Jump to a key chapter
Identity Operator in Python - Overview
In Python, identity operators are used to compare the memory locations of two objects. They help in determining if two variables point to the same object in memory. Understanding identity operators can be crucial when working with data structures, as it allows you to effectively manage and check data integrity. Let's explore these operators in detail through examples and definitions to better grasp their usage in Python.
What are Identity Operators?
The identity operators in Python are:
- is
- is not
Here is an example using the identity operators in Python:
a = [1, 2, 3] b = a c = a[:] # Creates a shallow copy # Check if b is the same object as a print(b is a) # Output: True # Check if c is not the same object as a print(c is not a) # Output: TrueAs demonstrated, b points to the same object as a, while c does not, creating a separate copy.
It's important to know how identity operators differ from equality operators. While equality operators like =
are concerned with the value an object holds, identity operators focus solely on the object's memory reference. This distinction becomes critical when handling mutable object types such as lists and dictionaries, where changes to one reference could inadvertently alter another if they point to the same object. Memory addresses in Python are essentially the 'id' of an object. Whenever a new object is created, Python allocates a distinct place in memory where the specific object resides. By applying identity operators, you learn if two different variables lead back to this very spot. For example, basic types like integers and strings often exhibit an interesting behavior called interning. Internally, Python may choose to store and reuse these immutable types across your program's runtime to conserve memory. Consequently, certain small immutable objects might naturally have the same memory location without explicitly setting them to be identical.
Identity operators are especially useful when you need to ensure that you are referencing the exact same object instance and not an identical copy.
What are Identity Operators in Python?
In the realm of Python programming, identity operators serve a unique purpose: they are used to determine the memory identity of different objects. While equality operators check if the values of two variables are the same, identity operators check whether two variables share the same memory location. This is particularly important for understanding how objects behave in memory, especially when working with more complex data structures.
Identity Operators are operators that ascertain the memory identity of objects. In Python, these include:
- is - Checks if two variables point to the exact same object in memory.
- is not - Confirms whether two variables point to different objects in memory.
Let's consider the usage of identity operators in a practical example:
a = {'name': 'Alice'} b = a c = {'name': 'Alice'} # Check if b is the same object as a print(b is a) # Output: True # Check if c is not the same object as a print(c is not a) # Output: TrueIn this example, b and a are references to the same dictionary object, while c is a distinct, separate dictionary with the same content.
A deeper understanding of identity operators can elucidate their effectiveness in memory management. Python dynamically allocates memory for every new object instance created during runtime, with each occupying a distinct memory address. The identity operators interrogate these addresses, allowing programmers to confirm object uniqueness and determine relationships between different references. Interestingly, Python optimizes memory usage through a process called interning. This is primarily applied to small strings and integers, where identical immutable objects are stored just once to save space. Thus, under certain conditions, these objects will appear to have the same memory address, a situation where identity operations may show both variables pointing to the same memory location unexpectedly.
- Mutable types like lists and dictionaries retain independent identity.
- Understanding immutability vs mutability aids in grasping Python's memory handling.
Use identity operators judiciously to manage object references, especially when working with complex data structures.
Identity Operator Definition in Python
In Python, identity operators are integral to determining whether two variables point to the same memory location. These operators are often used in situations where memory efficiency and management are crucial. Understanding them is vital for ensuring proper object referencing and manipulation in Python programming.
Identity Operators help determine if two objects are identical by comparing their memory addresses. They include:
- is: Returns True if both variables point to the same object.
- is not: Returns True if both variables point to different objects.
Consider the following Python example illustrating identity operators:
x = ['apple', 'orange'] y = x z = x[:] # Check if y is the same object as x print(y is x) # Output: True # Check if z is not the same object as x print(z is not x) # Output: TrueIn this scenario, y and x share the same memory allocation, but z, being a different copy, doesn't.
Going deeper, understanding identity operators empowers efficient memory management in Python. Every object is assigned a unique identity representing its address in memory. By using identity operators, you resolve these addresses and ascertain the memory relation between variables. Immutable types, such as strings and numbers, benefit from an optimization called interning. This technique allows Python to store a single instance for repeated values, sometimes leading identical literals to share the same memory location. It's important to note, however, that mutable objects like lists or dictionaries will usually have unique identities, even if their content seems identical. When working with large or intricate datasets, identity operators become invaluable in managing how objects are referenced, ensuring you can avoid unintended side effects from shared memory locations.
Identity operators can be surprisingly useful for debugging when you need to ensure that your code distinguishes between object instances properly.
Identity Operator Syntax in Python
The syntax for identity operators in Python is straightforward, yet understanding their application can significantly enhance your coding practices. These operators, is and is not, allow you to check if two variables point to the same object in memory. This capability is especially beneficial when managing complex data structures or when efficiency is of paramount importance.
List Identity Operators in Python
Identity operators in Python include:
- is: Used to ascertain if two references point to the same object.
- is not: Used to check if two references point to different objects.
Consider this Python code demonstrating the identity operators:
a = [4, 5, 6] b = a c = a[:] # Create a separate copy print(b is a) # Output: True print(c is not a) # Output: TrueIn this example, b and a both reference the same list object in memory, whereas c is a distinct copy, even though its content matches that of a.
The concept of identity in Python is tied directly to the concept of an object's memory address. When you create an object, Python automatically assigns it a unique identifier in memory. The identity operators, is and is not, access these identifiers to determine if different references point to the same memory space. When dealing with mutable versus immutable types in Python, it's critical to recognize how identity works.
- Immutable types like numbers and strings may be internally optimized by Python such that identical immutable objects, known as interned objects, share the same identifier.
- Conversely, mutable types like lists and dictionaries typically maintain distinct identifiers unless explicitly set to reference the same object.
Remember that using identity operators is essential when you need to ensure two variables do not just hold equal values but are literally the same object.
Understanding Identity Operators in Python with Example
Identity operators in Python play a crucial role in determining whether two variables point to the same object in memory. These operators are essential in managing how data is stored and accessed within your code, especially when dealing with complex data structures. By comparing memory locations, rather than values, they help maintain data integrity and efficiency.
Practical Examples of Identity Operators in Python
Let's delve into identity operators with some coding examples:
x = 'hello' y = 'hello' z = x # Check if y is x print(y is x) # Output: True, might be True due to interning # Check if z is x print(z is x) # Output: TrueIn this example, x and z are references to the same string object, while y may also show the same identity due to Python's string interning for optimization.
Remember, while identity operators can sometimes show seeming equals as the same object, this holds strongly true for immutable types.
Understanding memory management is pivotal when using identity operators. Every object has a unique identity (its memory address), accessed using the is
and is not
operators. This aspect is more noticeable with mutable objects, which naturally have distinct identifiers unless explicitly assigned to reference the same object. Consider the optimization technique known as interning. This is applied predominantly on small strings and integers to manage resources more efficiently. Repeated occurrences of these objects might share the same space in memory, leading to an identical identity. Mutable types, such as lists, cannot be similarly optimized, thus typically maintain unique identifiers across instances. Therefore, knowing when to apply identity operators goes a long way in making your code both efficient and void of companionship errors, where unintentional changes occur across seemingly different references.
Identity Operator in Python - Key takeaways
- Identity Operator in Python: An operator used to determine if two variables point to the same memory location.
- Identity Operators in Python: They include
is
andis not
. - Identity Operator Syntax in Python: Uses the syntax
variable1 is variable2
andvariable1 is not variable2
. - Functionality: The 'is' operator checks if two variables point to the same object, while 'is not' verifies if they point to different objects.
- Identity Operators with Example: Given variables
a = [1, 2, 3]
andb = a
,b is a
would returnTrue
. - Understanding Identity Operators: Crucial for checking object uniqueness, memory management, and distinguishing between mutable and immutable types.
Learn faster with the 41 flashcards about Identity Operator in Python
Sign up for free to gain access to all our flashcards.
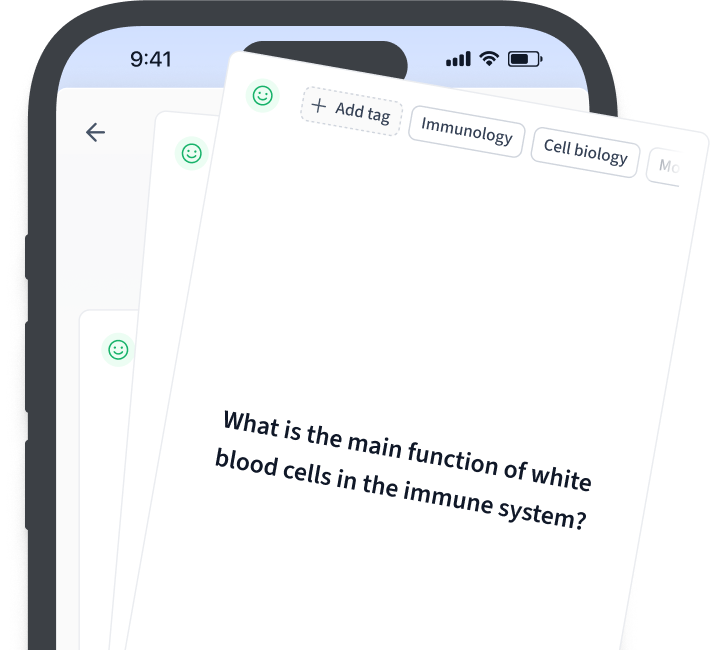
Frequently Asked Questions about Identity Operator in Python
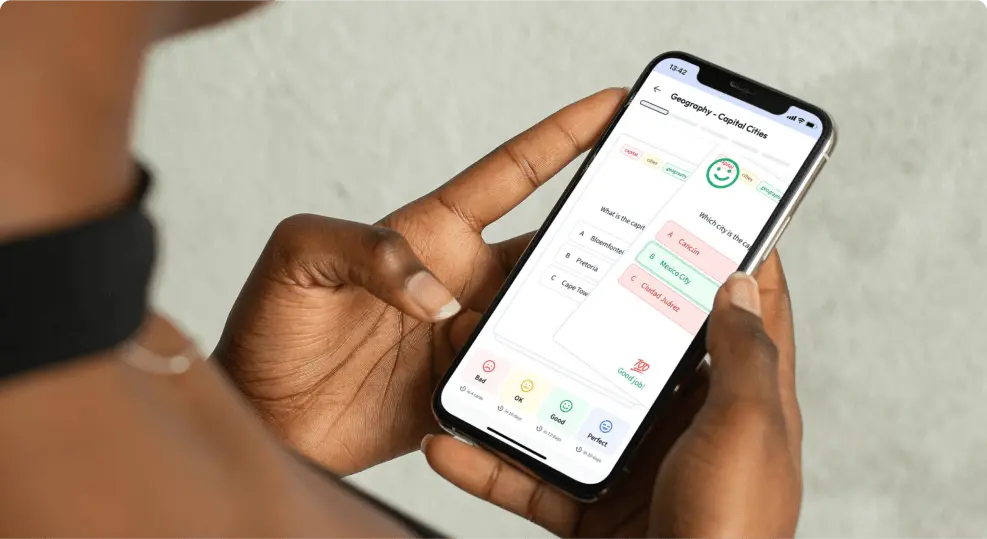
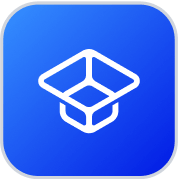
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more