Jump to a key chapter
What is Golang?
Golang, also known simply as Go, is a programming language that has rapidly gained popularity among developers for its simplicity, efficiency, and effectiveness in handling modern computing challenges. Developed at Google by a team of industry veterans, Golang offers a fresh approach to programming that prioritises ease of use and performance.
Origins and purposes of Golang
The story of Golang's origin is closely tied to the need for a scalable and efficient language that could handle Google's enormous computing and networking needs. Designed by Robert Griesemer, Rob Pike, and Ken Thompson in 2007, Golang was specifically created to improve programming productivity in the era of multicore, networked machines, and large codebases.
Golang was engineered to offer a robust standard library, garbage collection, native concurrency support through goroutines, and a straightforward way of managing dependencies. These features were carefully selected to address common issues faced by developers, such as slow compilation and execution times, difficulty in managing dependencies, and complications arising from parallel programming.
Ken Thompson, one of Go's creators, also played a pivotal role in the development of UNIX and UTF-8 encoding.
Understanding Golang's unique features
Golang distinguishes itself with several unique features that contribute to its growing popularity. Here, we'll delve into some of the most notable aspects of the language.
Concurrency model: One of the standout features of Golang is its built-in support for concurrency. Go's concurrency mechanisms, especially goroutines and channels, allow for efficient parallel execution of tasks.Static typing combined with simplicity: Despite being statically typed, Golang offers a high degree of simplicity and readability, making it easier for developers to write and maintain code.Performance: Golang is compiled to machine code, which allows it to run exceptionally fast and makes it suitable for applications that require high performance, such as web servers and data processing tools.
package main import "fmt" func main() { fmt.Println("Hello, World!") }This simple program demonstrates how to print 'Hello, World!' in Golang. Despite its simplicity, this example encapsulates the essence of Golang's design philosophy: allowing developers to achieve a lot with a little.
Goroutines are a fundamental part of Go's approach to concurrency. Unlike threads in other languages, goroutines are lightweight, meaning they use little memory and have low overhead. Go's runtime schedules them onto available CPU cores, enabling highly efficient parallel execution. This design is especially advantageous for writing scalable web servers and handling multiple simultaneous network connections, showcasing Golang's strengths in modern software development challenges.
Golang Basics for Beginners
Embarking on the journey to learn Golang opens up a world of programming possibilities. This gentle introduction aims to demystify the fundamentals of Go, from its straightforward syntax to the efficient ways it handles data types, variables, and functions.
Golang syntax explained
The syntax of Golang is designed to be clean and easy to read. It borrows elements from C but simplifies many aspects to reduce the amount of boilerplate code you need to write. For instance, the absence of semicolons at the end of every statement and the use of simple error handling mechanisms stand out as features designed to enhance code readability and maintainability.
package main import "fmt" func main() { fmt.Println("Learning Golang!") }This example illustrates a basic Golang program structure. Notable points include the package declaration, import statements for external packages, and the main function, which serves as the entry point of the program.
Golang data types and their uses
In Golang, data types are crucial for defining the nature of variables and how they can be manipulated within your program. Golang supports both basic data types such as integers, floats, and strings, and more complex types like arrays, slices, maps, and structures. Understanding when to use each type is key to writing efficient Go code.
Composite data types like slices and maps offer powerful ways to organize and manage data. Slices are dynamically-sized arrays that provide a flexible and efficient way to work with sequences of data. Maps, on the other hand, are key-value stores that are perfect for storing and retrieving data in a key-based manner.
Declaring Golang variables
Declaring variables in Golang is straightforward, with the language offering several formats to suit different needs. Whether you’re initializing variables with explicit types or letting Go infer the type based on assigned values, the clarity and simplicity of Go's variable declaration patterns are designed to keep your code clean and understandable.
var message string = "Hello, Golang!" age := 25In the first line, a variable message is explicitly typed as a string. The second line demonstrates short variable declaration, where age is implicitly typed, with Go inferring the variable’s type from the initial value.
Use short variable declaration for cleaner code and when the type of the variable is clear from the context.
Golang functions: A beginner's guide
Functions in Golang are building blocks that allow you to encapsulate reusable logic. They can take zero or more arguments and can return any number of results. Go’s support for first-class functions, which can be assigned to variables and passed as arguments to other functions, greatly enhances the language's flexibility and expressiveness.
func add(x int, y int) int { return x + y } result := add(2, 3)This example defines a simple function add that takes two integer arguments and returns their sum. It then shows how to call this function and store its result.
Understanding the concept of closure in Golang will unlock powerful programming patterns. Closures are functions that can capture and store references to variables from outside their scope. They are especially useful when you need an inline function that can access and interact with the context in which it was defined.
Control Structures in Golang
In programming, control structures are pivotal, dictating the flow of execution based on conditions and repetitions. Golang, with its concise and efficient syntax, offers a suite of control structures designed to enhance code conciseness and readability.
Mastering the Golang for loop
The for loop in Golang is a versatile control structure capable of iterating over sequences, making it a fundamental tool for developers. Unlike many languages that offer a variety of loop types, Golang opts for simplicity, providing a single for keyword that can handle traditional for loops, while-style loops, and infinite loops with ease.
for i := 0; i < 10; i++ { fmt.Println(i) }This snippet demonstrates a basic for loop, executing a block of code ten times. It initializes a counter i, continues the loop as long as i is less than ten, and increments i by one with each iteration.
Range clause: Used within a for loop to iterate over elements of an array, slice, string, map, or channel. It simplifies code by handling the iteration index or key and value automatically.
colors := []string{"red", "green", "blue"} for index, color := range colors { fmt.Printf("Color at index %d is %s\n", index, color) }This demonstrates a for loop with a range clause, iterating over a slice of colors. It prints both the index and the color, showcasing the range clause's ability to handle both simultaneously.
Use the blank identifier _ to ignore the index or value in a range iteration if it's unnecessary for your logic.
Using Golang control structures effectively
Golang's control structures are designed to organise and manage the flow of control in a program efficiently. Beyond looping, structures such as if, else, switch, and select offer robust mechanisms for conditional execution and decision-making. Leveraging these effectively can lead to more readable, maintainable, and performant Go code.
When working with if statements in Golang, you can initialisation statements, which allow you to streamline code by initialising one or more variables as part of the if statement itself. This is particularly useful for conditions that require a variable's scope to be limited to that of the if or else clauses.
if err := process(); err != nil { fmt.Println(err) } else { fmt.Println("Process succeeded") }This snippet demonstrates an if statement with an initialisation statement, where process() is called, and its returned error is immediately checked. This tight scoping of the err variable optimises readability and maintainability.
The switch statement in Golang does not require a break statement at the end of each case, unlike in many other languages. This simplifies the syntax and makes cases easier to write and understand.
Effectively using Golang's control structures often means adopting idiomatic Go practices. This includes leveraging short-circuiting in logical operations, utilising defer for resource management, and embracing the use of goroutines for concurrency. These practices, coupled with a deep understanding of Go's control structures, can greatly enhance the efficiency and scalability of your code.
Advanced Golang Concepts
Exploring advanced Golang concepts illuminates the depth and versatility of this programming language. This section delves into nuanced topics such as error handling, interfaces, and provides practical examples to showcase Golang's capabilities in real-world programming tasks. Embracing these advanced concepts not only enhances code efficiency and readability but also empowers developers to leverage Golang’s full potential.
Implementing error handling in Golang
Error handling in Golang is uniquely design-driven, advocating for explicit error checking over the use of exceptions. This approach encourages developers to deal with errors as ordinary values, allowing for more controlled error management. Understanding and implementing Golang's error handling paradigms can significantly improve application reliability and maintainability.In Golang, an error is an interface type defined in the standard library, which provides a standard way to report errors in Go programs. Embracing this model enables developers to write resilient code that can gracefully handle failures.
Error interface: The error interface in Golang is one of the language's cornerstone types. It consists of a single method:
Error() stringThis method returns a string describing the error, allowing easy identification and handling.
func divide(a, b int) (int, error) { if b == 0 { return 0, errors.New("division by zero") } return a / b, nil }This function demonstrates simple error handling in Golang. It attempts to divide two numbers and returns an error if the divisor is zero, adhering to Golang’s explicit error checking philosophy.
Use idiomatic Go practices by handling errors where they occur, instead of propagating them as exceptions.
Understanding Golang interfaces
Interfaces in Golang play a pivotal role in achieving polymorphism and designing flexible, modular code. An interface specifies a set of methods but does not implement them - it is up to the types that implement the interface to define the methods. This concept introduces a powerful way of defining contracts within your code without tying yourself to specific implementations.The beauty of Golang interfaces lies in their simplicity and the way they are implicitly implemented. A type implements an interface by implementing its methods, no explicit declaration is necessary.
The io.Reader and io.Writer interfaces are prime examples of interface use in Go. They abstract the reading and writing operations to allow for flexible code that can read from or write to various sources.
This abstraction enables a variety of types, whether files, buffers, or network connections, to be treated uniformly.Practical Golang examples for everyday programming.
Golang's robust standard library and expressive syntax make it an excellent choice for a wide range of programming tasks. From building web applications to scripting, and concurrent programming, Go's simplicity and performance are unparalleled. Here, we'll go through a set of practical examples, showcasing Golang's utility across different programming paradigms.
package main import ( "fmt" "net/http" ) func handler(w http.ResponseWriter, r *http.Request) { fmt.Fprintf(w, "Hello, Golang!") } func main() { http.HandleFunc("/", handler) http.ListenAndServe(":8080", nil) }This web server example exemplifies how Golang simplifies web development tasks. It uses the standard library's http package to listen on port 8080 and serve a simple greeting.
Goroutines and Channels are fundamental to Golang's approach to concurrency, enabling developers to write highly scalable and efficient parallel code with ease. A powerful example is using goroutines and channels to implement a concurrent pattern, such as a worker pool.
- Workers handle tasks concurrently, improving throughput and efficiency.
- Channels facilitate communication and task distribution among goroutines.
- Patterns like worker pools showcase Golang's capabilities in solving complex concurrency challenges.
Golang - Key takeaways
- What is Golang: Golang, also known as Go, is a programming language developed at Google that prioritises simplicity, efficiency, and performance in modern computing.
- Concurrency in Golang: Golang features built-in support for concurrency, including lightweight goroutines and channels for efficient parallel task execution.
- Golang Syntax: The syntax of Golang is clean and readability-friendly, resembling C but with fewer requirements such as semicolons.
- Golang Data Types: Golang supports basic data types like integers and floats, as well as composite types such as slices and maps for organising data.
- Error Interface: Golang handles errors through an interface, encouraging explicit error checking and avoiding exceptions for more reliable code handling.
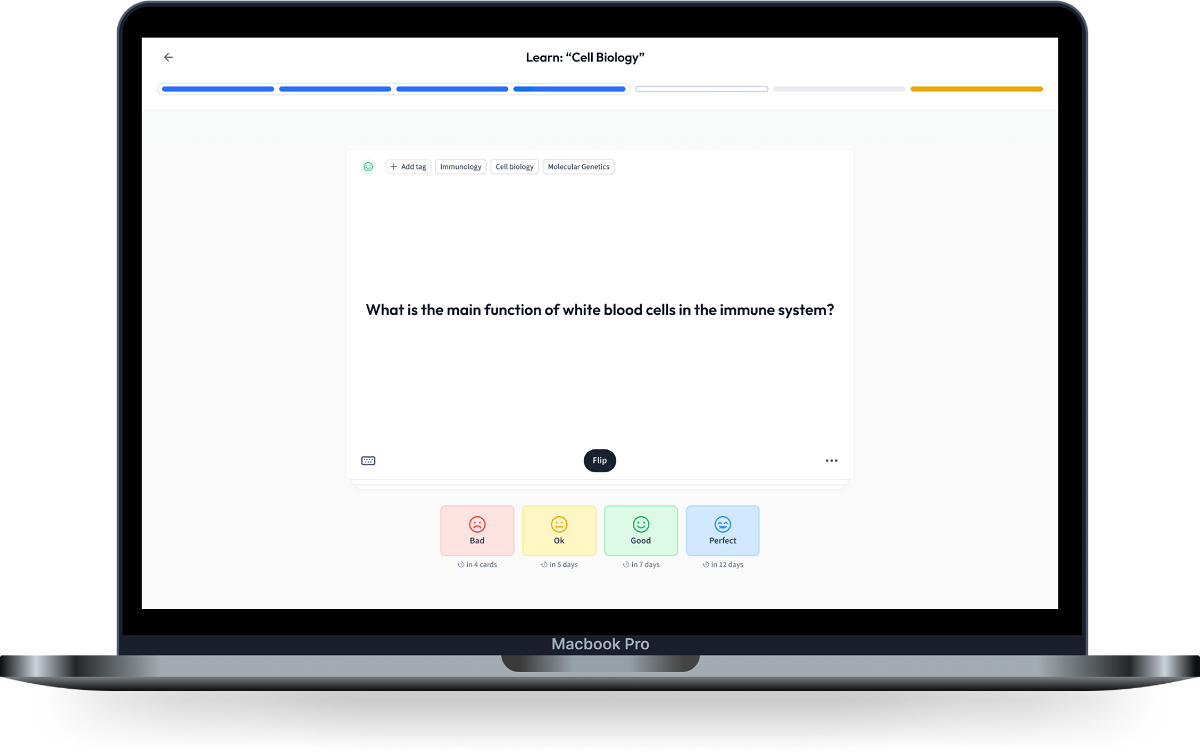
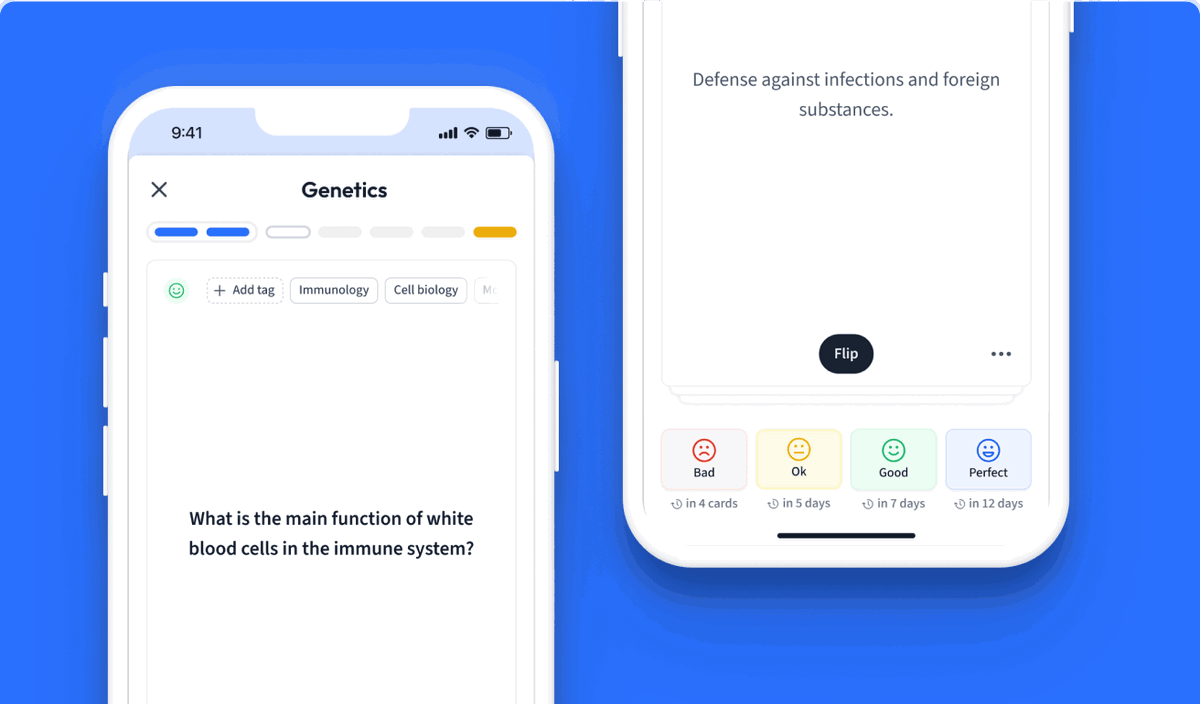
Learn with 27 Golang flashcards in the free StudySmarter app
Already have an account? Log in
Frequently Asked Questions about Golang
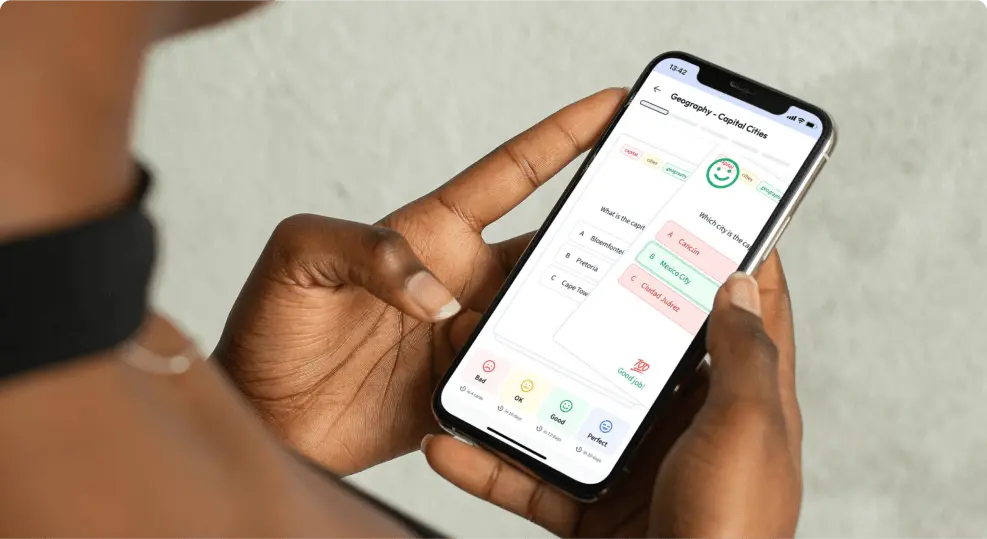
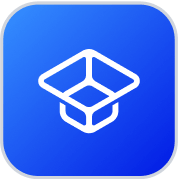
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more