Jump to a key chapter
Java Arraylist Definition and Uses
Java ArrayList is a part of the Java Collections Framework and is used to store a dynamically sized collection of elements. Unlike arrays that have a fixed size, ArrayLists can increase and decrease their capacity as objects are added and removed.
Definition of Java Arraylist
Java ArrayList is a resizable array implementation in Java's standard library. It allows for dynamic data structures in contrast to static arrays.
The ArrayList class is found in the java.util package, making it easy to use across Java applications.Some essential characteristics of ArrayLists include:
- Ability to store elements of various data types through generics.
- Capacity to automatically adjust as more elements are added.
- Maintain insertion order, allowing the same ordering of elements as they are inserted.
Uses of Java Arraylist
ArrayLists are frequently utilized in Java for several common tasks, including:
- Storing dynamically growing collections where the fixed size is a limitation.
- Manipulating collections with frequent additions and deletions.
- Facilitating data exchange, retrieval, and modification compared to standard arrays.
Example of Using Java ArrayList:
import java.util.ArrayList; public class Main { public static void main(String[] args) { // Create a new ArrayList ArrayListThis code snippet illustrates how to create an ArrayList, add elements, and print the list to the console.list = new ArrayList<>(); // Add elements list.add('Apple'); list.add('Banana'); list.add('Cherry'); // Display the ArrayList System.out.println(list); } }
Java ArrayLists are not synchronized, meaning they are not thread-safe by default. Consider using Collections.synchronizedList if multi-threaded access is anticipated.
Performance Characteristics of Java ArrayListArrayLists provide efficient random access, making them highly suitable for scenarios where such access patterns are predominant. However, when it comes to inserting and deleting elements, the performance might degrade, especially for larger lists, as these operations require shifting elements. The time complexity for accessing an element by its index is O(1), while for insertion, deletion, and search operations, it is O(n). Understanding these complexities is crucial when optimizing applications that rely on heavy list manipulations.
Java Arraylist Examples for Beginners
When starting with Java ArrayList, it's important to understand how to create, manipulate, and utilize them in your projects. ArrayLists offer great flexibility due to their dynamic nature, allowing you to perform a wide array of operations effectively.
Creating and Initializing an ArrayList
Basic Example of ArrayList Creation:
import java.util.ArrayList; public class Example { public static void main(String[] args) { // Create an ArrayList to store integers ArrayListThis code demonstrates initializing an ArrayList with integer values and printing it.intList = new ArrayList<>(); // Initialize with values intList.add(10); intList.add(20); intList.add(30); // Print ArrayList System.out.println(intList); } }
Manipulating Elements in an ArrayList
You can perform various operations on an ArrayList, including adding, removing, and retrieving elements. Here's how you can do each: - **Add Elements:** Use add()
method. - **Remove Elements:** Use remove()
method by specifying the index or the object itself. - **Access Elements:** Use get()
method by specifying the position index.These methods provide you with the tools needed to manipulate the list dynamically.
Example of Manipulating ArrayList:
ArrayListThe example shows how to add, remove, and access elements in an ArrayList.fruits = new ArrayList<>(); // Adding elements fruits.add('Apple'); fruits.add('Banana'); fruits.add('Cherry'); // Removing an element fruits.remove('Banana'); // Accessing an element System.out.println(fruits.get(0)); // Prints 'Apple'
Iterating Over an ArrayList
To process elements within an ArrayList, you might need to iterate over it using loops. The for-each loop is commonly used due to its simplicity: - Iterating allows you to access each element in sequence. - Useful for operations such as displaying elements or computing totals.
Example of Iterating Through an ArrayList:
ArrayListThis example demonstrates iterating over an ArrayList and printing each country.countries = new ArrayList<>(); countries.add('USA'); countries.add('Canada'); countries.add('UK'); // Iterate using for-each loop for (String country : countries) { System.out.println(country); }
When iterating over an ArrayList, using the
loop is more concise compared to a traditional for
loop, especially when you do not need the index.
Advanced ArrayList Operations and ConsiderationsBeyond basic manipulation, ArrayLists have advanced methods like clear()
to remove all elements, contains()
to check for an element's existence, and indexOf()
to find the index of a specific element.The choice between an ArrayList and a traditional array should be guided by the application needs. While ArrayLists provide flexibility with size, they generally incur overhead with resizing and boxing/unboxing operations when dealing with primitive data types.
Techniques for Using Arraylist in Java
To effectively utilize a Java ArrayList, you must understand some essential techniques. An ArrayList offers flexibility in storing dynamic data, but leveraging its full power requires knowledge of various methods and strategies.
Adding and Modifying Elements Efficiently
To add elements to an ArrayList, use the add()
method. This is one of the most straightforward operations, but how you manage additions can significantly impact performance if your list becomes large.Consider pre-sizing your ArrayList with the ensureCapacity(int minCapacity)
method to reduce the overhead of resizing:
- Initial Capacity: Define if the approximate number of elements is known in advance.
- Adding at Specific Index: Use
add(index, element)
to insert elements at specified positions.
Example of Pre-sizing an ArrayList:
ArrayListThis snippet demonstrates how to initialize and resize an ArrayList to accommodate up to 100 elements efficiently.numbers = new ArrayList<>(50); numbers.ensureCapacity(100);
Sorting and Searching in an ArrayList
ArrayLists can be easily sorted and searched, which is vital when dealing with data-intensive applications. You can use the Collections.sort()
method for sorting operations. For searching, use Collections.binarySearch()
after sorting, as it requires an ordered list. These methods are optimal for maintaining structured data flow and quick retrieval.
Example of Sorting an ArrayList:
import java.util.ArrayList; import java.util.Collections; public class SortArrayList { public static void main(String[] args) { ArrayListThis example sorts an ArrayList of strings alphabetically.list = new ArrayList<>(); list.add('Apple'); list.add('Mango'); list.add('Banana'); Collections.sort(list); System.out.println(list); } }
Understanding Sorting Algorithms in JavaJava uses a variant of the Timsort algorithm for sorting objects in an ArrayList. Timsort combines the advantages of merge sort and insertion sort, offering better stability and performance in various real-world applications. This reinforces the efficiency of Collections.sort()
for small and large datasets alike.
Using Iterators for Custom Operations
While loops and for-each constructs are straightforward for iterating over ArrayLists, Iterators
provide more controlled traversal, particularly useful for concurrent modifications or applying custom operations on data.Iterators allow you to iterate through an ArrayList using the hasNext()
and next()
methods and even remove elements safely with iterator.remove()
.
Example of Using an Iterator:
import java.util.ArrayList; import java.util.Iterator; public class IteratorExample { public static void main(String[] args) { ArrayListThis example shows how to remove an element ('Banana') from an ArrayList using anlist = new ArrayList<>(); list.add('Apple'); list.add('Banana'); list.add('Cherry'); Iterator iterator = list.iterator(); while (iterator.hasNext()) { String fruit = iterator.next(); if (fruit.equals('Banana')) { iterator.remove(); } } System.out.println(list); } }
Iterator
. When working with Iterators, remember that attempting to modify the ArrayList directly during iteration can lead to ConcurrentModificationException
. Use the remove()
method of the iterator.
Convert 2D Array into Arraylist Java
A common task in Java is converting a 2D Array into an ArrayList. This can be useful for dynamically managing elements that originate from a static array structure. Understanding how to manipulate data from a 2D array into an ArrayList increases the versatility of your code.
Converting Integer Array to Arraylist in Java
To convert an integer-based 2D array into an ArrayList, you can iterate over the array's rows and columns, adding each element to an ArrayList. This approach ensures that the ArrayList holds the same values and structure as the original 2D array.This method involves initializing an ArrayList for each sub-array (row) and then adding that list to a main ArrayList. Here's how you can accomplish this:
Example of Converting 2D Array to ArrayList:
import java.util.ArrayList; import java.util.Arrays; public class ArrayConversion { public static void main(String[] args) { int[][] array = { {1, 2, 3}, {4, 5, 6}, {7, 8, 9} }; ArrayListThis example effectively transforms a 2D array into an ArrayList of ArrayLists, preserving the data layout.> list = new ArrayList<>(); for (int[] row : array) { ArrayList rowList = new ArrayList<>(); for (int num : row) { rowList.add(num); } list.add(rowList); } System.out.println(list); } }
Alternative Data Structures for Converting ArraysAn ArrayList is a flexible alternative to arrays as it allows variable-length dynamic collections. For specific cases where additional functionality is needed—such as constant time access and update—a data structure like Arrays.asList() can initially wrap the arrays before conversion, though it does not support modifications like an ArrayList.
Synchronizing an Arraylist Java
When working in a multi-threaded environment, synchronizing your ArrayList ensures thread safety. An unsynchronized ArrayList is prone to ConcurrentModificationException when accessed by multiple threads concurrently. To synchronize an ArrayList, you can wrap it using Collections.synchronizedList()
which offers a straightforward solution.Here’s how you can synchronize an ArrayList:
Example of Synchronizing an ArrayList:
import java.util.ArrayList; import java.util.Collections; import java.util.List; public class SyncExample { public static void main(String[] args) { ListThis example illustrates creating a synchronized ArrayList for thread-safe operations.list = new ArrayList<>(); list.add('Apple'); list.add('Banana'); // Synchronize the ArrayList List syncList = Collections.synchronizedList(list); synchronized(syncList) { for (String fruit : syncList) { System.out.println(fruit); } } } }
Remember, even when an ArrayList is synchronized, iteration over it must be done in a synchronized block to maintain thread safety.
Java Arraylist - Key takeaways
- Java ArrayList Definition: A part of the Java Collections Framework, Java ArrayList is a dynamic array that allows the resizing of elements as needed, unlike fixed-size arrays.
- Characteristics: Supports generics for various data types, maintains insertion order, and allows dynamic capacity adjustments.
- Usage Examples: Ideal for collections with frequent additions/removals, and dynamic data manipulation; beginners can create, add, and print ArrayLists easily.
- Techniques for Efficient Use: Includes pre-sizing with
ensureCapacity
, sorting usingCollections.sort()
, and using iterators for modifications. - Conversion Techniques: Convert a 2D array to an ArrayList by iterating rows and creating sub-ArrayLists, preserving data layout.
- Synchronization: Synchronized with
Collections.synchronizedList()
for thread safety to avoid issues likeConcurrentModificationException
in multi-threaded environments.
Learn faster with the 24 flashcards about Java Arraylist
Sign up for free to gain access to all our flashcards.
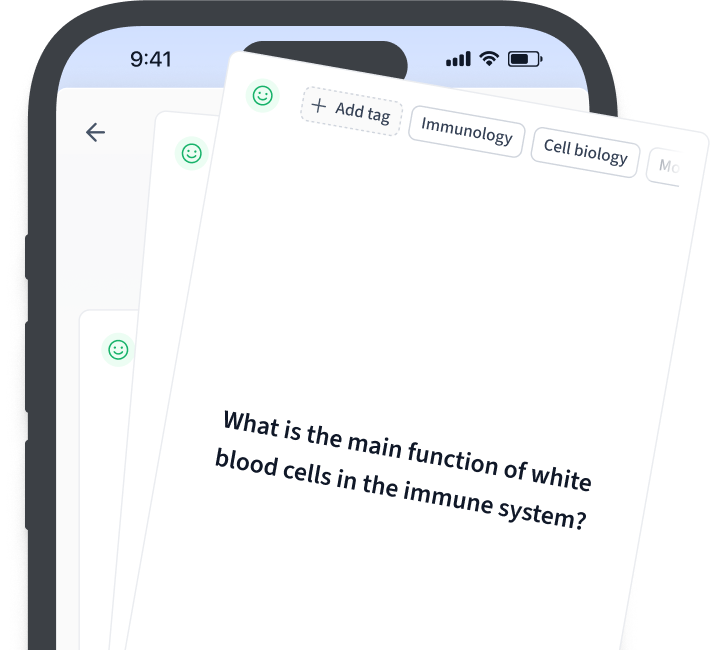
Frequently Asked Questions about Java Arraylist
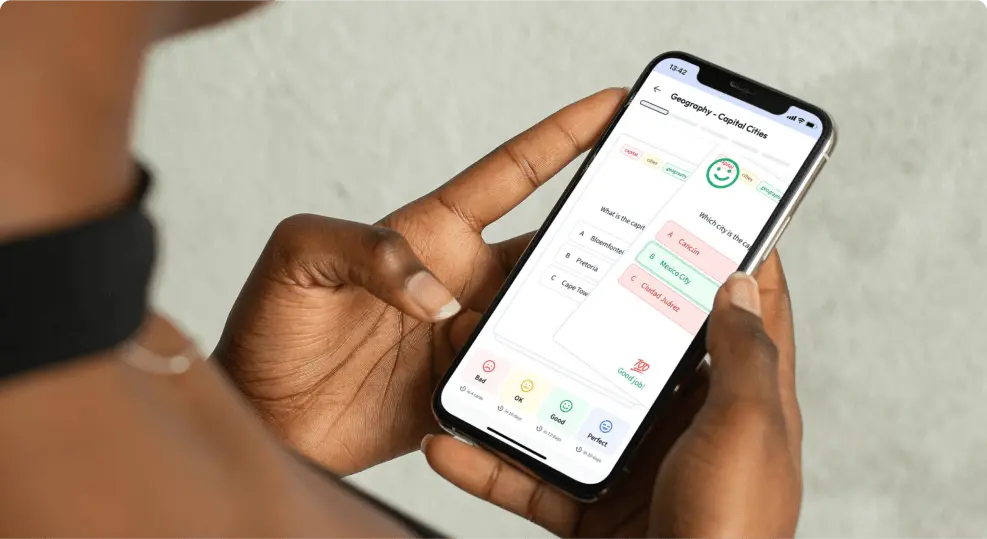
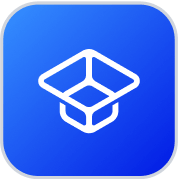
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more