Jump to a key chapter
Understanding Instantiation in Computer Programming
What is Instantiation?
Instantiation is an essential concept in computer programming, mainly in the realm of object-oriented programming (OOP). It is the process whereby a software object, otherwise known as an instance, is produced from a class. In essence, it is the creation of a real instance from a blueprint.
- Key aspects of instantiation include:
- - Creating an instance
- - Assigning initial values or parameters
- - Allocating memory for the instance
In the computer memory, each instance has its separate space and doesn't interfere with the other instances. Variables and functions are unique to the instances and won't affect others.
Relation between Class Instantiation and Object-Oriented Programming
In Object-Oriented Programming, a class can be defined as a template or blueprint from which objects are created. The instantiation of a class is the creation of an object of that class.
Let's consider a classic example comparing a class to a blueprint for a house. The class, like the blueprint, specifies the fundamental structure of the object. However, it does not itself constitute a house. That is where instantiation comes in. When you instantiate a house class, you are effectively building a real, concrete house.
Instantiation in Coding: The Basics
Instantiation involves translating high-level code syntax into hardware-interpretable instructions and allocating resources like memory for the instance.
// A basic instantiation in Java: class Car { String color; int speed; // Instance method void drive() { System.out.println("The car is driving."); } } class Main { public static void main(String[] args) { // Instantiate the Car class Car myCar = new Car(); } }
Step | Description |
Class Declaration | It's the blueprint or a template from which objects are created. Here, Car is the class. |
Instance Method | A non-static method that's called on an instance of an object. In this case, it's the drive() method. |
Instantiation | The 'new' keyword is used to instantiate a class, and a new object is formed. Here, myCar is the instance of the Car class. |
Instantiation in Various Programming Languages
When delving into computer programming, it's key to explore and understand instantiation across various languages. Different languages may have different syntax, but the core concept of creating an object from a class through instantiation remains the same.
The Method of Instantiation in Python
Python is a high-level, interpreted scripting language. It's known for its readability and simplicity. The process of object creation - instantiation - in Python is straightforward and can be achieved using function notation. A class in Python might be defined as follows:class Car: def __init__(self, color, speed): self.color = color self.speed = speedIn this class called Car, note a special method __init__. This method is built into Python classes and is called when an object is instantiated from a class. It's used to initialize the attributes of the class, with parameters being passed at the time of object creation. Here we're setting the 'color' and 'speed' attributes of the Car class, using 'self' to refer to the instance of the class.
How to Instantiate in Python: A Guide for Beginners
Instantiating a class in Python is as simple as calling the class name as if it were a function and passing in whatever arguments its __init__ method accepts. Let's look at the Car class and its instantiation:class Car: def __init__(self, color, speed): self.color = color self.speed = speed myCar = Car('Red', 100)In this Python snippet, we're instantiating the Car class and creating a new Car object with colour 'Red' and speed '100'. The resultant object is named myCar.
Grasping the Concept of Instantiation in Java
Java, an object-oriented programming language, utilises classes and objects like Python. Instantiation in Java requires the 'new' keyword. Here is a simple Java class called Book:public class Book { String title; int pages; public Book(String title, int pages) { this.title = title; this.pages = pages; } }This Book class has a constructor function, which is a special kind of method that determines how the object is initialized when instantiated.
How Does Instantiation Work in Java?
To instantiate this Book class in Java, you would use the new keyword:Book myBook = new Book("1984", 328);This line creates a new instance of the Book class, calls the Book constructor to initialize it, and assigns the new instance to the variable myBook. The instance has title '1984' and pages '328'.
Learning About Instantiation in C++
C++ is another object-oriented language that uses classes and objects. In C++, there's no 'new' keyword used for instantiation. Instead, you instantiate a class by declaring a variable of the class type. Let's consider an example. If you have a class called Student:class Student { public: string name; int age; Student(string n, int a) { name = n; age = a; } };
A Look at Instantiation Techniques in C++
You would instantiate this class in C++ by simply declaring a variable of the class type, like so:Student s1 = Student("John", 21);This line declares a variable s1 of type Student and it gets initialized with the name 'John' and age '21'. This process is the instantiation of the Student class. Thus, while instantiation might look different in each programming language, the underlying principle of creating an instance of a class remains consistent amongst them.
Real-Life Instantiation Examples and Scenarios
In computer science, understanding coding concepts can sometimes be facilitated by comparing them to real-world examples. This holds for the concept of instantiation too, presenting these examples can significantly simplify the learning process.Practical Examples of Class Instantiation
When teaching the concept of instantiation, it can be useful to employ real-world examples. This offers the learner a tangible perspective, enabling them to grasp the idea more efficiently. Consider a bookstore. This can be represented as a class.class Bookstore { String location; int sizeInSqFt; public Bookstore(String location, int sizeInSqFt) { this.location = location; this.sizeInSqFt = sizeInSqFt; } void display() { System.out.println("Bookstore located at " + location + " of size " + sizeInSqFt + " sq ft"); } }A bookstore class can have attributes like location and size. The display() method is used to print the details of the Bookstore. To create an instance of this class, or in real-world terms, to open a new bookstore, you would simply need to invoke the class and pass in the proper attributes. For example, you could open a bookstore in London that measures 1500 square feet.
// Instantiate the Bookstore class Bookstore londonStore = new Bookstore("London", 1500); // Call the display method londonStore.display();This code will print: "Bookstore located at London of size 1500 sq ft". By following this procedure, you can create as many instances (or bookstores) as you need, each with its unique location and size.
Understanding Instantiation Techniques through Examples
While learning about instantiation, having examples in various programming languages is beneficial. They help learners grasp the syntax differences while reinforcing the concept. Let's look at another example, this time using Python and a Website class.class Website: def __init__(self, domain, pages): self.domain = domain self.pages = pagesIn Python, the `__init__` method functions as a constructor, setting the initial values for the object to be instantiated. To create an object from this class, such as a new website, the class is called with the necessary parameters as if it were a function.
# Instantiate the class google = Website('www.google.com', 100)This new website 'google' has a domain of 'www.google.com' and hosts 100 pages. The process is simple, and by this technique, you can create any number of website instances as needed.
How Real-World Scenarios Translate to Instantiation in Coding
The translation of real-world scenarios to instantiation in coding is a helpful way to understand the concept correctly. Let's liken the procedure of creating objects to attending a school. Consider the 'School' as the class, with attributes including 'name', 'location', and 'noOfStudents'. In real-world terms, creating an instance of this class would be like opening a new school. Each school is a manifestation of the 'School' class but has its unique name, location, and number of students.class School: def __init__(self, name, location, noOfStudents): self.name = name self.location = location self.noOfStudents = noOfStudents # Creating instances of the School class school1 = School('Greenwood High', 'London', 500) school2 = School('Oakridge', 'Cambridge', 300)In the code, school1 and school2 are distinct instances of the 'School' class, each with its name, location, and number of students. This example illustrates how specific scenarios can be coded by defining a class and creating instances, an essential aspect of object-oriented programming. By understanding these examples and translating real-world scenarios into classes and objects in coding, you can effectively grasp the concept of instantiation.
Exploring the Role of Instantiation in Software Development
The Significance of Instantiation in Programming Projects
In the realm of software development, instantiation plays an invaluable role. It is at the heart of object-oriented programming (OOP), permitting you to create actual objects or instances from a class blueprint. This process is crucial for a multitude of reasons:- Using objects: Once you've established classes (blueprints), you can use them to create multiple instances (objects). The instances have the properties and methods defined in its class, allowing programming tasks to be carried out.
- Memory efficiency: As all instances of a class share the same methods and perform the same actions, instantiation enables memory efficiency. The code for the methods needs to be stored only once, irrespective of the number of objects.
- Code reuse: One of the primary principles of OOP is code reusability, and instantiation is key to this aspect. Once a class is created, it can be used to create multiple objects across different parts of a program or even in different programs. This significantly reduces the amount of code that needs to be written.
How Instantiation Technique Facilitates Software Development
For each class that you design, you have the ability to create numerous instances, each with their unique state and behaviours. Through doing so, you can replicate real-world objects and scenarios, which makes object-oriented software development such a powerful tool. To illustrate this, consider designing a shopping website. You can create a 'Product' class with attributes like 'name', 'price', and 'stock' and methods such as 'addToCart()' and 'checkOut()'. For each product available on the website, you merely need to create an object of the 'Product' class and initialise it with the specific details of each product.class Product { String name; int price; int stock; // Methods void addToCart(int quantity) { // Code for adding to cart } void checkOut() { // Code for checkout } } // Instantiate the class Product football = new Product("Football", 30, 100); Product basketball = new Product("Basketball", 40, 80);By doing so, you create various products like 'football' and 'basketball' each with their name, price, and stock, and equipped with the actions 'addToCart()' and 'checkOut()'. This is where the functionality of instantiation shines. It allows you to design classes that closely represent real-world objects and offer the functionality required to manipulate them.
The Impact of Class Instantiation on Coding Efficiency
One of the essential goals of any programming project is to optimise both the time taken to write the code and the efficiency of the finished application. Instantiation is fundamental to that goal. By defining a class, you're setting up a template that can be used to create as many instances as required. This promotes code reuse and decreases the overall amount of code written. Furthermore, the encapsulation of data (attributes) and behaviours (methods) within objects leads to clean, organised code. Each object manages its state and provides interfaces (methods) to interact with that state. This encourages a separation of concerns, where each class focuses on a specific task. When the code is neatly organised, it becomes easier to understand, modify, and maintain, paving the way for more efficient coding and robust applications. All in all, class instantiation paves the way for an optimal coding process and outcome in software development. The convenience, code efficiency, and real-world modelling capabilities provided by class instantiation bear testimony to its critical role in software development.Prevailing Challenges with Instantiation and Ways to Overcome Them
While the concept of instantiation enhances the functionality of OOP languages, certain difficulties can occur in the process. The complexities primarily arise from an insufficient understanding of classes and objects, and their roles in programming. Furthermore, the errors or issues can vary across languages, with each having its idiomatic challenges.Common Missteps with Instantiation in Python, Java, and C++
As each programming language has unique syntax and semantics, the challenges faced during instantiation tend to vary.Misunderstandings in Python
Python's dynamic typing can sometimes lead to confusion, especially for beginners. Failure to understand that classes are mutable can lead to errors.class MyClass: list = [] # Create two objects object1 = MyClass() object2 = MyClass() # Add to list of object1 object1.list.append('item') # Print list of object2 print(object2.list)The output of the above code would be ['item'], which might be surprising! This is because the list attribute belongs to the class, not to instances of the class. Therefore, modifications to the attribute by one object have an effect on all other objects of the class. To avoid this, make sure to create instance variables inside methods:
class MyClass: def __init__(self): self.list = []
Instantiation hurdles in Java
In Java, one common error is forgetting the 'new' keyword when creating an object, as it is required for object instantiation.Dog myDog; // Incorrect Dog myDog = new Dog(); // CorrectThe first line simply declares a variable of type Dog, but no Dog object is created, leading to a null pointer exception if you try to use myDog. The second line correctly instantiates a Dog object.
Instantiation confusion in C++
In C++, one tricky area involves constructor selection during instantiation.class MyClass { public: MyClass() {} MyClass(int a) {} }; MyClass m = MyClass(); // This line does not do what you may expect!This code doesn't call the default constructor as one might expect. Instead, it calls the copy constructor (which the compiler provides by default), resulting in a different object behaviour than expected.
Troubleshooting Tips for Smooth Instantiation in Coding
To navigate through these challenges, here are some general solutions:- Learn the language syntax: Understand the syntax for instantiation in the language you're learning. This includes knowing the appropriate keywords, how to call a constructor, etc.
- Master classes and objects: Ensure you have a strong grasp of classes and objects in the language. Especially focus on the difference between class variables and instance variables to avoid unexpected behaviour.
- Learn the roles of constructors: The constructor plays a crucial role in instantiation so knowing its syntax, roles and how to selectively call them will be beneficial.
- Error understanding: Review the common errors tied to instantiation in the language and the ways to resolve them.
Tips to Master Instantiation Techniques for Effective Coding
The key to effectively using instantiation lies in understanding the concepts and practising a lot. Some general tips to master these techniques include:- Make sure you understand the basics of OOP. This includes concepts like classes, objects, inheritance, polymorphism, etc.
- Practice creating and using classes and objects. One of the biggest steps towards mastering instantiation is getting comfortable with using classes to model real-world concepts.
- Experiment with different kinds of classes, including classes with various types and numbers of instance variables and methods.
- Try out different programming projects that require creating and manipulating numerous instances of a class.
Overcoming Hurdles in Instantiation: A Student's Guide
While encountering issues in coding is normal, especially while learning, overcoming them is crucial. Here are some guidance points to help you as a student:- Reading and Understanding error messages: They help reveal what's going wrong with your code. Interpret them, spot the issue, and you'll learn how to debug more effectively.
- Proper debug practice: While it takes time and patience, debugging step-by-step will help you determine where your code diverges from its expected behaviour. Tools like breakpoints and watchers in Integrated Development Editors (IDEs) can be handy.
- Master object-oriented programming: OOP is at the heart of instantiation. The better you understand it, the more adept you are likely to be at using instantiation correctly and efficiently.
- Understand the language: Each language has its peculiarities. Understanding them is useful in context. For instance, augmenting class variables when intending to manipulate an instance variable, forgetting to use 'new' keyword in C++ or Java, must be considered.
Instantiation - Key takeaways
- Instantiation is the process of creating an object from a class in programming and can be done in different ways depending on the language.
- In Python, instantiation is straightforward and using the special method __init__, which is called when an object is instantiated from a class. This method is used to initialize the attributes of the class.
- In Java, instantiation is performed using the 'new' keyword and a special method called a constructor function which initializes the object.
- C++ instantiation doesn't use the 'new' keyword. Instead, a class is instantiated by declaring a variable of the class type.
- Real-world examples can significantly simplify learning of the concept of instantiation. The concept can be likened to real-world objects or scenarios, with a class representing a model of a real-world entity, and each instance representing a specific example of that entity.
- Instantiation plays a crucial role in object-oriented programming, facilitating code reuse, memory efficiency, and the encapsulation of data and behaviors within objects, leading to clean, organized code. This significantly reduces the amount of code that needs to be written, thus increasing coding efficiency.
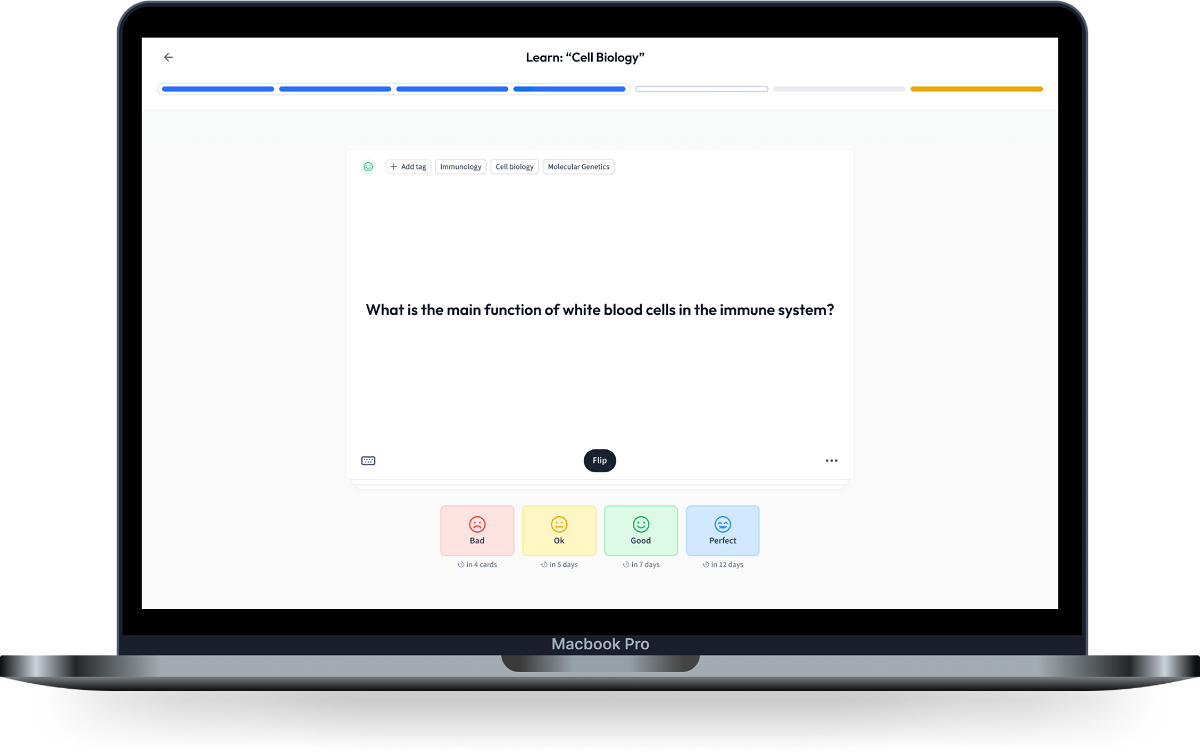
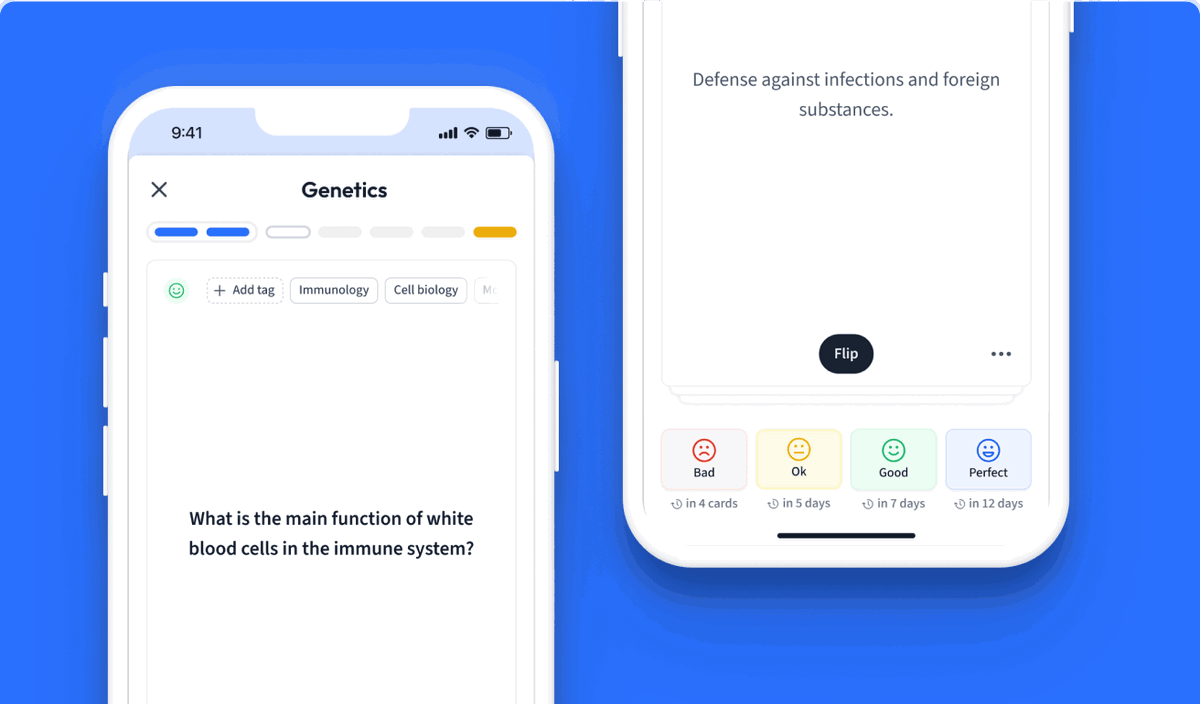
Learn with 15 Instantiation flashcards in the free StudySmarter app
Already have an account? Log in
Frequently Asked Questions about Instantiation
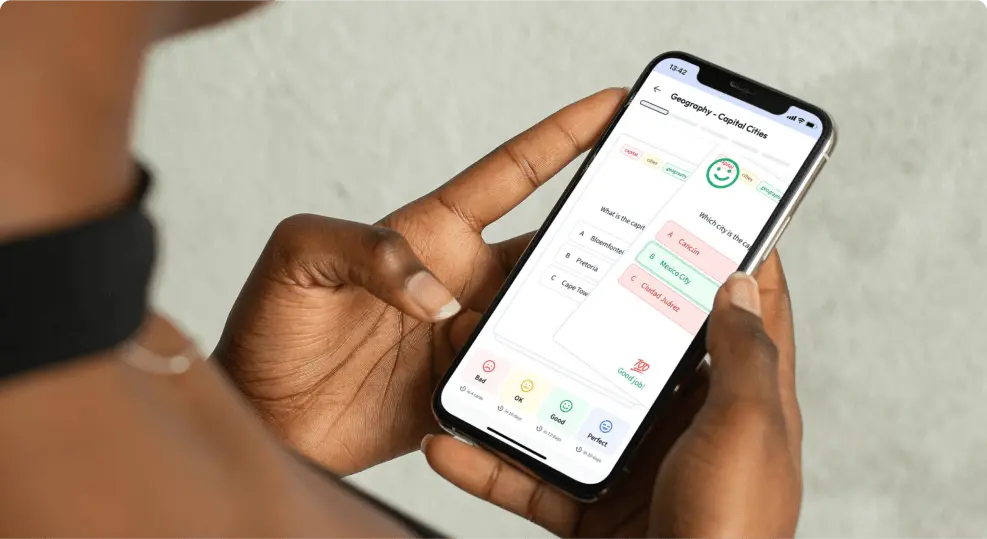
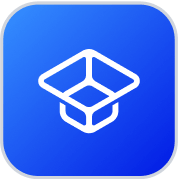
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more