Jump to a key chapter
C Compiler Definition
C Compiler is a specialized software tool that translates programs written in the C programming language into machine code that a computer's processor can execute. Depending on the platform and requirements, a C Compiler can produce different types of machine code.
Purpose of a C Compiler
The purpose of a C Compiler is twofold. First, it allows you to write programs in a high-level language like C, which is easier to understand and manage compared to machine code. Second, it transforms the source code into a format the computer can run efficiently. Here are some important purposes of a C Compiler:
- Translates C code into machine-specific instructions for execution.
- Optimizes the generated code for better performance.
- Checks for errors in the code to ensure it runs correctly.
Most modern C Compilers, like GCC (GNU Compiler Collection), not only compile C code but also support several other languages. These tools are crucial in software development as they enable the creation of complex and efficient applications.
Did you know? C was originally developed at Bell Labs in the early 1970s by Dennis Ritchie.
Types of C Compilers
There is a variety of C Compilers available, each with its unique features and optimizations. Some of the widely used C Compilers include:
- GCC - A widely used open-source C Compiler that supports multiple platforms.
- Microsoft Visual C++ - Commonly used for Windows applications.
- Clang - Known for its fast compile times and detailed error messages, it is part of the LLVM project.
How Does a C Compiler Work?
Understanding how a C Compiler works involves knowing its compilation stages:
- Preprocessing: It involves processing directives like #include or #define.
- Compilation: Converts the preprocessed source code into assembly code.
- Assembly: Turns assembly code into machine code, generating object files.
- Linking: Combines object files into a single executable program.
Below is a simple example of C code and how it is processed by a C Compiler:C code:
int main() { printf('Hello, World!'); return 0; }After passing through the compilation stages, this will eventually become machine code that the CPU can execute.
C Programming Compiler Techniques
C programming compiler techniques are essential for converting human-readable C code into machine-executable instructions. These techniques ensure that the code runs efficiently and accurately. Let's explore some key methods that C Compilers use to achieve this.
Syntax Analysis
Syntax analysis, also known as parsing, is a critical step in the compilation process. The compiler analyzes the C code to create a syntax tree that represents the grammatical structure of the code. This helps in identifying and correcting syntactical errors before further processing.
Syntax errors can include missing semicolons or unmatched brackets, which are detected during this phase.
Semantic Analysis
Semantic analysis checks for meaningfulness in the context of programming logic. It's about ensuring variables are declared, types are matched, and operations are valid. While syntax checks for correct form, semantics checks for correct use.
Advanced C Compilers perform intricate semantic checks to find errors such as type mismatches, incompatible variable operations, and out-of-scope variables. This phase enriches error detection, reducing runtime errors.
Code Optimization
Code optimization improves the performance and efficiency of the generated code. While maintaining the code’s logic, the compiler identifies opportunities to reduce execution time and resource usage. Common techniques include:
- Removing redundant code.
- Inlining functions.
- Unrolling loops.
Consider a loop in C Code:C code:
for (int i = 0; i < 100; i++) { // Some operations}Unrolling the loop may increase performance by reducing the loop overhead. The compiler may transform it into:
for (int i = 0; i < 100; i += 4) { // Operations duplicated 4 times}
Code Generation
In the code generation phase, the optimized intermediary representation of the code is converted into machine code. This machine code is what the processor understands and executes. Each line of C code is translated, taking advantage of the particular architecture of the target machine for efficient execution.
Register Allocation
Register allocation is the process of assigning a large number of target program variables onto a small number of CPU registers. Efficient register allocation is crucial for maximizing performance, as it minimizes accessing slower memory devices.
Compilers use algorithms like graph coloring to optimize register usage, ensuring that the limited CPU registers are used effectively without wasting resources. This technique plays a significant role in enhancing execution speed and reducing power consumption.
C Compiler Characteristics
C Compilers are designed to efficiently translate and optimize C code. Their characteristics determine how effectively they perform these tasks.
Efficiency and Performance
C Compilers are known for their high efficiency and performance, allowing programs to execute quickly and with minimal resource consumption. Key characteristics include:
- Fast processing of code.
- Effective resource management by minimizing memory usage.
- Optimizing code to use CPU instructions efficiently.
Optimization in a C Compiler refers to the various methods it uses to improve the performance and efficiency of the generated code without altering the program's intended functionalities.
Portability
A major benefit of using a C Compiler is portability. This means C code can be compiled and run on various hardware and operating systems with few changes. This characteristic is vital for developing cross-platform applications. With a consistent syntax and standardized libraries, C allows for smooth adaptation across platforms.
C's portability means that many operating systems and applications have a C Compiler, making it easier to distribute software.
Error Detection
Effective error detection is crucial in any C Compiler. Compilers use various methods to detect and report errors during the compilation process:
- Identifying syntax errors like missing semicolons or mismatched brackets.
- Warning about potential runtime errors, such as undeclared variables or type mismatches.
- Offering suggestions for error correction.
Suppose you forget to add a semicolon at the end of a C statement. The compiler might output an error message like:
Error: expected ';' before '}' tokenThis message indicates where the error is expected, guiding you to correct it.
Intermediate Representation
C Compilers often convert source code into an intermediate representation (IR) before translating it into machine code. This layer allows for:
- Optimization opportunities that are platform-independent.
- Simplification of complex code transformations.
- Ease in generating machine code for different architectures.
LLVM, a modern compiler framework, is well-known for its use of IR. The LLVM IR is highly flexible and used to perform sophisticated code optimizations that benefit many programming languages, including C. Using IR facilitates portability and retargetability of compilers and supports deploying efficient code across different platforms.
Compilation Process in C
The compilation process in C involves several stages that transform C source code into an executable program. This process ensures that the code is optimized and executed efficiently by the computer's processor.
Preprocessing
The first stage in the C compilation process is preprocessing. In this step, the preprocessor handles directives such as #include
and #define
.It performs tasks like:
- File inclusion: Incorporates header files into the source code.
- Macro expansion: Replaces macros with their defined values.
- Conditional compilation: Compiles code based on certain conditions.
Preprocessing is a textual substitution and does not understand the semantics of the language.
Compilation
During the compilation phase, the preprocessed code is translated into assembly language specific to the target processor. The compiler performs syntax analysis to ensure the source code adheres to C language rules.This phase involves:
- Checking for syntax errors and generating error messages.
- Translating C code to a lower-level representation.
- Creating an intermediate object file with readable machine instructions.
Consider this simple C code segment:
int add(int a, int b) { return a + b;}After compilation, it may translate to assembly-like code that the processor can interpret.
Assembly
Following the compilation, the assembly stage converts the assembly language code generated previously into machine code specific to the processor's architecture.This step involves:
- Generation of object files that contain machine code.
- Mapping of variables to memory locations and registers.
- Utilizing assembler directives for data declaration and code management.
Linking
The final phase in the C compilation process is linking, where various object files are combined into a single executable program.The linking process involves:
- Resolving function calls and variable references across multiple object files.
- Incorporating library functions and external modules.
- Creating the final executable by arranging machine instructions properly.
In modern development, linkers can also perform optimizations across object files, such as dead code elimination, allowing the removal of unused segments of code. This improves both execution performance and reduces the size of the final executable, crucial for embedded systems development.
C Compiler - Key takeaways
- C Compiler Definition: A C Compiler is a software that translates C language programs into machine code executable by a computer's processor.
- Compilation Process in C: Involves stages like preprocessing, compilation, assembly, and linking to transform C code into executable machine code.
- C Compiler Techniques: Include syntax analysis, semantic analysis, code optimization, code generation, and register allocation to ensure efficient code execution.
- Types of C Compilers: Examples include GCC, Microsoft Visual C++, and Clang, each with unique features and optimizations.
- C Compiler Characteristics: Known for efficiency, portability, error detection, and the use of intermediate representation (IR) for optimization.
- Purpose of a C Compiler: Facilitates programming in high-level C language by translating it into machine-specific instructions and optimizing code for better performance.
Learn faster with the 25 flashcards about C Compiler
Sign up for free to gain access to all our flashcards.
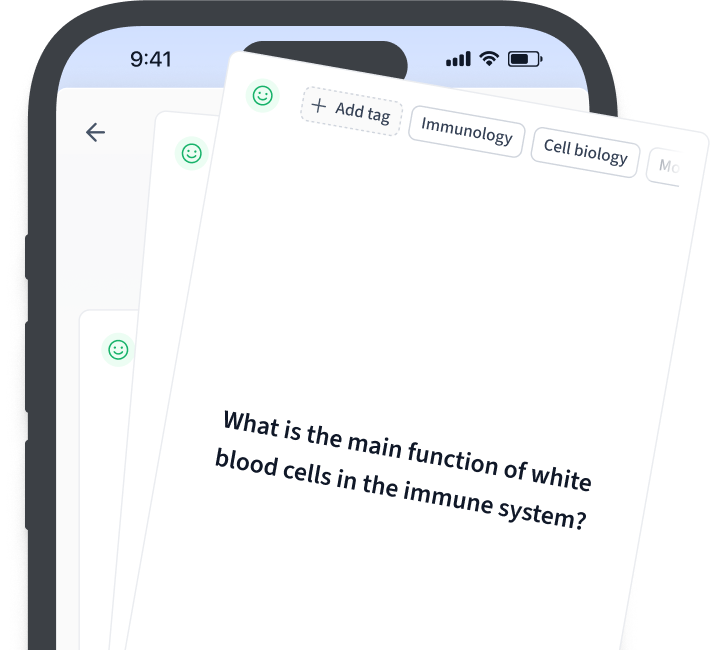
Frequently Asked Questions about C Compiler
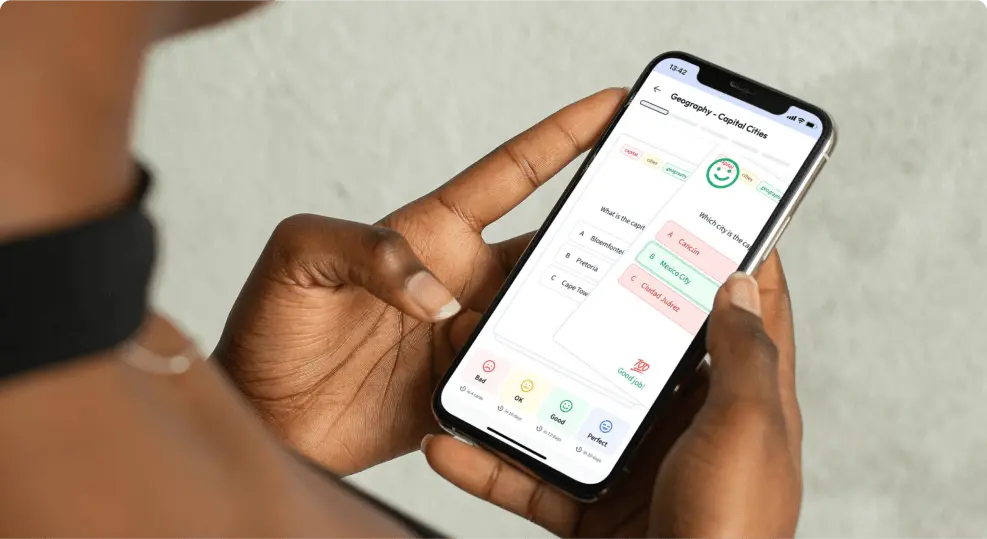
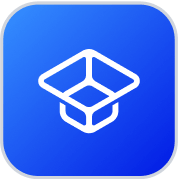
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more