Jump to a key chapter
Understanding Java Multiple Catch Blocks
Understanding Java Multiple Catch Blocks is key to programming in Java. These blocks allow multiple exceptions to be caught, resulting in better error handling in your code. Java programming often involves managing many types of errors and exceptions. These can range from file errors, network errors, to null pointer exceptions. Java Multiple Catch Blocks are functional programming blocks devised to deal with a diverse set of exceptions that can occur while executing Java code.In Java, an exception is an event that disrupts the normal flow of the program. It is an object which is thrown at runtime.
The function of Java Multiple Catch Blocks
Java Multiple Catch Blocks work to handle different exceptions separately. They serve the essential function of controlling the flow of execution when these assorted exceptions occur.For instance, you might want to print a personalised error message or perform a specific action when a FileNotFoundException occurs. But if it's an ArithmeticException (like attempting to divide by zero), you'd want to do something different - you might want to instantly halt the program or throw a custom exception. Java Multiple Catch Blocks allow you to do just this. They let you set up distinct responses for different exceptional circumstances.
try { // code that might throw different exceptions } catch (FileNotFoundException e) { // code to handle a FileNotFoundException } catch (ArithmeticException e) { // code to handle an ArithmeticException } catch (Exception e) { // code to handle any other Exceptions }
How Java Multiple Catch Blocks improve code readability and efficiency
Java Multiple Catch Blocks fundamentally enhance code readability by segregating error management code based on the type of exception. By using numerous catch blocks, you can understand swiftly which block pertains to which exception type - leading to more legible and maintainable code. On the efficiency front, Multiple Catch Blocks support very particular, individualised error handling approaches for each exception. This significantly improves the robustness of your code and also ensures resource efficiency. By tightly associating exception types with corresponding error-handling logic, you can avoid a one-size-fits-all approach and devise highly efficient, case-specific responses to exceptions. Another benefit lies in the ability of Multiple Catch Blocks to handle old code. Even if your Java program is calling a method from a library or an older piece of code that lacks thorough exception documentation, using multiples catch blocks can create a wider safety net.With Java 7 and onward, you can handle more than one type of exception in a single catch block, using a feature called multi-catch. In multi-catch, types of exceptions are disconnected by a pipe (|) character in the catch clause. Willing to go even deeper? Here's how it works in practice:
try { // code that might throw different exceptions } catch (FileNotFoundException | ArithmeticException e) { // code to handle either a FileNotFoundException or an ArithmeticException } catch (Exception e) { // code to handle any other Exceptions }
How To Use Multiple Catch Blocks In Java
Using multiple catch blocks in Java is a straightforward procedure once you understand the concept. Essentially, it involves identifying the potential exceptions your code might throw, then creating separate catch blocks for each of these. Every catch block should have a unique type of exception to handle potential pitfalls that may arise during the execution of your code.Step-by-step guide on adding multiple catch blocks in Java
When adding multiple catch blocks in Java, it is crucial you follow these steps with due care: 1. **Identify potential exceptions**: The first step lies in the proper identification of the exceptions your code might throw. These could include Input/Output exceptions, NullPointer exceptions, Arithmetic exceptions, and so forth. 2. **Creating the try block**: Once you've identified these exceptions, encapsulate the code that might cause these exceptions within a try block. The 'try' keyword is used to specify a block where reverse engineering is suspected to arise. 3. **Adding multiple catch blocks**: For each type of exception identified, you should add a catch block. The catch block captures the exception thrown by the try block and executes a series of instructions to handle the exception. Let's consider an example that illustrates the step-by-step process.try { int a = 30, b = 0; int c = a/b; // this will throw ArithmeticException System.out.println ("Result = " + c); } catch (ArithmeticException e) { System.out.println ("You cannot divide by zero " + e); } catch (Exception e) { System.out.println ("Exception caught = " + e); }In this example, the code within the try block throws an ArithmeticException. This exception is then caught by the ArithmeticException catch block.
The practical usage of multiple try-catch blocks in Java
To understand the practical usage of multiple try-catch blocks, let's analyse an example:try { // code that might throw different exceptions openFile(fileName); // may throw IOException processFile(); // may throw FileFormatException writeFile(); // may throw IOException } catch (IOException e) { // code to handle IOException System.out.println("Input-output exception occurred: " + e.getMessage()); } catch (FileFormatException e) { // code to handle FileFormatException System.out.println("The file format is incorrect: " + e.getMessage()); } catch (Exception e) { // code to handle any other Exceptions System.out.println("Unexpected error: " + e.getMessage()); }In this example, the functions openFile(), processFile(), and writeFile() may throw different types of exceptions. Here, exceptions are handled by appropriate catch blocks. Overall, the practical usage of multiple catch blocks provides a platform for Java programs to be more robust in the face of unexpected hurdles, enhancing their efficiency, readability, and reliability in the process.
Can We Have Multiple Catch Blocks In Java?
Yes, absolutely! In Java, you can indeed have multiple catch blocks. This is a key feature of Java's exception handling mechanism that enhances both code readability and your ability to effectively manage a wide variety of exceptions which may interrupt the normal course of a program's execution.Exploring the possibility of Java catch multiple exceptions in one block
Now let's dive into the nitty-gritty of catching multiple exceptions in one block in Java. Firstly, it's critical to understand that this capability was introduced only from Java 7 onward. Thus, if you're dealing with older versions of Java, you will not be able to leverage this feature and would need to stick with individual catch blocks for each exception type. However, with Java 7 and beyond, you can catch multiple exceptions in one single block using a syntax that is both simple and intuitive. This is commonly referred to as multi-catch. The procedure involves specifying multiple exception types in a single catch block, separated by the pipe ('|') symbol. Here's a small example to illustrate:try { // Block of code that might throw exceptions } catch (IOException | SQLException | ClassNotFoundException e) { // Handling for all three exception types }In the above example, the single catch block will catch and handle any of the three exception types: IOException, SQLException, and ClassNotFoundException. This streamlines your code and eliminates the need for duplicated handling logic across multiple catch blocks. It's noteworthy that the catch parameter (in our case, 'e') is implicitly final, which means you can't alter its value within the catch block. Additionally, the multi-catch feature only works with exceptions that are disjoint, i.e., not in an inheritance chain. This is to avoid hiding catch clauses for subclass exception types, which may lead to unreachable code.
Detailed review of Java Multiple Catch Blocks rules and restrictions
While the multiple catch blocks feature in Java makes exception handling significantly more flexible and efficient, there are a few important rules and restrictions you need to be aware of:- Order of catch blocks: The order of the catch blocks matters. You should place catch blocks for more specific exception types before those for more general types. This allows Java to match the most specific exception type first. Not abiding by this rule will give you a compilation error.
- Disjoint exceptions: When using multi-catch in Java 7 and onwards, the exceptions you list in the catch clause should be disjoint. That means they shouldn't have an inheritance relationship. If one exception is a subclass of another, a compilation error will occur.{ Keep in mind that a multi-catch clause becomes unreachable if a preceding catch block can catch the same exception class. }
- Final catch parameter: In a multi-catch block, the catch parameter is effectively final. That means you can't reassign it within the catch block. Attempting to do so generates a compilation error.
try { int data = 50/0; } catch (Exception e){ System.out.println(e); } catch (ArithmeticException e) { System.out.println(e); }When you try to run the code above, the Java compiler will let you know that there is an unreachable catch block because the catch block for ArithmeticException is unreachable. Correct ordering of catch blocks:
try { int data = 50/0; } catch (ArithmeticException e) { System.out.println(e); } catch (Exception e){ System.out.println(e); }In the revised code, you catch the more specific ArithmeticException first, followed by the more general Exception type. These rules uphold the principle of handling exceptions in a manner that's both efficient and robust, ensuring your Java code maintains high performance levels and reliability.
Practical Examples of Java Multiple Catch Blocks
It's time to roll up your sleeves and delve into some practical examples of Java Multiple Catch Blocks. This section will explain in detail how to implement multiple catch blocks in Java using straightforward and complex cases.Simple Java multiple catch blocks example
Let's start off with a simple example where two types of exceptions might occur: ArithmeticException and ArrayIndexOutOfBoundsException.try { int arr[] = new int[5]; arr[6] = 30 / 0; // This line will throw ArithmeticException and ArrayIndexOutOfBoundsException } catch (ArithmeticException e) { System.out.println ("Cannot divide by zero"); } catch (ArrayIndexOutOfBoundsException e) { System.out.println ("Array index out of bounds"); }In the code block above, if you were to run this program, it would catch the ArithmeticException, and the program would terminate after printing "Cannot divide by zero". Notice that the ArrayIndexOutOfBoundsException is not handled even though it's part of the code. This is because once an exception is thrown and matched with a catch block, the remaining code is not executed.
Advanced example: Java catch multiple exceptions in one block
Suppose you're interacting with a database. During this operation, you may encounter different types of exceptions such as ClassNotFoundException (if the database driver is not found) and SQLException (if there's a problem accessing the database). Here's how you can use multiple catch blocks to handle these exceptions:try { Class.forName("com.mysql.jdbc.Driver"); Connection con = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydb", "myuser", "mypassword"); } catch (ClassNotFoundException e) { System.out.println ("Database driver not found: " + e); } catch (SQLException e) { System.out.println ("Database access error: " + e); }In the code above, the try block contains database connectivity routines. If for some reason, the Java interpreter can't find the specified database driver (com.mysql.jdbc.Driver in this case), a ClassNotFoundException can be thrown. Alternatively, a SQLException might be thrown when there's an error accessing the database. These exceptions are individually caught and handled by their respective catch blocks. With multiple catch blocks, you're better prepared for the various exception types your code might throw. It's essential to remember that these catch blocks should be arranged from most specific to least specific exception types to ensure the correct catch block handles each exception. For better code structure and readability, Java has improved the handling of multiple exceptions in one block from Java 7 and onwards. Instead of writing separate catch blocks to handle each exception, you can handle more than one exceptions in one catch block, separated by a vertical bar (|).
try { Class.forName("com.mysql.jdbc.Driver"); Connection con = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydb", "myuser", "mypassword"); } catch (ClassNotFoundException | SQLException e) { System.out.println ("Error accessing database: " + e); }This doesn't just tidy up your code, but it also eliminates the need to repeat identical error-handling code in each catch block. Now, if either ClassNotFoundException or SQLException occurs, the combined catch block will handle it, streamlining the exception handling process.
Managing Errors Efficiently with Multiple Try Catch Block in Java
The importance of robust error management in Java programming cannot be overstated. Given Java's usage across a diverse range of applications, from web-apps to standalone solutions, effective error management can significantly impact the reliability and efficiency of your software. The multiple try-catch block feature in Java allows for a fine-grained error management strategy, distinctly capturing and processing different types of exceptions.How to add multiple catch blocks in Java for better error handling
It's no secret that Java's error-handling capabilities greatly contribute to its popularity. By adopting the right strategy, you can ensure the resilience and robustness of your software. Adding multiple catch blocks to a try block lets you handle different types of exceptions in their own specific ways, which can be highly beneficial for customized error reporting and handling. It's important to note that a 'try' block can be followed by more than one 'catch' block. The syntax for a try-catch block allows you to add any number of catch blocks. The catch blocks must directly follow the try block or another catch block. This is the general syntax for multiple try catch blocks in Java:try { // code that may raise exceptions } catch(exception_type1 e) { // handling code for exception_type1 } catch(exception_type2 e) { // handling code for exception_type2 } // any number of additional catch blocks... finally { // optionally, cleanup code. This block executes regardless of an exception being thrown or not. }Here, 'exception_type1' and 'exception_type2' are placeholders for actual exception classes in Java, such as IOException, SQLException, or even a custom-defined exception. The 'e' following the exception type is the exception object, which contains further information about the error, such as an explanatory message and stack trace. When an error takes place within the try block, Java will stop further execution and check the catch blocks from top to bottom. It pairs the exception with the first catch block that matches the exception type, executing the corresponding error-handling code. The rest of the catch blocks are ignored in this run. For instance, assume we have a try block, followed by two catch blocks catching an ArithmeticException and an ArrayIndexOutOfBoundsException, respectively. Now, if an ArithmeticException occurs within the try block, the first catch block executes and subsequent catch blocks are ignored. It's crucial to remember to order your catch blocks carefully, from the specific to the general. If a catch block for a general exception (like Exception) is placed before a catch block for a specific exception (like IOException), the compiler will generate an error, as the code would become unreachable. Here's an example to clarify:
try { // some risky code } catch(Exception e) { // catch all types of exceptions. 'Exception' is a super class of all exceptions } catch(IOException e) { // this block will never be reached, because 'IOException' is a subclass of 'Exception' }In the snippet above, an "unreachable code" error is thrown because the catch block for IOException will never be reached. The previous catch block that catches all exceptions, including an IOException, supersedes it.
The impact of smart error management on Java programming
Strategic error management can greatly improve the resilience and user experience of your Java programs. Proper exception handling can mean the difference between an application crashing and it gracefully recovering from an error while providing the user with a helpful message. Adding multiple catch blocks in Java is an effective way to provide customised error handling depending on the specific type of exception that gets thrown. It allows for greater flexibility and control over error handling.A common misconception among beginner programmers is that the "catch" in a catch block means it somehow prevents exceptions. This is not the case. The catch block only provides a way to respond to exceptions and continue operation - the errors have already occurred when the catch block gets control.
Java Multiple Catch Blocks - Key takeaways
- Java Multiple Catch Blocks allow handling of different exception types within the same try scope with individualised error handling strategies, leading to more robust and efficient Java programs.
- Java 7 onwards, 'multi-catch' feature is introduced, which allows handling of more than one type of exception in a single catch block. The different exception types are separated by a pipe (|) character. For instance, 'catch (FileNotFoundException | ArithmeticException e) {}'.
- The process of handling errors using multiple catch blocks in Java involves identifying potential exceptions your code might throw, encapsulating the code within a try block, and creating distinct catch blocks for each identified exception type.
- Multiple catch blocks in Java is capable of handling old code, even if the Java program is calling a method from a library or an older piece of code that lacks thorough exception documentation.
- 'Multi-catch' in Java works only with disjoint exceptions, i.e., exceptions that are not in an inheritance chain, to avoid unreachable code situations.
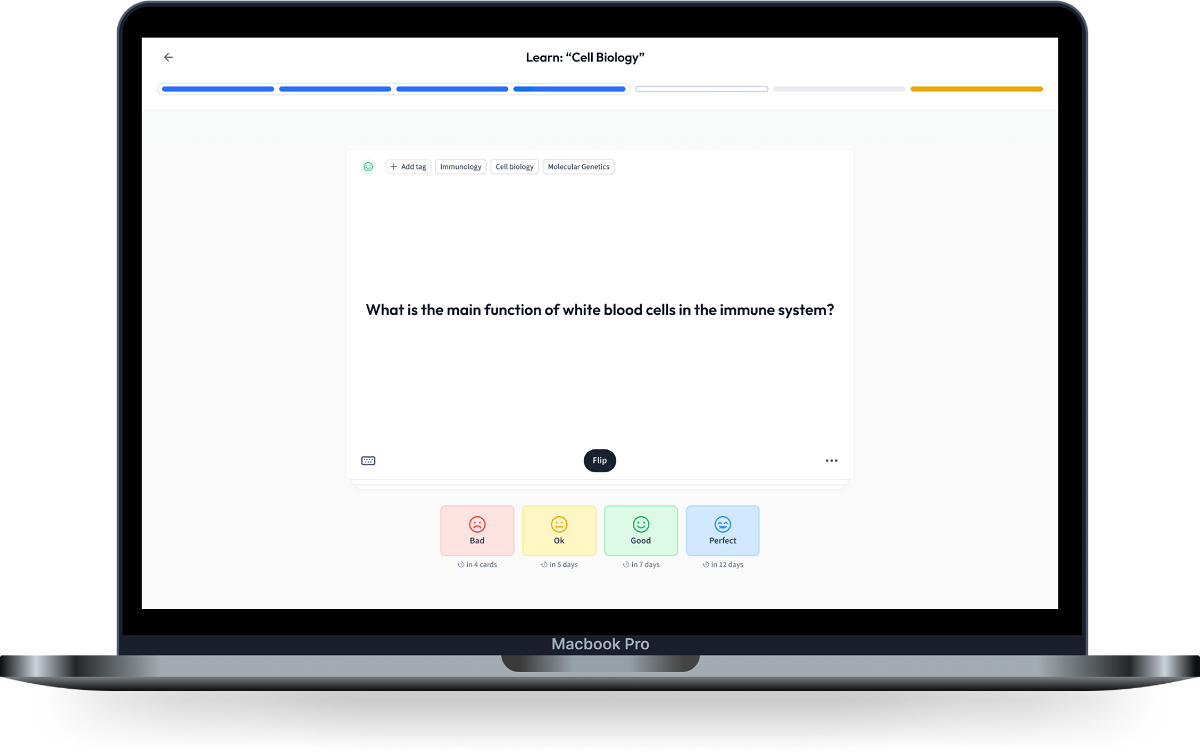
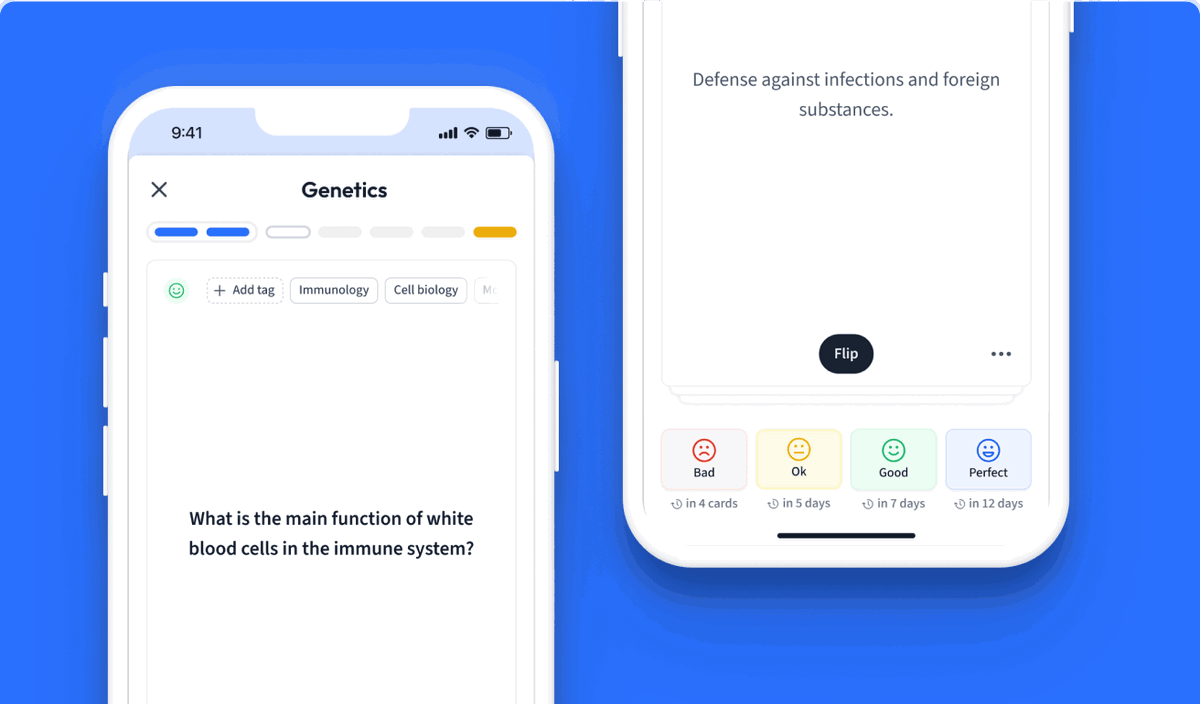
Learn with 15 Java Multiple Catch Blocks flashcards in the free StudySmarter app
Already have an account? Log in
Frequently Asked Questions about Java Multiple Catch Blocks
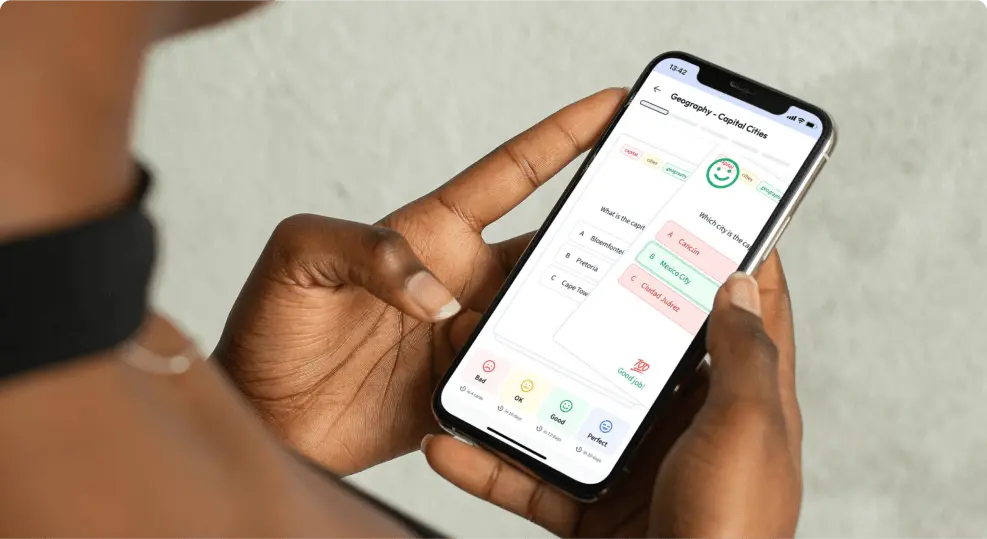
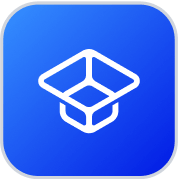
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more