Jump to a key chapter
Understanding Javascript Primitive Data Types
In the study of Computer Science, particularly when learning how to code with Javascript, understanding Javascript Primitive Data Types forms the basic pillar. Javascript Primitive Data Types are various forms of data that interact independently and don’t change their initial state or behaviour.
Overview of Javascript Primitive Data Types
Focusing on Javascript, you'll find that it uses a set of built-in types, also known as primitive data types. These types are the building blocks of all values in Javascript. There are five different types:
- String
- Number
- Boolean
- Undefined
- Null
Each of these data types behave differently under different circumstances and it's vital to understand these behaviours to efficiently write and execute code.
A String is a sequence of characters used to represent text.
Number represents numerical values and it can be integers, floats, etc.
Boolean are logical entities and can only have two values: true or false.
Undefined implies that no value has been assigned to a variable.
Null denotes that a variable has no value or no object. It is deliberately assigned to a variable.
The Significance of Javascript Primitive Data Types in Programming
In software development, each Javascript Primitive Data Type plays a strategic role—like the pieces on a chessboard, each with its unique importance and method of movement.
These fundamental data types are essential in accomplishing a variety of tasks such as performing arithmetic calculations, using conditional logic, managing arrays, and handling operations on text strings.
Exploring the Five Javascript Primitive Data Types
Now let's take a deeper look into each of these Javascript Primitive Data Types and how they function:
For a String, you can use either single or double quotes to create a string:
'Hello, World!' "Hello, World!"
While for a Number, numerical operations like addition, subtraction, division, and multiplication can be performed:
50 + 25 100 - 50
For Boolean, it is commonly used in conditional statements, it returns either true or false:
5 > 3 \(\longrightarrow\) true 5 \< 3 \(\longrightarrow\) false
The Undefined value is returned when you try to access a not yet declared variable:
var test; console.log(test); \(\longrightarrow\) undefined
While Null is an assigned value that means nothing:
var test = null; console.log(test); \(\longrightarrow\) null
Characteristics and Functions of the Five Primitive Data Types in Javascript
Here's a brief rundown of the unique characteristics that define each primitive data type:
Data Type | Characteristics |
String | Textual data enclosed in quotes (' ') or (" "). |
Numeral | Integers and floating-point numbers, no distinction between various kinds of numbers. |
Boolean | Only two values, true or false. |
Undefined | The type of a variable that hasn’t been initialized. |
Null | It is explicitly nothing or an empty value. |
Useful Examples of Javascript Primitive Data Types
Let's illustrate the usefulness of these Javascript Primitive Data Types with some practical code examples:
For example, if you want to store a user's name and age, you might use a string and a number respectively:
var userName = "James"; var userAge = 25;
And a boolean could be used if you want to check whether the user is of legal age (over 18 in most countries) to access certain resources:
var isLegalAge = userAge >= 18;
Note that 'userName', 'userAge', and 'isLegalAge' are all data variables, but they each store different types of primitive data. Understanding how and when to use these data types will allow you to write more effective Javascript code.
Distinguishing Between Primitive and Non-Primitive Data Types in Javascript
As we continue to delve deeper into the world of JavaScript, it becomes essential to understand the other side of data types - the Non-Primitive data types. These are called reference data types. They include Objects, Arrays and Functions. Contrary to Primitive Data Types, Non-Primitive Data Types can accept multiple values due to their dynamic behaviour.
Key Differences Between Primitive and Non-Primitive Data Types in Javascript
Let us establish the differences between the two, taking into consideration their unique functionalities.
- Mutability: One principal distinction is that while Primitive data types are immutable (which means they cannot be altered), Non-primitive data types are mutable and can be modified.
- Storage: Primitive data types store a single value and occupy a single memory space, whereas Non-primitive data types can store multiple values and hence, occupy multiple memory spaces.
- Methods: Primitive types do not have methods while Non-primitive types come with pre-defined methods.
- Sharing: Non-primitive data types can be shared and referenced at multiple places in the code, but Primitive data types cannot.
- Default Values: When no values are assigned, primitive data types have a default value, while non-primitive or reference data types have null as their default value.
Analysing the Contrast between Primitive and Javascript Non-Primitive Data Types
The fundamental differences between Primitive and Non-primitive data types as seen above merits a deeper examination of their key characteristics. These differences impact how variables are stored, accessed and manipulated in JavaScript. You see, when Primitive data types are assigned values, they store these values directly. This is not the case for Non-Primitive data types which store memory addresses of where the values are actually stored in your computer's memory.
Data Type | Category | Distinguishing Characteristics |
Boolean, String, Number, Null, Undefined | Primitive | Immutable, store single value, no methods, not shared, default value assigned |
Objects, Arrays, Functions | Non-Primitive | Mutable, store multiple values, have pre-defined methods, can be shared, default value is null |
Practical Examples Illustrating the Difference between Primitive and Non-Primitive Data Types in Javascript
Now that you've got a grasp of the key differences between Primitive and Non-Primitive data types in Javascript, let's explore this with some examples:
For example, if you declare a variable of a primitive data type and make changes to it, it does not affect any other variable. However, the scenario is different in the case of Non-Primitive data types:
// Primitive var nameFirst = "Lucas"; var nameSecond = nameFirst; // nameSecond also becomes "Lucas" nameFirst = "Anna"; // This change does not affect nameSecond console.log(nameSecond); // It will still output "Lucas" // Non-Primitive var arrFirst = [1, 2, 3]; var arrSecond = arrFirst; // arrSecond now points to the same array as arrFirst arrFirst[0] = 99; // Changing arrFirst also changes arrSecond since they reference the same memory location console.log(arrSecond); // Output will be [99, 2, 3]
An In-depth Study on the Behaviour of Primitive vs Non-Primitive Data Types in Javascript
Investigating further, you can see how these Primitive and Non-Primitive data types can be used, manipulated, and worked with in JavaScript. Primitive types are simple and straightforward - a number is a number and a string is a string. However, Non-Primitive types are complex; they store more than just a single value and can perform operations.
For instance, Non-Primitive types like arrays and objects even have their own unique set of methods. This is because JavaScript objects, such as arrays and functions, provide operation methods that can be applied directly. For example, the array's sort() method and length property, or the function's call() and bind() methods.
Understanding both Primitive and Non-Primitive data types gives you a robust toolkit to handle different data types and perform a range of operations in JavaScript. You now are a step closer to becoming proficient in handling data in your JavaScript coding adventures.
Insight into the Difference between Primitive and Reference Data Types in Javascript
In Javascript, data types are a fundamental concept, grouped into two categories—Primitive and Reference data types. The Primitive data types include Number, String, Boolean, Null, Undefined, and Symbol (introduced in ES6), while the Reference data types encompass Objects, Arrays, and Functions, including user-defined data types.
Primary Differences between Primitive and Reference Data Types in Javascript
Javascript's inbuilt data types, Primitive and Reference, have distinct characteristics that can significantly influence programming aspects such as memory allocation, variable assignment, and method invocation.
- Mutability: Primitive data types are immutable—they cannot be changed after they are created. This does not mean that if a Primitive value is assigned to a variable, that variable's value cannot be changed—it simply means that the actual value cannot be altered.
In contrast, Reference data types are mutable—their values can be altered. This is evident in manipulating objects and arrays.
- Storage: When dealing with Primitive data types, values are stored directly in the location that the variable accesses. Conversely, with Reference data types, the variable location is connected to a place in memory where the data is stored.
- Value comparison : For Primitive data types, the equality (==) or identity (===) operations check the actual values. However, these operations for Reference data types only check if the references match, not the actual values.
A Detailed Comparison between Primitive and Reference Data Types in Javascript
Digging deeper into the distinct differences between Primitive and Reference data types is critical for leveraging the power of Javascript.
Once a Primitive value is created, it can't be directly altered or 'mutated'. This feature is what makes it a 'primitive' or 'simple' data type—it's a straightforward and computationally efficient means of representing data. This might make Primitive data types seem like the less powerful Javascript data types. But there are benefits to their simplicity, such as predictable behaviour and easy management.
On the other hand, Reference data types offer a high degree of complexity and flexibility. They allow for more customisation and control over the data structure. An array, for example, can hold data in a structured format, allowing complex operations on data with methods like filter(), map(), and reduce(). The risk of mutable data, however, is that it can be challenging to track how data changes throughout a program, leading to potent potential pitfalls.
Javascript: Practical Scenarios Using Primitive and Reference Data Types
In Javascript programming, understanding when to use Primitive and Reference data types comes into play in various practical scenarios.
For instance, if you are adding a sequence of numerical responses to a questionnaire, Primitive numbers would be the ideal choice. When you are faced with the task to group these responses to representatives or to main categories, then an object (which is a Reference data type), with its key-value pairs, becomes more fitting.
Understanding the Applicability of Primitive vs Reference Data Types in Javascript Programming
The applicability and suitability of primitive versus reference data types in Javascript programming often comes down to what you wish to achieve with the data you have.
If you need to manage multi-faceted data sets and perform complex operations on them - say you're building a data visualisation application - the built-in methods provided by Javascript's Reference data types like arrays and objects would be beneficial and commonly used. On the other hand, if you're conducting mathematical operations or tokenising strings, Primitive data types would be the better choice.
Additionally, if you're dealing with data where consistency and data integrity throughout the application lifecycle is crucial, you might want to lean towards Primitive data types. The immutability of Primitives safeguards against unintended changes to data values that can happen with mutable Reference data types, which might be a critical feature in certain applications.
So in essence, the decision to use Primitive or Reference data types often depends on your application's requirements, and what you need to accomplish with your data.
Javascript Primitive Data Types - Key takeaways
- Javascript Primitive Data Types are basic forms of data that interact independently and don’t change their initial state or behaviour.
- The five Javascript Primitive Data Types are: String, Number, Boolean, Undefined, Null
- The String primitive data type is a sequence of characters used to represent text; Number represents numerical values such as integers or floats; Boolean is a logical entity with only two possible values: true or false; Undefined implies no value has been assigned to a variable; and Null means a variable has no value or no object.
- Non-Primitive data types, also known as reference data types, on the other hand, include Objects, Arrays and Functions and can hold multiple values due to their dynamic behaviour.
- Key differences between Primitive and Non-Primitive Data Types in Javascript include: Mutability - Primitive data types are immutable (cannot be altered) while Non-Primitive data types are mutable (can be modified); Storage - Primitive data types store a single value and occupy a single memory space, while Non-Primitive data types store multiple values and occupy multiple memory spaces; Methods - Primitive types do not have methods while Non-Primitive types do; Sharing - Non-Primitive data types can be shared and referenced at multiple places but Primitive data types cannot; Default Values - Primitive data types have a default value when no value is assigned, while non-primitive or reference data types default to null.
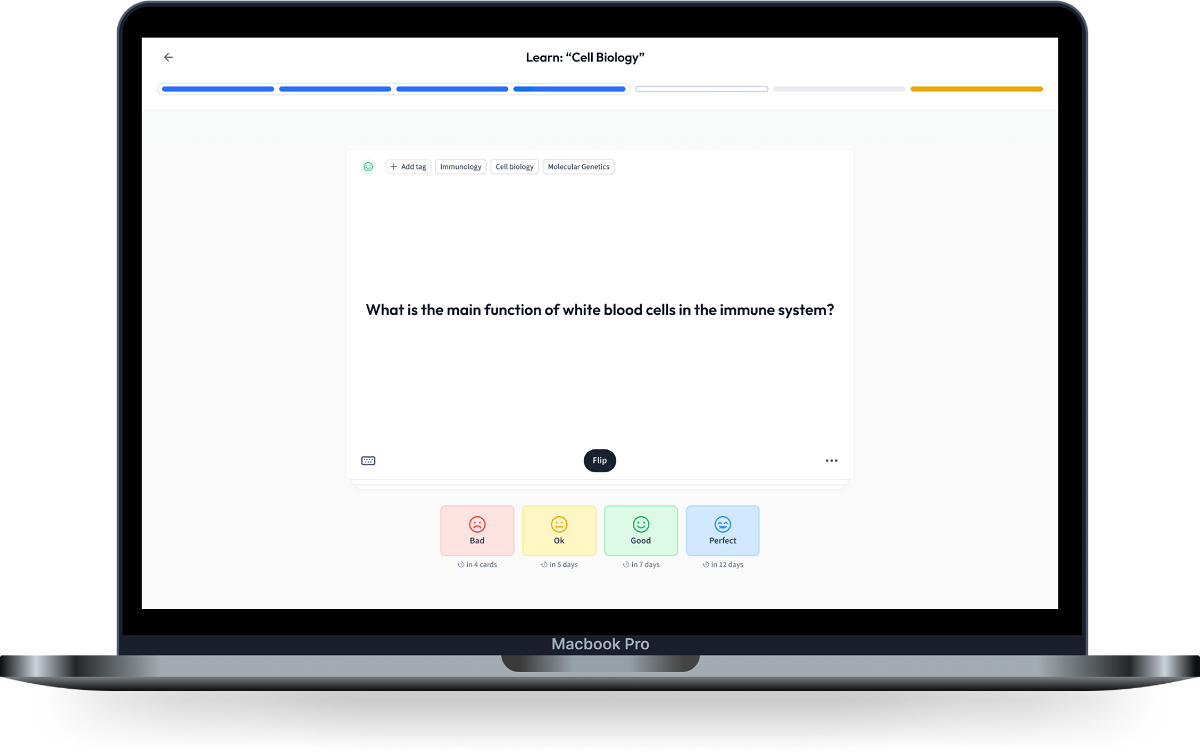
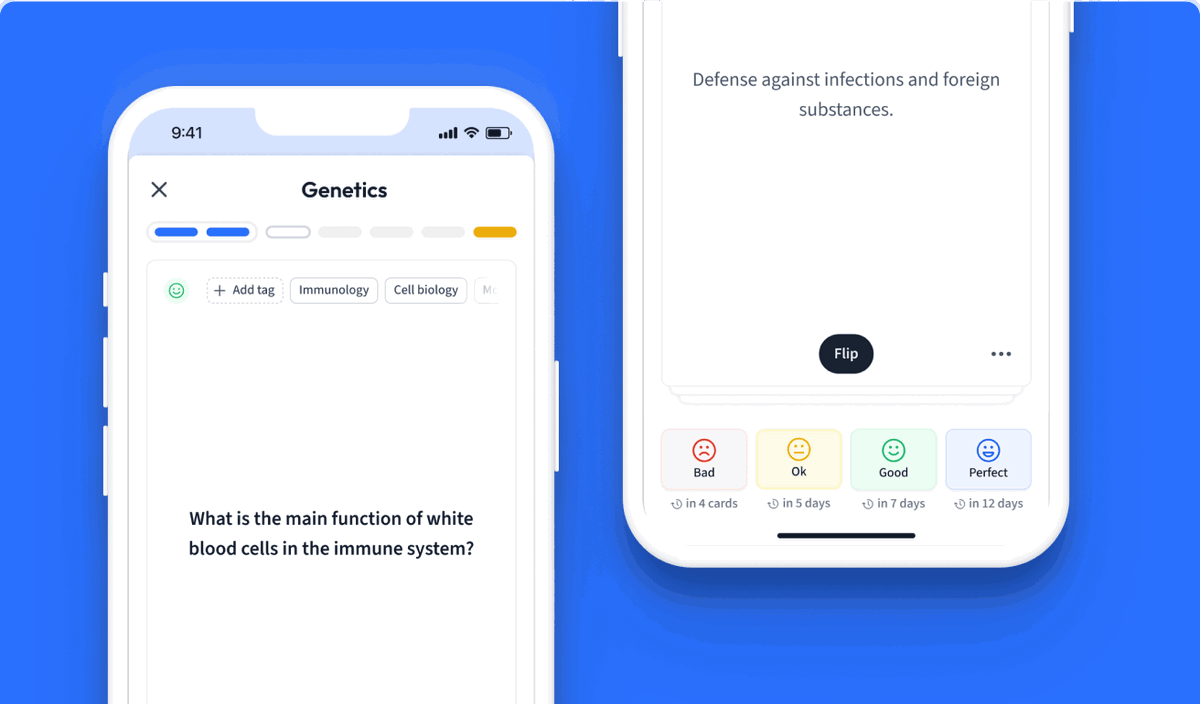
Learn with 12 Javascript Primitive Data Types flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Javascript Primitive Data Types
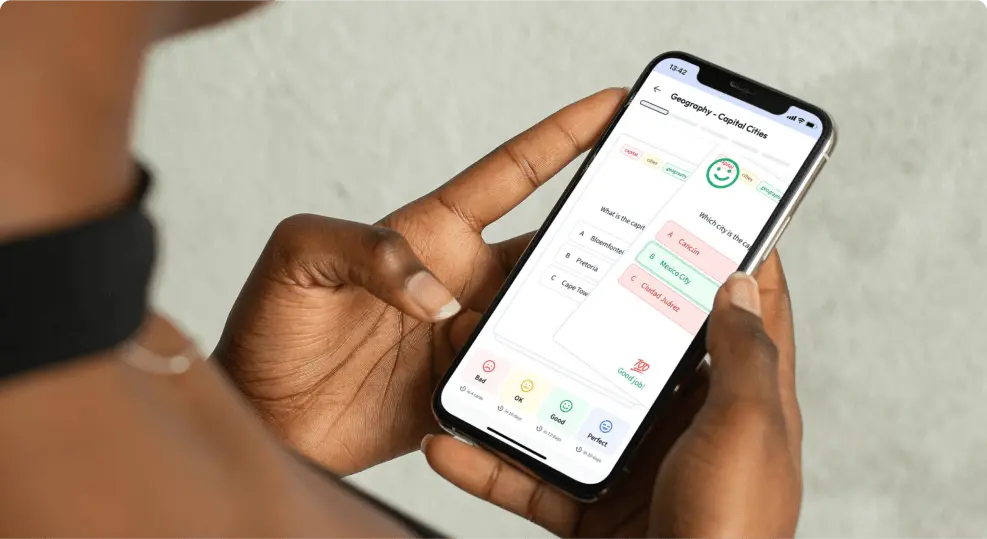
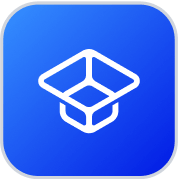
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more