operators: The AND Operator in C
Logical operators play a vital role in computer programming, and the AND Operator in C is no exception. It is one of the fundamental operators used for performing comparisons between two variables or values and helps make complex decisions. Moreover, it helps simplify and optimize codes, ensuring that the desired output is obtained efficiently and precisely.
Importance of Logical Operators in C Programming
Logical operators are essential components of C programming due to their ability to examine and manipulate individual bits within the chosen operands. This capability enables efficient and precise control over the execution of various tasks and operations within a C program. Some key benefits of logical operators in C programming include:
- Performing bitwise manipulation and bit masking
- Testing specific bits within a numerical value
- Facilitating control and access to peripheral device registers
- Enabling encoding and decoding functions
- Improving algorithms in cryptography or data compression
- Implementing custom puzzles or game logic
Role of the AND Operator in Making Complex Decisions
The AND Operator in C is instrumental in making complex decisions, as it can analyze multiple conditions simultaneously and provide accurate outcomes based on the given inputs. It accomplishes this by performing a bitwise AND operation on the binary representations of its operands, adhering to a set logic which returns true (1) if both input bits are true (1) and false (0) otherwise.
Here are some instances in which the AND Operator can be utilized to make complex decisions:
- Analyzing conditions that require multiple factors to be true simultaneously
- Implementing complex control structures such as nested conditional statements
- Developing intricate algorithms in various domains, including data security and encoding
- Evaluating whether specific bit patterns are present in given values
- Processing and manipulating binary data efficiently
Comparison between AND Operator and Other Logical Operators in C
While the AND Operator is popular in C programming, there are other logical operators like the OR Operator and the NOT Operator, each serving a unique purpose and following a distinct logic. Distinguishing between these operators is crucial for selecting the appropriate one depending on the problem at hand. Let's compare the AND Operator with other logical operators in C:
Situations Where the AND Operator is More Suitable in C
In certain situations, the AND Operator proves to be more suitable when compared to other logical operators. Understanding these scenarios can help programmers choose the appropriate operator to make their code more effective and efficient. Some situations where the AND Operator may be advantageous include:
- Comparing multiple conditions that must be simultaneously true: The AND Operator is ideal when analyzing conditions where all contributing factors must be true for the overall result to be true.
- Extracting specific sets of bits: The AND Operator can be used to extract particular bits from a numerical value by applying a code mask.
- Simplifying complex decision structures: Using the AND Operator can reduce the number of nested if statements and make the code easier to read and maintain.
- Performing calculations that need bitwise logic: The AND Operator is perfect for cases where bitwise logic is required, such as access control, encoding, or decoding.
In contrast, the OR Operator is typically more suitable for scenarios where only one (or more) of the conditions needs to be true. Similarly, the NOT Operator is ideal for inverting individual bits, which is useful for tasks such as bit toggling or error detection. By understanding the strengths and weaknesses of each logical operator, programmers can make more informed decisions and create efficient C programs tailored to specific needs.
Best Practices for Using the AND Operator in C
To make the most of the AND Operator in C programming and ensure that your code remains efficient, it's essential to follow programming best practices. Observing these practices helps improve code readability, maintainability, and performance, enabling you to develop robust and effective applications in a wide range of contexts.
Writing Efficient Code with the AND Operator in C
When using the AND Operator in C, it is crucial to write efficient code that maximizes performance and minimizes resource usage. Implementing efficient code involves several factors, such as identifying the optimal logical operators for specific tasks, simplifying expressions, and avoiding common mistakes. By adhering to these guidelines, you can create concise and effective C programs.
Tips for Simplifying AND Operator Expressions in C
To improve the readability and performance of your code, consider the following tips for simplifying AND Operator expressions in C:
- Make use of parentheses to ensure correct operator precedence and enhance code readability.
- Opt for short-circuit evaluation, where possible, to avoid executing unnecessary logic.
- Replace nested if statements with the AND Operator to reduce code complexity and improve maintainability.
- Utilise constants and macros to represent masks and other bitwise values, making your code more interpretable.
- Consider using bitwise shifts and other operators to further simplify your AND Operator expressions and operations.
Following these suggestions can help to create cleaner, more efficient, and more manageable code when using the AND Operator in C programming.
Avoiding Common Mistakes while Implementing the AND Operator in C
When working with the AND Operator in C, it's important to avoid common pitfalls that can lead to misinterpretations, incorrect outcomes, or inefficient code execution. By being aware of potential mistakes and implementing proper testing and debugging techniques, you can ensure the successful execution and desired functionality of your C programs.
Proper Testing and Debugging of AND Operator Conditions in C
To effectively test and debug AND Operator conditions in C, consider adopting the following strategies:
- Use test-driven development (TDD) to write tests for each function or operation that involves the AND Operator, and ensure that tests cover both positive and negative cases.
- Verify variable types, masks, and the order of operations to prevent arithmetic or logical errors that could lead to inaccurate results or unintended behaviour.
- Employ debugging tools, such as breakpoints and step execution, to inspect the state of variables, expressions, and bitwise operations at specific points during execution.
- Implement proper error handling and logging mechanisms to identify and resolve any issues that may arise during runtime.
- Regularly review and refactor your code to maintain high-quality standards and stay abreast of possible bugs and issues.
By applying these testing and debugging practices, you can avoid common mistakes and ensure a smooth implementation of the AND Operator in your C programs, helping create robust, precise, and reliable applications.
AND Operator in C - Key takeaways
Understanding the AND Operator in C: A logical operator that compares two operands bit by bit, following the rules of binary logic to produce a true (1) or false (0) value.
Implementing the AND Operator in C: Use the ampersand symbol (&) between two operands, follow the correct syntax, and enclose the expression in a suitable statement or condition.
Examples of Using the AND Operator in C: Employed in various scenarios, such as bit masking, testing specific bits, encoding and decoding functions, and implementing game logic.
Guidelines for Using the AND Operator in C: Write efficient code, simplify expressions, avoid common mistakes, and properly test and debug AND Operator conditions.
Logical Operators: The AND Operator in C is among other logical operators, such as the OR Operator and the NOT Operator, each serving a unique purpose and following a distinct logic.
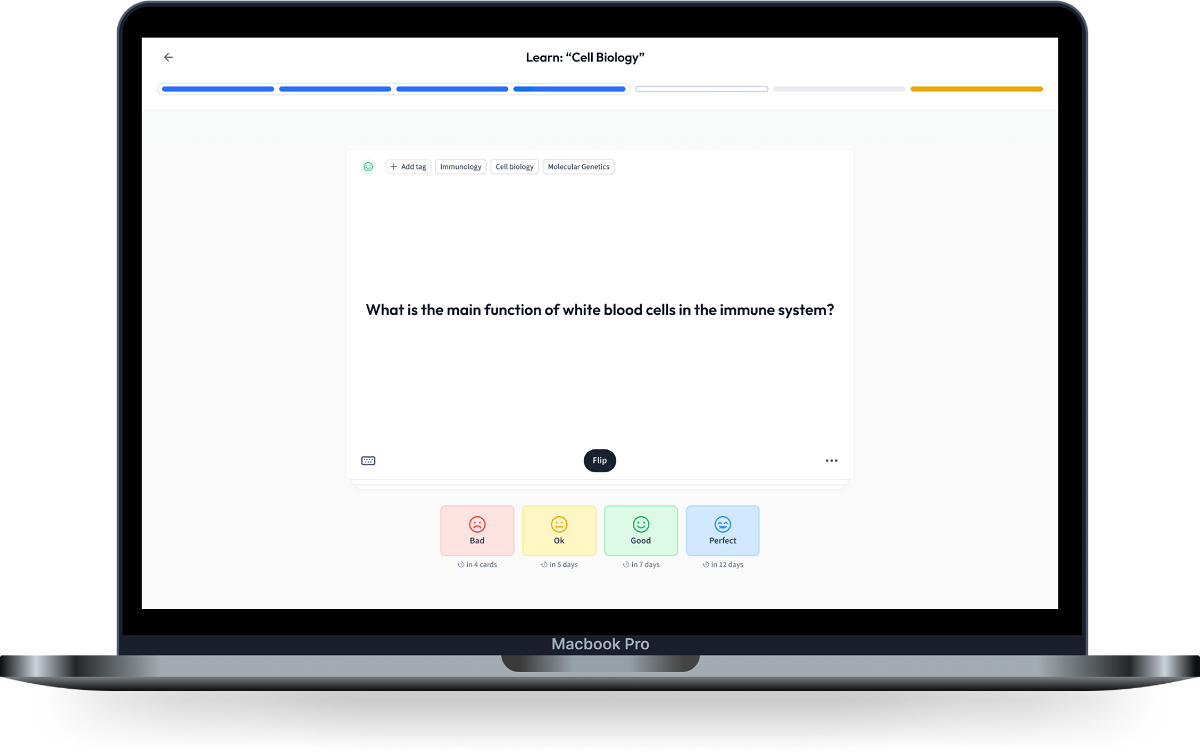
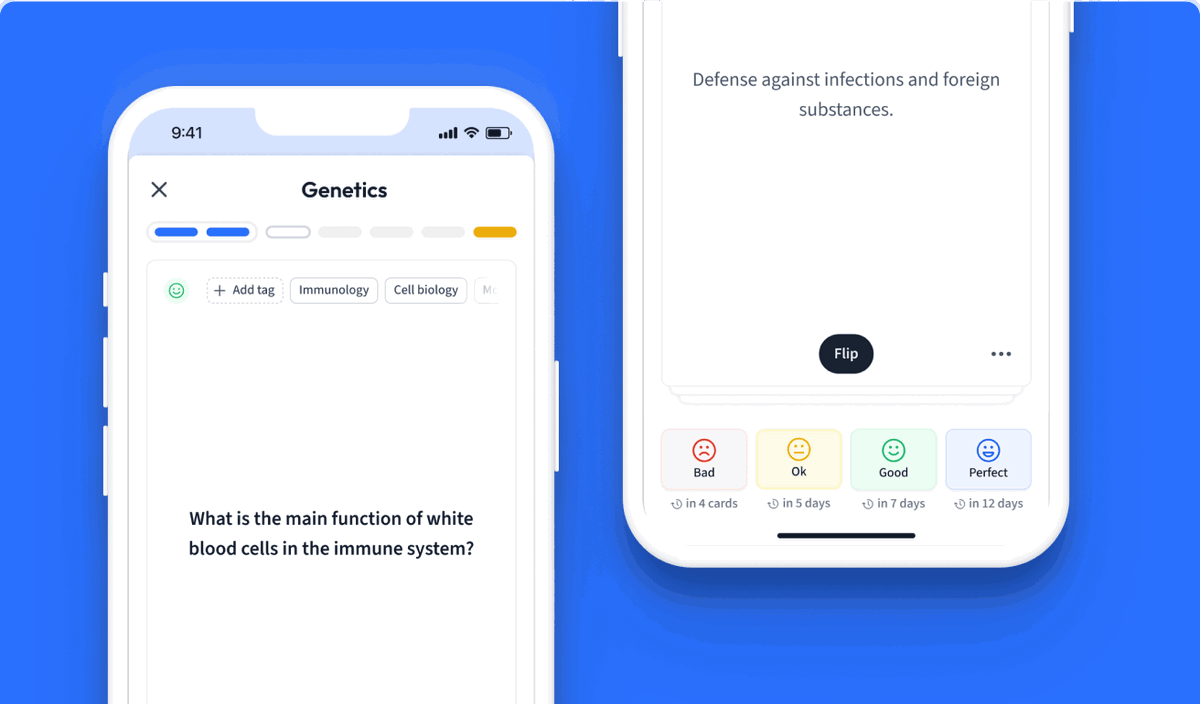
Learn with 9 AND Operator in C flashcards in the free StudySmarter app
Already have an account? Log in
Frequently Asked Questions about AND Operator in C
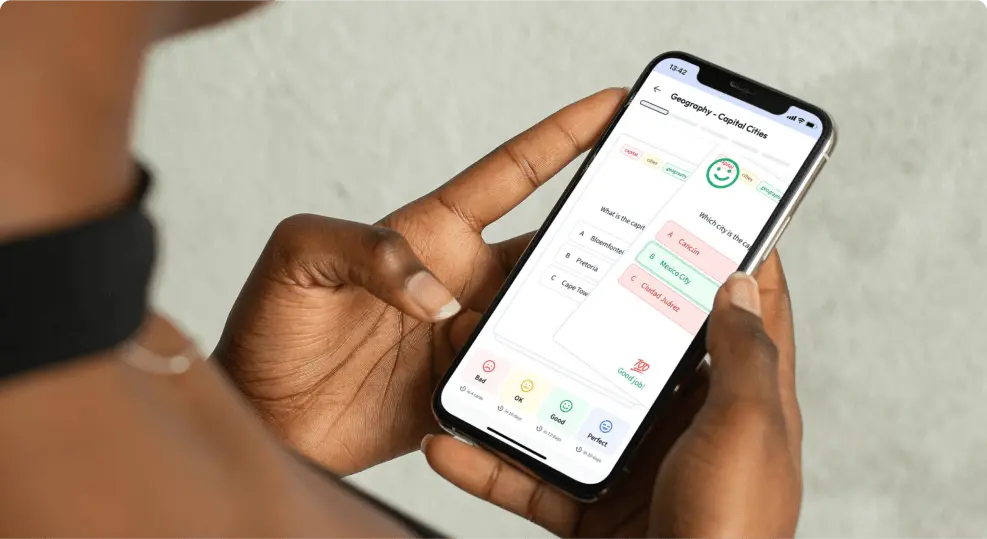
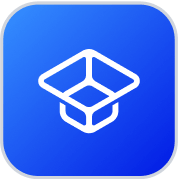
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more