Jump to a key chapter
Understanding Java Generics
Java Generics is a key concept in the world of Java programming that, when understood thoroughly, can effectively boost your productivity and ensure your code is robust.Java Generics is a feature that allows programmers to write code uses types (classes and interfaces) as parameters. Type checking is done at compile time to strengthen type checks, enhancing runtime type safety.
Java, what is Generics?
In simple terms, Generics in Java are methods or classes that are able to operate on objects of various types while providing compile-time type safety. This ensures that the type safety check occurs at compile time, highlighting any deviations and promoting effecient coding.For example, consider a scenario where you need to create a list that can store different data types. You could use an ArrayList and add elements of different types like Integer, String, etc. But this is risky as you can face a ClassCastException at runtime. Java Generics helps prevents such errors.
public class Namewhere T1 to Tn are type parameters{ /* ... */ }
The importance of Java Generics
The fundamental role of Java Generics is to offer benefits like:- Stronger type checks at compile time
- Type safety
- Elimination of casts
- Enable and ensure correct use of algorithms
Types serve as vital tools for programmers - they allow to express what a function or data can do. This is critical for debugging because types can help illuminate what operations are acceptable on data.
Getting started with Java Generics
For beginners attempting to get started with Java Generics, it's necessary to grasp key terminologies like:- Parameterized Types
- Type Parameters
- Generic Methods
- Bounded Type Parameters
- Wildcards
Term | Description |
Parameterized Types | It is a type that is parameterized over another type or types |
Type Parameters | These are the actual type that is passed to the type variable T |
Generic Methods | These are special methods that are declared with a type parameter |
Bounded Type Parameters | These restrict what types can be passed to a type parameter |
Wildcards | These are used to specify that any type is valid in a location |
Working with Java Generic Method
A crucial part of Java Generics is the use of Generic Methods. These involve methods that introduce their own type parameters. This is somewhat similar to declaring a generic type but the difference lies in its scope. Research indicates that Java Generic Methods are effective in increasing flexibility in code structure.Tutorial on Java Generic Method
Java Generic Methods can be distinguished by a type parameter section denoted by angle brackets (< >) which precedes the method's return type (\( \text{public class Utility { //Java Generic Method public static < T > void genericDisplay (T element){ System.out.println(element); } }
Here, < T > denotes that it can refer to any type. The type is passed as a parameter to the method genericDisplay and whatever parameter we pass to this method, it will become of that particular type.
Java Generic Method Sample Code
Here's a commonly used piece of Generic Method code which would help you understand better:public class Utility { public static < T > void genericDisplay(T element){ System.out.println(element); } public static void main(String args[]){ genericDisplay(11); genericDisplay("Welcome to the Geek Zone"); genericDisplay(1.0); } }The output of the above script would be the direct display of the parameters passed:
11 Welcome to the Geek Zone 1.0You see, the same function name is used for different types. This example also clarifies that there is no need to have a generic class in order to have generic methods.
Practical uses of Java Generic Method
Java Generic Methods increase flexibility in your code. An obvious benefit is that they hinder runtime ClassCastExceptions by ensuring that the correct type is used.Consider the situation where you need to count the number of elements in an array that have a specific property. You could write separate, almost identical methods for each element type or you could use a single generic method. Not only does the latter promote code reusability, but it also ensures type safety.
Deep Dive into Java Generic Class
Java Generic Classes extend the concept of Java Generics beyond methods and open the door for reusable and type-safe classes. This notion takes Java's concept of data abstraction a step further, allowing programmers to create a single class definition that can operate on a set of data types.Introduction to Java Generic Class
Circling back to the basics, classes in Java are the blueprint from which individual objects are created. These often contain methods and data members that work with different types of objects. The concept of Java Generic Class allows you to write a class once and then use it with any data type, including Integer, String, Double, or any user-defined types such as objects and arrays. Each Java Generic Class can have one or more type parameters in a comma-separated list. These classes define a set of generic datum that works on generic data types while ensuring full type safety. The performance is high because there's no requirement for type casting. It also reduces potential issues at runtime. A Java Generic Class has the following structure:public class JavaGenericClassThe \{ private T t; public void add(T t) { this.t = t; } public T get() { return t; } }
Exploring the structure of Java Generic Class
In the field of Computer Science, the structure or syntax of something is of great importance. So, let's delve a little deeper into understanding the structure of Java Generic Class. The crux of a generic class can be represented by the symbol < T > in the class definition. 'T' here is called the type parameter that indicates that it can refer to any type (like Integer or String). The type parameter can be used to declare the return type and the types of the parameters of the methods in a generic class, and also as a type of its fields. Here is a rudimentary structure of a Java Generic Class:public class GenericClassIn this structure, T is a type parameter that will be replaced by a real type when an object of GenericClass is created.{ T obj; void create(T obj) { this.obj = obj; } T obtain() { return obj; } }
Java Generic Class example in detail
Exploring Java Generic Class through an example is the best way to understand it better. Take a look at this comprehensive example to gain more clarity:public class GenericClassThe output of this code will be:{ private T t; public void add(T t) { this.t = t; } public T get() { return t; } public static void main(String[] args) { GenericClass integerBox = new GenericClass (); GenericClass stringBox = new GenericClass (); integerBox.add(new Integer(10)); stringBox.add(new String("Hello World")); System.out.printf("Integer Value :%d\n", integerBox.get()); System.out.printf("String Value :%s\n", stringBox.get()); } }
Integer Value :10 String Value :Hello WorldIn this code, a generic class named 'GenericClass' is created, which takes in parameters of generic type \
Exploring Java Generic Type and Interface Generic Java
The world of Java programming language carries a myriad of concepts to grasp, and at the heart of this expansive universe lies the idea of Java Generic Type and Interface Generic Java. It's crucial for you as an aspiring programmer to fortify your understanding of these concepts as they are known to enhance the functionality and flexibility of your code.Breakdown of Java Generic Type
In the realm of java programming, a Java Generic Type is essentially a generic class or interface that introduces a set of parameters. Highlighting its importance, the code snippet below demonstrates a Java program that uses Java Generic Type to create a simple box class:public class BoxTo add context to this, Java Generics were introduced to deal with type-safe objects. Before generics, we can store any type of objects in a collection i.e., non-generic. Now generics force the java programmer to store a specific type of objects. Here's an illustrative example of this concept:{ private T t; public void set(T t) { this.t = t; } public T get() { return t; } }
import java.util.ArrayList; import java.util.Iterator; class Test{ public static void main(String args[]){ ArrayListIn this example, the compiler checks to ensure that you add only a String to ArrayListlist=new ArrayList (); list.add("rahul"); list.add("jai"); //list.add(32);//compile time error String s=list.get(1);//type casting is not required System.out.println("element is: "+s); Iterator itr=list.iterator(); while(itr.hasNext()){ System.out.println(itr.next()); } } }
Java Generic Type Use Cases
Java Generic Type becomes incredibly powerful when it comes to creating collections of objects. For instance, when developing a method to sort an array of objects, you'd likely want to use different types of arrays like Integer, String, or perhaps a custom object Type. Java Generic Type lends itself well here, as it allows the sort method to have generic functionality. By using Java Generic Type, you can write a single method that sorts arrays of Integer, Double, String, or any type that supports comparison via the Comparable interface in Java. Ever wondered, how are you able to create an ArrayList that can store any type of objects? That's right, it's through the power of the Java Generic Type.Understanding Interface Generic Java
Diving into the concept of Interface Generic Java, you encounter yet another fascinating feature of Java Programming. Comparable and Comparator in Java are two interfaces, both present in java.util, which hold the power to permit Java Collection Classes (like ArrayList and LinkedList) to sort their elements. Interfaces in Java are similar to classes, but they contain only static constant variables or abstract method declarations. They are not fully defined classes, but they help to achieve complete abstraction. For illustration, consider this example of a simple Generic interface:public interface GenericInterfaceApproaching Interface Generic Java, you need to particularly bear in mind that when a class implements an interface, the methods provided by the class need to implement the interface-specific methods. Here's a simple usage example of Interface Generic Java:{ void performAction(final T action); }
public class ActionClass implements GenericInterfaceIn this example, ActionClass is implementing a generic interface and passing the required Type parameter, String, to this Interface Generic Java. Generic interfaces can be instantiated on the fly using anonymous classes, making them a versatile tool for generic method callbacks and the like. To summarise, Java Generic Type and Interface Generic Java are indispensable tools in any Java programmer's toolkit, harnessing the power of type-checking at compile-time and keeping your code bug-free and highly readable. Keep exploring these concepts and keep honing your skills for a robust and flexible programming experience.{ @Override public void performAction(final String action) { // Implementation here } }
Mastering Java Generics Syntax and Java Generics Restrictions
To obtain mastery over Java Generics, you need to have a grasp on Java Generics Syntax and its restrictions. This knowledge allows you to write better and more flexible code, while avoiding common pitfalls. These topics often come hand in hand, since understanding the restrictions is part and parcel of learning the syntax to implement Java Generics.Guide to Java Generics Syntax
The syntax of Java Generics encompasses a broad area and at its core, it consists of angle brackets <>, which contain the type parameter. Knowing the ins and outs of this syntax is vital because it empowers you to write code that’s less prone to bugs and is easier to read. Let’s delve into some crucial aspects of Java Generics syntax that you need to understand. • Type Parameter Naming Conventions: While you're free to choose any name for type parameters, it's common to use single, uppercase letters to make the code easier to read. The most commonly used type parameter names are:T – Type E – Element (used extensively by the Java Collections Framework) K – Key N – Number V – Value (used in Map) S, U, V, and so forth. - second, third, and fourth types in methods that have multiple type parameters• Generic Class and Interfaces: These are some key points about Generic Classes and Interfaces:
You define a generic class or a generic interface by adding a type parameter section, which is delimited by angle brackets, before the class or interface's name.
public class Box• Generic Methods: You can write a method that can accept parameters of different types. Here is a simple demonstration:{ // T stands for "Type" private T t; public void set(T t) { this.t = t; } public T get() { return t; } }
public staticvoid genericDisplay (T element){ System.out.println(element.getClass().getName() + " = " + element); }
Examples of using Java Generics Syntax
To better understand the use of Java Generics Syntax, here are some examples that showcase its usage: 1. Specifying a Type when Creating Objects: When creating an instance of a generic type, you must specify an actual type argument as a replacement for those angle brackets:Pair2. Creating a Pretty Pair: As an example, consider if you have a class called PrettyPair which extends Pair, you can do something like the following:p1 = new Pair<>("Even", 8); Pair p2 = new Pair<>("hello", "world");
PrettyPairpair = new PrettyPair<>(2, 4);
Understanding Java Generics Restrictions
As with any other programming methodology, Java Generics come with their own set of restrictions. These limitations are in place to ensure type safety and maintain the stability of your software. By getting a complete understanding of these constraints, you can prevent runtime errors and other common issues. Here are the primary restrictions that you should keep in mind whilst programming with Java Generics: • Primitive Types: You cannot use primitives as type parameters. Instead, you'll need to use the wrapper class corresponding to that primitive type. For example, instead of int, you would use Integer. • Static Fields or Methods: As a rule of thumb, you should know that a static field or method cannot reference the type parameters of its containing class. For example, this is not allowed:public class MyClass<T> { static T data = null; // Error: Cannot reference the type parameter T from a static context. }Then, there are some Overriding rules that one needs to follow pertaining to Java Generics.
How to navigate Java Generics Restrictions
Navigating around Java Generics restrictions requires understanding of both the restrictions and the alternate solutions available. Let's take a look at some of the common restrictions and how you can tackle them: 1. Instance Tests and Casts: Writing if (anObject instanceof Pair<T>) is illegal. You should utilise bounded wildcards when you wish to carry out an instance test or cast to a generic type. Additionally, you can utilise other workarounds like method overloading. 2. Create Arrays: You cannot create arrays where the element type is a type parameter, but you can declare variables of array types with a generic component. One way to do it is: Pair<Integer>[] pairArray = new Pair<Integer>[10]; 3. Varargs Warning: When you work with methods that have a variable number of type parameters (varargs), the compiler will generate a warning message as it is unsafe. The way to handle this is by using the @SafeVarargs annotation. Ensure you're acquainted with these restrictions and their alternatives to write clean and bug-free code that leverages the power of Java Generics.Java Generics - Key takeaways
- Java Generics is a concept in the Java programming language that enables developers to avoid passing objects into methods or classes and then casting them to another type. Instead, Java generics allow one to specify, at compile time, precisely what type of data a collection can contain. This avoids ClassCastException at runtime and provides stronger type checking.
- Java Generics can be applied in methods (Java Generic Method), classes (Java Generic Class), and interfaces (Interface Generic Java). By using Java Generics, developers can create a single method or class that can work with different datatypes, enhancing code reusability and type safety.
- Java Generic Method denotes a method that introduces its own type parameters, and can be distinguished by a type parameter section marked by angle brackets (< >) preceding the method's return type. It boosts code's flexibility by removing the need to create several methods for different types of data, thus enhancing code reusability and hindering runtime ClassCastExceptions.
- Java Generic Class enables the creation of a single class definition that can operate on a set of data types. This concept extends data abstraction further and eliminates the requirement for type casting, which improves performance. The same class can believe different types of data, showcasing the versatility of Java Generics.
- Java Generic Type and Interface Generic Java further extend the functionality of Java Generics. Java Generic Type introduces a set of parameters for a generic class or interface. On the other hand, Interface Generic Java allows classes implementing an interface to have type-specific methods, thus enhancing the functionality and flexibility of the code.
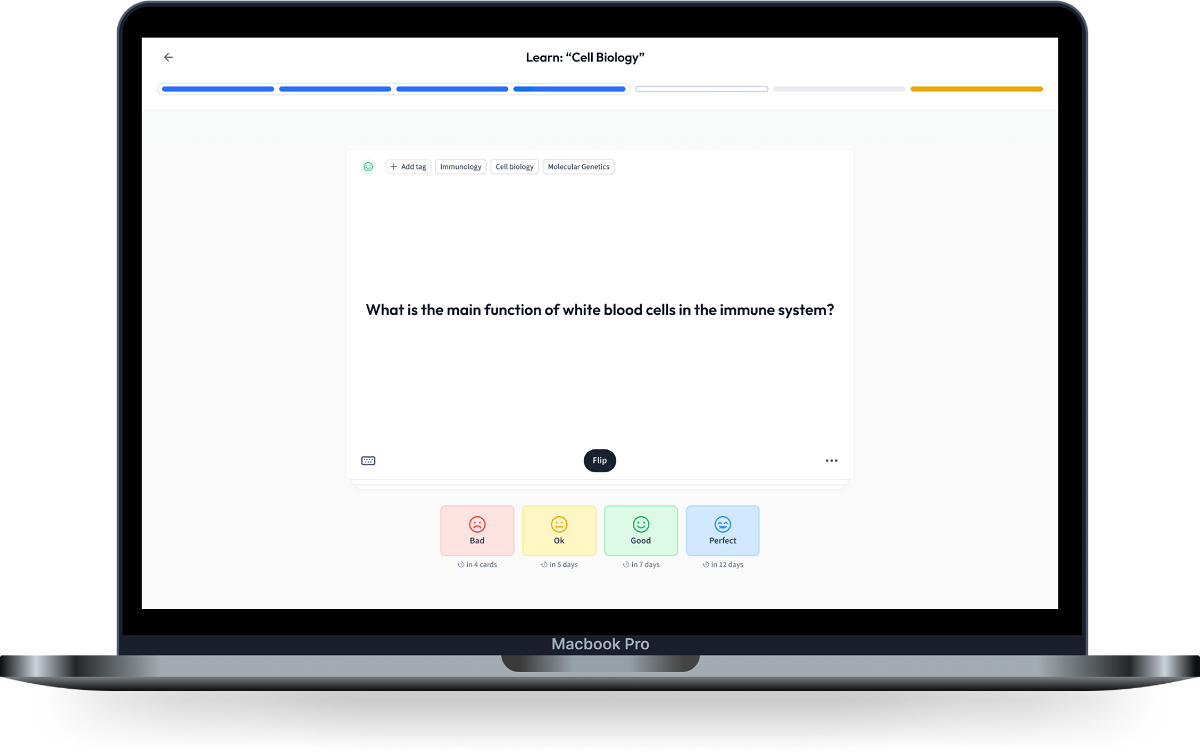
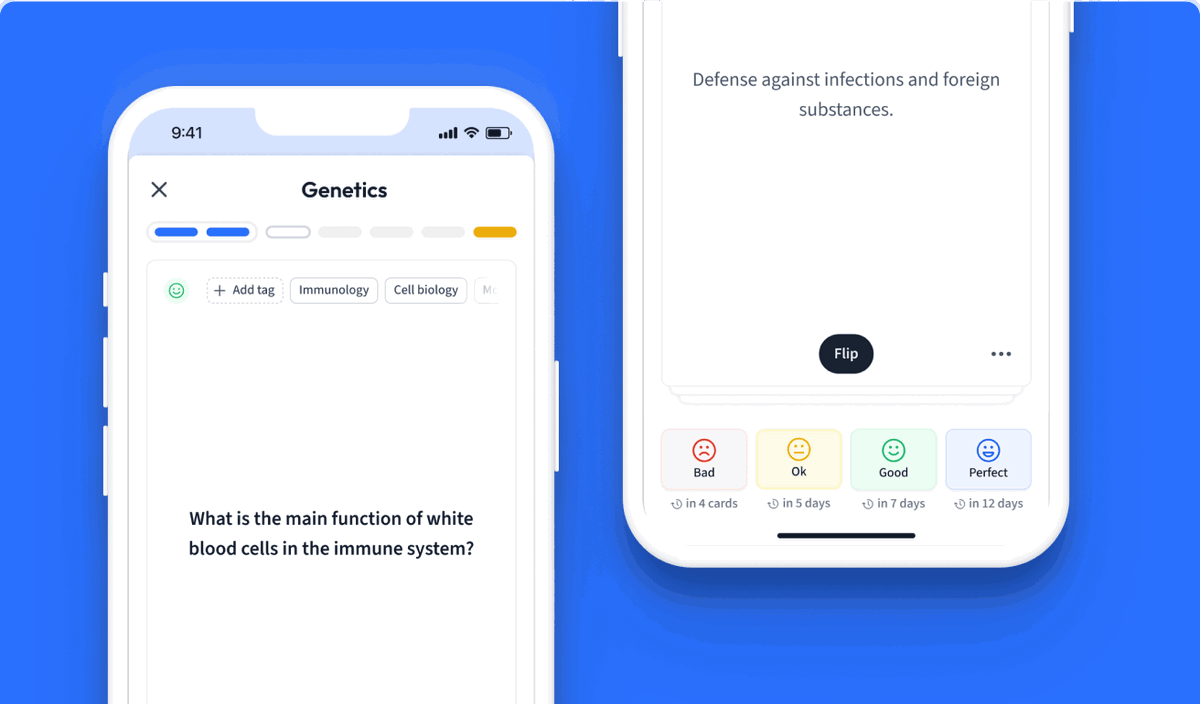
Learn with 15 Java Generics flashcards in the free StudySmarter app
Already have an account? Log in
Frequently Asked Questions about Java Generics
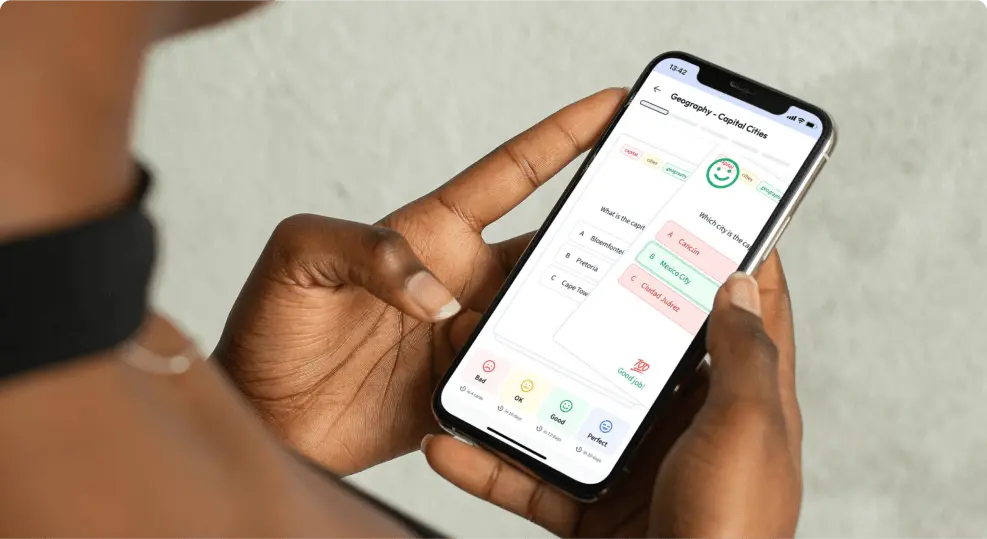
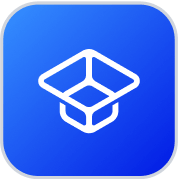
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more