Jump to a key chapter
Java Abstraction Overview
In Java programming, abstraction is a key concept that helps in simplifying complex reality by modeling classes based on essential properties and ignoring the irrelevant details. Through abstraction, you can focus on the functionalities you need without diving into the implementation details. This overview will walk you through the essentials of Java abstraction.
Understanding Abstraction in Java
Abstraction is the process of hiding the implementation details and exposing only the functionality to the user. In Java, abstraction is achieved through abstract classes and interfaces.
Here are the two key mechanisms:
- Abstract Classes: Used when you want to provide a common base class with some implementation that subclasses can take advantage of.
- Interfaces: Used when you expect unrelated classes to implement your interface and the interface doesn't need to store state.
By using abstraction, it's easier to manage complexity and change your program with minimal impact, enhancing its scalability.
Java Abstraction is a mechanism that provides abstraction by hiding implementation details and exposing only the required operations to the users using abstract classes and interfaces.
Consider a simple banking application:You might have two types of accounts, SavingsAccount and CurrentAccount, both having common operations like deposit and withdraw. Here's a simplified illustration of an abstract class:
abstract class Account { double balance; public abstract void deposit(double amount); public abstract void withdraw(double amount); public double getBalance() { return balance; }}
Now, both SavingsAccount and CurrentAccount will inherit from this abstract class and provide their specific implementations for the deposit and withdraw methods.
Think of abstraction as a way to define a contract that tells what the functions in your class should do, not how they do it.
Abstract Classes versus Interfaces
When deciding between using abstract classes or interfaces, consider the following:
Abstract Classes | Interfaces |
Can have both abstract and non-abstract methods. | Can only have abstract methods (before Java 8). |
Can have instance variables. | Cannot have instance variables. |
Can provide method implementations. | Cannot provide method implementations (before Java 8). |
Can use the extends keyword for inheritance. | Use the implements keyword for implementation. |
By understanding these differences, you'll better know when to use each in your Java applications.
With the introduction of default methods in interfaces from Java 8, interfaces are now allowed to have method bodies. This evolution leads to a debate on the blending of abstract classes and interfaces. Default methods bring some of the functionality that was previously limited to abstract classes into interfaces. This change was introduced to ensure backward compatibility with existing interfaces.
It's important to note that default methods still differ from methods in abstract classes in several ways:
- Default methods are overridden in implementing classes, providing concrete implementations if needed.
- Abstract classes can have constructors, interfaces cannot.
The shift also allows for the mixing of features where both abstract class hierarchy and interface hierarchy can coexist, promoting flexible code design and modularity.
Definition of Abstraction in Computer Science
Abstraction in computer science is a fundamental concept that refers to simplifying complex systems by focusing on the core aspects and ignoring the superfluous details. This technique allows you to manage complexity by working with models that are less detailed yet still fully functional.
Abstraction is utilized across various programming languages and paradigms, helping you to create more efficient and manageable code. It allows you to design and work with large, complex systems without overwhelming detail.
Abstraction is a concept in computer science that involves reducing complexity by focusing on the essential characteristics and behaviors of an object, while hiding the less relevant aspects.
Imagine you're building a simplified model of a car's transmission system to understand how power is transferred. You would deal with components like gears and shafts without delving into materials or manufacturing techniques. This example illustrates abstraction by highlighting necessary functionalities and neglecting irrelevant details.
Abstraction helps in understanding systems at different layers, making interaction between parts efficient without knowing every detail of the other components.
In the hierarchy of computer science, abstraction is often implemented at multiple levels:
- Data Abstraction: Focuses on representing data in terms of abstract data types, which are more intuitive and easier to manage.
- Control Abstraction: Involves the use of control structures to command logical flow without detailing specifics.
- Procedural Abstraction: Encapsulates operations with procedures and functions, abstracting the precise calculations involved.
Each level contributes toward making software more modular, easier to understand, and more adaptable to changes.
Abstract Class Java and Its Uses
In Java programming, an abstract class is an essential feature that enables you to define classes with abstract methods that subclasses need to implement. It helps to establish a common blueprint while allowing specific details to be provided by different subclasses. Abstract classes cannot be instantiated directly, but they can define fields and methods that all subclasses can inherit.
Using abstract classes is particularly useful in scenarios where you have a group of closely related classes and want to share some common code among them. This approach promotes code reusability and organization, making it easier to maintain and enhance your Java applications.
An abstract class in Java is a class declared with the abstract keyword, which may contain abstract methods (without implementations) and non-abstract methods (with implementations). Abstract classes cannot be instantiated and are intended to be inherited by subclasses that provide implementations for the abstract methods.
Consider a geometric application where you need to define different shapes. An abstract class named Shape could serve as the base for various types of shapes like Circle, Rectangle, and Triangle. Here's an abstract class example:
abstract class Shape { String color; // Abstract method (does not have a body) abstract double area(); // Method with implementation public String getColor() { return color; }}
Each subclass like Circle would then inherit from Shape and provide a specific implementation for the area() method.
An abstract class is like a template where the subclass fills in the details, ensuring a blueprint with some shared functionality is carried over.
Abstract Method in Java
An abstract method in Java is a method that is declared without an implementation, using the abstract keyword. These methods act as placeholders for functionality that must be defined in subclasses. Abstract methods ensure that subclasses implement specific behaviors while allowing the superclass to focus on higher-order logic.
The advantage of using abstract methods is that they enable you to define methods that the subclasses are expected to implement, thus ensuring a contract between the abstract class and its subclasses. This mechanism helps in enforcing design patterns and ensuring coherent behavior across different class implementations.
Abstract Method | Description |
Declaration | Uses the abstract keyword and ends with a semicolon. |
Implementation | Must be provided in the subclass. |
Abstract methods are part of an abstract class's mechanism to enforce implementation details in subclasses. They are crucial in defining API contracts and play an integral role in structuring code that adheres to certain design principles like the Template Method Pattern. By requiring subclasses to implement abstract methods, you facilitate polymorphism in your code, making it easier to introduce new behavior by simply defining new subclasses. Abstract methods combined with fully implemented methods offer a way to let class hierarchy provide a rich set of functionalities while customizing specific parts.
Examples of Java Abstraction
Java abstraction allows you to create blueprints for classes that mandate defining certain functionalities while hiding unnecessary details. To better understand this concept, consider practical examples that showcase how abstraction is implemented in Java.
Bank Account Example
In a banking application, you might have a variety of accounts such as savings accounts and current accounts, all sharing some common operations—like depositing or withdrawing money. Here, abstraction can be achieved using an abstract class to define these common behaviors.
abstract class BankAccount { double balance; public abstract void deposit(double amount); public abstract void withdraw(double amount); public double getBalance() { return balance; }}
This core BankAccount class provides a template for specific account types to define their methods. Each subclass can also extend this abstract class to provide additional functionalities.
Let's illustrate further with a specific account type:
class SavingsAccount extends BankAccount { public void deposit(double amount) { balance += amount; } public void withdraw(double amount) { if(balance >= amount) balance -= amount; }}
The SavingsAccount class provides specific implementations of deposit and withdraw methods, fulfilling the template's obligations in the BankAccount abstract class.
When using abstraction, think of it as creating a contract for others to follow. This ensures consistency across different implementations.
Shape Drawing Example
When creating a drawing application, you're likely working with various shapes that have similar characteristics but distinct operations. Abstract classes and interfaces facilitate this process by defining a common structure for different shapes.
interface Drawable { void draw();}abstract class Shape implements Drawable { abstract double area();}
Here, the Shape abstract class can be extended by specific shapes like Circle and Rectangle, each having a dedicated area calculation while mandating the draw function.
Within a drawing library or simulation graphics engine, different geometric shapes require interface or abstract class definitions to ensure they implement consistent behaviors. Abstract classes play a significant role in defining core elements like spatial positions, color, and transformations, which all derived shapes inherit. These designs allow creating rich user interfaces, scenarios, and backend modeling with ease.
Consider a more complex rendering scenario, where you further manage resources effectively:
- Memory Management: Reusing objects through abstraction can reduce memory footprint.
- Performance Optimization: Centralized control over rendering procedures for optimization purposes.
Java Abstraction - Key takeaways
- Definition of Abstraction in Java: It is a process of hiding the implementation details and exposing only the functionality to the user, achieved through abstract classes and interfaces in Java.
- Abstract Classes in Java: These provide a common base with some implementations that subclasses can inherit and extend, including both abstract and non-abstract methods.
- Interfaces in Java: Used when you expect unrelated classes to implement the same set of methods without saving state, enhanced with default methods from Java 8.
- Abstract Method in Java: Defined without implementation in an abstract class, requiring subclasses to provide specific implementations, ensuring consistency across different class implementations.
- Examples of Java Abstraction: In banking or drawing applications, abstract classes define common behaviors while allowing specific implementations in subclasses like SavingsAccount or Circle.
- Definition of Abstraction in Computer Science: A fundamental concept for simplifying complex systems by focusing on essential characteristics and abstracting from unnecessary details, applicable across various programming paradigms.
Learn faster with the 24 flashcards about Java Abstraction
Sign up for free to gain access to all our flashcards.
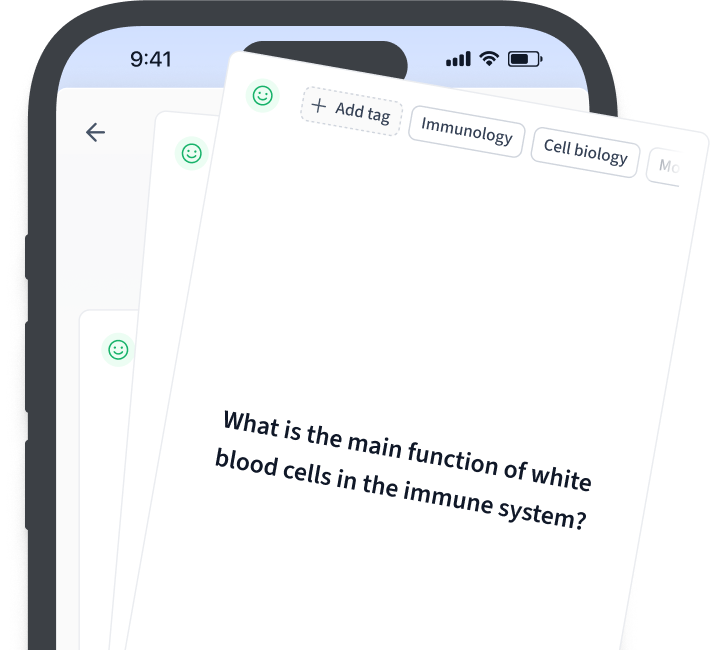
Frequently Asked Questions about Java Abstraction
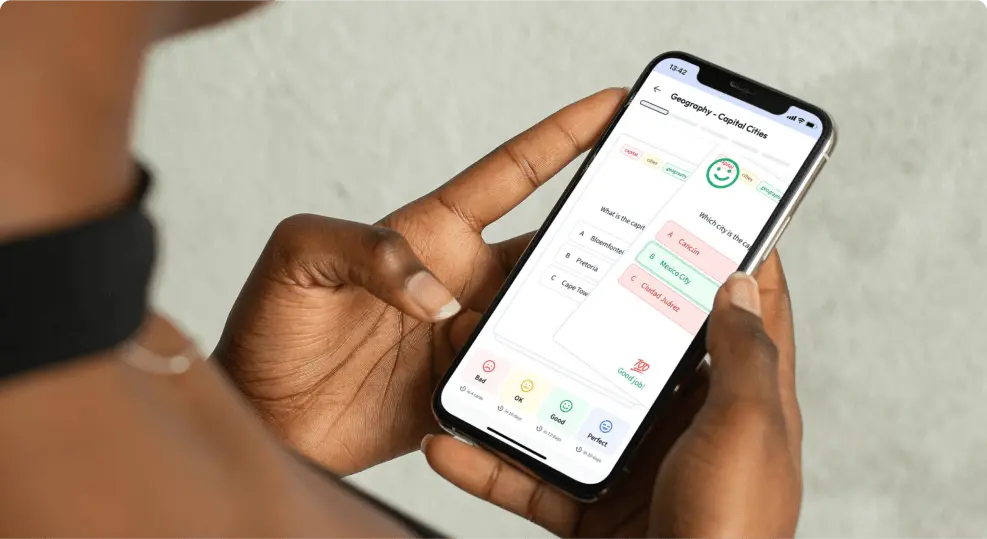
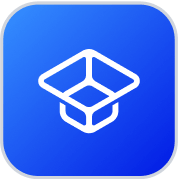
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more