Jump to a key chapter
Definition of Java Type Casting
Java Type Casting is a method used in Java to transform one data type into another. This transformation enables seamless and efficient interaction between distinct data types in Java programming.
Explanation of Type Casting in Java
When working with Java, you might come across different data types. Each data type has its own range and characteristics which make it unique. Type casting is the process of converting data from one type to another. This can be necessary when, for instance, a method specifically requires a parameter of a particular type, or when a more flexible coding approach is desired. There are two primary types of type casting in Java:
- Implicit Casting (Widening Conversion): This occurs automatically when a smaller data type is converted to a larger data type, for example from an
int
to adouble
. - Explicit Casting (Narrowing Conversion): This requires explicit data conversion where a larger data type is converted into a smaller one using the cast operator. An example of this is converting a
double
to anint
. This is usually accompanied by the risk of data loss.
Here is an example of both implicit and explicit casting in Java:
int myInt = 9;double myDouble = myInt; // Automatic casting: int to doubleSystem.out.println(myInt); // Outputs 9System.out.println(myDouble); // Outputs 9.0// Explicit castingdouble myDouble2 = 9.78;int myInt2 = (int) myDouble2; // Manual casting: double to intSystem.out.println(myDouble2); // Outputs 9.78System.out.println(myInt2); // Outputs 9
Remember, narrowing conversions in Java might lead to data loss, so handle them with care.
Importance of Data Type Casting in Java
Data Type Casting is a crucial component in Java for enhancing the versatility and functionality of your code. By ensuring that variables can be adjusted to appropriate types when necessary, it ensures efficient memory utilization and precision in data representation. Data type casting supports:
- Enhanced Compatibility: Allows integration of operations involving different data types without raising type mismatch errors.
- Memory Management: By converting to a more suitable data type, memory allocation can be optimized, thus improving system efficiency.
- Code Reusability: Methods and functions become more flexible when they can cater to different data types through casting.
In complex Java programs, generics can be leveraged alongside type casting to create even more robust and reusable code. Generics enable type-safe code without the need to know the specific class types at compile time, and they often work hand in hand with casting to ensure that collections and other data structures can manage different data types effectively. This is particularly useful in data structures like ArrayList
or HashMap
, where explicit type definitions may not always be possible. Consider the effect of generics in reducing common runtime errors and increasing the safety of your Java applications.
Java Type Conversion and Casting
In programming with Java, understanding how to efficiently manage and manipulate data types is crucial. Two common processes that facilitate this are type conversion and type casting, each of which serves distinct purposes in ensuring compatibility and efficiency within Java applications. Grasping these concepts helps you make informed decisions about data handling in your code.
Difference Between Type Conversion and Type Casting in Java
While type conversion and type casting may seem similar, they have key differences that you need to understand:
- Type Conversion: This is mainly a process managed by Java itself. Such conversions usually occur automatically. Java handles implicit type conversion or widening conversion when a value of a smaller data type is assigned to a larger type, such as an
int
to along
without any explicit instructions. - Type Casting: It is defined by explicit instructions in your code. When a developer needs to cast a type explicitly, they use narrowing conversion, which happens when converting a larger data type to a smaller one, like a
double
to anint
. This may involve a potential loss in precision, so it's essential to code carefully.
Let's consider an example in Java code distinguishing between type conversion and type casting: Automatic type conversion:
byte myByte = 100;int myInt = myByte; // Automatic conversion from byte to intSystem.out.println(myInt); // Outputs 100Explicit type casting:
double myDouble = 9.78;int myInt = (int) myDouble; // Explicit casting from double to intSystem.out.println(myInt); // Outputs 9
Be cautious when using type casting, as it might lead to data truncation or rounding errors.
Java Type Casting Technique
To effectively utilize Java Type Casting, you'll often follow specific techniques designed to ensure stability and accuracy in your programs. Here are some critical steps and considerations to keep in mind for type casting in Java:
- When performing explicit casting, use parentheses to clarify the data type transformation, such as
(int)
. This serves an essential role in ensuring intent is clear both for you and anyone else reading the code. - Monitor data precision: When casting from floating-point numbers to integers, understand that the fractional part will be discarded. Sometimes, rounding might be necessary to achieve intended results.
- Test extensively: Thoroughly test code sections involving casting to detect potential issues correlated to data precision and integrity.
A deeper dive into real-world applications of casting in Java reveals fascinating insights, particularly in game development and graphics rendering. In these environments, controlling and converting between types ensures optimized memory usage and enhanced performance. By transforming data types, developers manage the vast array of data inputs and outputs seamlessly, adapting the calculation precision according to the needs of the rendering engine. Additionally, casting plays a significant role in serializing and deserializing data for network communication, contributing to efficient data transmission mechanisms. As you progress in your Java programming journey, weighing the advantages and potential drawbacks of casting allows you to develop more robust and performant applications.
Data Type Casting in Java: Practical Applications
In Java programming, data type casting is a powerful technique that plays a significant role in various applications. By converting a variable from one type to another, type casting allows for smoother operations and effective memory management within your code. From enhancing compatibility to enabling complex calculations, type casting proves to be indispensable in practical scenarios.
Real-World Examples of Java Type Casting
Java type casting finds its application in numerous real-world contexts, ensuring efficient data handling. Here's how it is applied in various scenarios:
- Financial Calculations: Casting is commonly used in financial computations where data accuracy is pivotal. Converting from
double
toint
is often necessary when handling currency conversions or calculating tax to ensure precision and efficiency. - Game Development: In gaming, precise data representation is key to rendering graphics correctly. Type casting enables developers to handle large numerical data and convert it to required types for smoother game performance.
- Data Processing: In data handling applications, such as converting CSV data to database formats, type casting ensures that data types match expected formats, which prevents errors during data manipulation.
Consider a situation in a financial software where precise calculation of tax is needed from floating-point operations. Here's how you might apply casting:
double taxRate = 4.75;double income = 5000.75;int totalTax = (int) (income * taxRate / 100); // Casting double to int for precisionSystem.out.println(totalTax); // Outputs 237This example demonstrates explicit casting to handle financial calculations accurately.
Expanding beyond basic applications, Java type casting becomes essential in advanced computational algorithms. In fields such as machine learning and artificial intelligence, where colossal volumes of data are processed, casting ensures that algorithms function optimally by converting data types as required for different calculations. Leveraging casting, developers can fine-tune algorithms by ensuring type compatibility across different models and libraries. This multidimensional application highlights the utility of type casting in cutting-edge technology solutions.
Java Type Casting Technique in Action
Harnessing Java type casting techniques allows you to refine your programming skills by integrating versatility into your applications. Key techniques include:
- Utilizing Explicit Casting: With explicit casting, you intentionally convert between types using a cast operator. For instance, converting a
double
into anint
ensures you've foreseen potential data loss. - Applying Implicit Casting: This occurs automatically in widening conversions, where smaller types like
byte
are effortlessly converted into larger ones such asint
. - Implementing Error Checking: Given the potential pitfalls of type casting, incorporate error management to prevent data truncation or unexpected behavior.
Here's a practical illustration of both implicit and explicit casting that displays type compatibility in Java:
byte smallNumber = 100;int largeNumber = smallNumber; // Implicit casting: byte to intSystem.out.println(largeNumber); // Outputs 100double bigDecimal = 23.99;int receivedValue = (int) bigDecimal; // Explicit casting: double to intSystem.out.println(receivedValue); // Outputs 23This showcases the seamless interaction of different types thanks to casting.
Understanding the data type ranges in Java is beneficial to prevent issues arising from casting operations, especially when seeking to maintain data integrity.
Type Casting Exercises in Java
Type casting is an essential concept in Java that allows you to convert data types. Practicing type casting exercises can help reinforce your understanding and improve your coding skills.
Practice Problems for Type Casting in Java
Below are some practice problems to help you understand and apply Java type casting: 1. Problem 1: Convert an integer value to a double and then back to an integer to understand implicit and explicit casting. 2. Problem 2: Create a program that takes a user's age as a string and converts it to an integer for arithmetic operations. 3. Problem 3: Challenge yourself by creating a method that can convert a float
into a short
and handle potential data loss. Working through such exercises will enhance your ability to utilize data types effectively in your Java programs.
Example solutions for these problems will further illustrate how to apply type casting effectively. Let's see how you can tackle these practice problems:
// Problem 1int integerNum = 100;double doubleNum = integerNum; // Implicit castingSystem.out.println(doubleNum); // Should output 100.0int newIntegerNum = (int) doubleNum; // Explicit castingSystem.out.println(newIntegerNum); // Should output 100
Let's delve deeper into the nuances of type casting practice. One advanced technique involves generics and interoperability among different data types. By practicing with generics, you can write more cohesive and flexible Java code. Generics allow you to specify a single method that can be expanded upon a range of data types, reducing the need for specific casts while minimizing runtime errors. Consider a generic method for data processing, where casting occurs seamlessly behind the scenes, thus optimizing your code's efficiency.
Solutions and Approaches to Java Type Casting Exercises
Here are some suggested solutions and approaches for the type casting exercises mentioned:
- For Problem 1: Recognize the role of both implicit and explicit casting in converting data types without losing data integrity.
- For Problem 2: Use the
Integer.parseInt()
method or similar functions to comfortably convertString
types intoint
, considering error handling for non-numeric inputs. - For Problem 3: Consider building error-checking mechanisms to alleviate data loss when type casting from
float
toshort
.
When dealing with string-to-number conversions, always validate the string to prevent runtime exceptions, especially for unexpected inputs.
Java Type Casting - Key takeaways
- Java Type Casting: A technique in Java for transforming one data type into another to facilitate efficient data interaction.
- Implicit vs. Explicit Casting: Implicit casting occurs automatically, such as converting an
int
to adouble
, whereas explicit casting requires specific code instructions, converting adouble
to anint
. - Java Type Conversion and Casting: Type conversion happens automatically in Java (widening conversion), while type casting (narrowing conversion) is explicit and requires developer intervention.
- Importance: Data type casting in Java enhances compatibility, memory management, and code reusability by enabling flexible data type handling.
- Real-World Applications: Financial calculations, game development, and data processing benefit from type casting for precision and compatibility.
- Type Casting Exercises in Java: Practical exercises help reinforce understanding of Java type casting by experimenting with implicit and explicit conversions.
Learn faster with the 24 flashcards about Java Type Casting
Sign up for free to gain access to all our flashcards.
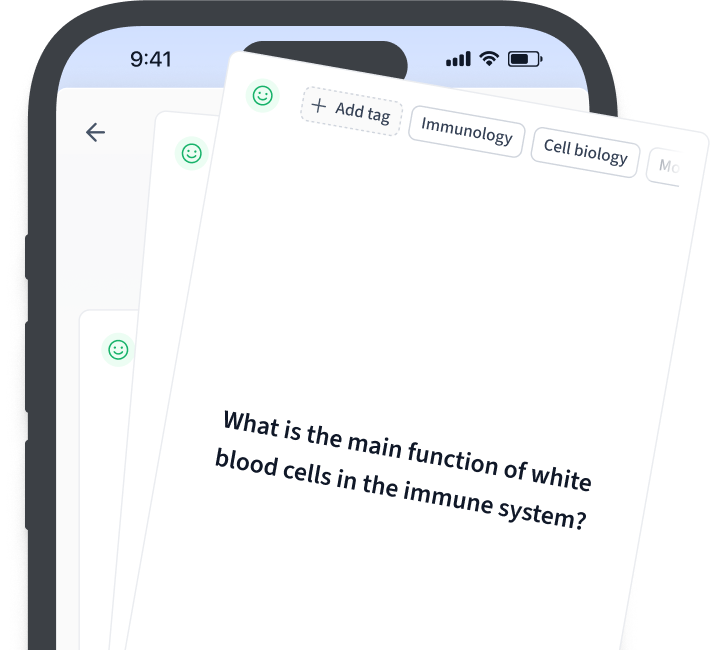
Frequently Asked Questions about Java Type Casting
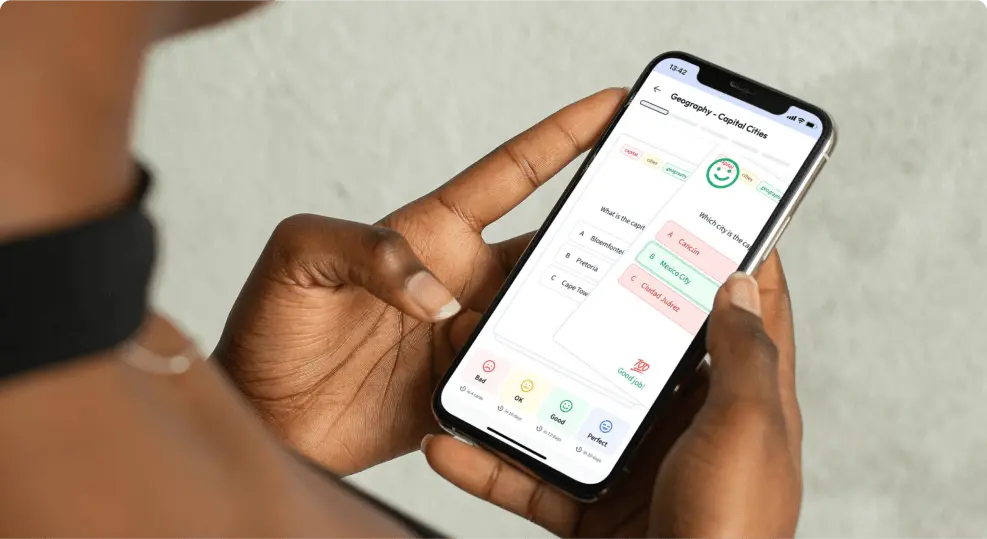
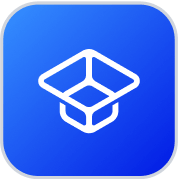
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more