Jump to a key chapter
Understanding Javascript Selecting Elements
Javascript Selecting Elements is a fundamental concept in web development that allows you to interact and manipulate webpage elements. It involves using Javascript to identify and select specific elements, like , ,, and so forth, within your HTML document's DOM (Document Object Model).
The Document Object Model (DOM) is a platform- and language-neutral interface that treats an XML or HTML document as a tree structure wherein each node is an object representing a part of the document.
An Introduction to Javascript Select Element by ID
In Javascript, you can select elements with a specific ID using the Document methodgetElementById(). This method returns the first element with specified ID.
Considering you have an element like this:
Hello, world!To select this element by ID in Javascript, you simply write:
var element = document.getElementById("example");
Practical Steps to Javascript Select Element by ID
To practically implement Javascript selection of elements by ID, follow these steps:- Ensure that the HTML element has an ID.
- Type
var element = document.getElementById("yourElementID");
in your Javascript file. - Replace "yourElementID" with the actual ID of the element you intend to select.
How to Javascript Select Element by Class
Javascript also enables selecting elements by their class. This is done by using the Document methodgetElementsByClassName(). This method returns an array-like object of all child elements with the specified class.
Suppose we have these two elements:
You can select these elements by class in Javascript like this:Hello, world!
Another element
var elements = document.getElementsByClassName("example");
Remember, while getElementById returns a single element, getElementsByClassName returns a live HTMLCollection of elements with the specified class name. It means the returned collection will automatically update when elements are added or removed from the document.
Improving Skills in Javascript Select Element by Class
To master the skill of selecting elements by class in Javascript, follow these guidelines:- Make sure the HTML elements have a class attribute.
- Write
var elements = document.getElementsByClassName("yourElementClass");
in your Javascript file. - Replace "yourElementClass" with the actual class of the elements to be selected.
- Since
getElementsByClassName()
returns an array-like object, use array methods to access and manipulate the selected elements.
var firstElement = elements[0];
Mastering Parent and Child Elements in Javascript
In the road to mastering Javascript, understanding the relationship between parent and child elements is essential. Navigating these relationships allows you to traverse the DOM and effectively manipulate web page elements. Two critical concepts discussed in this context are: how to select parent elements and creating select elements in Javascript.Guide to Javascript Select Parent Element
Before diving into selecting parent elements, it's crucial to familiarise ourselves with the concept of a 'parent element'.A parent element in the DOM is an element that has other elements, generally known as 'child elements', nested within it. For example, in the code snippet
the 'div' element is the parent of the 'p' element.Hello, world!
parentNodethat can be used to get the parent element of a specified element. Suppose a
element is nested within aHello, world!
element. To select the parent element of the paragraph in Javascript, simply write:var parentElement = document.getElementById("textElement").parentNode;How to Navigate Javascript Select Parent Element
Navigating parent elements in Javascript mainly involves understanding the DOM structure and knowing how to use theparentNodeproperty. Take these steps to select the parent element in Javascript:
- Select the child element first. Using the
getElementById()
or any other Javascript selection method is a practical approach. - Once you've selected the child element, use the
parentNode
property to select its parent element. Here's an example:var parentElement = document.getElementById("myElement").parentNode;
- Confirm the selection by displaying the parent element. Use
console.log(parentElement);
to ensure the right element has been selected. If you've done everything correctly, it'll display the HTML content of the parent element in your browser console.
Techniques to Create Select Element in Javascript
Creating a select element, typically a dropdown list, in Javascript involves generating the select element and its options dynamically. This is useful when you want to display a variety of options for application users to choose from, which could be arising from a database, file, or an API response. Here, the Javascript methodcreateElement()is utilised. This method generates the specified HTML element. For instance, to create a select dropdown with two options, you can write the following code: ```javascript var selectElement = document.createElement("select"); var option1 = document.createElement("option"); var option2 = document.createElement("option"); option1.text = "Option 1"; option1.value = "1"; option2.text = "Option 2"; option2.value = "2"; selectElement.add(option1); selectElement.add(option2); ``` You now have a select element with two options ready to be added to your HTML document.
Step-by-step Process to Create Select Element in Javascript
Creating a select element involves certain steps. Let's enumerate them:- Create the select element, using
var selectElement = document.createElement("select");
- Define the options that you want to add to the select element. This is done by creating "option" elements and setting their text and value. For instance,
var option = document.createElement("option"); option.text = "Your Option"; option.value = "Your Value";
- Add the options to the select element using the
add()
method:selectElement.add(option);
- Finally, append the select element to your HTML document. One way to do this is by selecting an existing element in the document and appending the select element as its child:
document.body.appendChild(selectElement);
Delving into the DOM and Arrays Using Javascript
Interacting with the DOM (Document Object Model) is a critical part of utilizing Javascript for front-end development. At the same time, being comfortable withHow to Select a DOM Element in Javascript
In Javascript, selecting DOM elements is achieved through various methods provided by thedocumentobject. Some popular methods include
getElementById(),
getElementsByClassName(),
getElementsByTagName()and
querySelector(). The method
getElementById()is used for selecting an element with a specific id. It's important to note that id values are unique to each element, so this method will return just one element. On the other hand, methods like
getElementsByClassName()and
getElementsByTagName()can select multiple elements as they return an array-like object of all child elements which match the specific class name or tag name. The
querySelector()method, however, allows for more flexibility as it uses a CSS selector to identify elements, and it only returns the first matching element. There's also
querySelectorAll()which returns all matching elements. These methods provide a variety of ways to select elements within the DOM and work with them using Javascript. As a developer, understanding when and how to use these methods allows you to write more efficient and effective scripts.
Examples of How to Select a DOM Element in Javascript
Understanding the concept of selecting DOM elements is easier when looked at with examples. Consider we have the following HTML structure:Now if you want to select the div with the id of "main", you would use:Hello!
Goodbye!
Other text
var mainDiv = document.getElementById('main');To select all paragraph elements with the class of "text", you would use:
var textElements = document.getElementsByClassName('text');This would return an array-like object of elements, and you can access individual elements like
textElements[0]To select the first paragraph element regardless of its class, you could use:
var firstParagraph = document.querySelector('p');These examples should give you an idea of how different methods can be utilized to select elements from the DOM using Javascript.
How to Select a Random Element from Array in Javascript
Selecting a random element from an array in Javascript is a common task encountered during coding challenges or while building certain features in a project. This requires an understanding of arrays and the built-in methods and properties in Javascript. The logic involves generating a random number that serves as the index to pick from the array. Javascript's Math object provides methods that can be used for this purpose, namelyMath.random()and
Math.floor().
Math.random()generates a random number between 0 (inclusive) and 1 (exclusive). To get a random index, you multiply the random number by the length of the array, which gives a number between 0 and one less than the length of the array, which correlates exactly with the indexes of an array.
Math.floor()is used to round off the random number to a whole number, which can be directly used as an array index. By combining these methods, you can access a random element from your array.
Tips and Tricks to Select a Random Element from Array in Javascript
Here's a step-by-step explanation of selecting a random element from an array in Javascript. Let's assume you have an array like this:var arr = ["red", "blue", "green", "black", "white"];First, generate a random index:
var randomIndex = Math.floor(Math.random() * arr.length);Then, use this random index to pick a random element from the array:
var randomElement = arr[randomIndex];That's how you can select a random element from an array in Javascript! To put it in a function that you can use repeatedly: ```javascript function getRandomElement(arr){ var randomIndex = Math.floor(Math.random() * arr.length); return arr[randomIndex]; } ``` With this function, you can call
getRandomElement(arr)at any point in your code to get a random element from the array `arr`.
Working with Class Oriented Selection in Javascript
Class-oriented selection in Javascript is a fundamental concept that plays a vital role in designing interactive web pages. Leveraging the ability to select all elements in a document that share the same class can enable more efficient manipulation of DOM elements. It's a vital technique for applying CSS styles dynamically and performing batch modifications on HTML elements, such as toggling visibility and adding event listeners.How to Select All Elements with Same Class in Javascript
There's a simple and straightforward approach to select all elements with the same class in Javascript – thegetElementsByClassName()method. This method provides a live HTMLCollection which contains every element in the document with the specified class name or names.
The term 'live' is exceptional as it denotes that the returned HTMLCollection is automatically updated when the document changes; thus, it always holds the current list of elements with the specified class.
document.getElementsByClassName(className)Replace \( \text{{'className'}} \) with the name of the class you wish to select. Remember that if the class name contains any spaces, it will be treated as multiple names. This method returns an HTMLCollection of all descendants of the node on which it was called that have the given class names. Here's an in-depth example for better understanding:
Div 1
Div 2
Div 3
In this example, the getElementsByClassName()method selects both div elements with the class name "class2", and the console will log these two elements.
Practical Examples: Selecting All Elements with Same Class in Javascript
Often developers need to perform operations on groups of elements like changing the content, changing the style, adding/removing attributes and so on. Selecting elements with the same class section enables us to do this easily.
This example changes the value of all input tags with the class name “className” to "New Value".
Javascript Selecting Elements Example
Understanding how to work with elements in a document is at the heart of Javascript's most fundamental operations. Selecting HTML elements involves using Javascript browser or DOM methods to get references to the elements you want to work with. Earlier, we discussed thegetElementsByClassName()method. Now let's talk about the
getElementById(),
getElementsByTagName()and
querySelector()methods. The
getElementById()method returns the element that has the ID attribute with the specified value. For example:
document.getElementById("myID")
The getElementsByTagName()method retrieves all elements that share the provided tag name, returning an HTMLCollection of the matching elements. For example, to get all paragraph elements in a document, you would use:
document.getElementsByTagName("p")
The querySelector()is a method that returns the first element that matches a specified CSS selector. For example, to get the first element with class "myClass", you would use:
document.querySelector(".myClass")
In-depth: Javascript Selecting Elements Example
To highlight the use ofgetElementById(),
getElementsByTagName(), and
querySelector(), let's examine an HTML document containing various elements and manipulate them using Javascript.
In this example,To be or not to be.
That is the question.
Whether 'tis nobler in the mind to suffer.
getElementById()is used to select the 'div' element with the ID "myDiv".
getElementsByTagName()is used to select the paragraph elements within this 'div', and a for loop is used to set the text color of each paragraph to red. Finally,
querySelector()is used to change the text color of the first paragraph element with the class "class2" to blue.
Javascript Selecting Elements - Key takeaways
- Javascript provides several methods to select elements such as:
getElementById()
- select an element with a specific id.getElementsByClassName()
- select elements with a specific class name. Returns an 'array-like' HTMLCollection of elements.getElementsByTagName()
- select elements with a specific tag name.querySelector()
- uses a CSS selector to identify elements, and only returns the first matching element.querySelectorAll()
- uses a CSS selector to identify elements, and returns all matching elements.
- A parent element in the DOM is one that has other elements nested within it. These nested elements are known as 'child elements'. Javascript provides the property
parentNode
to get the parent element of a specified element. - A select element (typically a dropdown list) can be created in Javascript using the
createElement()
method, defining the options, adding the options to the select element and finally appending select element to the HTML document. - Selecting a random element from an array in Javascript involves generating a random number using the
Math.random()
andMath.floor()
methods to serve as the index and retrieve the item at the index. - Using the method
getElementsByClassName()
, all elements with the same class can be selected in Javascript. The method returns a live HTMLCollection, which means it automatically updates when the document changes.
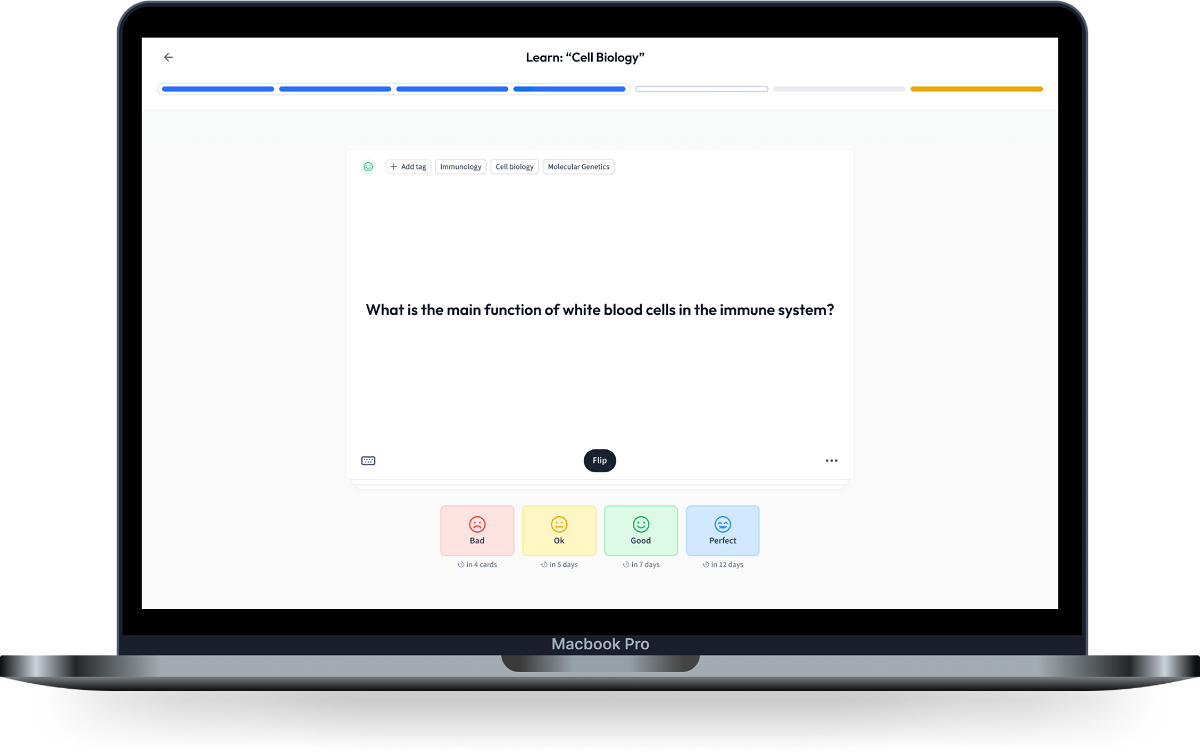
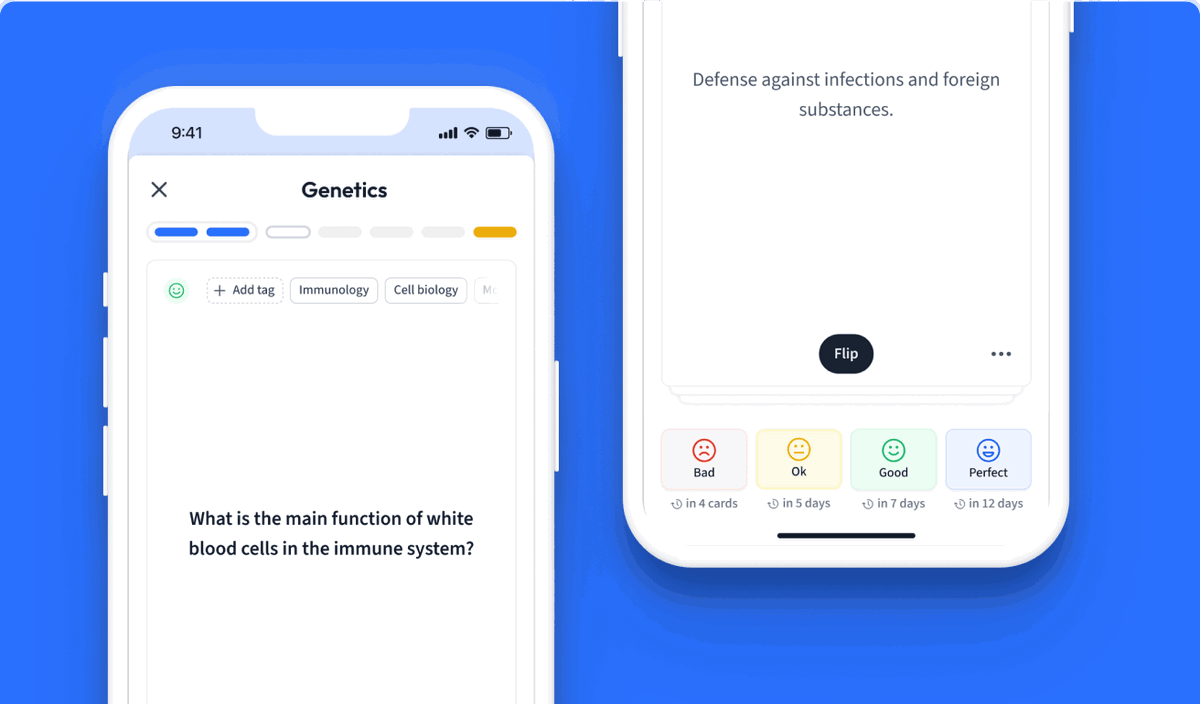
Learn with 12 Javascript Selecting Elements flashcards in the free StudySmarter app
Already have an account? Log in
Frequently Asked Questions about Javascript Selecting Elements
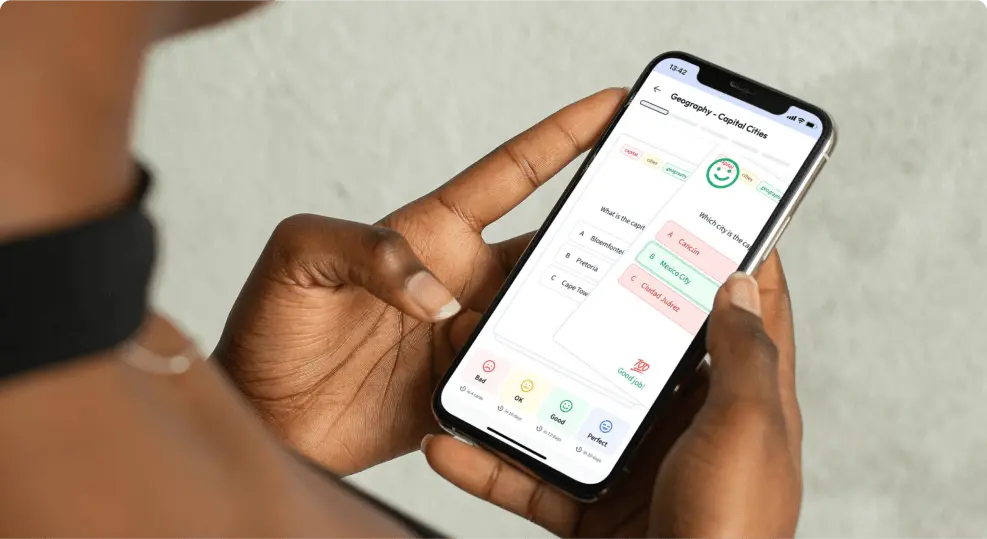
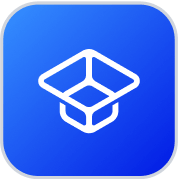
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more