Jump to a key chapter
Javascript Function Expressions Definition
Javascript Function Expressions are fundamental tools in modern programming that allow you to define a function inline and assign it to a variable. This approach gives you the flexibility to create functions at runtime, making your code more dynamic and adaptable. Function expressions are often used when the exact function logic is determined by conditions or input data that isn't available until runtime.
What is a Function Expression in Javascript?
A Function Expression in Javascript is simply a way to define a function inline using an expression, where the function itself is assigned to a variable, object property, or array element. Unlike a function declaration, a function expression is not hoisted to the top which means it cannot be called before it is defined in the code.
In the context of Javascript, a function expression is an expression that defines a function and can be stored in a variable, passed as arguments, or used as closures.
// Function Expression stored in a variabletheSquare = function(x) { return x * x;};// This function can be called using the variable nametheSquare(8); // Returns 64
Since function expressions are not hoisted, you must define them before you call them.
Understanding Javascript Function Expressions
To understand Javascript Function Expressions, it's essential to differentiate them from function declarations. Here are some key points to note:
- Syntax: While a function declaration uses the 'function' keyword followed by a name, a function expression can be either named or anonymous.
- Usage: Function expressions allow for the creation of functions within other code blocks or conditions, enabling more compact and sometimes cleaner code structures.
- Anonymity: A function expression does not require a name, which means it can be an anonymous function. This can be useful for functions that are used only once or are passed as arguments to other functions.
Function Declaration vs Function Expression in Javascript
In Javascript, the way you define functions can affect the behavior and usage of your code. The two main approaches are function declarations and function expressions. Understanding the differences and choosing the appropriate method is crucial for writing effective and efficient code.
Differences Between Javascript Function Declarations and Expressions
Both function declarations and expressions serve the purpose of defining functions, but they have some significant differences:
- Hoisting: Function declarations are hoisted to the top of their containing scope, which means they are available before reaching their declaration in the code. Conversely, function expressions are not hoisted, and you must define them before use.
- Syntax: A function declaration looks like this:
function example() { // code}
While a function expression might look like this:let example = function() { // code};
- Naming: Function expressions can be anonymous or named, while function declarations always have a name.
- Immediate Invocation: Function expressions are often used with Immediately Invoked Function Expressions (IIFE), allowing you to execute the function as soon as it is defined.
// Function Declarationfunction greet() { console.log('Hello!');}greet();// Function Expressionlet greet = function() { console.log('Hello!');}greet();
Choose a function declaration if you need a simple, always-available function. Use function expressions for more controlled scopes and lifespan.
Choosing Between Function Declaration and Expression in Javascript
Selecting between function declarations and function expressions depends on the specific needs of your code. Consider the following aspects:
Aspect | Function Declaration | Function Expression |
Hoisting | Yes | No |
Use Case | Reusable, frequently called | Conditional, one-time use |
Naming | Named | Anonymous or Named |
- Handling code that requires execution only when certain conditions are met.
- Defining functions that should exist only in specific scopes.
- Using closures to maintain private state and data encapsulation within a function.
Understanding the concept of IIFE (Immediately Invoked Function Expression) can further enhance the use of function expressions. An IIFE is a design pattern which executes a function as soon as it is defined. This pattern is commonly used to create a scope around code to avoid variable collisions in the global environment. Here's how an IIFE looks in practice:
(function() { let message = 'Hello, IIFE!'; console.log(message);})();This pattern is beneficial for modularizing code and ensuring that the defined function's variables do not interfere with others.
Immediately Invoked Function Expression in Javascript
Immediately Invoked Function Expressions (IIFE) in Javascript are a powerful approach that allows a function to be executed right after it is defined. This pattern is useful in controlling scope, preventing pollution of the global namespace, and encapsulating code. Let's delve deeper to better understand this concept.
JavaScript Immediately Invoked Function Expression Explained
An Immediately Invoked Function Expression, commonly abbreviated as IIFE, is a function in Javascript that runs as soon as it is defined. An IIFE is typically used to create an isolated scope, encapsulating variables and functions, which prevents them from affecting the global scope. This is particularly advantageous when you want to avoid namespace collisions and maintain modular code structures.Here is the basic syntax of an IIFE in Javascript:
(function() { // Your logic here})();The use of parentheses before the function keyword signifies that the function is being treated as an expression. The immediate call at the end with () executes the function immediately.
Below is a simple example of an IIFE in Javascript:
(function() { let userName = 'Alice'; console.log('Hello, ' + userName);})(); // Outputs: Hello, AliceThis function runs immediately and allows the variable userName to exist only within its isolated scope.
An IIFE can be written with an arrow function to achieve a more concise syntax:
(() => { /* code */})();
While the typical use of the IIFE pattern is for scope encapsulation, it can also incorporate additional logic to enhance its utility. Consider an IIFE that accepts parameters. This is beneficial when you need to pass data directly into the function:
(function(x, y) { console.log('Sum:', x + y);})(5, 10); // Outputs: Sum: 15In this deeper dive, note that the IIFE still serves its role of executing immediately, but now brings in external data to work with. This example demonstrates how versatile the IIFE pattern can be, enabling functionalities such as module patterns, data privacy, and avoiding dependency conflicts in larger scripts.
Use Cases for Immediately Invoked Function Expression in Javascript
Understanding when to utilize Immediately Invoked Function Expressions (IIFE) can greatly enhance your Javascript programming skills. Below are some common use cases for IIFE:
- Scope Encapsulation: IIFEs are perfect for creating a private scope. Variables defined inside an IIFE are not accessible outside, thereby avoiding global scope pollution.
- AJAX or Event-Driven Code: Enclosing asynchronous code (such as AJAX requests or event handlers) inside an IIFE can help maintain a clean and independent scope.
- Module Patterns: IIFEs are widely used to create modules in JavaScript. This pattern encapsulates specific functionality and exposes only necessary parts to the public API.
- Performance Optimization: By using IIFEs, certain functions can access the most specific optimizations the Javascript engine can perform, improving load times and execution.
Practical Examples of Javascript Function Expressions
Exploring practical examples of Javascript Function Expressions allows you to grasp how they can be effectively utilized in real-world applications. Function expressions offer flexibility and specificity in various coding scenarios.
Writing a Simple Javascript Function Expression
A simple Javascript function expression involves defining a function inline and assigning it to a variable. This is particularly useful when the function is tied to a specific context or is required only within a local scope. Below is a basic example demonstrating how to write a simple function expression:
// Function Expression assigned to a variablelet addNumbers = function(a, b) { return a + b;};console.log(addNumbers(5, 3)); // Outputs: 8
Function expressions can also be anonymous, which means they do not need a function name.
The above example showcases a function expression used to add two numbers. Notice how the function is not available until it is defined, emphasizing the non-hoisting nature of function expressions. This provides an opportunity to control the visibility and availability of functions within your scripts. Here's a breakdown of the process:
- Define the function inline using the 'function' keyword.
- Assign it to a variable, making the function accessible through this variable name.
- Invoke the function using the variable, passing any necessary arguments.
When using function expressions, it's crucial to understand the notion of closures. A closure is a feature that allows the function to access variables from the enclosing scope, enabling powerful patterns like data privacy and stateful functions. Consider this deeper example of closures within a function expression:
let createMultiplier = function (multiplier) { return function (x) { return x * multiplier; };};let double = createMultiplier(2);console.log(double(5)); // Outputs: 10In this example, createMultiplier returns another function that behaves as a closure, maintaining the multiplier value even after createMultiplier finishes execution.
Examples of Javascript Immediately Invoked Function Expression
Immediately Invoked Function Expressions (IIFE) are often employed to enforce a local scope and execute code immediately. This is a particularly useful technique for code modularization, ensuring it does not interfere with the global namespace. Below is an IIFE example that demonstrates its immediate execution:
(function () { let score = 0; console.log('Initial Score:', score);})(); // Outputs: Initial Score: 0
IIFEs are wrapped in parentheses to indicate that they are expressions, not statements.
The example above initiates a new local scope, where score is defined and used immediately. This encapsulation ensures variables do not leak into the global scope. The typical syntax involves:
- Wrapping the function in parentheses to establish it as an expression.
- Appending parentheses directly after to invoke the function instantly.
IIFEs can be instrumental in establishing modular code structures. In the following example, an IIFE is used to create a counter module that safely encapsulates data:
let counterModule = (function() { let counter = 0; return { increment: function() { return ++counter; }, decrement: function() { return --counter; }, value: function() { return counter; } };})();console.log(counterModule.increment()); // Outputs: 1console.log(counterModule.value()); // Outputs: 1This module pattern utilizes an IIFE to protect the counter variable, only allowing interaction through exposed methods. The IIFE secures the internal state, thereby preventing direct manipulation, which aids in maintaining data integrity across the application.
Javascript Function Expressions - Key takeaways
- Javascript Function Expressions Definition: Define a function inline and assign it to a variable for dynamic and adaptable code, especially when logic depends on runtime conditions.
- Function Expression in Javascript: Functions defined using expressions are assigned to variables, are not hoisted, and can be named or anonymous.
- Javascript Function Declaration vs Expression: Declarations are hoisted and always named; expressions are not hoisted, can be anonymous, and offer controlled scopes.
- Immediately Invoked Function Expression in Javascript (IIFE): Functions executed right after definition, used for scope isolation and preventing global namespace pollution.
- Javascript Immediately Invoked Function Expression: Key for modular code, immediately runs to create private scopes, often used for performance optimization and data encapsulation.
- Practical Uses of Javascript Function Expressions: Useful in creating localized functions, closures for data privacy, and IIFEs for initializing modules without global conflicts.
Learn faster with the 24 flashcards about Javascript Function Expressions
Sign up for free to gain access to all our flashcards.
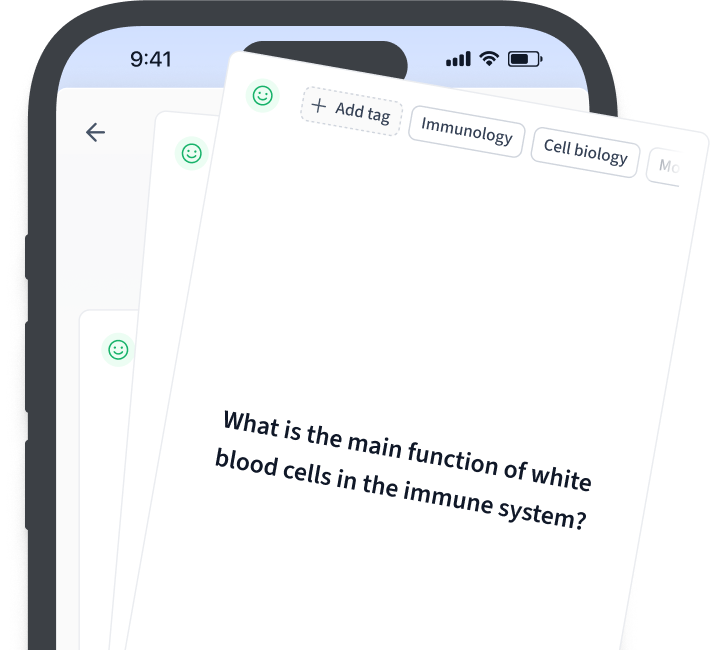
Frequently Asked Questions about Javascript Function Expressions
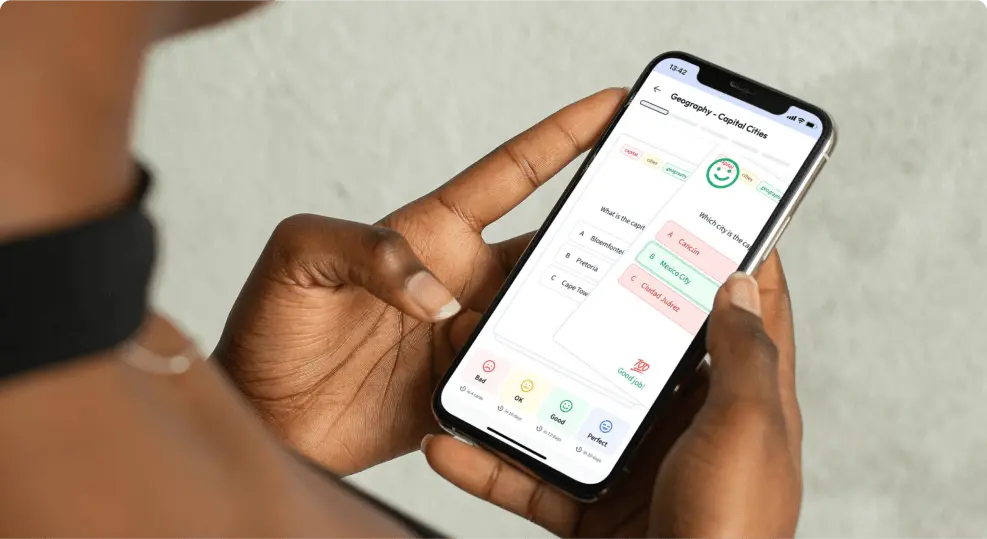
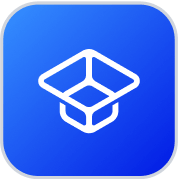
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more