Jump to a key chapter
Understanding Java Static Keywords
Java Static Keywords form an integral part of the Java programming language. They hold the key to understanding how certain aspects of the language function.
Definition: What is the static keyword in Java?
In Java, the static keyword is used mainly for memory management. It is a non-access modifier that, when applied to a method or field, creates a single copy of that variable or method for the entire class, as opposed to each individual instance of the class.
For instance, consider a scenario where you have a class 'BankAccount' and the attribute 'bankName' as part of this class. If you create multiple bank accounts, keeping 'bankName' as a static field ensures that there's only one 'bankName' field for all bank accounts, saving memory space.
public class BankAccount { static String bankName; }
Decoding Static Keyword in Java Programming
The function of Java Static Keywords can be divided into four major categories:
It's important to note that Static methods cannot reference non-static variables or methods as they belong to the instance of the class.
Type | Description |
Static Variables | Belong to the class, not instance. |
Static Methods | Can be called without class instantiation. |
Static Blocks | Used to initialise static variables. |
Static Classes | Nested classes that are static. |
The role and use of Java Static Keywords in Computer Programming
The Java Static Keywords serve to simplify many facets of computer programming.
For example, static variables can help eliminate the need for additional code to manage memory allocation and storage, potentially reducing the risk of memory leaks and enhancing performance.
It's crucial to judiciously use Java Static Keywords. Overuse can lead to issues related to memory management and debugging, as static fields exist for the entire runtime of the program.
Practical Examples of Java Static Keywords
In the world of Java programming, Static Keywords play a quintessential role. Let's delve into practical examples and applications to gain a profound understanding of their functionality.
Can we use static keyword with class in Java?
Yes, you can use the static keyword with a class in Java, but only for a nested or inner class. A static nested class is one which is directly within another class but marked with the keyword 'static'. This type of class is different from regular nested classes in a couple of key ways:
- Independence: A static nested class doesn't require an instance of the enclosing class. It can be created and accessed independently.
- Access to class variables: A static nested class can access only the static members of its outer class, not the non-static ones.
Here is a Java code snippet that illustrates a static nested class:
public class OuterClass { static int staticVariable = 10; static class StaticNestedClass { void display() { System.out.println("Static Variable: " + staticVariable); } } }
Here, the static nested class 'StaticNestedClass' is accessing the static variable of the outer class 'OuterClass'.
Effective Examples of static keyword in Java
The static keyword in Java is used with variables, methods, blocks and nested classes. Let's see each of them in action:
1. Static Variables:
class Test { static int count = 0; // constructor Test() { count++; } }
In this example, the variable 'count' is static and gets incremented each time an object of the class is created.
2. Static Methods:
class Utility { static int multiply(int a, int b) { return a * b; } }
In this case, the 'multiply' method is static and can be called without creating an instance of the 'Utility' class.
3. Static Blocks: They are used for static initialisation of a class.
class Test { static int num; static String str; // static block static { num = 68; str = "Static keyword in Java"; } }
Here, the static block initialises 'num' and 'str' before they are used in the program.
Step-by-step guide: How to use static keyword in Java
Follow the steps below to effectively use the static keyword in Java:
1. Declare static variables: Static variables are declared using the static keyword before the data type of the variable.
static int count;
The above declaration signifies 'count' is a static variable.
2. Define static methods: Static methods, similar to static variables, use the static keyword. In this case, it's put before the return type of the method.
static String greet() { return "Hello, static keyword!"; }
This creates a static method 'greet' which can be called without creating an instance of the class.
3. Create a static block: Static blocks help in initialising the static data members, just like constructors help in the initialisation of instance data members.
static { // Static block }
When creating this block, remember it will run only once when the class is loaded into memory.
4. Make a static class: Finally, you can create a nested static class using the static keyword.
class OuterClass { static class InnerClass { // Inner class code } }
This creates a nested static class which can be accessed independently of the outer class.
The Importance of Java Static Keywords
The importance of Java Static Keywords in computer programming and Java development, in particular, lies in its key role in memory management and code organisation. Static Keywords immensely boost performance by reducing the need for duplication of variables and functions.
Analyzing the Benefits: Importance of static keyword in Java
Java Static Keywords provide programmers with several benefits that make coding in Java more efficient, manageable and optimised in many ways. Let's delve into the specifics:
- Memory Efficiency: Static variables or fields are class-level variables that are shared between all instances of the class. This means that the static variable only has one piece of memory allocated, no matter how many instances of the class you create, making it more memory efficient.
- Utility Methods: Static methods in Java often serve as utility methods. They contain functionality related to the class as a whole, not to a specific instance of the class.
- Less Overhead: With static methods, there is no need for a class instance. This means less overhead, as you can call the method without instantiating the class.
- Independent Nested Classes: Static classes also have their niche uses, particularly when defining nested classes that are meant to be used independently of the outer class.
It's crucial to keep in mind: While static keyword provides these benefits, they should be used judiciously. Overuse or inappropriate use of static members can lead to issues related to memory management and debugging, as well as a possibility of overcomplicating the code structure.
Exploring why Java Static Keywords are crucial in Computer Programming
Java is an object-oriented programming language, which means that it organises the program around objects rather than actions. However, not everything needs to be part of an object, and that's where the static keyword fits brilliantly. Here's a little more depth on its crucial role:
- Global Access: Static members, be it a method, variable, or a class, are globally accessible and can be used without creating an object of the class.
- Constant Usage: Static keyword helps in creating class-level constants. In Java, we often use 'public static final' modifiers to create constants.
- Singleton Classes: The concept of Singleton classes in Java, which allows only a single instance of a class to be created, is made possible with the help of static keyword. The object creation process is controlled by a static method.
Let's look at implementing a Singleton class:
public class Singleton { private static Singleton single_instance = null; private Singleton() {} // private constructor public static Singleton getInstance() { if (single_instance == null) single_instance = new Singleton(); return single_instance; } }
This exemplifies the usage of static keyword in Singleton pattern. You can see here that it is the static method 'getInstance' that controls object creation.
The Significant Role of Java Static Keywords
It's safe to say that the static keyword in Java holds significant importance. Through various aspects mentioned above, it's clear how indispensable they are.
However, it's not just about the benefits at the code level. The importance of static keywords also extends to the impact on the final software product:
- Performance Improvement: By improving memory efficiency and reducing overhead, static keyword can contribute to increased overall performance of the software.
- Improved Code Readability: Static methods can increase readability by clearly defining their independent nature. Similar is the case with static nested classes which can be treated as top-level classes, hence, improving code organisation and readability.
- Ease of Use: One of the benefits of static variables and methods is that they can be accessed directly using the class name, without needing to create an object of the class. This makes them easier to use in certain situations.
Big picture: You're more likely to use static variables for constants, static methods for utilities, and static classes for independent nested classes. It's important to remember that while static can increase efficiency, judicious use is a must.
Exploring Static Final Keyword in Java
Peeling the layers of Java programming deeper, let's traverse to a more mature concept: the Static Final Keyword in Java. This unique keyword mixture allows us to define constants in a way that their value never changes and their definition remains static throughout the application.
Concept and Application: Static Final Keyword in Java
The combination of static and final keywords in Java targets the creation of constants, i.e., variables that cannot be changed once they have been assigned. These constants are usually declared as a combination of static, final, and usually set to public so that they can be accessed anywhere. By making them static, we ensure that they belong to the class itself, rather than to any instances of the class, thus leading to memory improvements.
public class Constants { public static final int MAX_SIZE = 100; }
This is an example of defining a constant, MAX_SIZE, in Java, which can be accessed directly with the class name, like so: Constants.MAX_SIZE.
Let's decipher Static Final Keyword in more detail:
- Static: This keyword, when applied to a member, means that it belongs to the type itself, rather than to any instance of the type. Hence, the static member is not tied to any particular object instance of that class.
- Final: Final variables have the feature that once they receive a value, it cannot be changed. Final fields are often used for values which never change, like the mathematical constant \( \pi \) (Pi), and are almost always declared as static.
Consider the example of representing mathematical constant \( \pi \) as a static final variable:
public class Constants { public static final double PI = 3.141592653589793; }
The Influence of Static Final Keyword in Java Programming
The static final keyword has its vast influence in Java programming, especially when creating constants and defining immutable values. Let's discuss how they leave their mark:
- Global Constants: Declare static final variables when you need values that are accessible across multiple classes and whose value remains constant throughout the application's entire life cycle. Using the static final keyword combination keeps these values free from accidental changes.
- Immutable Class Entities: In Java, make class entities immutable by declaring them as static final. It enforces the constant properties of your instances. This is often used when creating utility classes, like 'Math' which holds constants like PI and methods that don't need to be changed.
- Fixed External Settings: In software applications, sometimes we have settings defined which are external and should not change once loaded. These can be represented as static final constants, ensuring their values remain constant during application execution.
An illustration of 'Math' utility class where we use static final for constants:
public final class Math { public static final double PI = 3.141592653589793; public static final double E = 2.718281828459045; }
Detailed Study: Static Final Keyword in Java
Diving deeper into Java's static final keyword, we can evaluate its pros and cons:
Pros | Cons |
It improves performance by saving memory. | Incorrect use can lead to restrictive access and modify operations. |
Prevents accidental modification of constants. | Constants declared in inner classes may cause issues during inheritance. |
Standard way of declaring constants in Java. | Requires correct understanding and usage to avoid potential issues. |
While the static final keyword is the most common and straightforward way to declare constants in Java, remember to declare these constants based on their intended scope. If a constant should be available across multiple classes, declare it in a centralised area (like a settings or a constants class) as public static final. If it's needed only within a single class, limit it to private or package-private visibility, to maintain encapsulation.
Advanced Utilisation of Java Static Keywords
Propelling into the advanced realm of Java Static Keywords, one mustn't ignore the nuances and intricacies that significantly impact their application. Supervising the code's behaviour, they remain a dominant force in creating optimised, readable, and reusable Java code.
Elevated usage: Java Static Keywords and their supremacy
You may view the usage of static keywords as a means of defining select aspects of your code. However, static keywords are much more than that—they take a more assertive stance, ensuring that aspects of your code have uniform behaviour across the board and can be thought of as supervisors controlling the minutiae of your program.
For instance, let's contemplate why you might want to use a static function. To begin with, you must understand that static methods have direct access to static variables and can change their states. This property allows static methods to interact with static class variables without creating an instance of the class, making them gravely efficient.
public class MyClass { static int count = 0; static void incrementCount() { count++; } }
In the above code snippet, the static method `incrementCount` can directly manipulate the static variable `count` due to its accessibility.
Furthermore, moving onto static blocks, you'll unearth another aspect of control that static keywords exert. Static blocks, also known as static initialisers, are used to organise or initialise static fields. They get executed once when the class gets loaded into memory, which proves quite advantageous when initialising complex static variables or capturing exception handling around their initialisation.
public class MyClass { static int[] values = new int[10]; static { for(int i=0; iThis class-initialiser block of code is setting up the static `values` array elements with their index values, an operation that happens automatically when the class is loaded into the JVM.
Java Static Keywords: Going beyond the basics
Static nested classes—a datum of the Java Static Keyword's pantheon—are another superb that lies beyond the rudimentary utilisation of static keyword. These are nested classes declared as static and are still part of their outer class. Unlike inner classes, which have access to instance variables of the outer class, static nested classes do not. They exist independently of the instance of an outer class and interact with the outer class through objects only.
public class OuterClass { static int staticVar = 0; int nonStaticVar = 0; static class StaticNestedClass { void displayValues() { System.out.println("Static Variable: " + staticVar); // Can't access non-static variable here! } } }In the above example, the `StaticNestedClass` can directly access and print the static variable `staticVar` of `OuterClass`. However, it cannot access `nonStaticVar` because it's not static, hence illustrating the autonomous nature of static nested classes.
Diving into the depth of the static keyword in Java
Since we've dove into the advanced usage of Java static keywords, let's focus on some of the limitations and constraints around static keyword usage - particularly around why they cannot be used with attributes of classes or methods. This misunderstanding could lead to logical errors and bugs.
Let's first address class-level attributes. A common mistake is to try to apply the static keyword to class level properties, particularly with interfaces. However, this might not work as expected and compile-time errors could be thrown because interfaces in Java are implicitly abstract, and adding static to interface properties would violate the abstraction principle. Moreover, static steps up a level of hierarchy and belongs to a class rather than an instance, so applying it to class-level properties leads to redundancy.
Now, wading into the realm of methods, recall that static methods cannot refer to instance variables or instance methods directly and cannot use the keyword `this`. Why so? Because they are not attached to an object and hence don’t have a `this` reference. This becomes very important when designing applications, as the constraints around `this` make it impossible for static methods to call non-static methods or access non-static fields.
public class MyClass { int nonStaticVar = 0; static void staticMethod() { // Can't access nonStaticVar directly // System.out.println(nonStaticVar); -> Will result in an error } }To access `nonStaticVar` in `staticMethod`, an object of `MyClass` would be needed:
public class MyClass { int nonStaticVar = 0; static void staticMethod() { MyClass mc = new MyClass(); System.out.println(mc.nonStaticVar); } }So, bear in mind that the static keyword holds great power in Java. However, it must be used with great caution and understanding to steer clear of the known pitfalls.
Java Static Keywords - Key takeaways
- Java Static Keywords: They have a significant role in memory management and code organisation. These keywords reduce the need for duplication of variables and functions, thus improving performance.
- Use of Static Keyword in Java: The static keyword can be used with variables, methods, nested classes, and blocks. For variables, it means the variable gets shared between all instances of the class; for methods, they can be called without an instance of the class; for nested classes and blocks, they can be accessed independently and help in static initialization of a class.
- Constant Usage: The static keyword helps in creating class-level constants. In Java, "public static final" modifiers are often used to create constants.
- Static Final Keyword in Java: This unique keyword combination allows the definition of constants in a way that their value never changes and their definition remains static throughout the application. They can be accessed directly with the class name without needing to create an object of the class.
- Advanced Usage of Java Static Keywords: Static keywords ensure uniform behaviour across the code. Static methods have direct access to static variables and can change their states. They can interact with static class variables without creating an instance of the class, making them efficient.
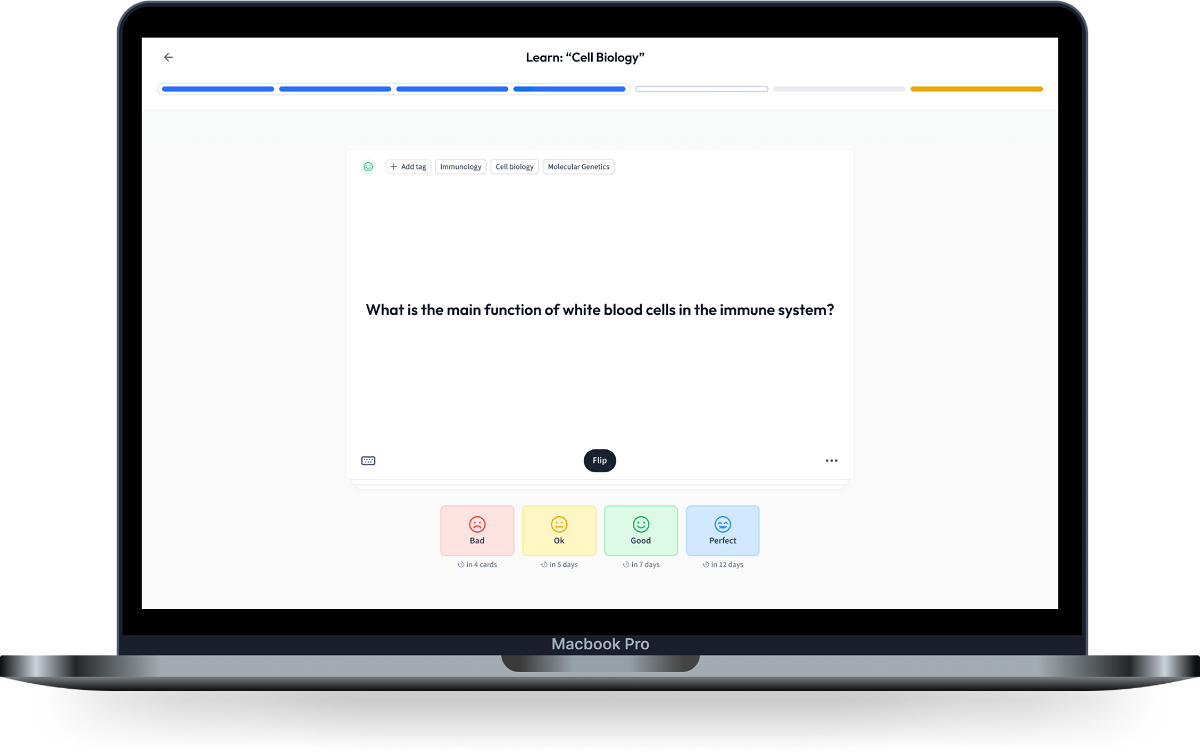
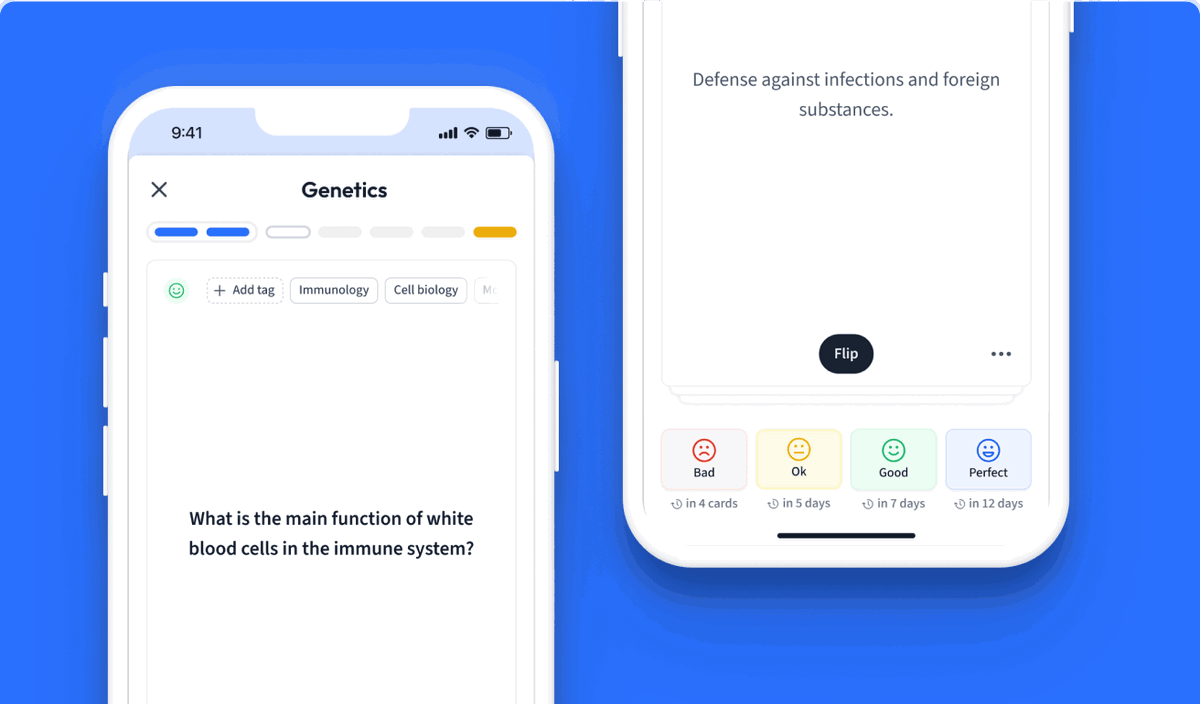
Learn with 15 Java Static Keywords flashcards in the free StudySmarter app
Already have an account? Log in
Frequently Asked Questions about Java Static Keywords
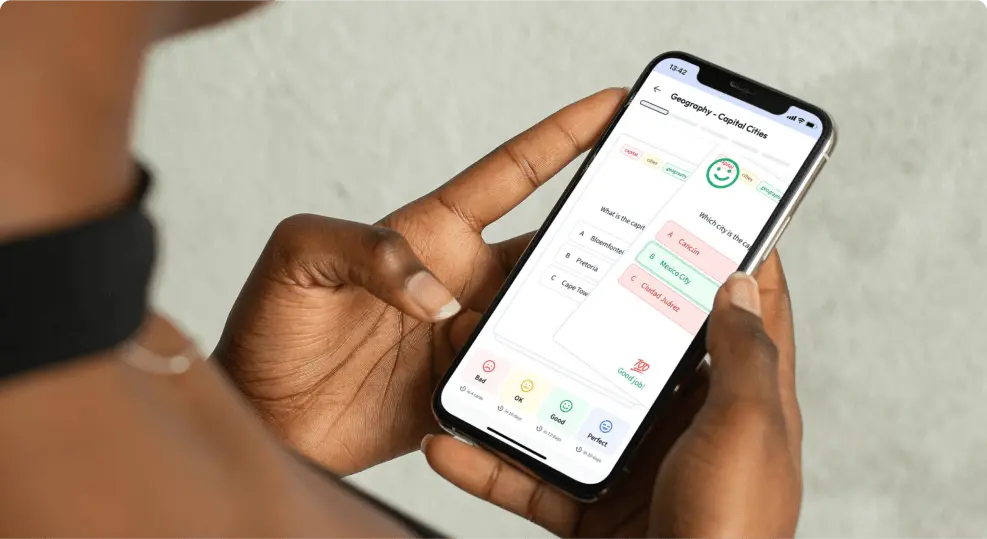
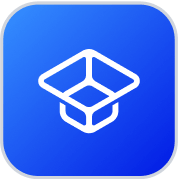
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more