Jump to a key chapter
Java Interfaces - Definition
Java Interfaces are a crucial concept in the world of programming with Java. They allow you to define a contract that classes are expected to follow, without dictating how the classes should fulfill that contract. This provides flexibility and helps in achieving a clean design in code architecture.
Java Interface Definition and Examples
Java Interface: In Java, an interface is a reference type, similar to a class, that can contain only constants, method signatures, default methods, static methods, and nested types. Interfaces cannot contain instance fields but can define methods that implementing classes must override.
Using interfaces, you can achieve:
- Abstraction: Abstract out the details and outline a structure.
- Multiple Inheritance: A class can implement multiple interfaces.
- Loose Coupling: Work with implementations only through specified contracts.
Examples of Java Interfaces are prevalent in various scenarios, from iterators in collections to listeners in graphical user interfaces. Consider the following example implementation of an interface:
public interface Animal { void eat(); void move(); }
Here, Animal is an interface with two method declarations: eat and move. Any class that implements this interface must provide implementations for these methods.
Java achieved multiple inheritance concept through interfaces, which is a powerful feature. A single class can implement multiple interfaces, allowing it to inherit the behavior from different sources safely, without the complications associated with multiple inheritance of classes. For instance, if an interface Vehicle and another EnvironmentFriendly exists, a class like ElectricCar can implement both, gaining functionalities from both through a well-organized design.
Understanding Java Interface Syntax
When defining a Java Interface, you follow a specific syntax distinct from class declarations. The interface keyword is used in place of class, and methods are left without implementations. Here's a breakdown of the syntax:
interface InterfaceName { returnType methodName(parameterList); }
Key components include:
- Interface Keyword: Indicates the definition of an interface.
- Method Signatures: Declared within the interface without any body.
- Modifiers: Methods in interfaces are implicitly public and abstract by default, while fields are public, static, and final.
For example, the java.util.Comparable interface is defined as:
public interface Comparable{ int compareTo(T o); }
Any class implementing Comparable must provide code for compareTo, with practical use in sorting objects.
Remember: Interfaces cannot have constructors because they cannot be instantiated.
Java Interface Examples and Usage
Learning through examples is one of the best methods to deeply grasp concepts, especially in programming. Below are some practical scenarios and examples where Java Interfaces play a vital role.
Basic Java Interface Example
A basic understanding of how interfaces are defined and implemented provides a strong foundation for further exploration. Consider a simple example using a Java Interface to define operations for a basic calculator:
public interface Calculator { int add(int a, int b); int subtract(int a, int b); }
In this case, the Calculator interface specifies two methods: add and subtract. A class implementing this interface would provide its own definitions for these methods.
Let's see how a class implements this interface:
public class SimpleCalculator implements Calculator { @Override public int add(int a, int b) { return a + b; } @Override public int subtract(int a, int b) { return a - b; } }
The SimpleCalculator class provides functional logic for the add and subtract operations as defined by the interface.
Interfaces promote code reusability and consistency without enforcing specific implementation details.
Advanced Examples in Java Interfaces
Advancing to more complex examples, Java Interfaces allow for intricate designs where different classes require adhering to the same set of behaviors. Such designs are commonplace in Java's internal libraries.
Consider the following interface for multiple types of payment methods:
public interface Payment { void initiateTransaction(double amount); boolean confirmPayment(); }
Below is an example of a class implementing this interface for a credit card payment:
public class CreditCardPayment implements Payment { @Override public void initiateTransaction(double amount) { // Call payment gateway API } @Override public boolean confirmPayment() { // Confirm transaction status return true; } }
With this design, you can implement numerous payment methods like PaypalPayment, CryptoPayment, etc., all conforming to the Payment interface, ensuring uniformity across payment operations.
By using interfaces, you can implement the powerful Strategy Design Pattern, which defines a family of algorithms, encapsulates each one of them, and makes them interchangeable within the same family. This dynamic selection of operational methods can drastically reduce code complexity when managing slightly different processes, akin to sorting different data structures or handling various data formats.
Importance of Interfaces in Java Programming
Understanding the importance of interfaces in Java equips you with the knowledge to design flexible and scalable applications. Interfaces define a set of methods that implementing classes must include, thus establishing a contract for what a class can do.
Why Use Java Interfaces?
Java interfaces are used for a variety of reasons, primarily to achieve abstraction and multiple inheritance in Java. They allow for separation of implementation and enable multiple classes to share a common set of methods, which aids in maintaining and extending code.
Abstraction: In Object-Oriented Programming, abstraction is the concept of hiding complex implementation details and showing only essential features of an object. Interfaces help achieve this by defining what a class must do, not how it must do it.
Consider the following scenario:
public interface Rechargeable { void recharge(); }
public class ElectricCar implements Rechargeable { @Override public void recharge() { // Code to recharge car battery } }
By using an interface, any class implementing Rechargeable must provide the recharge method, ensuring all rechargeable items can be managed consistently.
Interfaces allow for the implementation of multiple interfaces by a single class, providing greater flexibility than single inheritance.
Advantages of Java Interfaces
Utilizing Java interfaces offers numerous advantages that contribute to cleaner and more efficient code.
When designing applications, applying interfaces can facilitate the Dependency Inversion Principle of software development. It suggests that high-level modules should not depend on low-level modules but both should depend upon abstractions. This is easily achieved with interfaces, as shown in common enterprise solutions where you might have:
- Data access interfaces that various database engines implement.
- User authentication mechanisms that support various protocols.
This approach allows swapping out the underlying implementation without altering the overall architecture, enhancing the application's ability to evolve over time.
Here are the primary advantages of using interfaces:
- Flexibility: Define behaviors that different classes may adopt and implement, reducing duplication.
- Code Organization: Separate the definition of methods from their implementations.
- Customize Class Behavior: Use interfaces to vary behavior without impacting the larger application.
Good interface design leads to designing modular systems that are easy to maintain, scale, and debug.
How to Use Interfaces Properly Java
Proper use of interfaces in Java ensures that your code achieves the right level of abstraction, reduces redundancy, and supports flexibility. By outlining a set of methods that classes implementing these interfaces must provide, interfaces dictate how functions should be used without detailing what each class must do.
How to Use Function from Interface in Java
To effectively use a function from a Java interface, you need to understand its basic mechanics: specifying the function within the interface, implementing the function in a class, and accessing it through an instance of the class. Interfaces help maintain consistency in your code by ensuring that certain functionalities follow predefined patterns.
For instance, consider an interface that defines sorting behavior:
public interface Sortable { void sort(); }
A class implementing this interface would look like:
public class ArraySorter implements Sortable { @Override public void sort() { // Sorting logic for arrays } }
When calling the sort method:
Sortable sorter = new ArraySorter(); sorter.sort();
Here, the sort method is accessed through the implemented class, ArraySorter.
Incorporating interfaces into real-world scenarios can greatly enhance code practices. For example, consider the Java Collection Framework, where interfaces like List, Set, and Map allow you to interact with various data structures consistently. You use methods from these interfaces to modify collections without worrying about the underlying implementation, whether it is an array-based list or a linked list.
Remember that interfaces can also contain default and static methods since Java 8, providing additional shared implementation.
Do You Need Override for Interface Java?
When implementing a Java interface, it is necessary to provide an implementation for each method declared in the interface unless the class is abstract. The @Override annotation is commonly used for this purpose. Although not mandatory, using @Override when implementing interface methods is considered good practice as it makes your intention clear and helps catch errors.
Consider this example:
public interface Notifiable { void notifyUser(); }
public class EmailNotifier implements Notifiable { @Override public void notifyUser() { // Send email notification logic } }
Here, the @Override annotation indicates that notifyUser is an implementation of the interface-defined method.
One important consideration when working with interfaces is exploring how they interact with inheritance. If an interface method signature matches a method in a class hierarchy, the implementing class effectively 'inherits' functionality from its superclass and any additional interfaces it implements. This provides a high degree of method specificity and reuse within Java's robust inheritance model.
Using @Override ensures you are correctly fulfilling the intended contract of the interface.
Java Interfaces - Key takeaways
- Java Interface Definition: A reference type in Java similar to a class that contains constants, method signatures, and default/static methods but no instance fields.
- Usage of Java Interfaces: Interfaces allow for abstraction, enable multiple inheritance, and promote loose coupling in Java, making code flexible and maintainable.
- Java Interface Examples: Common in scenarios like iterators, listeners, and defining operations for objects, such as the
Animal
interface witheat
andmove
methods. - Syntax for Interfaces: Interfaces use the
interface
keyword, require method signatures without bodies, and have methods implicitly public and abstract. - Importance of Interfaces: They outline method contracts for classes to enhance design flexibility, scalability, and adherence to the Dependency Inversion Principle.
- Using @Override: While not required, using @Override when implementing interface methods clarifies intention and helps minimize errors in adherence to interface contracts.
Learn faster with the 27 flashcards about Java Interfaces
Sign up for free to gain access to all our flashcards.
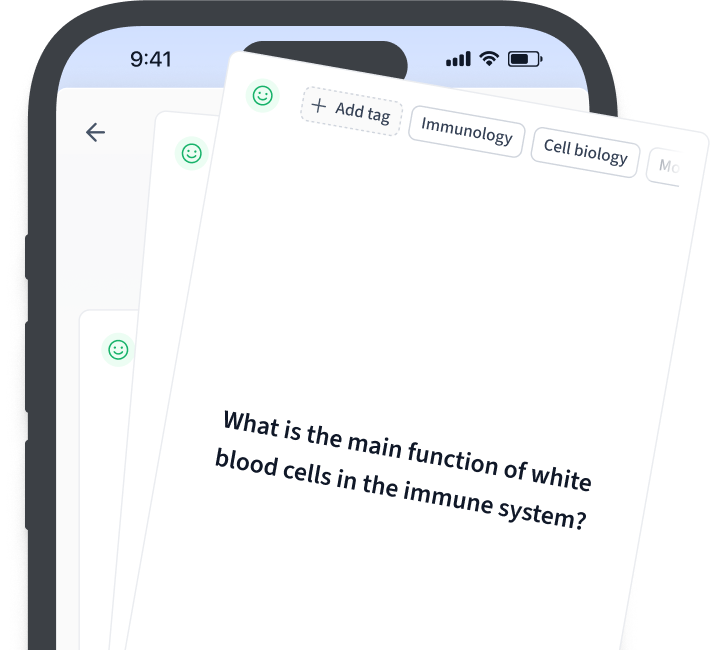
Frequently Asked Questions about Java Interfaces
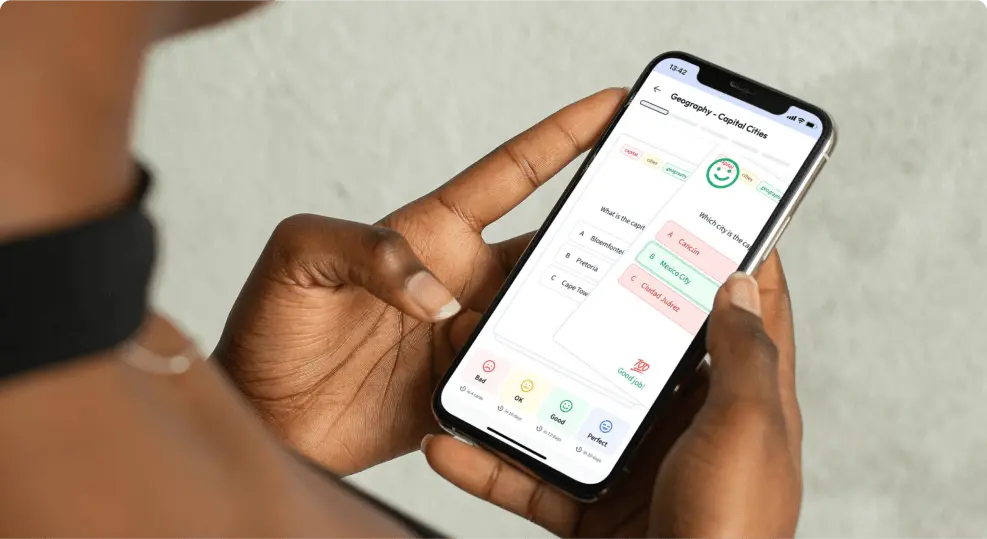
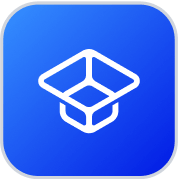
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more