Jump to a key chapter
Unraveling Java Recursion: A Comprehensive Guide
Recursion in Java, like other programming languages, is a concept that students often find a bit tricky to grasp. But worry less! You're about to embark on a journey that will elucidate Java recursion, providing an in-depth understanding of what it is, how to construct recursive methods, practical examples, and delving into recursive binary search.
Unveiling the Intricacies of What is Recursive in Java
In Java, the term 'recursion' refers to a method that calls itself in order to solve a complex problem by breaking it down into smaller, more manageable tasks. Recursion can be very effective when working with data structures like trees and graphs, or for implementing algorithms for tasks like sorting.
Essentially, a recursive function will repeatedly call itself in order to whittle down the problem, until it reaches a condition where it can provide a definitive answer without any further recursion.
Recursive function requirements | Description |
Base condition | This is the condition under which the function will stop calling itself and produce a direct answer. |
Recursive call | This is the function calling itself with some altered parameters, aiming to bring the problem closer to a base case. |
Deconstructing Recursive Method in Java
A recursive method in Java operates under the same concept. It's a method that employs the principle of recursion - it calls itself in the process of execution.
Similar to a recursive function, a recursive method also requires a base case to avoid the possibility of it calling itself infinitely and causing a stack overflow memory issue.
Theoretically, \(N\) number of method calls can be made as long as \(N\) is within the limits of the call stack size. Call stack size can potentially vary between operating systems, but will generally accommodate a significant number of method calls.
Crafting your First Recursive Function in Java
In light of theory, let's deliberate on how you can craft your first recursive function in Java. A common and simple example to start with is calculating the factorial of a number using recursion.
public class Factorial { static int factorial( int n ) { if (n == 1) return 1; else return(n * factorial(n-1)); } public static void main(String[] args) { System.out.println("Factorial of 5 is: " + factorial(5)); } }
In this code, the base case is established as \(n == 1\) where \(n\) is the input number. Beyond this base case, the method calls itself with parameter \(n-1\). This drives \(n\) towards the base case in each recursive call. When \(n == 1\), the function returns \(1\), effectively ending the recursion and producing the final result.
Mastering Examples of Recursion in Java
Java provides a robust platform to implement recursive solutions. The factorial example provides a fundamental grasp on recursion. However, recursion in Java is extensively used in context of data structures and algorithmic problems. To further enhance your understanding, let's inspect several examples.
Fibonacci series, reversing a string, or traversing a binary tree are a few instances where recursion is immensely beneficial.
Diving into the World of Recursive Binary Search Java
If you wish to get your hands dirty with a more complex problem, binary search is a classic example using recursion. Binary Search is an efficient algorithm to find the position of a specific value in a sorted array.
public class BinarySearch { int binarySearch(int arr[], int left, int right, int x) { if (right >= left) { int mid = left + (right - left) / 2; if (arr[mid] == x) return mid; if (arr[mid] > x) return binarySearch(arr, left, mid - 1, x); return binarySearch(arr, mid + 1, right, x); } return -1; } }
The method binarySearch recursively divides the array into halves until it locates the wanted value or concludes that the value is not present. If the selected element is found, the index is returned, otherwise -1 is returned.
Empowering Yourself: Java Recursion Applications
In your journey to master the art of Java programming, gaining proficiency in applying recursion opens up endless possibilities. It's not merely a theoretical concept but a practical tool that can simplify complex problems, increase code efficiency, and is extensively used in computer science problems.
Understanding Practical Examples of Recursion Applications in Java
Empowering your Java coding capabilities, recursion finds its applications in a myriad of problems, be it in accentuating the efficiency of algorithms, manipulating data structures, or programming problems that require repeated or nested actions. Let's delve deeper and scrutinize various applications where recursion plays a key role.
- Factorial operations
- Fibonacci series generation
- Binary Search
- Data structure equivalents like binary trees, graphs, and linked lists
- Sorts - quicksort, mergesort
- Algorithm design - Backtracking, Divide and Conquer, Dynamic Programming
In each of the aforesaid applications, recursion is a handy tool to simplify complicated tasks. In factorial operations and fibonacci series, you use recursion to reduce similar and repetitive calculations. When traversing a binary tree or a graph or processing a linked list, recursion is often the optimal approach. Plus, when dealing with complex algorithms to solve puzzles, recursion can help you break down a tough problem into simpler steps that make more sense.
Real World Scenarios for Recursive Binary Search Java
The Binary Search application is a quintessential illustration of recursion and it's not just reserved for academic exercises. Its true power is harnessed in real-world problems where a key needs to be searched from a sorted list.
In programming situations where an array or list is sorted, and you have to find the presence or position of a certain element, a recursive binary search can do this faster by repeatedly dividing the list into half until the search item is located.
public class BinarySearch { static int binarySearch(int arr[], int x, int low, int high) { if (high >= low) { int mid = low + (high - low) / 2; if (arr[mid] == x) return mid; if (arr[mid] > x) return binarySearch(arr, x, low, mid - 1); return binarySearch(arr, x, mid + 1, high); } return -1; } }
In fact, recursive binary search is often the preferred method as compared to iteration because it doesn't require the additional overhead of handling loop counters and maintaining state across iterations.
Exploring Recursive Function Uses in Computer Programming
Recursion, with its elegant way of reducing complex tasks into simpler ones, is a pivotal concept in computer programming. It's behind the scenes in numerous algorithms and data structures. Here are a few places where the recursive function appears:
Application | Purpose |
Algorithms | Recursive functions are inherent in algorithms such as QuickSort, Merge Sort, Binary Search, etc. They simplify the coding process and increase code readability. |
Tree and Graph Data Structures | In data structures like trees and graphs, recursion is able to simplify operations like insertion, deletion, and searching. |
Dynamic Programming Problems | In dynamic programming, problems are often solved by breaking them down into sub-problems. The function that works on the main problem can also solve the sub-problems using recursion. |
Recursive functions are quite intrinsic in the calculation of Towers of Hanoi, a classic algorithmic problem, which can be solved by breaking it down into simpler sub-problems using recursion.
Remember, no matter what the use, the secret to mastering recursion is understanding the problem well and being able to break it down into simpler, solvable components. The final result is then constructed by combining answers from these smaller problems.
Enhancing Your Skills: Exploring Java Recursion Techniques
Moving forward on your journey to mastering recursion in Java, let's delve into more advanced techniques. An increased understanding of Java recursion techniques will allow you, as a computer scientist, creating more efficient and shorter code that's easier to debug.
Advancing Your Knowledge with Recursive Binary Search Java
One of the key techniques involving recursion in Java is the application of recursive binary search. Unlike linear search, which scans each element in the data structure, binary search exploits the benefit of a sorted data set by recursively dividing it into halves until the desired value is located.
Recursion in binary search is pivotal because it simplifies the problem and makes the code more maintainable. By allowing you to work on smaller subsets of the data, recursive methods can greatly improve the efficiency of your code.
Intuitively, Binary Search works by comparing the middle element of the array with the search key. If the search key matches the middle element, its position in the array is returned. If the search key is less or more than the middle element, the search is repeated in the lower or upper half of the array respectively by making a recursive call until the search key is found or the sub-array reduces to zero.
Binary Search: A Classic Example of Recursion in Java
Solidifying our understanding of the application of recursion in Java, let's work with the classic example of a binary search. Binary search is a technique used to search a sorted data set by recurrently dividing the search interval in half.
public class BinarySearch { int binarySearch(int arr[], int left, int right, int x) { if (right >= left) { int mid = left + (right - left) / 2; if (arr[mid] == x) return mid; if (arr[mid] > x) return binarySearch(arr, left, mid - 1, x); return binarySearch(arr, mid + 1, right, x); } return -1; } }
In the above example, the binarySearch function accepts a sorted array, search key \(x\), and two indices \(left\) and \(right\) representing the current interval being searched. If the search key is equal to the element at the mid-position, it returns the mid-position else the function calls itself after adjusting the search interval based on whether the search key is less or more than the middle element. This is an apt demonstration of how recursion can simplify a problem, thereby producing concise and maintainable code.
Advanced Usage of Recursive Method in Java
Recursion in Java is not just constricted to algorithms or searching and sorting techniques. You can expand its use beyond basic exercises to more complex simulations and intricate structures otherwise difficult to handle through iterative methods. These recursive methods offer a more streamlined, elegant solution in accomplishing the tasks that require repeated or nested actions.
There is no question that recursion can be an invaluable tool when used correctly. Understanding the advanced usage of recursive methods can significantly heighten your programming skills and improve the quality of your code.
Getting Creative: Building Complex Recursive Functions in Java
Inspiring your creativity in Java recursive functions, consider the creation of more complex examples that reinforce and expand your knowledge base. For instance, consider investigating the calculation of mathematical series, such as the Fibonacci series or the Taylor series, which otherwise require complex iterative logic.
public class Fibonacci { public static int fib(int n) { if (n <= 1) return n; else return fib(n - 1) + fib(n - 2); } public static void main(String[] args) { System.out.println("Fibonacci series at index 8 is: " + fib(8)); } }
In the Fibonacci example above, each number in the series is the sum of the two preceding ones and the series starts from \(0\) and \(1\). The recursive function fib operates by taking an index \(n\), it roughly splits the problem into two smaller problems, symbiotically expressing the nature of the Fibonacci series leading to a cleaner and readable implementation.
However, with power comes responsibility. Recursive methods, especially for larger inputs or intricate problems, if used without consideration, can lead to performance issues like stack overflow error or algorithmic complexities. Therefore, always understand the recursive behaviour and monitor your stack levels while deciding recursion as the go-to approach.
Java Recursion - Key takeaways
- Java Recursion is a concept where a method in Java calls itself to solve a problem, breaking it down into smaller, more manageable tasks.
- A recursive function in Java repeatedly calls itself to address a problem until it reaches a base condition - the condition under which the function will stop calling itself and produce a direct answer. This function uses a recursive call, which is the function calling itself with altered parameters, to bring the problem closer to a base case.
- Recursive methods in Java operate under the same concept and also require a base case to avoid calling itself endlessly, which could cause a stack overflow memory issue.
- Examples of recursion in Java include calculating the factorial of a number and implementing a binary search. The binary search algorithm uses recursion to divide an array into halves until it locates a specific value or concludes that the value is not present.
- Applications of recursion in Java range from enhancing the efficiency of algorithms, manipulating data structures, performing complex sorting operations like quicksort and mergesort, and aiding in the design of specific algorithms like in backtracking, divide and conquer strategies, and dynamic programming.
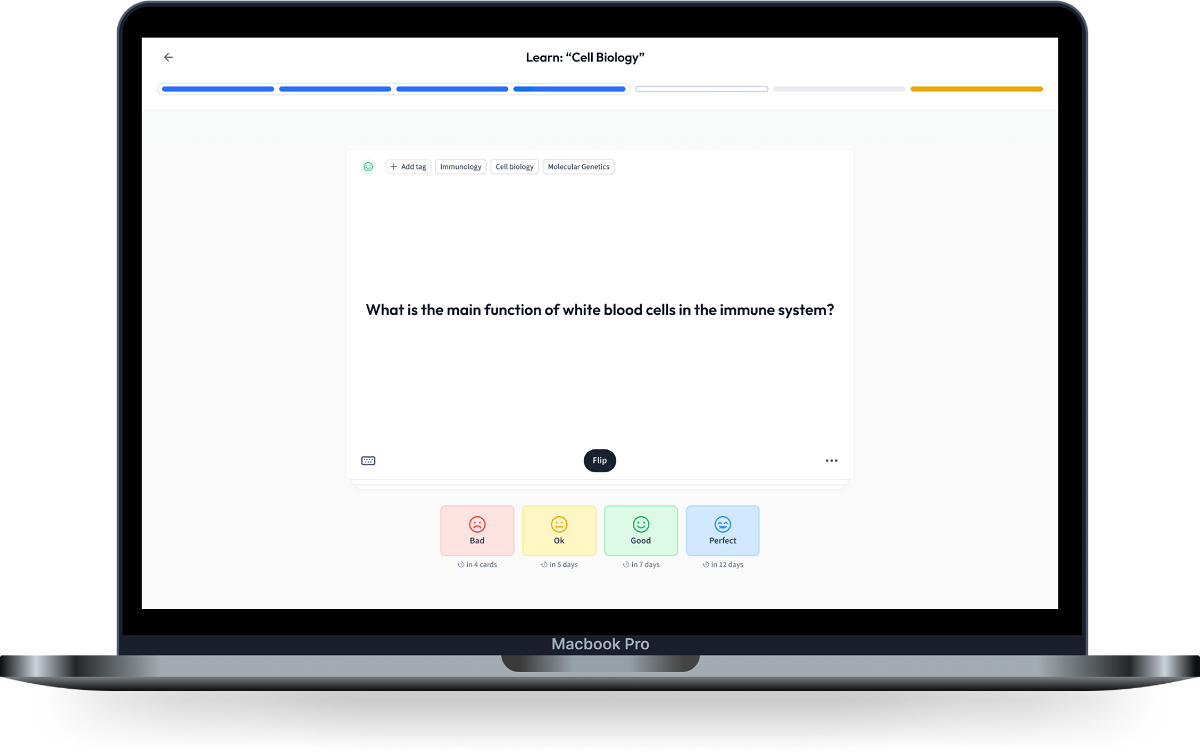
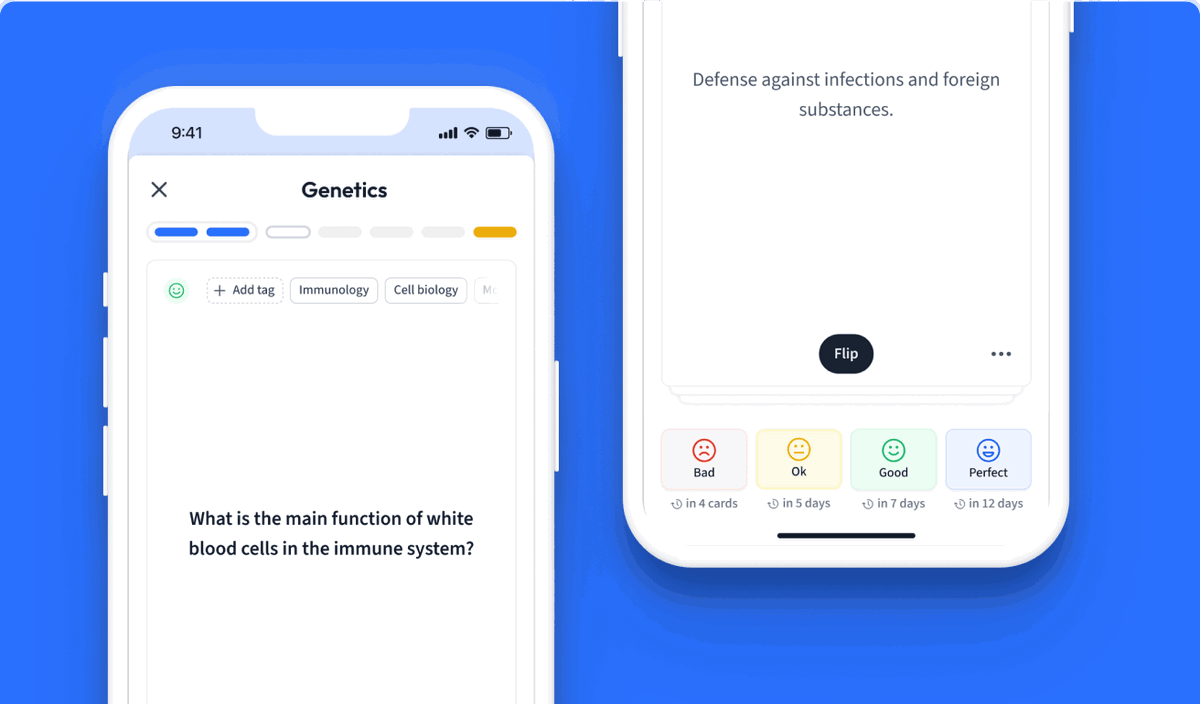
Learn with 12 Java Recursion flashcards in the free StudySmarter app
Already have an account? Log in
Frequently Asked Questions about Java Recursion
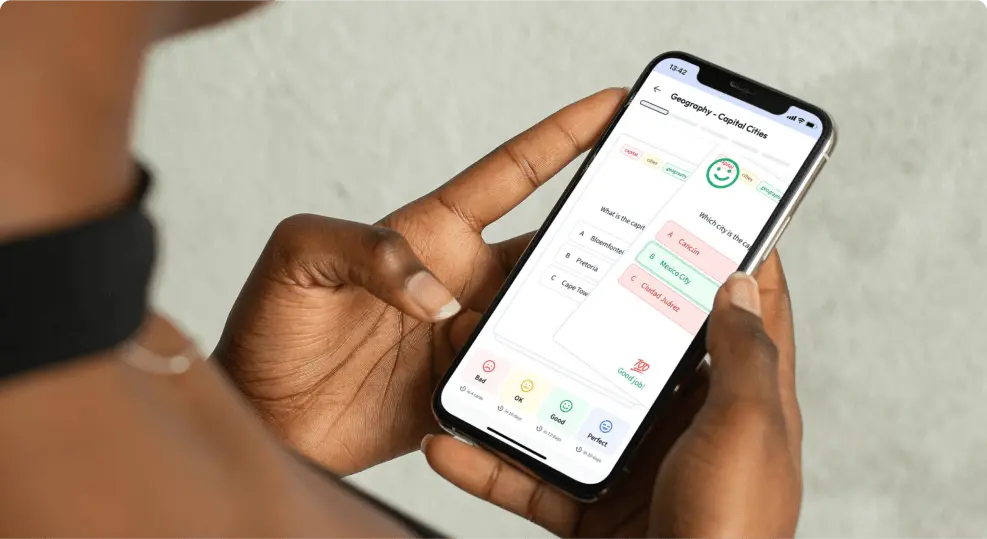
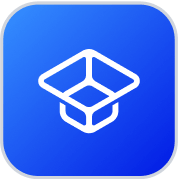
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more