Jump to a key chapter
Understanding the Java Enhanced For Loop
In the realm of computer programming, loop constructs are essential for repetitive tasks. However, as you delve deeper into programming languages, you'll discover that not all loops are created equal. Take for instance, the Java Enhanced For Loop.The Enhanced For Loop, also known as the 'For-Each' loop, offers an advanced way to traverse arrays or collections in Java, making it easy and efficient.
This special loop was introduced in Java 5 as a part of Java's language enhancements. While it's chiefly used with arrays and collections, it comes with some limitations. For example, it's impossible to modify the current sequence element or to obtain the current index while looping. Despite this, it's a super effective tool that boosts code readability and provides a concise and bug-resistant method for iterating through arrays or collection instances.
Definition of the Enhanced For Loop in Java
It's pivotal to understand how the Enhanced For loop in Java works for ease of use.The Enhanced For Loop is designed for iteration over arrays and collections, simplifying the structure of a standard loop by eliminating the need for a counter variable and stopping condition. It's capable of iteratively processing each element without the use of an index.
for (int num : arr) { System.out.println(num); }This loop will iterate through each element in 'arr', with each element temporarily stored in 'num', which is then printed to the console.
Breaking Down the Syntax of the Enhanced For Loop Java
To fully harness the power of the Enhanced For loop, it's necessary to delve deeper into its syntax.The syntax of the Enhanced For loop in Java follows this pattern:
for (type var : array) { //Statements using var }
Where 'type' is the data type of the array elements, 'var' is the variable that temporarily holds the array element for each iteration, 'array' is the array to be looped through, and 'Statements using var' are the operations to be performed on 'var'.
int[] arr = {1, 2, 3, 4, 5};To print each element to the console, the Enhanced For loop would look like this:
for (int num : arr) { System.out.println(num); }It's critical to remember that the variable 'num' only holds a copy of the array element. Any changes to 'num' within the loop do not affect the actual element in the array.
Analysing Enhanced For Loop Java Examples
In the quest to master the Enhanced For Loop in Java, analysing practical examples is highly beneficial. Not only does it bolster understanding, but it provides a clear picture of how to apply this tool within your own programs.Practical Example of Using Enhanced For Loop in Java
Consider a simple, practical example of using the Enhanced For loop in Java. Suppose, you have an array of marks obtained by students and you want to display them.int[] marks = {85, 91, 78, 88, 94}; for (int mark : marks) { System.out.println(mark); }In this example, the loop iteratively retrieves each mark from the 'marks' array and prints it to the console. In a more complex scenario, you might want to calculate the average mark in the 'marks' array:
int[] marks = {85, 91, 78, 88, 94}; int sum = 0; for (int mark : marks) { sum += mark; } double average = sum / (double) marks.length; System.out.println("Average mark: " + average);The Enhanced For loop in this scenario iterates through each mark, adding it to the variable 'sum'. After all marks have been added to 'sum', the average mark is calculated by dividing 'sum' by the total number of marks (which is given by 'marks.length'). The average mark is then printed to the console.
Using Enhanced For Loop Java with an ArrayList
The Enhanced For loop is not only useful for arrays, but is equally powerful when used with collections, such as ArrayLists. Consider the following example with an ArrayList of names:ArrayListIn this example, the Enhanced For loop iterates through each element in the 'names' ArrayList, temporarily storing each element in the 'name' variable. Each 'name' is then printed to the console. Understand that an ArrayList allows you to dynamically add, remove and retrieve elements - something which arrays cannot do in Java. In a slightly more complex example, you may want to remove all names that start with 'J' from the ArrayList:names = new ArrayList<>(); names.add("John"); names.add("Jane"); names.add("Jill"); names.add("Jack"); for (String name : names) { System.out.println(name); }
ArrayListNote that the Enhanced For loop isn't used in this case, because it's not possible to remove elements from a collection while looping over it with this tool. Instead, an iterator is used. The iterator loops through each element in 'names', and any that start with 'J' are removed.names = new ArrayList<>(); names.add("John"); names.add("Jane"); names.add("Jill"); names.add("Jack"); Iterator iterator = names.iterator(); while (iterator.hasNext()) { if (iterator.next().startsWith("J")) { iterator.remove(); } }
Discovering the Technique of Enhanced For Loop Java
Unveiling the technique of Enhanced For Loop reveals its core purpose in Java. Prized for its simplicity and efficiency, the Enhanced For Loop, or 'for-each' loop, is quintessential for manipulating arrays and collections. For beginners and seasoned programmers alike, mastering the Enhanced For Loop is certain to transform your programming proficiency.Advantages of Using the Enhanced For Loop Structure
Without a doubt, the Enhanced For Loop structure has multiple advantages which make it a prominent feature in Java.Primarily, the Enhanced For loop drastically simplifies syntax when iterating over arrays and collections, consequently resulting in code that is easier to read and maintain.
int[] nums = {1, 2, 3, 4, 5}; for (int i = 0; i < nums.length; i++) { System.out.println(nums[i]); }Enhanced For Loop:
int[] nums = {1, 2, 3, 4, 5}; for (int num : nums) { System.out.println(num); }The difference is apparent. The Enhanced For Loop eliminates the need for an iterator and checks for array length. Moreover, it also reduces the risk of errors related to incorrect indices or off-by-one errors.
Secondly, the Enhanced For loop is remarkably beneficial when it comes to accessing collections. The collections framework in Java doesn't support indexing, so you cannot access elements using an integer index without calling the get() method. With the Enhanced For Loop, you can directly access each element.
ArrayListHere, each name is accessed directly from the ArrayList and printed to the console. Without the Enhanced For Loop, you would have to use an iterator or call the get() method which would result in more verbose code.names = new ArrayList<>(); names.add("John"); names.add("Jane"); for (String name : names) { System.out.println(name); }
Streamlining Code with the Enhanced For Loop Java Syntax
The syntax of the Enhanced For Loop is designed for compactness and simplicity. Its unique structure makes it possible to streamline your code, making it easier to read and maintain.for (type var : array) { //Statements using var }In this syntax:
- 'type' is the data type of the items in the array or collection.
- 'var' is a variable that will hold one item from the array or collection on each iteration.
- 'array' is the array or collection to loop through.
- The statements inside the loop can then manipulate or display the contents of 'var'.
Difference between For Loop and Enhanced For Loop in Java
Java stands out particularly due to its robust, versatile looping mechanisms, namely the standard For Loop and the Enhanced For Loop. Delving into the core differences between these two loops is not only fascinating but also essential when establishing efficient, effective programming habits.Standard For Loop in Contrast with Enhanced For Loop in Java
The notable disparity between these two loop types lies in their syntax and usage. The standard For Loop, often deemed as the 'counter loop', operates based on a counter (an integer index). This is used to access the array elements in a sequential manner by incrementing or decrementing this counter. Here's a simple demonstration of the 'For Loop' to print the elements of an array:int[] nums = {1, 2, 3, 4, 5}; for(int i = 0; i < nums.length; i++){ System.out.println(nums[i]); }In this example, 'i' is an index counter initialised to 0. For every iteration, 'i' is incremented until it reaches the length of the array 'nums'. Each element of 'nums' is accessed sequentially using the index 'i' and printed to the console. On the other hand, the Enhanced For Loop, also known as the 'For-Each' loop, operates slightly differently. Rather than providing an index to access the elements, the Enhanced For Loop directly presents each element of the array or collection in the loop. Let's consider the same task of printing array elements with the Enhanced For Loop:
int[] nums = {1, 2, 3, 4, 5}; for(int num : nums){ System.out.println(num); }In this case, 'num' represents each element in the 'nums' array during each iteration. The 'num' variable holds a copy of the array element for each iteration and prints this to the console. The inherent disparity is apparent - the certainly more streamlined and expressive Enhanced For Loop doesn't require an index counter nor explicit length checks.
When to Use Standard For Loop versus Enhanced For Loop Java
The decision between employing the standard For Loop versus the Enhanced For Loop in Java often hinges on the precise nature and complexity of your task. For tasks demanding the exact index of elements while iterating over the array or when you need to modify the array elements, the Standard For Loop would be advantageous. Additionally, the conventionally structured For Loop allows for more flexibility. The increment operations can be adjusted, elements can be skipped, or the loop can be moved backwards, to name a few scenarios. Conversely, the Enhanced For Loop shines brightly when the task solely requires accessing and using array or collection elements, without any requirements for element indexes or adjustments to the traversal order. Its clean syntax, devoid of explicit counter variables and boundary conditions, makes the code more readable and decreases the potential for errors. However, note that while the Enhanced For Loop can be used with both arrays and collections, it lacks the capability to make alterations to the array or collection elements. Trying to modify elements merely changes the copy in the variable used for iteration, leaving the source data unaffected. In summary, the essence would be, if your task necessitates unconventional loop traversals, alterations to the data or access to the index of elements, then opting for a standard For Loop might be optimal. On the other hand, if conciseness, readability, and simplicity take precedence, generally when performing read-only operations on arrays or collections, the Enhanced For Loop is your champion. Understanding when to utilise each of these powerful tools effectively will equip you with the ability to write optimised, error-resistant, and understandable code in Java.Working with Arrays Using the Enhanced For Loop in Java
Learning to manipulate arrays using the Enhanced For Loop opens the door to writing cleaner, more efficient Java code. This valuable tool can make your tasks, from handling data sets to solving complex programming problems, notably simpler and more efficient.Simplifying Array Manipulation with Enhanced For Loop Java
Regarding arrays, the power of the Enhanced For Loop, or 'for-each', is evident. Its practicality surfaces when we need to traverse or 'iterate' over an array, accessing each element in turn. In scenarios where you don't need to modify the array elements, the Enhanced For Loop exhibits its sterling potential. Since it allows you to focus on what you want to do with each array element rather than fuss over the specifics of the iteration process, it keeps your code concise and readable. A typical array iteration syntax with the Enhanced For Loop looks something like this:for (int num : numsArray) { ... }Here, 'int' signifies the type of the array. 'num' is a placeholder value that represents each array element in each iteration, and 'numsArray' is the array you're iterating over. Think of it as the loop saying: "For each number 'num' in 'numsArray', do the following...". It doesn't get much more readable than that! Remember, though, that the Enhanced For Loop operates with a copy of each array element. You can use 'num' within the loop to work with the array values, but any changes made to 'num' itself won’t affect the original array.
Practical Example of Enhanced For Loop Array Java
Consider this real-world application: you have an integer array representing the score of each student in an exam and you want to calculate the average. Here's how we can approach this task using the Enhanced For Loop:int[] scores = {85, 90, 88, 92, 76}; int sum = 0; for (int score : scores) { sum += score; } double average = (double) sum / scores.length; System.out.println("The average score is: " + average);In this snippet, we are iterating over the scores array using the Enhanced For Loop. 'score' represents each score in the array during each loop iteration. We add the value of 'score' to 'sum' during each pass of the loop. After the loop ends, we have the total sum of all scores, which we then divide by the length of the scores array, yielding the average score. This is a basic, yet powerful example of how the Enhanced For Loop can simplify tasks. The code written is readable and easy to understand, demonstrating the advantages of using the Enhanced For Loop when working with arrays in Java. Bearing this in mind, it should be noted that while the Enhanced For Loop is a mighty tool, careful thought needs to be applied before using it. If your tasks require altering the original array, or you're looking to use the index of the elements, then an ordinary For Loop might be a better choice. However, for simplified iteration through arrays, the Enhanced For Loop stands peerless.
Java Enhanced For Loop - Key takeaways
- The Enhanced For Loop in Java is designed for iteration over arrays and collections, eliminating the need for a counter variable and stopping condition.
- The syntax of the Enhanced For loop follows the pattern: "for (type var : array) //Statements using var", where 'type' is the data type, 'var' is the variable that holds the array element and 'array' is to be looped through.
- The variable 'var' in the for loop only holds a copy of the array element, thus, any changes to 'var' within the loop does not affect the actual element in the array.
- When using the Enhanced For Loop with ArrayLists in Java, it is not possible to remove elements from the array list while looping over it with the "for-each" loop, an alternative to use is Iterator.
- The Enhanced For Loop (also known as 'for-each' loop) is pivotal for manipulating arrays and collections in Java, providing simplified syntax, improved readability and compactness.
- Difference between For Loop and Enhanced For Loop: the standard For Loop uses a counter to access the array elements in a sequential manner, whereas the Enhanced For Loop directly presents each element of the array or collection in the loop.
- The choice between standard For Loop and Enhanced For Loop hinges on the task requirements. For tasks that require the index of elements or modifying the array elements, standard For Loop is more appropriate. For tasks that require accessing and using array or collection elements with clean syntax and no requirements for adjustment to the traversal order, Enhanced For Loop is more suitable.
- The Enhanced For Loop can be beneficial when applying read-only operations on arrays or collections, making the code more readable and decreasing potential for errors.
- Arrays in Java can be manipulated using Enhanced For Loop for cleaner and more efficient code for handling data sets or solving complex programming problems.
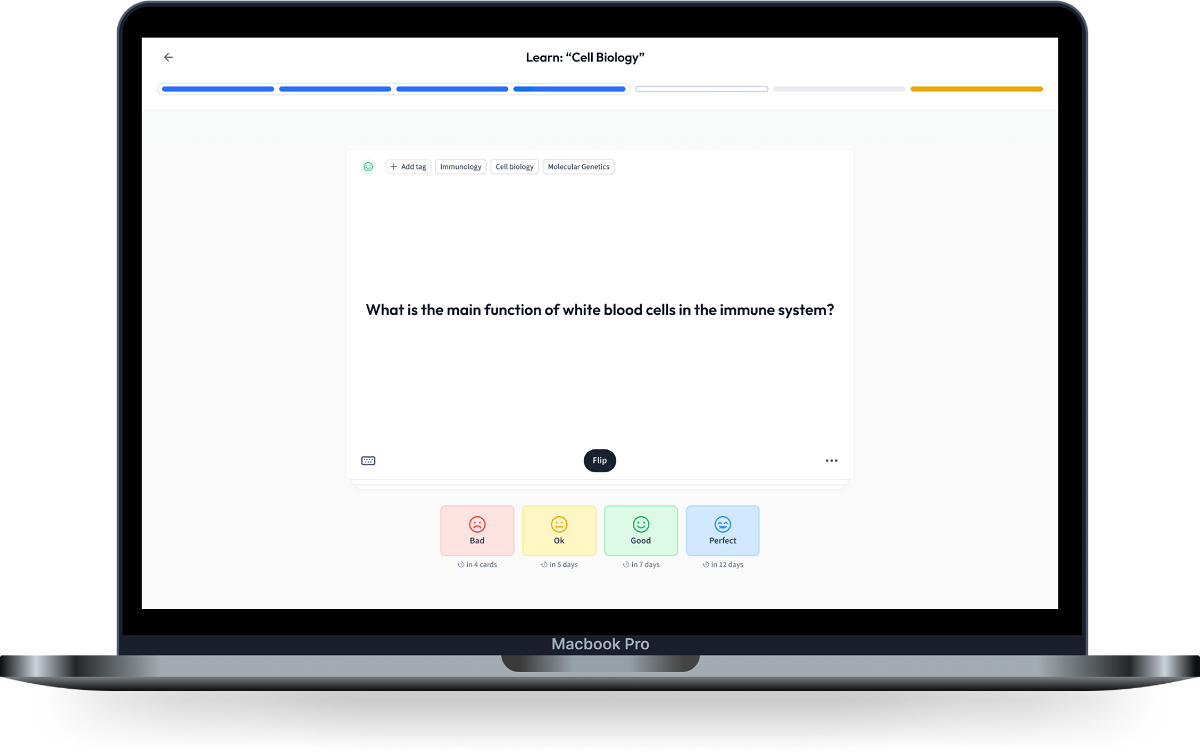
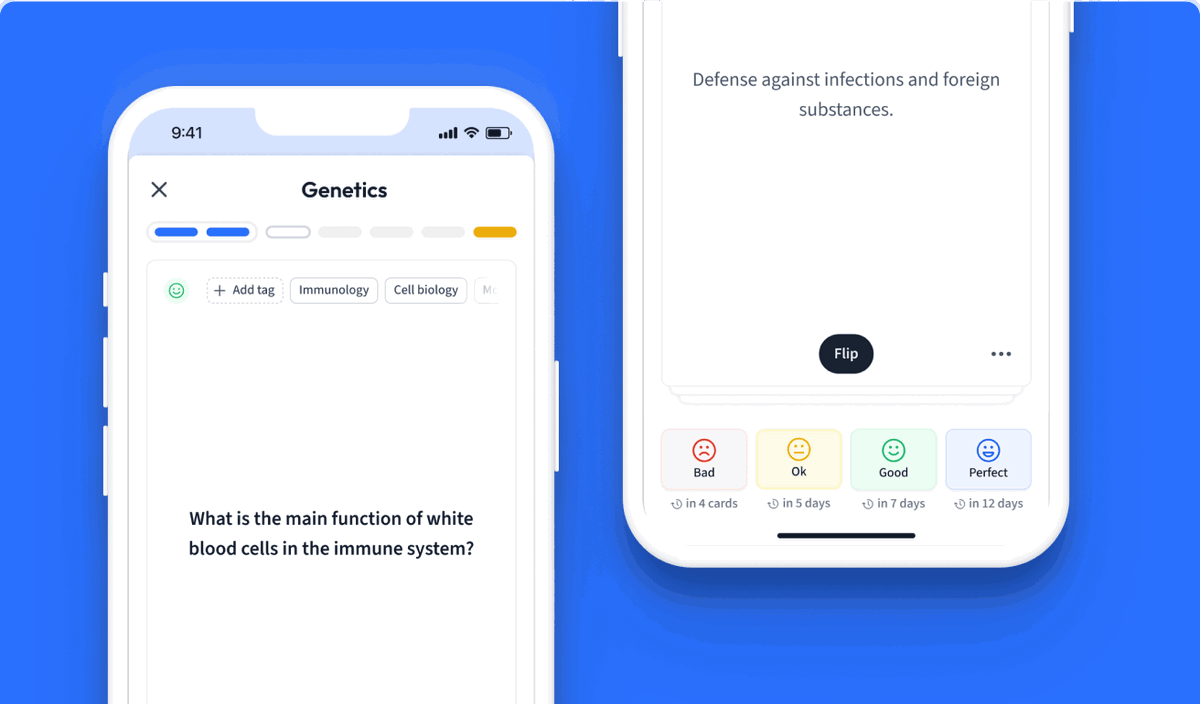
Learn with 15 Java Enhanced For Loop flashcards in the free StudySmarter app
Already have an account? Log in
Frequently Asked Questions about Java Enhanced For Loop
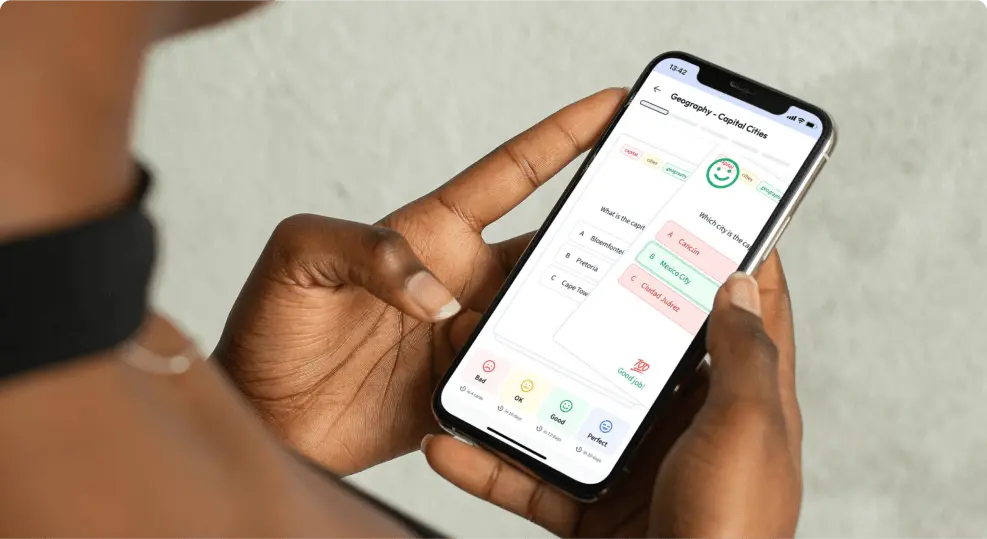
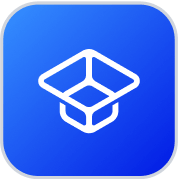
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more