Jump to a key chapter
Java Loops Overview
Learning how to use loops in Java is crucial to developing effective programming skills. Loops allow you to run a block of code multiple times under certain conditions, facilitating tasks like iterating over data structures and automating repetitive tasks.
Types of Loops in Java
Java offers several types of loops to suit different programming needs. Understanding each type will help you choose the best loop for your specific task.
- For Loop: Ideal for situations with a specific number of iterations.
- While Loop: Best for iterating when the number of iterations is not known upfront.
- Do-While Loop: Ensures at least one execution of the loop body regardless of the iteration condition.
For Loop is a control flow statement for specifying iteration, which allows code to be executed repeatedly.
for (int i = 0; i < 5; i++) { System.out.println(i); }This example prints numbers from 0 to 4 using a for loop.
Use a for loop when the number of iterations is clearly defined and predictable.
Understanding While Loops
The While Loop is perfect for scenarios where the looping condition is not predefined. It continues execution as long as a specified condition is true, making it useful for applications where conditions may evolve during runtime.
int count = 0; while (count < 5) { System.out.println(count); count++; }This example demonstrates a while loop printing numbers from 0 to 4.
Behind the scenes, both the while loop and the for loop perform similar tasks, but they offer different syntactical conveniences. For loops are generally more compact, especially when dealing with a counter. However, while loops offer more flexibility when dealing with conditions that do not neatly fit into a counting pattern. Choosing between them often comes down to personal preference and the specific requirements of the problem you are trying to solve.
Exploring Do-While Loops
The Do-While Loop guarantees that the code block inside the loop executes at least once, regardless of the initial state of the condition. This type of loop checks the condition after executing the loop's body, making it unique among Java loops.
int count = 0; do { System.out.println(count); count++; } while (count < 5);The above example uses a do-while loop to print numbers from 0 to 4.
Tailor your use of do-while loops for situations where at least one iteration is necessary, such as menu processing where the user is prompted repeatedly until a valid input is entered.
Java Loops Explained
Loops are essential in Java programming, allowing you to execute a block of code multiple times efficiently. Java offers several types of loops to suit different scenarios, including for loops, while loops, and do-while loops. They play a critical role in tasks like iteration over collections, performing repetitive tasks, and controlling complex workflows.Every type of loop in Java is designed to handle specific conditions, making it important for you to understand the nuances of each. Whether you're iterating over a fixed number of elements, responding to dynamic conditions, or ensuring at least one execution, there's a loop that fits your need.
Understanding Loops in Java
Java provides three basic types of loops: for loop, while loop, and do-while loop. Each loop type offers distinct benefits based on the programming requirement.
- The for loop is used when the number of iterations is known beforehand.
- The while loop is suitable where iterations occur based on a specific condition, whose count isn't initially known.
- The do-while loop ensures that the loop body executes at least once before evaluating the condition.
for (int i = 0; i < 10; i++) { System.out.println(i); }Here, the for loop iterates from 0 to 9.
The primary difference between these loop types can be understood by considering their execution flow. In a for loop, all loop-related expressions are consolidated into a single line, making it compact and convenient for index-based iterations. On the other hand, while and do-while loops are condition-based, primarily used when the number of iterations is not predetermined. A while loop checks its condition before executing, whereas a do-while loop executes its body first and validates the condition later, ensuring the loop executes at least once.
Java Loop Concepts
Java loops use control statements to direct the flow of execution. Using control statements like break and continue, you can manipulate loop behavior.These concepts offer flexibility and control over the sequences of operations.Break: Terminates the loop immediately, and the control moves to the next statement following the loop.Continue: Skips the remaining statements in the current iteration and moves to the next iteration.
for (int i = 0; i < 10; i++) { if (i == 5) { break; } System.out.println(i); }This code demonstrates the use of the break statement to terminate the loop when i equals 5.
Remember, use break to exit a loop early when a condition is met, whereas continue can be used to skip specific iterations.
Java For Loop
The Java For Loop is a fundamental control flow statement in Java programming used for iterating a block of code a specific number of times. It is particularly useful in scenarios where the number of iterations is known beforehand. This loop is valued for its compact structure, combining initialization, condition-checking, and iteration increment in a single line.
Java For Loop Syntax
The syntax of the Java for loop is detailed and allows customization for different scenarios. Here's the basic form:
for (initialization; condition; increment/decrement) { // Code to be executed }
- Initialization: Sets the starting point; typically initializes a counter variable.
- Condition: Evaluated before each loop iteration - the loop runs as long as this is true.
- Increment/Decrement: Updates the loop control variable after each iteration to eventually terminate the loop.
Optimize performance by making your loop condition statement direct and simple, ensuring the loop ends properly.
Java For Loop Examples
Let's explore some practical examples to understand how the Java for loop can be applied in different coding scenarios.
for (int i = 0; i < 5; i++) { System.out.println('Hello World'); }This loop will print 'Hello World' five times, illustrating the basic use of a for loop.
The behavior and efficiency of the Java for loop can greatly influence the performance of your application. For example, consider a loop iterating over an array:
int[] numbers = {1, 2, 3, 4, 5}; for (int i = 0; i < numbers.length; i++) { System.out.println(numbers[i]); }This demonstrates how a for loop iterates through an array. The use of the array's length property ensures the loop runs through all elements without causing an ArrayIndexOutOfBoundsException. Using loops on data structures efficiently can optimize application performance significantly by reducing unnecessary computation and enhancing readability.
for (int i = 10; i > 0; i--) { System.out.println('Countdown: ' + i); }This countdown example illustrates decrementing the loop variable, an effective use for reverse ordering tasks.
While Loop Java
The While Loop in Java is essential when you need a loop whose iteration count isn’t known beforehand. It repeatedly executes a block of statements as long as a given condition evaluates to true. This makes it incredibly valuable in various scenarios where conditions dictate the loop's completion.
While Loop Java Syntax
Understanding the syntax of the while loop is crucial for implementing it effectively in your Java programs. Here's the general structure of a while loop:
while (condition) { // Code block to be executed }
- Condition: This is a boolean expression. The loop continues as long as this evaluates to true.
- Code Block: Contains the statements that are executed in each iteration of the loop.
Infinite Loop: A loop that continuously executes because the termination condition is never met.
Always make sure the condition within a while loop eventually evaluates to false to prevent infinite loops.
While Loop Java Examples
Examples help clarify the application and utility of the while loop. Here’s how it can be utilized in different scenarios:
int i = 0; while (i < 5) { System.out.println(i); i++; }This loop prints numbers from 0 to 4, displaying a practical use of index incrementation within a while loop.
In the context of more complex data handling, while loops provide flexibility. Consider processing user input until a specific termination condition is met. In such cases, the while loop accommodates dynamic control flow changes influenced by user interactions.For example, you may want to read input until a 'quit' command is issued:
Scanner scanner = new Scanner(System.in); String command; while (!(command = scanner.nextLine()).equals('quit')) { System.out.println('Your input: ' + command); }This example emphasizes using a while loop to handle varying data dynamically. By examining its versatility, you’ll find it often fits well in data processing loops, even in complex scenarios where real-time conditions affect loop execution.
Java Loops - Key takeaways
- Java Loops: Essential for executing a block of code multiple times under certain conditions, aiding tasks like iteration and automation.
- For Loop in Java: Ideal for situations with a known number of iterations, with syntax including initialization, condition, and increment/decrement.
- While Loop Java: Suitable when the number of iterations isn't predefined, as it runs code blocks while a condition remains true.
- Do-While Loop: Ensures the loop executes at least once before checking the condition, useful in scenarios needing at least one execution.
- Java Loops Explained: Different loops are suited for different tasks; choosing the right one depends on the iteration requirements.
- Java Loop Concepts: Control flow using loops like for, while, and do-while, with tools like break and continue for managing loop executions.
Learn faster with the 27 flashcards about Java Loops
Sign up for free to gain access to all our flashcards.
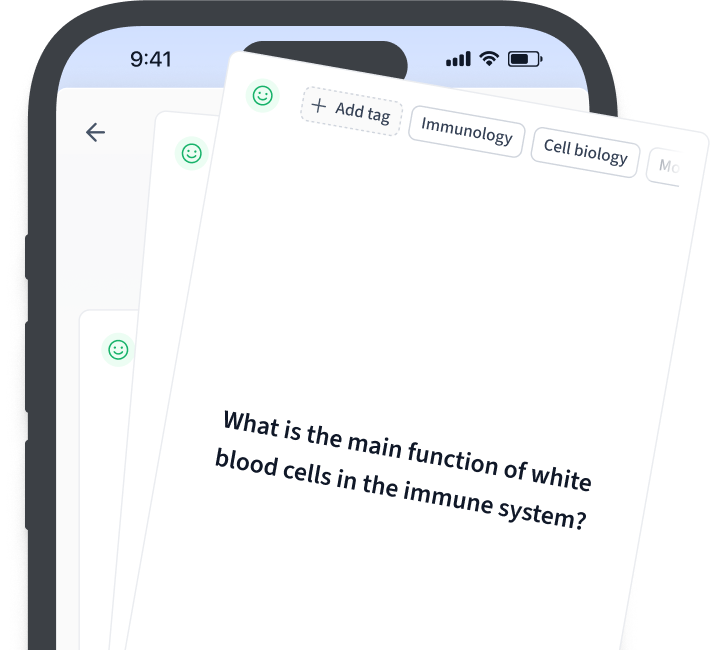
Frequently Asked Questions about Java Loops
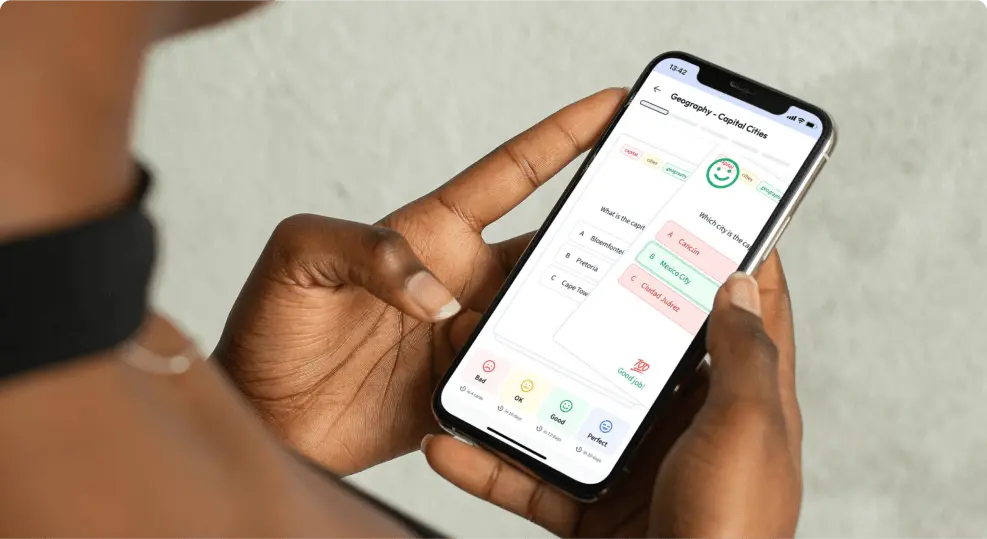
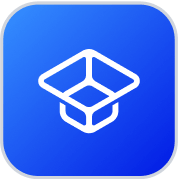
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more