Jump to a key chapter
What is Event Driven Programming
Event Driven Programming is a programming paradigm where the flow of the application is determined by events. These can be user actions, sensor outputs, or messages from other programs.
What are Events in Event Driven Programming
In the context of Event Driven Programming, events are crucial as they trigger the functions or methods to execute. Events can originate from various sources, and recognizing them is essential for seamless interactivity in applications.
Event: An event is an action or occurrence recognized by software that may be handled by the software program.
Consider a scenario in a graphical user interface: A button press by a user constitutes an event. When the button is pressed, an event is fired, and the application executes the associated function.
- User Inputs: Mouse clicks, key presses, touch inputs.
- Sensor Outputs: Data from motion detectors, temperature sensors.
- System Events: Opening files, beginning a network connection.
In modern web development, events are managed using listeners in languages such as JavaScript which listen for specific event types.
Event Driven Programming Technique
The Event Driven Programming technique entails structuring your code around events and their handlers.
Event Handler: A callback function that is executed when a specific event occurs.
Below is an outline of the typical steps involved in implementing Event Driven Programming techniques:
- Identify Events: Determine what events the application needs to respond to.
- Set Up Event Listeners: Use code that waits and listens for these events.
- Write Event Handlers: Create functions that will execute when the events are triggered.
- Trigger Events: Some events are natural (like a user's click), but you may also need to trigger events programmatically.
To illustrate, consider a Python program monitoring a file. When the file is modified, an event listener detects the change and calls the appropriate handler:
import watchdog.observersimport watchdog.eventsclass ChangeHandler(watchdog.events.FileSystemEventHandler): def on_modified(self, event): print('File has been modified')observer = watchdog.observers.Observer()handler = ChangeHandler()observer.schedule(handler, path='.', recursive=False)observer.start()
Explore the following interesting aspects of Event Driven Programming:- Asynchronous Processing: In many cases, events are handled asynchronously, meaning they can be processed without waiting for the completion of other tasks. This is crucial in applications like web servers where multiple requests may need to be handled concurrently.- Decoupling Code: Event Driven Programming offers a way to decouple the code for better scalability and maintainability. As events and their handlers are independent, they can be modified or extended without affecting other parts of the application.- Event Bubbling and Capturing: Especially relevant in web development, these concepts determine how events are propagated in the DOM hierarchy when an action occurs, such as a click event.
Python Event Driven Programming
Python is a versatile language that supports Event Driven Programming, making it an excellent choice for building dynamic and interactive applications. By using event-driven frameworks and libraries, you can manage and control how your application responds to different events, providing a seamless user experience.
Event Driven Programming: A programming paradigm where the flow of the program is determined by events—user actions like mouse clicks, sensor outputs, or messages from other programs.
Python's libraries such as Tkinter for GUI applications and Twisted for network applications are perfect for implementing Event Driven Programming techniques.
Python Event Driven Programming Examples
Exploring practical examples can enhance your understanding of Event Driven Programming in Python. It involves setting up events, adding event listeners, and defining event handlers.Let's examine a simple GUI application setup using Tkinter, a standard GUI toolkit in Python:
import tkinter as tkdef on_button_click(): print('Button Clicked!')root = tk.Tk()button = tk.Button(root, text='Click Me', command=on_button_click)button.pack()root.mainloop()This example demonstrates an event (button click) and its handler (printing a message to the console).In another context, consider a websocket server using the asyncio library, which handles connections as an event:
import asyncioimport websocketsasync def echo(websocket, path): async for message in websocket: await websocket.send(f'Received: {message}')start_server = websockets.serve(echo, 'localhost', 12345)asyncio.get_event_loop().run_until_complete(start_server)asyncio.get_event_loop().run_forever()In this code snippet, the event is a received message, and the handler sends back an acknowledgment.
Event Driven Programming in Python isn't limited to GUI applications. It extends to network operations, automation scripts, and data handling processes. By utilizing Python's asynchronous capabilities, you can enhance performance and scalability:
- Concurrency: Python's asyncio and threading libraries facilitate concurrent execution, making them ideal for handling events in parallel.
- Framework Support: Libraries like Flask and Django offer event-driven capabilities for web applications.
- Real-time Systems: Python can leverage event-driven designs in IoT and robotics for real-time data processing.
Developing with Python Event Driven Programming
Developing applications using Event Driven Programming in Python involves utilizing different tools and strategies to manage the events efficiently. Here are some practices and technologies to consider:
- Frameworks: Depending on the application type, use appropriate frameworks. For web apps, Flask and Django; for GUIs, Tkinter or PyQt.
- Asynchronous Programming: Employ asyncio for non-blocking code execution, allowing better responsiveness.
- Event Queues: Use libraries like Celery for task queues to handle events effectively in distributed environments.
Tools | Description |
Flask | A micro web framework for building web applications with ease. |
Celery | A distributed task queue library for handling asynchronous tasks. |
PyQt | An application framework and GUI toolkit for Python applications. |
Event Driven Programming Examples
Event Driven Programming is extensively used in numerous real-world applications, making systems responsive and interactive. Discovering its applications helps in understanding how this paradigm practically plays out in various fields.
Real-World Applications of Event Driven Programming
Event Driven Programming provides the backbone for many systems and applications you interact with daily. Here are some prominent real-world applications:
- Graphical User Interfaces (GUIs): In GUIs, users interact through events like clicks, drags, and key presses. Applications use frameworks such as Tkinter or PyQt to manage these events.
- Web Development: Events like form submissions, mouse clicks, or API calls are handled with JavaScript or frameworks like Node.js to create dynamic web applications.
- Embedded Systems: Event driven techniques are used in devices like smart thermostats and alarms, which react to inputs from sensors.
- Real-time Systems: Systems that require immediate response, such as stock trading platforms, use event-driven designs to ensure timely data processing.
- Games: Event-driven paradigms manage user inputs and game events, forming the foundation for interactive gaming experiences.
Consider a mobile application for a smart home system. When a door sensor detects that the door has been opened, an Ethernet message is sent, triggering an event-driven system to activate the house's alarm and notify the owner via a smartphone app.
For gaming applications, Event Driven Programming is vital since games often depend on user inputs and system events. Games typically use engines such as Unity or Unreal Engine which operate on event-driven architectural patterns to capture in-game events, determine actions and change states based on these inputs.
Modern web applications often use WebSockets to handle real-time communication, making events crucial for persistent data connections and live content updates.
Common Event Driven Programming Exercises
Practicing Event Driven Programming through exercises helps solidify your understanding of how events and handlers interact. Some common exercises you might encounter include:
- Building a Simple Counter: Create a button in a GUI application that increments a count each time it's clicked, using event listeners in frameworks like Tkinter or Java Swing.
- Handling Keyboard Input: Develop a game or application that changes based on key presses, where each key represents a different action.
- Event Logging System: Write a program that logs system events, such as file changes, into a text file using libraries like python-logging.
An example exercise might involve creating a simple GUI calculator in Python using Tkinter. Each button represents a number or operation. Each button press triggers an event, performing a calculation and updating the display.
import tkinter as tkdef click(event): current = entry.get() entry.delete(0, tk.END) entry.insert(tk.END, current + event)root = tk.Tk()entry = tk.Entry(root)entry.pack()buttons = [tk.Button(root, text=str(i), command=lambda i=i: click(str(i))) for i in range(10)]for button in buttons: button.pack(side='left')root.mainloop()
Incorporating advanced Event Driven Programming exercises can enrich your skill set:
- Simulating a Network Protocol: Implement a simple network protocol where events are generated and processed upon receiving specific packets.
- Asynchronous File Operations: Develop applications that handle file read/write operations asynchronously, using libraries like asyncio, ensuring the UI remains responsive.
Benefits of Event Driven Programming
Event Driven Programming is a popular paradigm due to its multiple benefits across different domains. It supports the creation of flexible, modular, and user-responsive applications.Let's delve into particular areas where Event Driven Programming shines.
Advantages in Software Development
In software development, Event Driven Programming provides numerous advantages that lead to the creation of robust and efficient systems. It caters to the dynamic requirements of modern software solutions.
- Responsiveness: Enables applications to respond promptly to user actions or system inputs, improving user experience.
- Modularity: Allows code reuse by encapsulating event handling logic, making applications easier to maintain and extend.
- Scalability: Facilitates scaling by handling asynchronous and parallel processing effectively, crucial for high-performance applications.
Asynchronous Processing: A method in which tasks are completed independently of the primary program flow, often improving the efficiency and responsiveness of a system.
Consider how Event Driven Programming impacts modern web development. With an event-driven architecture, web servers like Node.js handle requests more efficiently because they manage incoming requests without waiting for the execution of the previous one to finish. Events and callbacks run asynchronously, leading to significant performance benefits.
Utilizing frameworks like React.js for building user interfaces leverages Event Driven Programming to handle user events in a seamless and efficient manner.
Imagine developing a chat application where messages must be sent and received in real-time. With Event Driven Programming, the system listens for incoming messages and dispatches them instantly, ensuring fluid communication among users. Below is a simple representation using JavaScript and Node.js:
const io = require('socket.io')(server);io.on('connection', (socket) => { console.log('a user connected'); socket.on('chat message', (msg) => { io.emit('chat message', msg); });});Here, the socket.on listens for 'chat message' events and broadcasts the message to all connected sockets when an event is detected.
Event Driven Programming in Modern Computing
Event Driven Programming continues to gain significance in modern computing environments, facilitating various cutting-edge technologies and application architectures.With its versatility, this paradigm is helpful across several areas:
- Microservices Architecture: Event driven methods allow for loosely coupled services that can independently handle events, improving fault tolerance and scalability.
- Internet of Things (IoT): Devices react to environmental or user-initiated events to perform actions, crucial for smart technology solutions.
- Cloud Computing: Services handle events and requests over distributed systems, optimizing resource usage and availability.
In the context of IoT, Event Driven Programming enables devices to operate intelligently. For example, smart sensors in a home automation system can trigger events that adjust heating, lighting, or security settings based on input from the environment, demonstrating the paradigm’s power in creating responsive and automated systems.
Event Driven Programming is extensively used in serverless architectures to execute functions in response to events, optimizing cloud resource management.
Consider a modern retail platform that needs to update inventory quantities and notify users in real-time about item availability. By deploying event-driven techniques, every sale, return, or inventory input triggers immediate updates across multiple systems:
const AWS = require('aws-sdk');const sns = new AWS.SNS();exports.handler = async (event) => { const message = JSON.stringify(event.salesData); await sns.publish({ Message: message, TopicArn: 'arn:aws:sns:us-east-1:123456789012:InventoryUpdates' }).promise();};In this serverless computing example, an AWS Lambda function is triggered by inventory events, publishing updates to an SNS topic that notifies the interested parties.
Event Driven Programming - Key takeaways
- Event Driven Programming: A programming paradigm where the application's flow is dictated by events such as user actions, sensor outputs, or messages from other programs.
- Events: Actions or occurrences recognized by software that trigger functions or methods to execute, essential for application interactivity.
- Event Driven Programming Technique: Involves structuring code around events and handlers, including identifying events, setting up listeners, writing handlers, and triggering events.
- Python Event Driven Programming: Utilizes Python's frameworks and libraries like Tkinter and asyncio to manage and control how applications respond to events.
- Event Driven Programming Examples: Examples include a Python GUI application using Tkinter for button clicks and a websocket server using asyncio for message handling.
- Event Driven Programming Exercises: Activities include building a GUI counter, handling keyboard inputs, and logging system events to understand events and handlers interaction.
Learn faster with the 25 flashcards about Event Driven Programming
Sign up for free to gain access to all our flashcards.
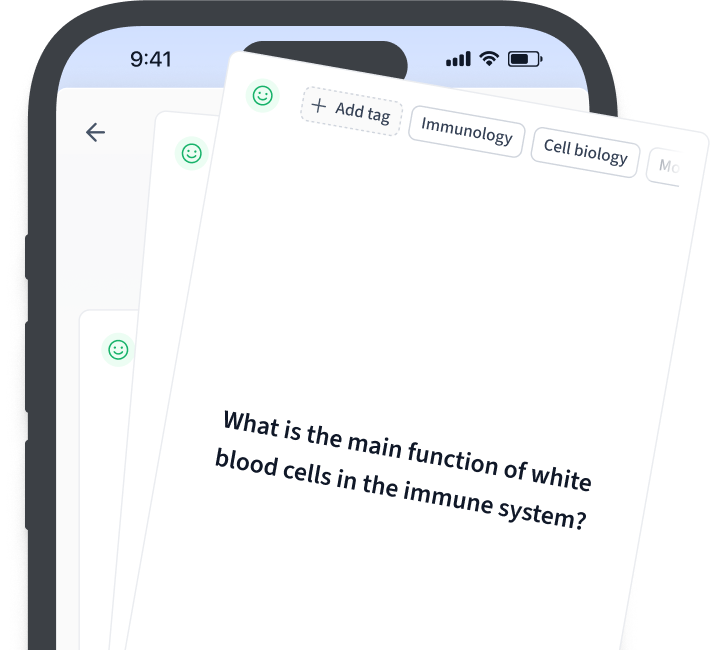
Frequently Asked Questions about Event Driven Programming
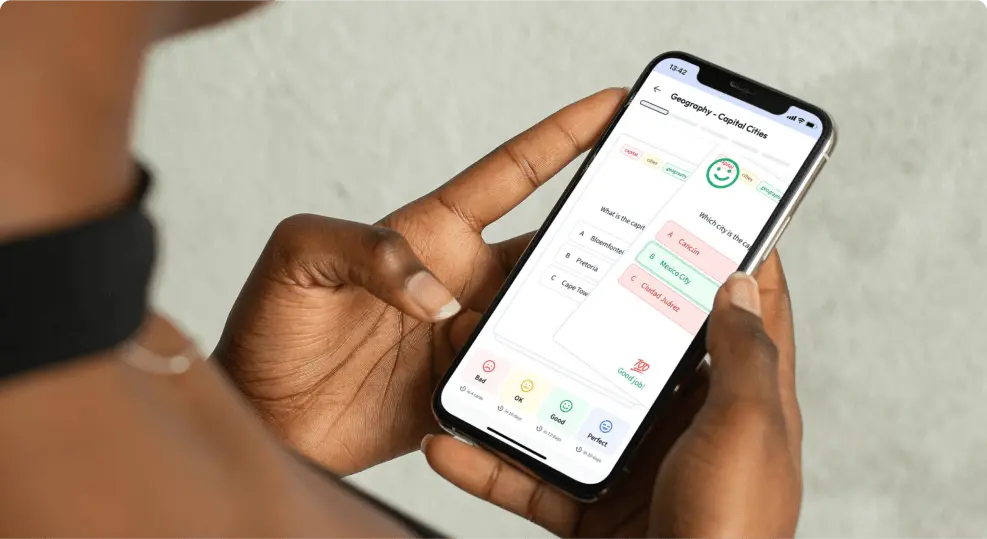
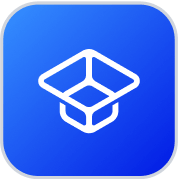
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more