Jump to a key chapter
Concurrent Programming Definition
Concurrent Programming is a computing method where multiple computations are executed during overlapping time periods rather than sequentially. This involves components of a computer program being designed so they can run simultaneously, often improving efficiency and performance. Through the use of threads, processes, and synchronized tasks, concurrent programming allows applications to perform multiple operations concurrently, such as processing data, computation, and handling user input. Understanding this concept is key in environments where multitasking is imperative.
Benefits of Concurrent Programming
Concurrent programming offers several advantages in programming and systems design. It allows you to:
- Improve the efficiency of applications by maximising CPU usage.
- Enhance responsiveness in applications, especially where user interaction is vital.
- Manage and execute multiple tasks concurrently, reducing wait times.
- Utilize resources efficiently, such as memory and processing power.
- Create scalable systems that grow with additional tasks and processes.
Thread: A thread is the smallest unit of processing that can be performed in an OS (Operating System). In concurrent programming, multiple threads can run in parallel to handle different tasks within a single process.
Imagine a web server that needs to handle multiple user requests simultaneously. Using concurrent programming, the server assigns each request to a separate thread or process, allowing it to respond to each client independently and efficiently. Here is a simple Python example of concurrent programming using threads:
import threading def task(name): print(f'Task {name} is running') threads = [] for i in range(5): thread = threading.Thread(target=task, args=(i,)) threads.append(thread) thread.start() for thread in threads: thread.join()This code creates and starts five threads that execute the 'task' function concurrently.
Concurrent programming is different from parallel programming. While both involve multiple computations, concurrency focuses on dealing with multiple tasks at once without the assumption of simultaneous execution, whereas parallel programming involves computations actually occurring at the same time.
Concurrency vs. Parallelism: Although often used interchangeably, concurrency and parallelism are distinct concepts. Concurrency is about dealing with multiple tasks making progress, whereas parallelism involves performing multiple tasks at the same time. In essence, concurrency is a broader concept that encompasses parallel execution but is not limited to it. In concurrent programming, tasks might be executed on a single core with context switching giving the illusion of simultaneous execution. Parallel programming typically requires a multi-core system where each core runs separate tasks, leading to actual simultaneous execution. Effective concurrent systems often balance these two approaches to maximize efficiency and performance.
What is Concurrency in Programming
Concurrency in programming refers to the execution of multiple sequences of operations at the same time. It's a method used to structure and manage operations within software, enabling tasks to be handled concurrently to improve performance and responsiveness. By understanding and leveraging concurrency, you can create programs that efficiently handle numerous operations, such as managing multiple user requests or processing vast datasets.
Core Principles of Concurrency
Concurrency is built on several core principles that guide how tasks are managed and executed. Here are some key principles:
- Task Coordination: Organizing how tasks communicate and share resources.
- Synchronization: Ensuring tasks run in a specific order when necessary.
- Resource Sharing: Multiple tasks accessing shared data or files.
- Deadlock Prevention: Avoiding situations where tasks wait indefinitely for resources.
Deadlock: A situation in concurrent programming where two or more tasks hold and wait for each other's resources, creating a cycle of dependency that prevents progress for any of the tasks involved.
Consider a banking system where multiple transactions are occurring at the same time. Using concurrent programming, each transaction can run independently, ensuring that all operations are handled efficiently. Here is a Java example illustrating concurrency using threads:
class Account { private int balance = 1000; public synchronized void deposit(int amount) { balance += amount; } public synchronized void withdraw(int amount) { balance -= amount; } } public class Bank { public static void main(String[] args) { Account account = new Account(); Thread t1 = new Thread(() -> account.deposit(500)); Thread t2 = new Thread(() -> account.withdraw(200)); t1.start(); t2.start(); } }This example shows how deposit and withdrawal operations can be run concurrently on a shared Account object.
Java includes built-in synchronization features to handle concurrency effectively. Understanding these features is crucial to prevent data corruption in multi-threaded applications.
When implementing concurrency, you may encounter various challenges that require careful consideration:
- Race Conditions: Occur when two or more threads access shared data at the same time and try to change it.
- Non-deterministic Outputs: The output or behavior of programs may vary because threads can be scheduled in different orders.
- Thread Starvation: Happens when certain threads are repeatedly denied access to resources they need to progress.
Techniques in Concurrent Programming
Concurrent programming employs various techniques to achieve efficient execution of multiple operations. These techniques are essential for managing tasks that can run simultaneously, creating responsive and effective software applications. By leveraging these techniques, you can optimize the use of resources, improve application performance, and ensure that your software can handle complex operations without slowdown.
Multithreading and Concurrency
Multithreading is a core technique in concurrent programming that allows multiple threads to be executed simultaneously. Each thread represents a separate path of execution within a process, enabling tasks to be managed in parallel. Multithreading is particularly useful in scenarios where tasks are independent and can be performed concurrently without interfering with each other. This helps in maximizing CPU usage and enhancing the efficiency of applications.
Consider a text editor that performs spell-checking, file-saving, and content rendering simultaneously. Using multithreading, each of these operations can run in its own thread, allowing the editor to remain responsive to user input while these background tasks are handled concurrently. Here is an example of Python code using multithreading:
import threading def spell_check(text): print('Spell checking:', text) def save_file(filename): print('Saving file:', filename) text_thread = threading.Thread(target=spell_check, args=('document.txt',)) save_thread = threading.Thread(target=save_file, args=('document.txt',)) text_thread.start() save_thread.start()Each function runs as a separate thread, executing its respective task.
Many programming languages offer libraries and frameworks that simplify multithreading, such as Java's concurrency utilities and Python's threading module.
Thread Lifecycle: In multithreading, it is important to understand the lifecycle of a thread, which includes several states such as new, runnable, waiting, and terminated. Proper management of these states ensures efficient execution and resource optimization. Threads often start in the 'new' state when created, move to 'runnable' when they are ready to run, may enter 'waiting' while paused for a resource, and finally reach 'terminated' once execution ends. Effective thread lifecycle management requires handling state transitions carefully to prevent inefficiencies and ensure application stability.
Shared Resources in Concurrency
Managing shared resources is a crucial aspect of concurrency. When multiple threads or processes access the same resources, such as memory or files, coordination is necessary to prevent errors, such as data corruption. Issues like race conditions, deadlocks, and starvation can arise if shared resources are not managed properly. Implementing synchronization mechanisms such as locks, semaphores, and monitors can help in preventing these problems.
Semaphore: A signal used to control access to a common resource by multiple processes in a concurrent system. It is a variable or abstract data type used to manage concurrent access efficiently.
Imagine multiple threads trying to write to the same file. Without proper synchronization, the file could become corrupted. Using semaphores ensures only one thread can access the file at a time. Here's a C-style pseudocode example of using semaphores for synchronization:
semaphore fileAccess = 1; void writeFile() { wait(fileAccess); // code to write to file signal(fileAccess); }The wait and signal operations manage access, ensuring the file is modified safely.
Mutexes (short for mutual exclusions) are similar to semaphores but provide locking at a more granular level, specifically for ensuring that only one operation can execute a particular code section at a time.
Advanced Synchronization Techniques:
- Read-Write Locks: Specialized locks that allow concurrent reads but ensure mutually exclusive write access. This can significantly enhance performance in scenarios with more frequent reads than writes.
- Barriers: Synchronization primitives used to block a set of threads until a specified condition is met or all threads have reached the barrier point. This is useful for coordinating complex multithreaded tasks.
Programming Concurrency Best Practices
When developing concurrent programs, adhering to best practices is crucial for creating efficient and reliable software. Proper guidelines enable you to leverage the full potential of concurrent processes while minimizing common pitfalls. By following these practices, you can ensure your applications make optimal use of resources, remain scalable, and maintain a high level of responsiveness.
Designing for Concurrency
Designing software with concurrency in mind involves careful planning and structuring of your code. Here are some best practices to consider:
- Identify Independent Tasks: Break down your program into tasks that can run independently. This enhances the program’s ability to perform multiple operations simultaneously.
- Utilize Thread Pools: Instead of creating new threads for every task, use a thread pool to manage and reuse existing threads, reducing overhead.
- Avoid Global State: Minimize reliance on global variables, which can lead to conflicts and data corruption when accessed by multiple threads.
Thread Pool: A collection of pre-instantiated, reusable threads that can be used to execute tasks. Thread pools limit the number of threads that can be running at a time, optimizing resource use.
Consider an example where a web server processes incoming requests using a thread pool. This approach avoids the overhead of constantly creating and destroying threads. Here's a pseudocode example:
initialize ThreadPool pool with size 10; while (server is running) { incomingRequest = waitForRequest(); pool.execute(incomingRequest); }This structure helps in efficiently managing concurrent requests by reusing available threads in the pool.
When using thread pools, always set an upper limit to the number of concurrent threads to avoid resource exhaustion and potential server crashes.
Synchronization Mechanisms
Proper synchronization is key to safely managing shared resources in concurrent applications. Here are some mechanisms you can implement:
- Locks: Ensure only one thread can access a resource at a time by wrapping code in a lock.
- Monitors: Provide a higher-level synchronization construct that combines mutual exclusion with condition variables.
- Atomic Variables: Enable thread-safe operations on integers and booleans without the need for explicit synchronization.
Monitor: A mechanism that provides both mutual exclusion and the ability for threads to wait for certain conditions to be true before continuing execution.
When implementing synchronization, using data structures designed for concurrency can further enhance performance. Here are a few examples:
- Concurrent Collections: Java's ConcurrentHashMap and CopyOnWriteArrayList are thread-safe collections optimized for concurrent use.
- Non-blocking Algorithms: Algorithms that use atomic operations to ensure that computation can proceed without locks, reducing contention and improving throughput.
Concurrent Programming - Key takeaways
- Concurrent Programming Definition: A computing method where multiple computations are executed during overlapping time periods rather than sequentially, improving efficiency and performance.
- Concurrency in Programming: Involves the execution of multiple sequences of operations simultaneously, improving performance and responsiveness.
- Multithreading and Concurrency: A technique in concurrent programming allowing multiple threads to execute simultaneously, maximizing CPU usage and efficiency.
- Shared Resources in Concurrency: Involves managing resources like memory and files that multiple tasks access in concurrency, requiring synchronization to prevent errors.
- Techniques in Concurrent Programming: Includes multithreading, synchronization mechanisms like locks and semaphores, and advanced methods like read-write locks and barriers to manage concurrent processes.
- Programming Concurrency Best Practices: Includes identifying independent tasks, using thread pools, and employing proper synchronization to handle resources safely.
Learn faster with the 28 flashcards about Concurrent Programming
Sign up for free to gain access to all our flashcards.
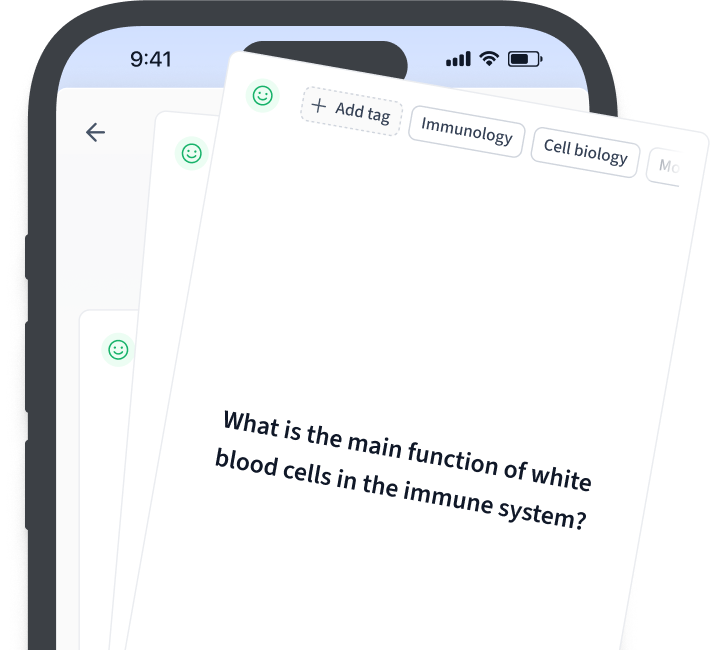
Frequently Asked Questions about Concurrent Programming
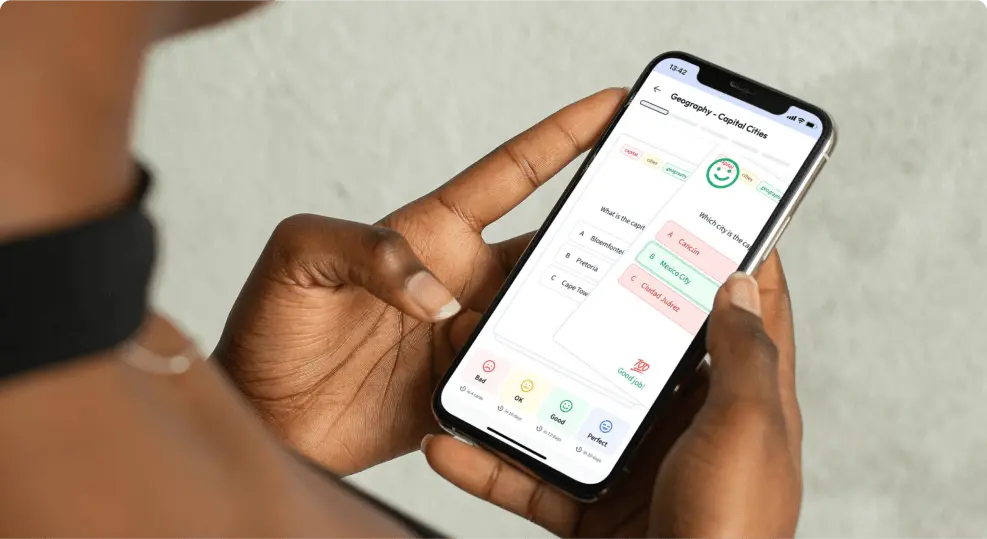
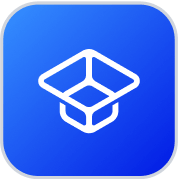
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more