Jump to a key chapter
Understanding the Java Collections Framework
The Java Collections Framework (JCF) is a significant aspect of Java programming language that's highly utilised in creating efficient, maintainable, and reliable Java applications.The Basics: What is Collection Framework in Java?
The Java Collections Framework is a suite of interfaces and classes in the Java programming language designed for storing and managing groups of objects. It comprises several pre-packaged data structures, such as ArrayList, LinkedList, HashSet, HashMap, TreeMap, and more.In Java, a Collection is an object that can hold references to other objects. The Collection interfaces declare the operations that can be performed on each type of collection. The classes provide concrete implementations to these interfaces.
Key Principles of Java Collections Framework
The Java Collections Framework operates on a few crucial principles:- Reusability: JCF provides reusable data structures, reducing the time and effort to develop custom data structures.
- Interoperability: Since collections are based on interfaces, they can be passed around and used interchangeably.
- Performance: The standard collections are high-performance implementations that can deal with large quantities of data.
Collection Framework in Java: Why it Matters
Java Collections Framework's functionality and flexibility matter as it addresses common programming needs seamlessly and effectively.For example, sorting a list, queuing jobs in a scheduler, or storing a map of objects are all standard tasks handled remarkably smoothly by the Java Collections Framework.
Map Collection Framework in Java Explained
The Map is an interface in the Java Collections Framework that stores data in key/value pairs, such that every key is unique. It provides get and put methods for storing and retrieving data.
- HashMap - LinkedHashMap - TreeMap
Understanding Map Interface in Collection Framework
Map Interface’s primary purpose is to maintain mappings of keys to values. The keys are unique, allowing each key to map to a single value. That offers a valuable method to store data when you want to link information in a key-value pair.Take a straightforward example: a map of students in a class, where each student (the key) is linked to their grades (the value). Using the Map Interface, you can easily set up this data structure and retrieve the grades for each student by their name.
Delving into the Hierarchy of Collection Framework in Java
Java Collections Framework features a well-organised hierarchy that makes it capable of handling various data structures. It consists of numerous interfaces and classes that contribute to creating a robust, flexible, and efficient data handling mechanism in the Java programming language.Structure and Components of the Hierarchy in Java Collections Framework
In the Java Collections Framework, the hierarchy begins with the Collection and Map interfaces. These interfaces are further divided into more specific types to handle different kinds of data. The Collection interface houses the Set, List, and Queue interfaces. The main structural difference is that while Map interface handles key/value pairs, Collection interface handles a group of individual objects. Below is a simplified breakdown of the Collection part of the hierarchy:Collection - Set - HashSet - LinkedHashSet - TreeSet - List - ArrayList - LinkedList - Queue - PriorityQueueThis outline shows just a portion of the Java Collections Framework. There are more implementations for each interface, and each of those can be used to handle data in various ways.
Role and Functionality of Interfaces in Java Collections Hierarchy
In the hierarchy of the Java Collections Framework, the interfaces play the vital role of defining the types of collections, namely Set, List, and Queue. Each of these interfaces has a set of methods that allows different kinds of operations on the collections. The Set interface is for collections that do not maintain a specific order and do not allow duplicate elements. The List interface supports collections that maintain their elements in a specific sequence. The Queue interface is for collections aimed at holding elements for processing.Digging Deeper: Data Structures and the Java Collections Framework
Each of the structures in the Java Collections Framework is designed to fulfil specific data handling needs. For instance, the ArrayList class is essentially a dynamic array offering random access to elements. On the other hand, the LinkedList class is a doubly linked list that provides better performance for add/remove operations compared to ArrayList. The various classes of Map provide similar versatility. HashMap provides an unordered, unsorted Map. In contrast, the TreeMap gives a sorted Map, and the LinkedHashMap maintains the insertion order. Each of these classes provides a suitable way to handle data according to specific programming needs, giving you the flexibility and efficiency to tackle a wide range of programming challenges. Remember, it's essential to choose the right data structure for your application, as it can significantly impact the performance and functionality of your program.Mastering Java Collections Framework with Practical Examples
The Java Collections Framework isn't just a vast and powerful resource for organising your data, it's a vital skill for any aspiring Java developer. Understanding how this complex library works and how to use it effectively can make a significant difference in your programming tasks. Let's make it practical by examining its application through examples and case studies.Collection Framework in Java with Example: Case Studies
Knowledge is best solidified by having hands-on experience. To fully comprehend the Java Collections Framework, it's vital to see it in action. Let's go through some case studies:Consider you have to manage a list of students. You'd use the List interface in this case because it provides an ordered collection and can include duplicate elements. Here's a simple way to do that with ArrayList:
ListIn the above code, you've created an ArrayList of students and added some names. Note that "John" is added twice, and the List interface positively accepts duplicates.students = new ArrayList<>(); students.add("John"); students.add("Sara"); students.add("Mike"); students.add("Anna"); students.add("John");
SetIn the above code, the "ID002" is added twice, but the size of the Set will be 3 because it doesn't allow duplicates.studentIds = new HashSet<>(); studentIds.add("ID001"); studentIds.add("ID002"); studentIds.add("ID002"); studentIds.add("ID003"); System.out.println(studentIds.size());
Practical Tips for Using Collection Framework Programs in Java
Here are some practical tips that would be helpful to effectively use Java Collections Framework:- Choose the right Collection type: Depending on whether you need duplicates, ordering, or key-value pairs, you can choose between Set, List, and Map.
- Consider performance requirements: Different collection classes have different performance characteristics. For example, ArrayList offers efficient random access, while LinkedList provides efficient insertion and removal.
- Use the Collections class for utility operations: The Collections class provides several utility functions like sorting and searching which can be quite handy.
Common Challenges and Solutions When Using Java Collections Framework
Despite Java Collections Framework's power and utility, a few common challenges might arise during its usage. We will address these challenges along with potential solutions:One common challenge is the infamous ConcurrentModificationException. This exception is thrown when a collection is modified while iterating over it. The best way to handle this is to use concurrent collection classes like CopyOnWriteArrayList or ConcurrentHashMap. Another common issue is dealing with null values. Some collection classes like HashSet, ArrayList, and HashMap allow null values. However, classes like TreeMap and Hashtable do not. It's crucial to understand these differences to avoid a NullPointerException.
Advanced Topics: Collection Framework Programs in Java
Once you're comfortable with the basics, you can start exploring more advanced topics in the Collections Framework like:- Sorting collections using the Comparable and Comparator interfaces
- Creating and using custom collections
- Understanding the performance characteristics of different collections
- Working with streams and lambda expressions with collections
Advanced Coding Practices with Java Collections Framework
At the advanced stage, you should focus on items like:- Generic Collections: Always use Generic Collections to ensure type-safety and avoid ClassCastException.
- Immutable Collections: Creating unmodifiable collections can help prevent unwanted side-effects in your code.
- Effective Use of Iterators: Proper usage of Iterators could improve the performance of your application.
- Concurrent Collections: In a multi-threaded environment, you'll need to use concurrent collections to prevent data inconsistency.
Java Collections Framework - Key takeaways
- The Java Collections Framework (JCF) is a significant part of the Java programming language, providing reusable, interoperable, and high-performing data structures such as ArrayList, LinkedList, HashSet, HashMap, TreeMap.
- The 'Collection' in Java is an object that holds references to other objects and allows operations to be performed on each type of collection. The classes provide concrete implementations to these interfaces.
- The Map Interface of the Java Collections Framework stores data in unique key/value pairs and provides methods for storing and retrieving data. It's used for maintaining mappings of keys to values.
- The hierarchy of the Java Collections Framework starts with the Collection and Map interfaces. The Collection interface is further divided into Set, List, and Queue interfaces, each having specific methods allowing different kinds of operations on the collections.
- Examples of Collection Framework programs in Java include handling lists with ArrayList where duplicates are allowed, or managing unique values using the Set interface with HashSet.
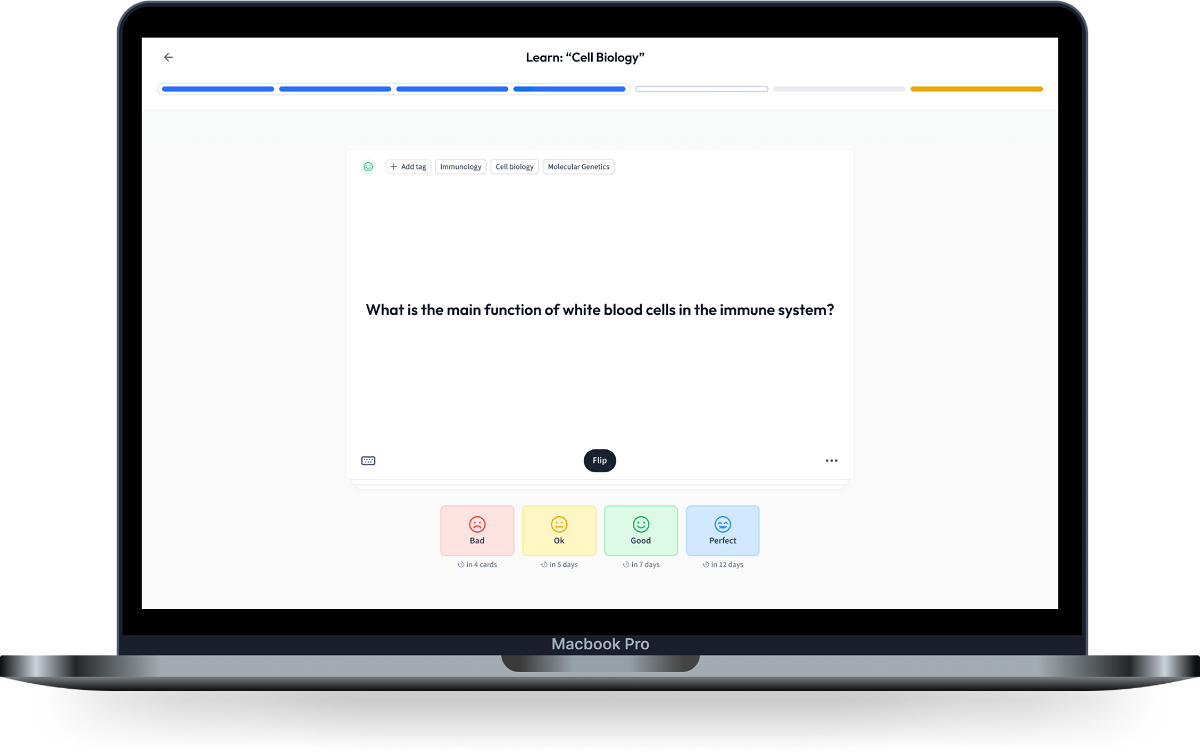
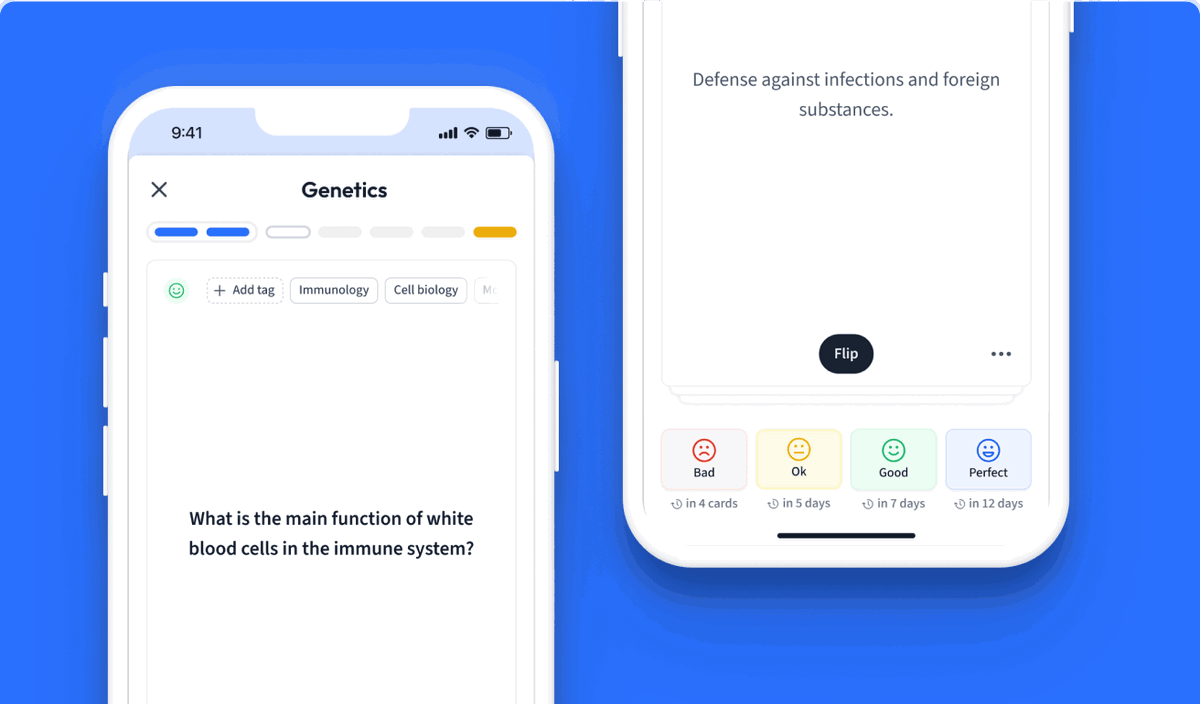
Learn with 12 Java Collections Framework flashcards in the free StudySmarter app
Already have an account? Log in
Frequently Asked Questions about Java Collections Framework
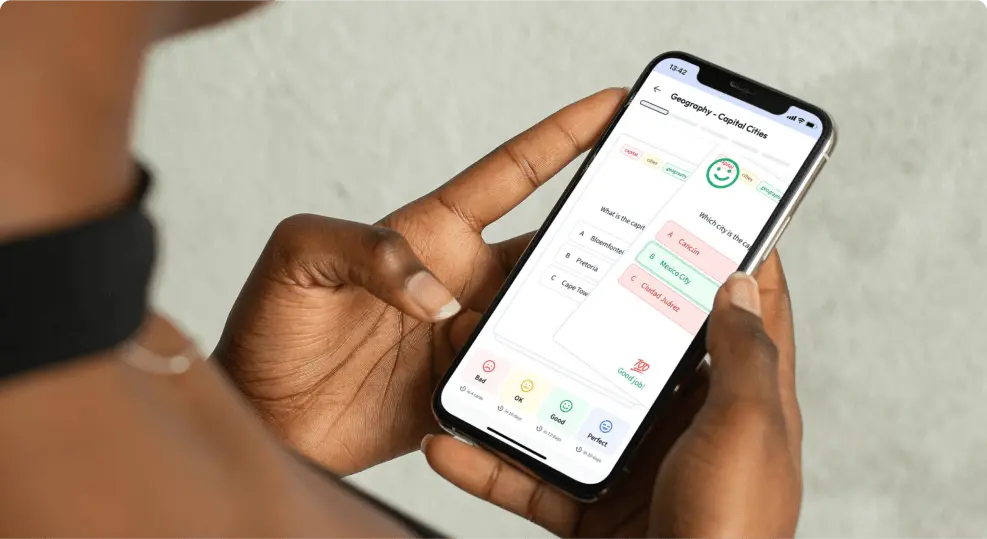
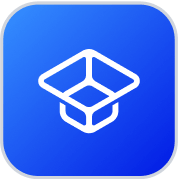
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more