Jump to a key chapter
Cout C in Computer Programming
In the realm of computer programming, especially when using C++, cout is an essential command often utilized for displaying output. It serves as a fundamental component to interact with the console, providing the mechanism to output text or variables during the run-time of a program.
Understanding Cout in C++
The cout command is part of the C++ standard library and is utilized primarily within the context of basic input/output operations. It acts as the standard output stream and is generally used to output data to the console window.
The command cout is typically used in conjunction with the insertion operator (<<), forming a fundamental aspect of C++ syntax for output operations. It is part of the iostream header file, which must be included in your program to use cout.
Here's a simple example of using cout:
#includeIn this code, 'Hello, World!' is an output displayed in the console using cout.using namespace std;int main() { cout << 'Hello, World!'; return 0;}
When implementing cout, keep in mind the following:
- Include
: Always include the iostream library at the beginning of your C++ program. - Insertion Operator (<<): Use the << operator to send data to the cout stream.
- Namespace: Ensure you're using the correct namespace with
using namespace std;
to simplify code by avoiding std::cout syntax.
The history of cout is rooted deeply in the evolution of C++ and offers a fascinating look into its adoption. In earlier versions of C++, programming often involved the use of printf, a C function. However, cout was introduced to leverage C++'s object-oriented features and streamline the syntax. The adoption of cout led to a more modern, manageable approach to output operations, with functionality including not only simple text output but also allowing the usage of manipulators to format output in ways that were not straightforwardly possible before. Manipulators like endl (to insert a newline) or setprecision (to control numeric precision) showcase how cout not only replaced printf due to elegance but expanded output functionality significantly.
What Library is Cout in C++
To effectively utilize cout in your C++ programs, it's essential to understand the libraries involved. The cout command is a part of the C++ standard library, which provides a wealth of classes and functions essential for performing input and output operations.
In C++, cout is included in the iostream library, which stands for Input Output Stream. This library is crucial as it not only contains cout but also other input/output utilitarian elements such as cin, cerr, and clog.
Here is a brief look at the key components of the iostream library:
- cout: Used for standard output.
- cin: Used for standard input.
- cerr: Used for error messages.
- clog: Used for logging information.
To see cout in action, consider this simple program:
#includeThis example highlights that the iostream library must be included to use cout. The output will be displayed in the console as 'This is a test of cout.'using namespace std;int main() { cout << 'This is a test of cout.'; return 0;}
Remember that the insertion operator (<<) used with cout can be chained to output multiple variables or strings in a single line.
The incorporation of cout within the iostream library marked a significant innovation in C++ programming. Historically, C++ was derived from the C language, which used function-based input/output operations such as printf and scanf. However, with C++, a more object-oriented approach was desired, leading to the adoption of stream-based I/O, making cout and its relatives a better fit for the language's philosophy. Employing the iostream library, developers can take advantage of overloading and flexibility inherent in streams, encouraging a cleaner and more intuitive code base. The ability to use manipulators with cout also provides versatile control over the output format, which is invaluable in various programming scenarios.
How to Cout a Vector C
When working with C++ programming, you often encounter situations where you need to output complex data structures, such as vectors, to the console. Understanding how to cout a vector efficiently can greatly enhance your debugging and program tracing capabilities.
Printing a Vector using Cout
To print a vector using cout, you'll need to iterate through the elements of the vector and output each element. Here's how you can effectively do this.
Here's an example showing how you can use cout to print the elements of a vector:
#includeIn this code, a vector of integers is created and initialized, followed by a loop that uses cout to print each element separated by a space.#include using namespace std;int main() { vector myVector = {10, 20, 30, 40, 50}; for (int i = 0; i < myVector.size(); i++) { cout << myVector[i] << ' '; } return 0;}
You can also use a range-based for loop to access each element of the vector when using cout for simplicity and readability.
Printing vectors in more sophisticated ways can be achieved using iterators or lambda functions in conjunction with the for_each algorithm. Iterators abstract away direct index manipulation, akin to a pointer but designed to work within STL's structure:
#includeThis method affords greater flexibility as it seamlessly integrates into more complex functional programming patterns, which can be highly useful in large codebases.#include #include using namespace std;int main() { vector myVector = {10, 20, 30, 40, 50}; for_each(myVector.begin(), myVector.end(), [](int n) { cout << n << ' '; }); return 0;}
Cout Usage Examples in C
When programming in C++, the cout command plays a crucial role in displaying output to the console. It is an indispensable tool for programmers who wish to debug their code or present information to users. This section discusses various effective ways cout is utilized.
Cout C++
In C++, cout is a command used to send output to the standard output stream, typically the console. It is coupled with the insertion operator (<<) to facilitate the output of various data types.
Consider this typical usage example of cout:
#includeThis simple program demonstrates how cout is used to print a string to the console output.using namespace std;int main() { cout << 'Welcome to C++ Programming!'; return 0;}
Always include the appropriate namespace or prefix cout with std:: to avoid undefined reference errors in C++.
Going deeper, cout can be used with manipulators to alter the output format. For example, to ensure a precision of floating-point output, manipulators like setprecision
can be applied:
#includeThis allows for more control over numerical outputs, making cout versatile in various programming situations.#include using namespace std;int main() { double pi = 3.14159265; cout << fixed << setprecision(2) << pi; // Outputs: 3.14 return 0;}
Cout Definition in Computer Science
In computer science, specifically in C++, cout is a predefined object in the iostream library representing the standard console output stream. It is used extensively for printing output to the screen.
The cout object is integral to C++ syntax, as it facilitates interaction between the program and the user through output. Understanding how to use it effectively is fundamental for any aspiring C++ programmer.
Here are some common ways to employ cout:
- Output text strings to the console.
- Display integer and floating-point values.
- Print multiple outputs in a single line of code.
C Ways to Use Cout
The utility of cout spans a broad spectrum of programming applications. Below are detailed ways to maximize its use:
Here is an example utilizing cout to output the result of a calculation:
#includeThis example demonstrates how calculations can be printed alongside text for user information.using namespace std;int main() { int num1 = 15, num2 = 30; cout << 'Sum is: ' << num1 + num2; return 0;}
A deeper dive into using cout effectively involves understanding how to use it with custom objects. You can overload the << operator to tailor how an object should be outputted via cout.
#includeThis customizes output, providing clarity and improving data presentation.using namespace std;class Point {public: int x, y; Point(int x, int y) : x(x), y(y) {} friend ostream& operator<<(ostream& os, const Point& pt) { os << '(' << pt.x << ', ' << pt.y << ')'; return os; }};int main() { Point pt(5, 10); cout << pt; // Outputs: (5, 10) return 0;}
cout C - Key takeaways
- cout in C++ Programming: 'cout' is a command in C++ used for displaying output during a program's run-time, part of the standard library, primarily for basic input/output operations.
- Library for cout in C++: 'cout' is included in the
iostream
library, a necessary component for utilizing various input/output operations like 'cin', 'cerr', and 'clog'. - Usage of cout for vectors: To output vectors using 'cout', iterate through elements to display each, or employ iterators and lambda functions for enhanced control and readability.
- Cout in C++ Standard Syntax: 'cout' requires the
iostream
library and works with the insertion operator (<<) to output data, usually used with thenamespace std;
. - Cout Definition in Computer Science: In C++, 'cout' represents the standard output stream, facilitating interaction and output in programs and is foundational for C++ syntax.
- Cout Usage Examples: Common 'cout' examples include outputting strings, integers, operations results, and custom object representations by overloading the << operator.
Learn faster with the 28 flashcards about cout C
Sign up for free to gain access to all our flashcards.
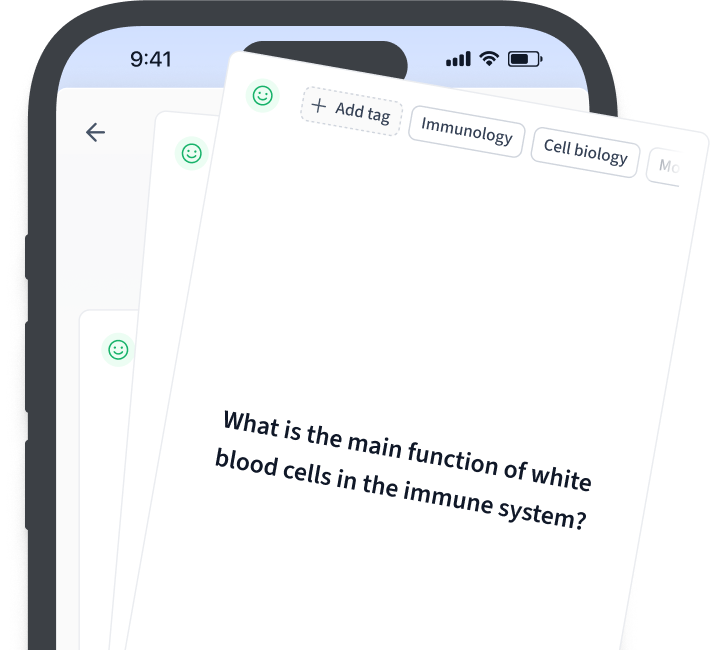
Frequently Asked Questions about cout C
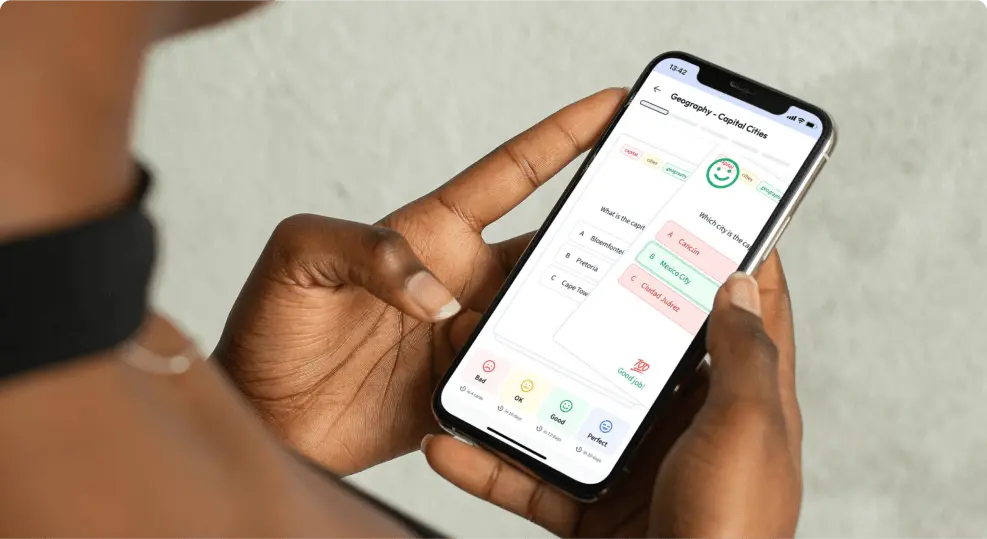
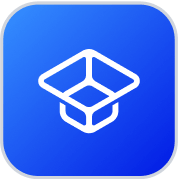
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more