Jump to a key chapter
Introduction to Break in C
When learning C programming, you'll come across various control statements that help you write efficient and flexible code. One such widely used control statement is the break statement, which provides you with the ability to exit a loop or switch statement prematurely if a specific condition is met.
Understanding the Break Function in C
In C programming, the break statement is used to exit a loop structure, such as 'for', 'while', or 'do-while', and switch statements, once a specified condition is satisfied. It's particularly useful when you need to stop the execution of a loop without waiting for the loop condition to become false.
Break Statement: A control statement in C programming that allows you to exit a loop or switch statement when a specific condition is met.
The break statement can be beneficial in situations when:
- You need to exit a loop when a specific value is found,
- There is an error or exception condition that needs to be handled, or
- You want to skip the remaining iterations of a loop if a certain criterion is met.
Here is a basic example showcasing the use of the break statement in a for loop:
#include
int main() {
for (int i = 1; i <= 10; i++) {
if (i == 5) {
break;
}
printf("%d\n", i);
}
return 0;
}
In this example, the loop is supposed to iterate from 1 to 10, and print the values. However, the break statement will exit the loop when the given condition, i.e., i==5, becomes true. As a result, only the values 1 to 4 will be printed, and the loop will terminate early.
Note: One key limitation of the break statement is that it can only be used within a loop or switch statement. If placed outside these structures, the compiler will produce an error.
Importance of the Break Function in C Programming
The break function holds significant importance in C programming because it:
- Helps create more efficient and optimized code,
- Facilitates the handling of error conditions and exceptions,
- Eliminates the need for additional variables and nested if statements to control the loop execution, and
- Improves overall code readability and maintainability.
Using break statements wisely can lead to reduced execution time and increased code efficiency. However, it is equally important to avoid overusing the break statement, as it may lead to unintended consequences, like skipping important steps in the code.
In conclusion, understanding the break statement in C programming is crucial in order to manage loop execution and achieve efficient, clean, and readable code. Using break wisely can help you achieve better control over your program flow and make your code easier to understand and maintain.
Break Use in C: Various Scenarios
In this section, we will discuss various scenarios where the break statement can be utilised in C programming, such as in single loops, nested loops, and in combination with the continue statement. Understanding these scenarios will help you master the use of break statements in diverse programming situations.
Break in C: Single Loop
As discussed previously, the break statement is employed in C programming to exit a loop or switch statement when a specific condition is met. This can be particularly useful for terminating a single loop structure like 'for', 'while', or 'do-while' loops, without having to iterate through all the scheduled repetitions.
Consider the following single loop scenarios:
- Searching for a specific value in an array,
- Reading text input until a certain keyword is encountered, and
- Monitoring sensor data and stopping data collection when a threshold is reached.
#include
int main() {
int target = 7;
int values[] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
for (int i = 0; i < 10; i++) {
if (values[i] == target) {
printf("Target value (%d) was found at index %d\n", target, i);
break;
}
}
return 0;
}
In the example above, we have an array of integer values in which we search for our target value. The moment our target value is found, the break statement is executed, and the loop terminates earlier, saving resources and time.
Break in C Nested Loop
When working with nested loops in C programming, using break statements can help you terminate the execution of inner loops, based on specific conditions. However, it is important to understand that the break statement will only exit the innermost loop it is placed in. To exit multiple levels of nested loops, you may need to use additional control variables or conditional statements.
Here's an example illustrating the use of break in a nested loop:
#include
int main() {
for (int outer = 0; outer <= 5; outer++) {
for (int inner = 0; inner <= 5; inner++) {
printf("(%d, %d)\n", outer, inner);
if (inner == 2 && outer == 4) {
break;
}
}
}
return 0;
}
In the example above, the break statement is placed inside the 'if' condition, which is evaluated within the inner loop. Once the specified condition (inner == 2 && outer == 4) is met, the break statement is executed, and the inner loop is terminated. However, the outer loop will continue to execute.
Break and Continue in C
In addition to break, the 'continue' statement is another useful control statement used in loops. While the break statement helps you terminate a loop early, the continue statement enables you to skip the current iteration in the loop and move on to the next one, based on certain conditions. It can be employed both in single loops and nested loops, similarly to the break statement.
Here's an example that demonstrates the use of the break and continue statements together:
#include
int main() {
for (int i = 1; i <= 10; i++) {
if (i % 2 == 0) {
continue;
}
if (i == 7) {
break;
}
printf("%d\n", i);
}
return 0;
}
In this example, the loop iterates from 1 to 10. The continue statement skips even numbers, while the break statement terminates the loop once the value of i reaches 7. Consequently, only the odd numbers between 1 and 7 will be printed in the output.
Understanding the use of the break, and continue statements, in various scenarios such as single loops, nested loops, and in combination can greatly improve your mastery of loop control mechanisms in C programming. This will help you create streamlined and efficient code that caters to specific requirements and optimises the overall execution time of your programs.
Break in C Explained: Real-Life Examples
Applying the break statement in real-life programming scenarios can help you solve complex problems with ease and write efficient code. In this section, we will discuss some case studies involving the use of break statements in C programming and delve into the analysis of break statements within loops and switches.
Break in C: Case Studies
Now let's explore some real-life examples of using the break statement in C programming for various applications, such as fetching real-time data, managing user input, and utilising it within nested loops.
1. Fetching Real-Time Data: Consider a program that fetches real-time data from a server and processes the information. If the server sends a specific "stop" command or an error is encountered, the program should stop fetching any more data. A break statement can be used to exit the loop that fetches the data in such a scenario.
while (1) {
data = fetchDataFromServer();
if (data == "stop" || isError(data)) {
break;
}
processData(data);
}
In this example, the break statement will exit the loop if the "stop" command is received or an error situation occurs while fetching data from the server.2. Managing User Input: Suppose you are writing a program that receives user input and performs certain operations accordingly. If the user enters a particular keyword, for instance, "exit", the program should terminate immediately. You can use the break statement to exit the loop handling user input as shown below:
char userInput[100];
while (1) {
printf("Enter a command: ");
scanf("%s", userInput);
if (strcmp(userInput, "exit") == 0) {
break; // Break the loop and terminate the program
}
performOperation(userInput);
}
In this example, when the user enters "exit", the break statement will be executed, and the program will terminate.3. Nested Loops: Let's say you have a program that navigates through a grid of cells until it finds a target element. A break statement can be employed to exit the inner loop when the target is found, without completing the remaining iterations.
int i, j;
int target = 42;
bool targetFound = false;
for (i = 0; i < numberOfRows; i++) {
for (j = 0; j < numberOfColumns; j++) {
if (grid[i][j] == target) {
targetFound = true;
break;
}
}
if (targetFound) break; // Break the outer loop
}
printf("Target found at cell (%d,%d).\n", i, j);
In the nested loop example above, the break statement terminates the inner loop when the target element is found, and utilising 'targetFound' allows the program to exit the outer loop as well.Analysing Break Statements within Loops and Switches
By examining the use of break statements within loops and switches in the C programming language, we can better understand its impact on the structure and execution of code. The following points highlight the crucial factors associated with using break statements in loops and switches:
- Code Readability and Maintainability: Employing break statements can make the code more readable and maintainable by reducing the complexity of loop control structures and removing the need for additional variables or nested if statements.
- Optimised Code Execution: Break statements can help minimise the number of iterations a loop or switch must perform, thus decreasing the execution time of a program, especially when dealing with large datasets or complex computations.
- Error Handling and Exception Management: In cases where you need to terminate the execution of a loop or switch based on an error condition or specific exception management criteria, break statements can be a powerful tool to achieve the desired outcome.
- Proper Usage and Limitations: Using break statements judiciously is necessary to avoid any unintended consequences. Be mindful that a break statement only exits the innermost loop or switch it is placed in, and should never be used outside loop and switch structures, as it will lead to compilation errors.
Overall, understanding and employing break statements effectively within loops and switches can lead to cleaner, more efficient, and maintainable code in C programming. By analysing real-life examples and case studies, you can further enhance your expertise in using break statements and other control structures to create elegant and high-performance applications.
Break in C - Key takeaways
Break in C: A control statement used to exit loop or switch statements when a specific condition is met.
Importance: It helps create efficient, optimized code and manages error conditions and exceptions.
Scenarios: Break statements can be used in single loops, nested loops, and combined with continue statements.
Nested Loop Break: Break exits only the innermost loop and may require additional variables or conditions to exit outer loops.
Break and Continue in C: While the break statement terminates a loop, the continue statement skips the current iteration and moves on to the next one.
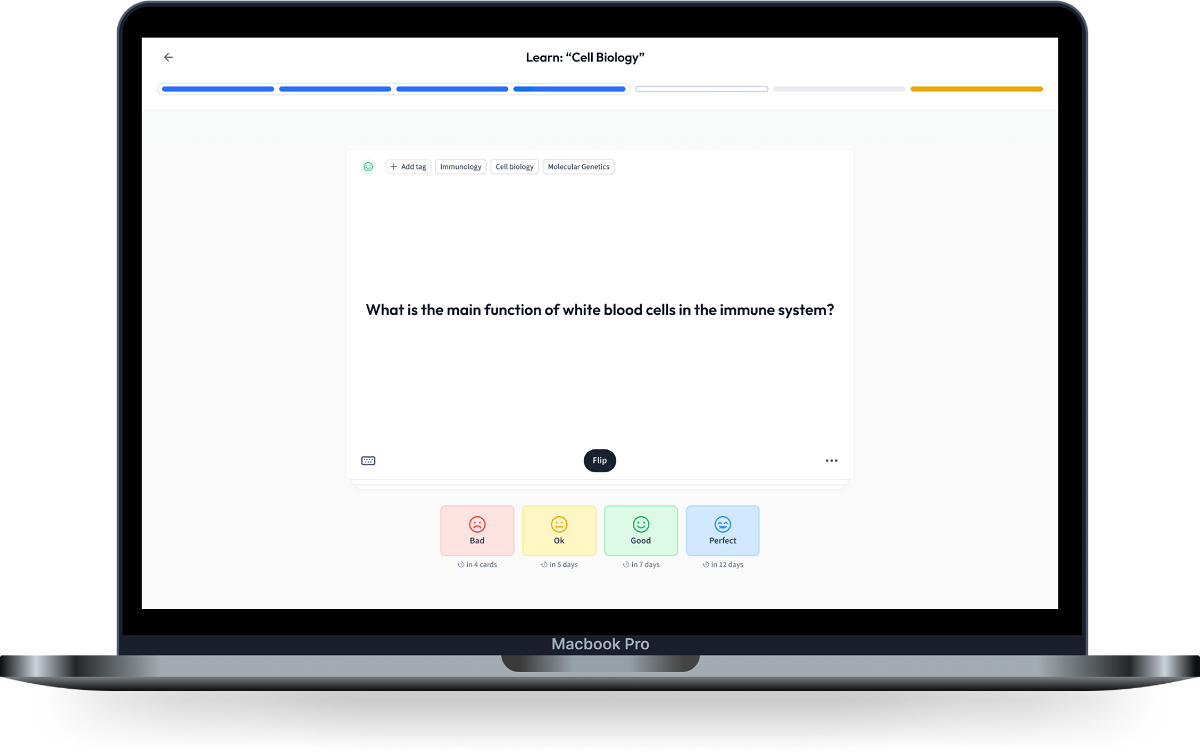
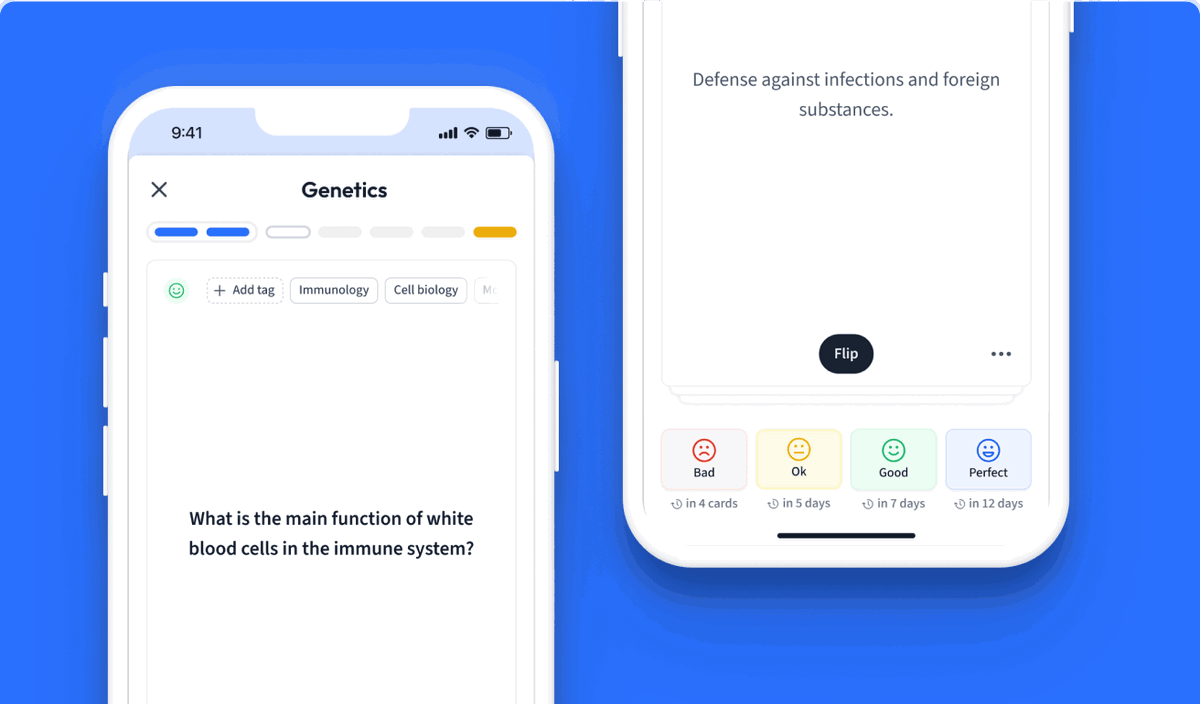
Learn with 7 Break in C flashcards in the free StudySmarter app
Already have an account? Log in
Frequently Asked Questions about Break in C
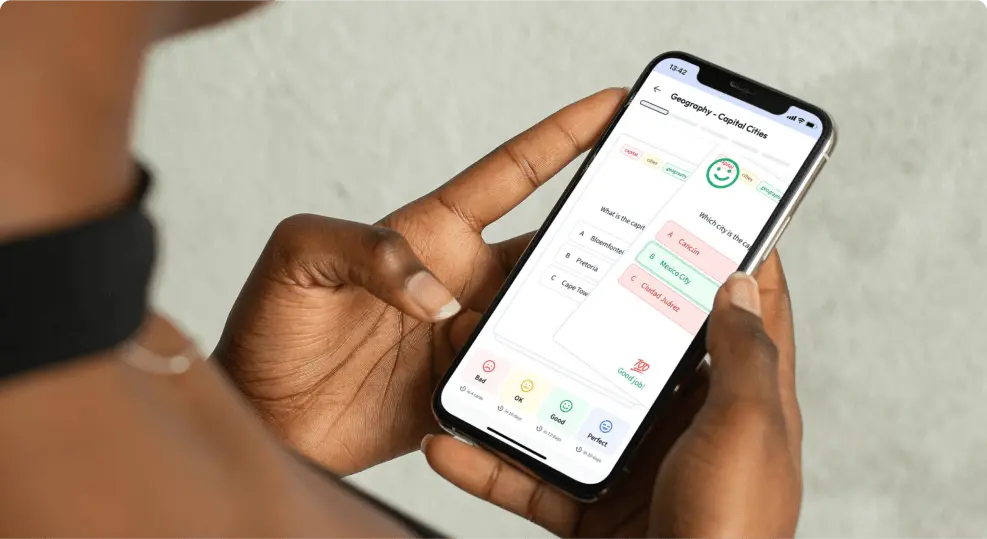
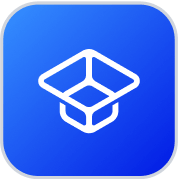
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more