Jump to a key chapter
Understanding Java File Handling: An Overview
Java file handling is a collection of procedures that a Java programmer utilises to create, read, update, and delete files.
Introducing Java File Handling Concepts
Java file handling can be broken down into various concepts – each important in its own way:- Streams
- Classes
- Methods
Streams in Java are a sequence of data. There are two main types of streams in Java:
- Byte Stream: handles binary I/O of 8-bit bytes
- Character Stream: handles I/O of characters
Why Java File Handling is Essential in Computer Programming
Java File Handling is a core aspect of programming for several key reasons. Here are a few:- It allows data to be stored persistently and retrieved conveniently.
- It facilitates the recording of log data for analysis.
- It enables handling of binary data like images and audio files.
Basic Principles of Java File Handling
It's essential to understand the following underlying principles of Java File Handling:- Stream Management
- Error Handling
- File Pointer Management
Stream management includes opening a stream to a file, performing I/O operations and closing the stream effectively.
Breaking Down Java File Handling Theory for Beginners
Let's break down the theory of Java File Handling:Opening a File: | In Java, the FileOutputStreamor FileInputStreamclasses are used to open a file for writing or reading, respectively. |
Reading/Writing: | The read()and write()methods from the FileInputStreamand FileOutputStreamclasses perform reading and writing operations, respectively. |
Closing the File: | To prevent memory leaks, the close()method of the FileInputStream or FileOutputStream class is used to close the file after performing all the operations. |
```java import java.io.FileOutputStream; public class Main { public static void main(String[] args) { String data = "This is a line of text inside the file."; try { FileOutputStream output = new FileOutputStream("file.txt"); byte[] array = data.getBytes(); output.write(array); output.close(); } catch(Exception e) { e.getStackTrace(); } } } ```
"This is a line of text inside the file."to a file called "file.txt". When running this program, if the "file.txt" file does not exist on disk, the JVM will create it. If it already exists, the JVM will overwrite it.
Exploring Key Java File Handling Techniques
Java File Handling techniques encompass various dynamic methods that can be used to manipulate files in a Java program.Different Java File Handling Methods: A walkthrough
In Java File Handling, prolific methods exist that are utilised to read and write data to files, create files and directories, delete files and directories, and more. Understanding these multiple methods is integral to your use of Java File Handling. For instance, theread()and
write()methods are two of the basic methods you will frequently use. The
read()method is used to read data from a file, and the
write()method is used to write data into a file.
The read() method returns an integer representing the next byte of data, or -1 if the end of the file is reached.
available(), which is used to find the number of bytes available in the input stream. This method returns an estimate and can be handy when you do not know the size of the file you are reading in advance.
The available() method returns an integer which represents the number of bytes available in the input stream.
catchand
finallyblocks are predominant. The
catchblock is used to catch exceptions that occur in the try block, and the
finallyblock is designed to execute important code such as closing a file, whether an exception has occurred or not.
Proven Java File Handling Techniques Enriching Computer Science
Effective Java File Handling techniques have significantly enriched computer science by providing efficiency and dynamism in data management. Java's built-in classes likeFileReader,
BufferedReader,
FileWriter, and
PrintWriterare commonly used in Java File Handling for more efficient and higher-level file operations.
The BufferedReader and BufferedWriter classes, for instance, use a buffer to reduce the number of read and write operations by accumulating bytes/characters into a buffer before actually reading or writing them. This technique greatly enhances the performance of I/O operations.
Step by Step Java File Handling Examples
Let's elaborate a step-by-step example demonstrating the use of a few Java File Handling methods.```java import java.io.*; public class Main { public static void main(String[] args) throws IOException { // Create a new file File file = new File("test.txt"); file.createNewFile(); // Write data into the file FileWriter writer = new FileWriter("test.txt"); writer.write("Hello, world!"); writer.close(); // Read data from the file FileReader reader = new FileReader("test.txt"); int character; while ((character = reader.read()) != -1) { System.out.print((char) character); } reader.close(); } } ```
createNewFile()method from the
Fileclass is used to create a new file called 'test.txt'. The
FileWriterclass and its
write()method are used to write data into the file, and the
FileReaderand
read()methods are used to read data from the file. The output of this program will be
Hello, world!.
Comprehensive Analysis of a Java File Handling Example
Let's analyse, in-depth, the Java File Handling example given above. Theimport java.io.*;at the top of the file means that we are importing all classes and interfaces from the java.io package into our program. The java.io package contains all the classes and interfaces related to input/output operations. The
createNewFile()method returns
trueif it successfully creates the new file and
falseif the file already exists. It throws an
IOExceptionif an I/O error occurs. The
write()method of
FileWriterwrites the string passed to it into the file specified in its constructor. After performing all the operations on a file, it is very important to close it to free up the resources held by the file. Hence the
close()method is used at the end to close the
FileWriterobject. The
read()method of
FileReaderreads a single character from the connected file and returns it as an integer. If it has reached the end of the file, it returns -1. The data read from the file can then be converted to the char type and printed on the console. Moreover, special attention is needed on the use of
throws IOExceptionin the main method. This is used to signify that the main method may throw an IOException while executing the code. These Java File Handling techniques, methods and examples enrich the study of computer science, providing a robust mechanism for file management in programming.
Dealing with Exceptions: Handle File Not Found Exception in Java
Java programming entails dealing with various types of exceptions, and one common exception you will encounter is the File Not Found Exception. This exception occurs when an attempt is made to access a file that does not exist.How to Handle File Not Found Exception in Java: an in-depth guide
In Java, exceptions are issues that arise during the execution of a program. When an erroneous situation occurs within a method, that method creates an object and hands it off to the runtime system. This object, called an exception object, contains information about the error, including its type and the state of the program when the error occurred. The process of creating an exception object and handing it to the runtime system is called throwing an exception. Java's exception handling framework is robust and mostly based on the use of try, catch, and finally blocks. TheFileInputStreamand
FileOutputStreamclasses in Java are used for reading from and writing to a file, respectively. However, it's vital to remember that these classes can throw a FileNotFoundException if the file does not exist or cannot be opened for some reason. Let's discuss how you handle a FileNotFoundException when using these classes. Initially, you need to include the file operations inside a
tryblock. The
tryblock encloses a set of statements where an exception can occur. If any statement within the
tryblock throws an exception, that exception is handled by an exception handler associated with it. Afterwards, the
tryblock is followed by a
catchblock, which includes the method to handle the situation after the exception is thrown. The
catchblock can receive the exception thrown in the
tryblock. Should the
FileInputStreamand
FileOutputStreamthrow a FileNotFoundException, you can catch and handle it accordingly in your
catchblock.
Practical Examples to Handle File Not Found Exception in Java
To better understand how to handle a FileNotFoundException in Java, let's consider this simple code example:```java import java.io.File; import java.io.FileInputStream; import java.io.IOException; public class Main { public static void main(String[] args) { File file = new File("NonExistentFile.txt"); try { FileInputStream fis = new FileInputStream(file); } catch (IOException e) { System.out.println("An error occurred accessing the file."); e.printStackTrace(); } } } ```
FileInputStreamconstructor. The code then catches this exception in the
catchblock. The
catchblock here catches exceptions of type
IOException, which covers FileNotFoundException as it is a subclass of
IOException. If the exception is thrown (as expected), a message "An error occurred accessing the file." is printed on the console, followed by the stack trace of the exception. Remember, it is important to provide user-friendly error messages when catching exceptions. The users should be able to easily understand that an error has occurred, and the provided message should help them understand what went wrong. Also, you should limit exception handling to areas of your code where they are actually needed. Unnecessary exception handling could decrease the performance of your code and may even make it more difficult to debug. Real-world applications often require you to work with files. Reading and writing to files are common operations, and during these operations you are likely to encounter a FileNotFoundException in case the file does not exist. Therefore, understanding how to handle such exceptions is crucial in Java programming for performing smooth file operations. Thus, restricting the files to those that exist or handling exceptions effectively when they don't, provides a much more seamless user experience.
Broadening Your Skill Set: Advanced Java File Handling Principles
As part of your computer science journey, it's essential to explore advanced Java File Handling Principles. With Java emerging as one of the most popular programming languages in the field of technology, gaining proficiency in advanced file handling helps solve complex problems in efficient ways. Let's delve deeper into learning advanced aspects of Java File Handling, such as manipulating file attributes, working with random access files, and dealing with object stream files.Understanding and Applying Advanced Java File Handling Techniques
File handling in Java is truly empowering, providing greater control and functionality. Incorporating advanced techniques opens a vast array of possibilities to favourably manipulate files. These skills lead to more efficient and performance-enhanced programs. Java provides thejava.nio.filepackage, which allows the modification of detailed file attributes. These attributes range from simple properties, such as file size and creation time, to more specialised attributes like file ownership and permissions. Accessing and altering these attributes have been made hassle-free with classes like
Filesand
Paths.
The Files class provides static methods for reading, writing, and manipulating files and directories, while the Paths class provides static methods for constructing Path objects from one or more string representations.
A Path object represents a path in the file system and may include the root, directory, filename, and the extension.
The RandomAccessFile class in Java allows reading from and writing to any part of a file. It operates by using a pointer moving around the file, defined by the method seek() which moves the pointer to a desired location.
The ObjectOutputStream class allows writing primitive data types and objects to an OutputStream. Similarly, ObjectInputStream allows reading primitive data and objects from an InputStream.
Advanced Java File Handling Examples
Let's better understand these advanced Java File Handling techniques with some practical examples.For instance, if you were creating a large-scale data processing application and wanted to record progress by writing current processing stats to a file, the RandomAccessFile class would be immensely helpful. Instead of appending stats to the file or overwriting the entire file every time you wanted to record progress, you could merely update the specific line in the file that houses the stats you want to change.
// Creating instance of RandomAccessFile RandomAccessFile file = new RandomAccessFile("samplefile.txt", "rw"); // Writing to a random position file.seek(100); file.writeBytes("Hello, world!"); // Reading from a specific position file.seek(100); byte[] bytes = new byte[12]; file.read(bytes); String output = new String(bytes);Equally riveting is the use of Object Streams in Java. Let's ponder on an illustrative example:
// Writing objects to a file using ObjectOutputStream ObjectOutputStream outputStream = new ObjectOutputStream(new FileOutputStream("objects.dat")); outputStream.writeObject(new YourSerializableClass()); outputStream.close(); // Reading objects from a file using ObjectInputStream ObjectInputStream inputStream = new ObjectInputStream(new FileInputStream("objects.dat")); YourSerializableClass object = (YourSerializableClass) inputStream.readObject(); inputStream.close();
Recap: Key Concepts and Techniques in Java File Handling
To best comprehend Java File Handling and its accompanying advanced techniques, it's necessary to revisit key concepts and foundational principles. These techniques ensure high flexibility, accuracy, and overall better performance in handling reading and writing operations in Java.Comprehensive Summary: Java File Handling Theory and Practice
Java File Handling revolves around the creation, opening, reading, writing, and closing of files. There are several classes and methods that Java provides to handle these files and directories effectively. The fundamentals begin with the crafting of File Objects: The File class represents file and directory pathname. The FileReader and FileWriter classes are used for reading from and writing to character files. Similarly, BufferedReader and PrintWriter embed buffering to the reading and writing operations, enhancing performance.// Creating a new file File myFile = new File("myfile.txt"); // Writing to a file FileWriter fw = new FileWriter(myFile); PrintWriter pw = new PrintWriter(fw); pw.println("Hello, world!"); pw.close(); // Reading from a file FileReader fr = new FileReader(myFile); BufferedReader br = new BufferedReader(fr); String line = br.readLine(); br.close();
- DataInputStream and DataOutputStream allow reading and writing of primitive data.
- RandomAccessFile enables reading and writing to any part of a file.
- Manipulating file metadata
- Creating a directory or a directory hierarchy
- Iterating over directory listing
- Symbolic file link handling
- Watch services: Enables a program to watch a directory for changes (create, delete, and modify events)
Key Takeaways: Java File Handling Principles for Advanced Learning
Java File Handling offers robust methods and classes to read from and write to files, providing superior flexibility and control. Here are the key takeaways when it comes to grasping these principles:- The File class is essential for creating, renaming or deleting a file or directory, checking if a file or directory exists, etc.
- Buffered classes provide an added layer of efficiency by buffering the reading and writing operations.
- The RandomAccessFile class is a powerful tool that allows random read and write operations. It is highly useful when you have to perform operations on a large file and don't want to read or write the entire file.
- The java.nio.file package and its associated classes brings advanced file handling capabilities to perform a variety of operations with increased efficiency. Path manipulation and various filesystem related tasks are taken care of using these classes.
- Finally, understanding Object Streams allows you to write objects directly to files and read objects back, which can greatly enhance readability and maintenance of your code.
Java File Handling - Key takeaways
- The method
available()
returns the number of bytes available in the input stream, useful when the file size is unknown. - Java file handling often involves the
catch
andfinally
blocks for error handling. - Classes like
FileReader
,BufferedReader
,FileWriter
, andPrintWriter
are commonly used for efficient and high-level file operations in Java file handling. - The
BufferedReader
andBufferedWriter
classes improve the performance of I/O operations by using a buffer to reduce the number of read and write operations. - Java File Handling involves handling exceptions like the File Not Found Exception when a non-existing file is accessed.
- The
try
,catch
, andfinally
blocks are used in Java's exception handling framework. - The
FileInputStream
andFileOutputStream
classes can throw a FileNotFoundException if the file does not exist or cannot be opened for some reason. - Advanced Java File Handling techniques include manipulation of file attributes, working with random access files, and dealing with object stream files.
- The
java.nio.file
package allows modification of detailed file attributes with classes likeFiles
andPaths
. - The
RandomAccessFile
class allows reading from and writing to any part of a file. ObjectOutputStream
andObjectInputStream
classes allow direct writing and reading of objects to and from a disk.
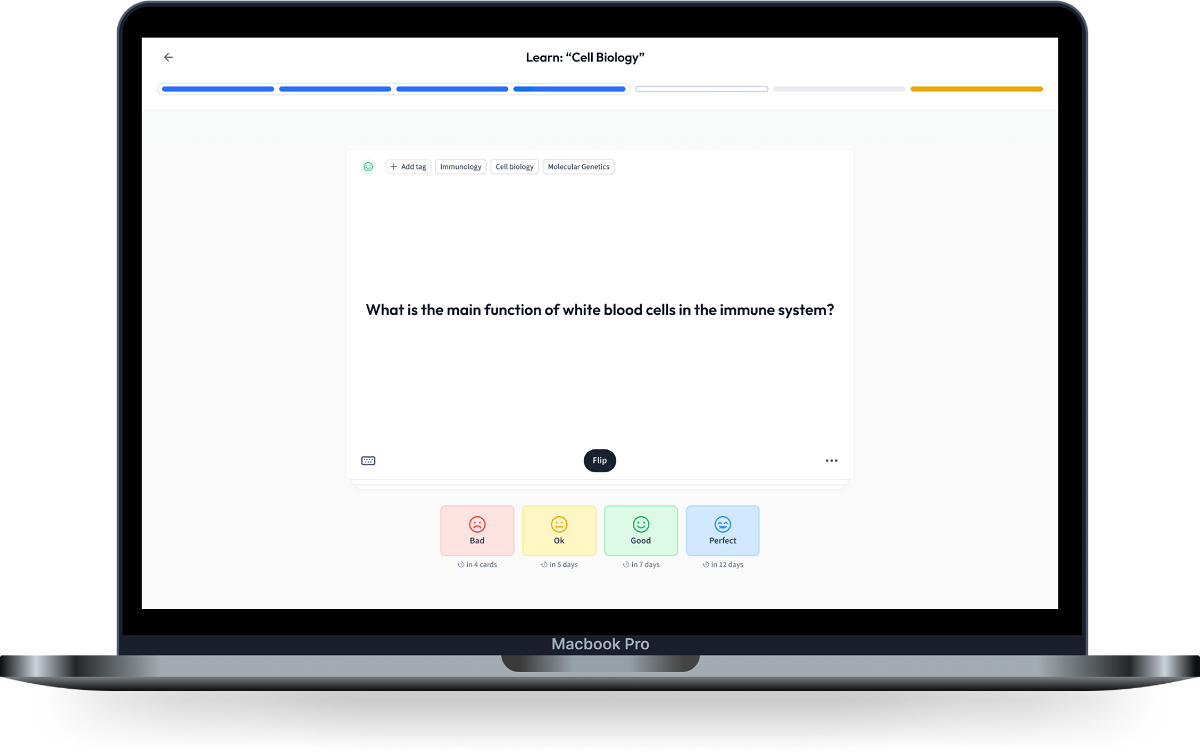
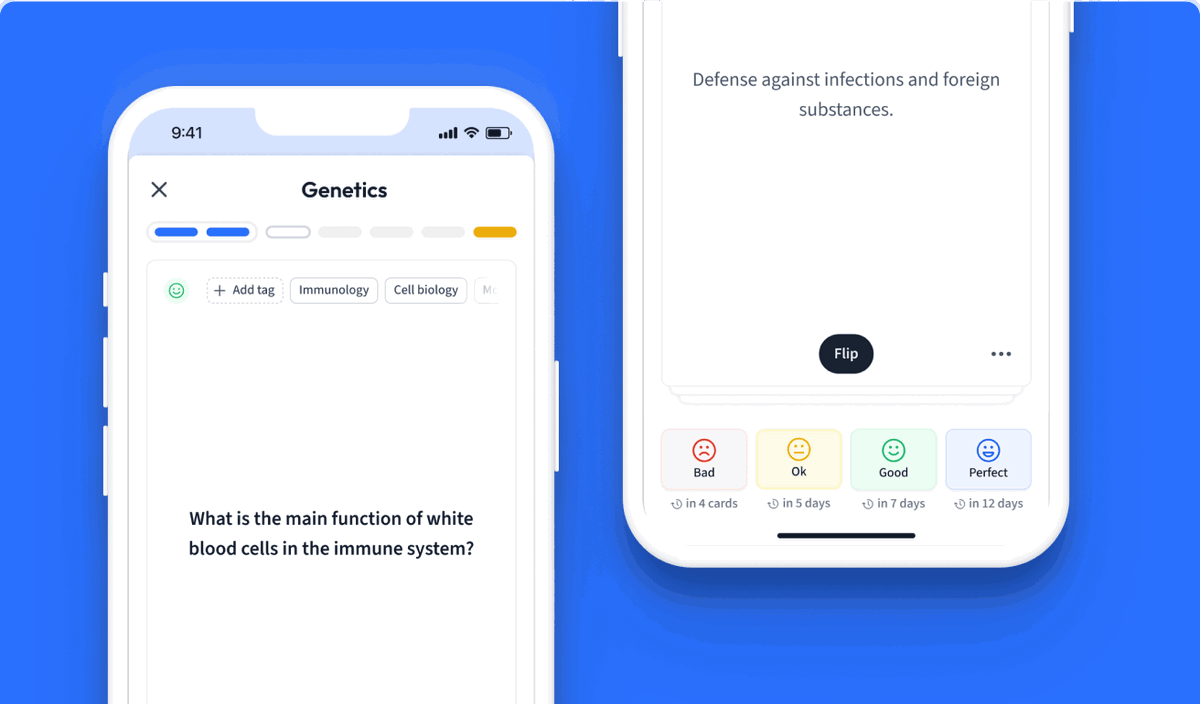
Learn with 15 Java File Handling flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Java File Handling
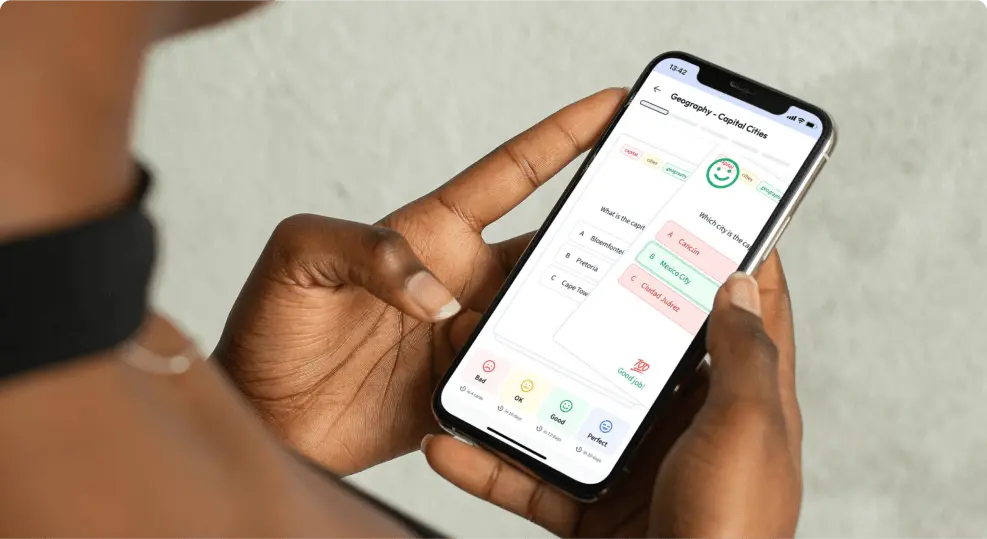
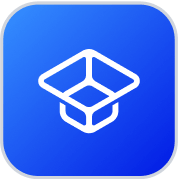
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more