Jump to a key chapter
What is a C Constant?
A C constant is an immutable value or data that remains unchanged throughout the execution of a program. In C programming, constants are used to provide fixed data or values to a program, ensuring efficiency and consistency. Using constants instead of variables with fixed values can prevent accidental modifications and enhance the code readability.
A C Constant refers to a value that remains unchanged during the execution of a program. It is used to provide fixed data or values to the program, ensuring efficiency and consistency.
Types of C constants
In C programming, constants can be broadly categorized into the following types:
- Integer constants
- Floating-point constants
- Character constants
Integer constants in C
Integer constants are whole numbers that can be positive or negative. They are expressed in the base of 10 (decimal), base of 8 (octal), or base of 16 (hexadecimal). A few rules apply to integer constants:
- No commas or blanks are allowed within the constant.
- Decimal constants must not be prefixed with zeroes.
- Octal constants are prefixed with a '0' (zero).
- Hexadecimal constants are prefixed with '0x' or '0X'.
Examples of Integer Constants:
- 10 (Decimal constant)
- 012 (Octal constant)
- 0xA (Hexadecimal constant)
Floating-point constants in C
Floating-point constants represent real numbers and can be represented in two forms: fractional form and exponent form. The fractional form includes a decimal point, while the exponent form includes an 'e' or 'E', followed by an integer exponent. A few rules apply to floating-point constants:
- At least one digit must be present before and after the decimal point in the fractional form.
- An exponent in exponent form must be a whole number.
- The use of 'f' or 'F' suffix denotes a single-precision floating-point constant, whereas 'l' or 'L' denotes a long double. Without these suffixes, the constant is considered a double.
Examples of Floating-point Constants:
- 123.45 (Fractional form)
- 1.23e2 (Exponent form)
- 3.14F (Single-precision constant)
Character constants in C
Character constants represent single characters, enclosed within single quotes. They can be letters, digits, or special characters, as well as escape sequences. Each character constant is of the integer type, corresponding to the integer value of the character in the ASCII table.
Examples of Character Constants:
- 'A' (Character constant)
- '5' (Digit constant)
- '\$' (Special character constant)
- '\n' (Escape sequence constant - newline)
While string constants in C might seem similar to character constants, they are collections of characters enclosed within double quotes. Unlike character constants, string constants are arrays of characters, terminated by a null character ('\0').
Implementing C Constants in Programming
In C programming, constants are defined using the 'const' keyword, followed by the data type, and then the constant name, which is assigned a specific, unchangeable value. The syntax for defining a C constant is:
const data_type constant_name = value;
It is a good practice to use uppercase letters for constant names to differentiate them from variable names. Additionally, constants can be defined as preprocessor directives (macros) using the '#define' directive, as follows:
#define CONSTANT_NAME value
This method does not involve using the 'const' keyword or declaring a data type for the constant. Instead, the preprocessor replaces all occurrences of the constant name with their corresponding value during the compilation process.
Examples of defining constants in C:
const int SIZE = 10; // using the 'const' keyword const float PI = 3.14159F; // using the 'const' keyword #define LENGTH 50 // using the '#define' directive #define AREA (LENGTH * WIDTH) // macro with an expression
C Constant Value Usage
Constants can be utilized in various programming elements, including expressions, arrays, function parameters, and structures. Employing constants in programming constructs ensures consistent behavior, improves code readability, and maintains data integrity by safeguarding against unintentional modifications.
Constant expressions in C
A constant expression evaluates to a constant value entirely during the compilation process. Constant expressions can include integer, floating-point, or character constants, as well as enumeration constants and certain function calls. They are used in the following situations:
- Defining array sizes for static or global arrays
- Declaring case labels in switch statements
- Specifying values for enumerators
- Setting constant function arguments
Examples of constant expressions:
const int ARR_SIZE = 50; // array size int values[ARR_SIZE]; // static array switch (grade) { case 'A': // case label // code break; }
Enumeration constants in C
Enumeration constants are symbolic names assigned to a set of integer constant values. Enumerations are user-defined data types used to represent a collection of related values in a more readable and self-explanatory manner. They are declared using the 'enum' keyword, followed by the enumeration name, and the enumerator list enclosed within curly braces. Each enumerator in the list is assigned a unique integer value, starting from 0 by default and incrementing by 1 for each subsequent enumerator. However, you can explicitly specify integer values for enumerators as needed.
enum enumeration_name { enumerator1 [= value1], enumerator2 [= value2], ... };
Enumeration constants can be used in various constructs, such as switch statements, conditional expressions, and looping statements. In addition, they can be used as arguments for functions that expect integer values.
Example of enumeration constants:
enum Days { SUNDAY, // 0 MONDAY, // 1 TUESDAY, // 2 WEDNESDAY, // 3 THURSDAY, // 4 FRIDAY, // 5 SATURDAY // 6 }; int main() { enum Days today; today = WEDNESDAY; // ... }
C Constant Examples and Applications
Using constants in C programs can help prevent errors and improve the code's maintainability. In the following example, we will use constants to calculate the area of a circle:
#includeconst float PI = 3.14159; int main() { float radius, area; printf("Enter radius: "); scanf("%f", &radius); area = PI * radius * radius; printf("Area of the circle is: %.2f\n", area); return 0; }
In this example, we define the constant 'PI' as a floating-point value representing the mathematical constant π. The value of PI remains constant throughout the program and cannot be modified. By using the constant, we eliminate the risk of inadvertently altering the value of PI, leading to incorrect results or inconsistent behavior. The program then calculates the area of the circle using the constant PI and the user-provided radius.
C Constant Array
A constant array in C is an array whose elements cannot be modified. You can create a constant array by declaring it with the 'const' keyword, signifying that its contents should remain constant throughout the program. Here is an example of declaring a constant array in C:
#includeconst char DAYS_OF_WEEK[7][10] = { "Sunday", "Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday" }; int main() { for (int i = 0; i < 7; i++) { printf("%s\n", DAYS_OF_WEEK[i]); } return 0; }
In this example, we define a constant two-dimensional character array 'DAYS_OF_WEEK', which contains the names of the days of the week. The array is marked as constant using the 'const' keyword, indicating that its elements cannot be modified throughout the program's execution.
Modify a constant array in C (possible or not?)
Attempting to modify a constant array in C will lead to compilation errors, as constants are meant to remain immutable throughout the program's execution. If you attempt to modify a constant array, the compiler will raise an error, alerting you to the violation of constancy. The following example demonstrates what happens when you attempt to modify a constant array:
#includeconst int NUMBERS[5] = { 1, 2, 3, 4, 5 }; int main() { // Attempt to modify a constant array NUMBERS[0] = 10; // This line will cause a compilation error return 0; }
When attempting to compile the above code, the compiler will generate an error message due to the attempted modification of the constant array 'NUMBERS'. The error message will indicate that you cannot assign a new value to an element of a constant array.
C Constant Pointer
A constant pointer in C is a pointer that cannot change the address it points to once assigned. You can create a constant pointer by using the 'const' keyword with the pointer declaration and placing it after the data type. The syntax for declaring a constant pointer is:
data_type *const pointer_name = memory_address;
Declaring a constant pointer ensures that the address it points to remains immutable throughout the program's execution. Here is an example of declaring and using a constant pointer in C:
#includeint main() { int num = 42; int const *ptr = # printf("Value at address %p is %d\n", ptr, *ptr); // The following line would result in a compilation error // ptr = &another_variable; return 0; }
Use a constant pointer in C
Once declared and assigned to a memory address, a constant pointer cannot be changed to point to a different address. However, the value at the pointed address can still be changed. This is useful when you want to ensure that the pointer always points to the intended address and avoids inadvertently assigning it to a different memory location. Here is an example of using a constant pointer in C:
#includeint main() { int num = 42; int another_variable = 99; int *const ptr = # printf("Value at address %p is %d\n", ptr, *ptr); // The following line will NOT result in a compilation error num = 100; printf("Updated value at address %p is %d\n", ptr, *ptr); // However, this line would result in a compilation error: // ptr = &another_variable; return 0; }
In this example, we have a pointer 'ptr', which points to the address of variable 'num'. After declaring the pointer as constant, we can still update the value of 'num', but we cannot change the address that 'ptr' points to. If we try to update the address, the compiler will generate an error message.
C Constant - Key takeaways
C Constant: A value that remains unchanged during the execution of a program.
Types of C constants: Integer constants, Floating-point constants, and Character constants.
Defining a constant in C: Use the 'const' keyword or '#define' directive.
Constant expressions: Evaluate to a constant value during compilation and are used in various programming constructs.
Constant arrays and pointers: Immutable elements or addresses that improve code maintainability and prevent errors.
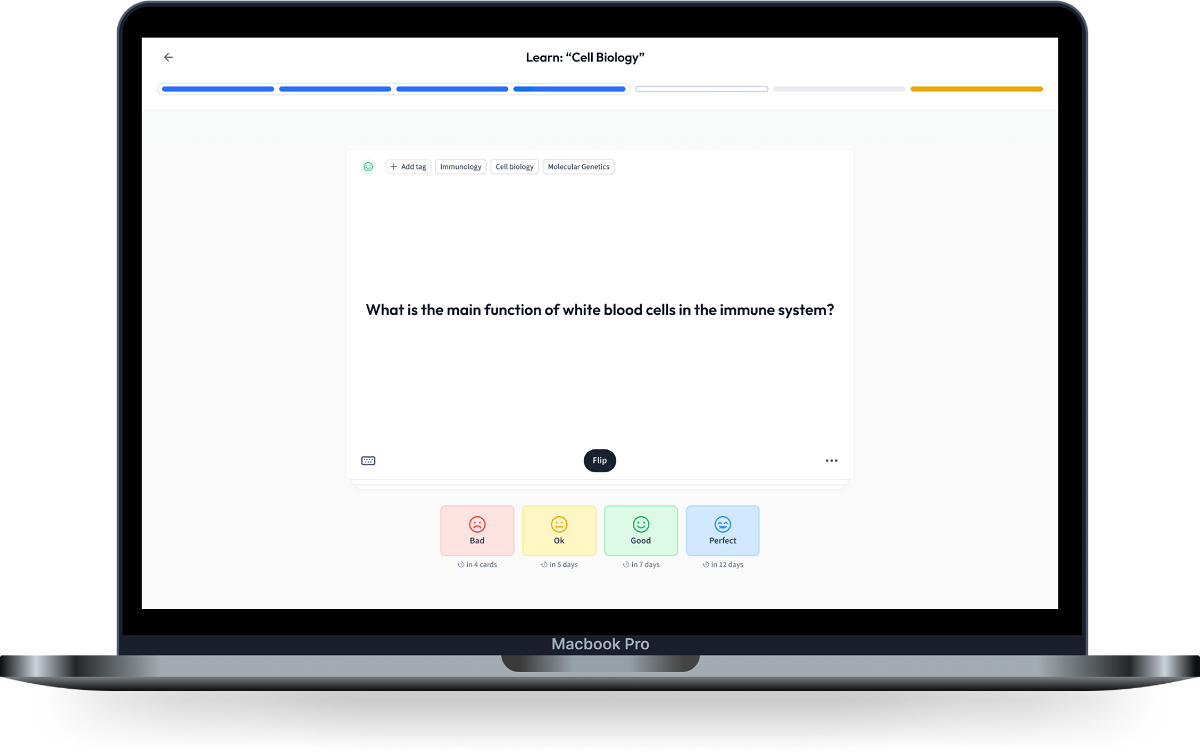
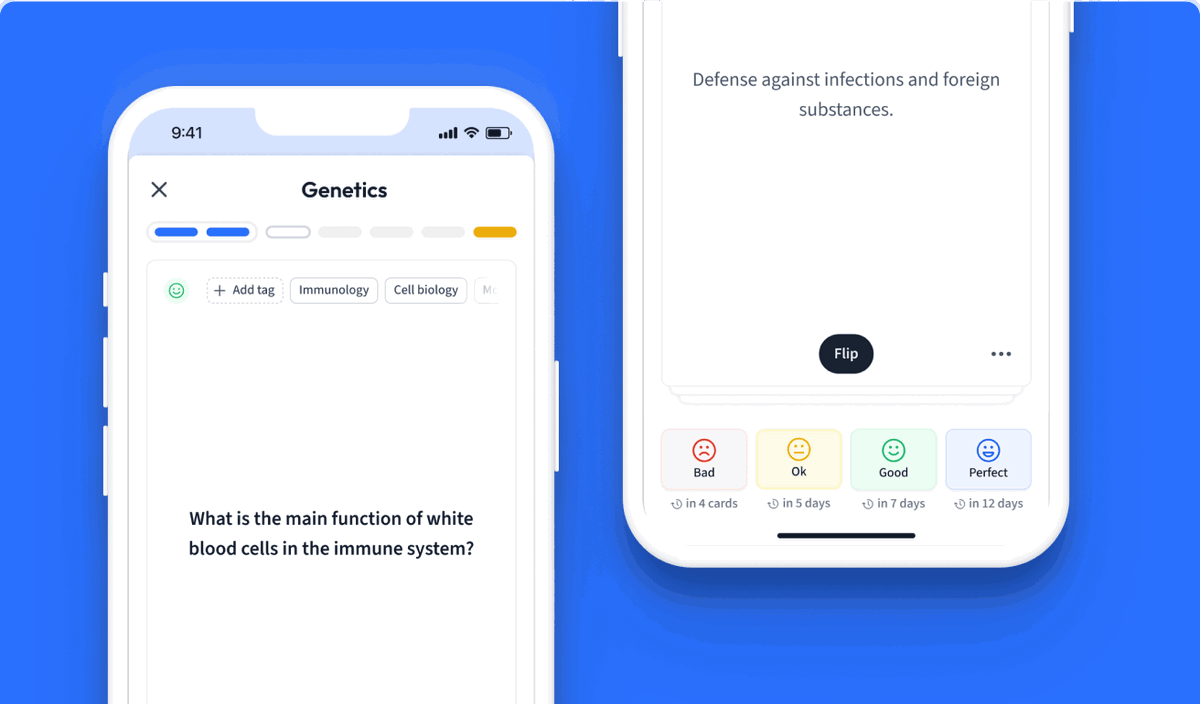
Learn with 15 C Constant flashcards in the free StudySmarter app
Already have an account? Log in
Frequently Asked Questions about C Constant
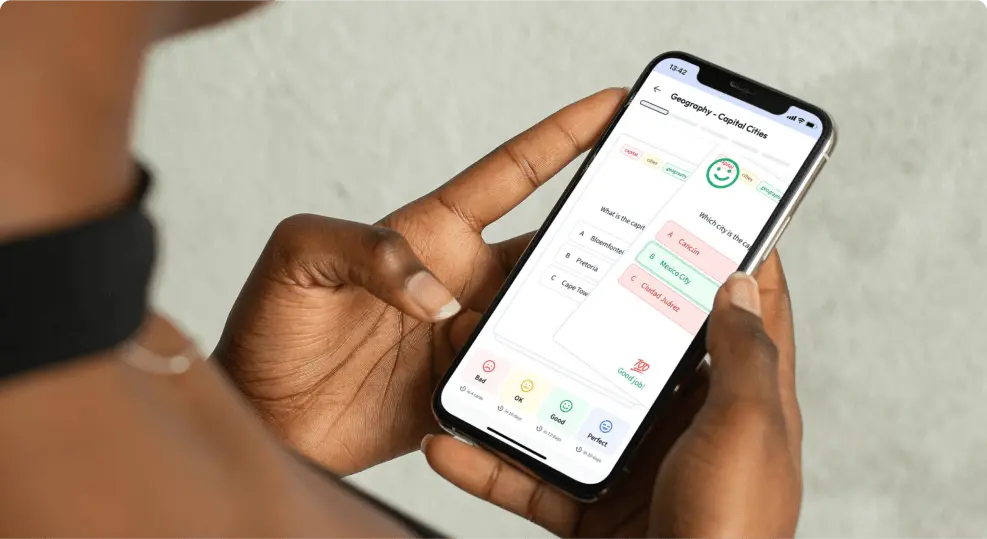
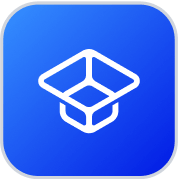
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more