Jump to a key chapter
Introduction to Javascript Statements
JavaScript statements are the core instructions that tell the web browser to perform specific tasks. Understanding these statements is vital for anyone looking to explore the dynamic capabilities of web development.
What is a JavaScript Statement?
A JavaScript statement is an individual instruction designed to perform a specific action, like declaring a variable or displaying information. When a statement is completed, it is commonly followed by a semicolon (;
). These instructions, when combined, form the code necessary to drive your web applications.
Definition: A JavaScript statement is an individual instruction in a JavaScript program that performs a particular task. Each statement tells the browser to perform a certain operation.
Here is a simple example to illustrate:
var greeting = 'Hello, world!';alert(greeting);
In the above example, the first line is a statement that declares a variable named greeting and assigns it the value Hello, world!. The second line is another statement that prompts an alert box displaying the value of greeting.
Types of JavaScript Statements
JavaScript comprises various types of statements, each having unique functions. Here are some common types:
- Variable Declaration: Used to declare variables, e.g.,
var
,let
,const
. - Conditional Statements: Execute code based on conditions, e.g.,
if
,else
,switch
. - Loops: Automate repetitive tasks, e.g.,
for
,while
,do-while
. - Functions: Define reusable blocks of code, e.g.,
function
. - Expression Statements: Evaluate expressions that result in values.
- Return Statement: Specify the value a function should return.
Deep Dive: Functions in JavaScript are considered objects. This means you can store them in variables, pass them as arguments to other functions, and return them from functions. They also have their own methods and properties, making them powerful tools in JavaScript.
Hint: JavaScript is case-sensitive. Always use consistent capitalization when writing statements.
Understanding Javascript Conditional Statement
In JavaScript, conditional statements are crucial constructs that allow you to execute code differently based on the conditions you specify. These statements enable your web applications to make decisions and perform actions accordingly.
Javascript If Statement
The if statement is one of the most fundamental conditional statements in JavaScript. It evaluates a given condition, and if the condition evaluates to true, the code block associated with the if statement executes.
- Syntax: It begins with the keyword
if
followed by a condition in parentheses()
and a code block inside curly braces{}
. - Use else to execute an alternative code block if the condition is false.
- Use else if to check multiple conditions.
Definition: The JavaScript if statement is a control structure that executes a block of code based on a specified condition being true.
Consider an example to understand how the if statement works:
var score = 85;if (score > 70) { console.log('Pass');} else { console.log('Fail');}
In this code, the console will display 'Pass' because the condition score > 70
is true.
Hint: Conditions inside if statements should always be logical expressions, i.e., those that provide boolean outcomes.
Javascript Switch Statement
The switch statement is another conditional statement in JavaScript, offering a more readable way of evaluating expressions against a set of possible values. This statement is particularly useful for comparing the same expression with different values.
- Syntax: It begins with the keyword
switch
followed by an expression in parentheses()
. The possible case values are defined within curly braces{}
. - Each case represents a potential value of the expression, followed by a colon
:
and the code block to execute. - The optional default case executes when the expression doesn't match any case.
Here is an example to illustrate the switch statement:
var day = 3;switch (day) { case 1: console.log('Monday'); break; case 2: console.log('Tuesday'); break; case 3: console.log('Wednesday'); break; default: console.log('Another day');}
The console will output 'Wednesday' because the day
variable's value is 3.
Deep Dive: When using a switch statement, control falls through the cases unless you explicitly use the break statement. This means that without a break, the program executes the remaining cases until it encounters a break or the switch statement ends. This behavior is sometimes used intentionally for so-called 'fall-through' scenarios.
Looping with Javascript Statements
Looping is a fundamental concept in programming that allows you to repeat a block of code multiple times. JavaScript offers several looping constructs to make repetitive tasks easier and more efficient. Among them, the For and While loops are commonly used.
For Statement Javascript
The for statement in JavaScript is a powerful tool that allows you to iterate over a sequence of numbers, arrays, or objects. It's an ideal choice when you know in advance how many times you want to loop through a block of code.
- The syntax consists of three parts inside the loop's parentheses: initialization, condition, and increment.
- The loop begins with the
for
keyword, then it executes the code block as long as the specified condition is true. - Updates the loop variable in the increment section.
Here is an example of a for loop displaying numbers from 1 to 5:
for (var i = 1; i <= 5; i++) { console.log(i);}
This loop initializes i
to 1, continues as long as i
is less than or equal to 5, and increments i
by 1 each iteration.
Hint: Nested for loops are possible and often used for tasks such as iterating over a matrix or multi-dimensional data structures.
Deep Dive: For-loops can be swapped with other loop structures, but they provide a clean and minimal way to define loops with a specific number of iterations. They are incredibly versatile and can be adapted to various iterative processes in programming, which is why they are a staple in the world of coding.
While Statement Javascript
The while statement is another looping mechanism in JavaScript, suitable when the number of iterations isn't predetermined. It continuously executes the associated code block as long as the specified condition remains true.
- Syntax: It starts with the
while
keyword, followed by a condition in parentheses and a block of code. - Can lead to infinite loops if the condition never evaluates to false, so handle the loop control judiciously.
- Does not automatically include an iteration expression, so this must be managed within the loop.
Consider this while loop example that repeats as long as count
is less than 5:
var count = 0;while (count < 5) { console.log(count); count++;}
The loop initializes count to 0 and increments it in each iteration until count becomes 5.
Hint: Use the break statement to exit a while loop prematurely if needed, such as when a certain condition inside the loop is met.
Deep Dive: The conditions driving a while loop can be complex and based on external data input or changing application state. This makes them a great choice for scenarios where the loop's termination point can't be clearly predetermined at the start, allowing for dynamic and responsive coding structures.
Practical Javascript Statement Examples
JavaScript statements form the foundation of any script. These statements help you define the flow, functionality, and interactivity of your web applications. Here, you'll find practical examples of using different types of JavaScript statements effectively.
Variable Declaration in JavaScript
Variables are fundamental components in JavaScript used for storing data. You declare variables using statements such as var, let, and const. Here are examples of each:
- var: For general use across different scopes.
var name = 'Alice';
- let: Useful for block-scoped variable declarations.
let age = 30;
- const: For declaring constants, meaning their values can't be reassigned.
const PI = 3.14;
An example with a const declaration:
const MAX_USERS = 100;console.log('Max users: ' + MAX_USERS);
This prints out the maximum number of users allowed in a system, which is defined as a constant value.
Control Statements in JavaScript
Control statements direct the flow of execution in programs. Common control structures include if, else if, else, and switch statements.
Here's an example using an if-else statement:
var temperature = 32;if (temperature <= 32) { console.log('It is freezing');} else { console.log('It is not freezing');}
This checks if the temperature is less than or equal to 32 degrees, printing the corresponding message based on the condition.
Deep Dive: Nested if-else statements can be employed when multiple conditions need to be evaluated in a hierarchical manner. This structure can get complicated, so careful planning of logical conditions is necessary to avoid errors.
Looping Constructs in JavaScript
Loops are essential for repeating tasks, making them vital for operations involving arrays or collections. Two frequently used loop types are for and while loops.
Example of a for loop:
for (var j = 0; j < 10; j++) { console.log(j);}
This loop prints numbers from 0 to 9, iterating until the condition becomes false.
Deep Dive: JavaScript also accommodates looping constructs like do-while loops, which guarantee the execution of the loop's code block at least once before checking the condition. This is particularly useful when you need an action performed at least once regardless of the primary condition check.
Function Declarations in JavaScript
Functions in JavaScript allow you to create reusable blocks of code that perform specific tasks. They make your code modular and easier to maintain.
Here's how you define a simple function:
function greet() { console.log('Hello!');}greet();
The above defines a greet function that prints 'Hello!' and calls it immediately.
Hint: Functions can also take arguments that give you flexibility in performing tasks with variable data inputs.
Javascript Statements - Key takeaways
- JavaScript Statements: Core instructions that direct the web browser to perform tasks, such as declaring variables or displaying information, often ending with a semicolon (;).
- JavaScript Conditional Statements: Used for executing code based on specified conditions. Examples include
if
,else
, andswitch
statements. - JavaScript If Statement: A control structure that executes a block of code if a specified condition is true. Incorporates variations like else and else if.
- JavaScript Switch Statement: A conditional statement evaluating expressions against potential values, with case options, allowing more readable comparisons.
- For Statement in JavaScript: A looping construct used when the number of iterations is known in advance, includes initialization, condition, and increment sections.
- While Statement in JavaScript: Executes a code block as long as the specified condition remains true, suitable for executing tasks without a predetermined iteration count.
Learn faster with the 27 flashcards about Javascript Statements
Sign up for free to gain access to all our flashcards.
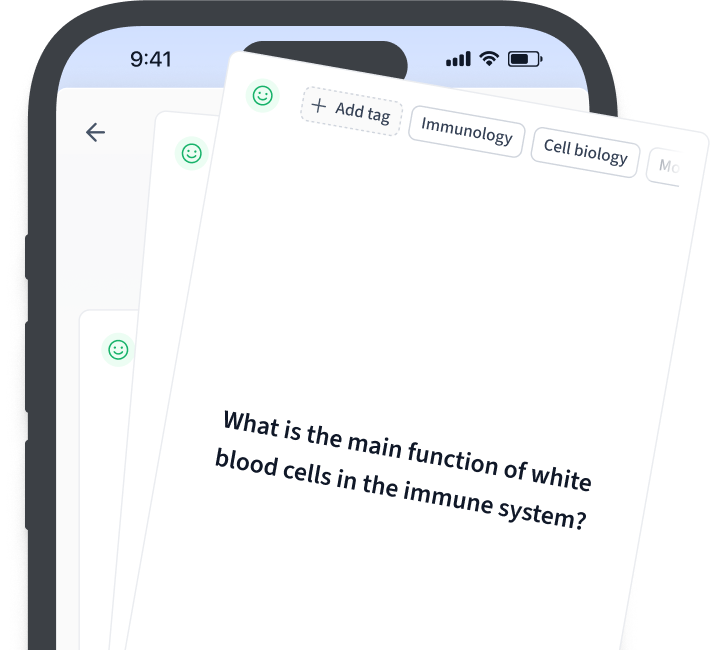
Frequently Asked Questions about Javascript Statements
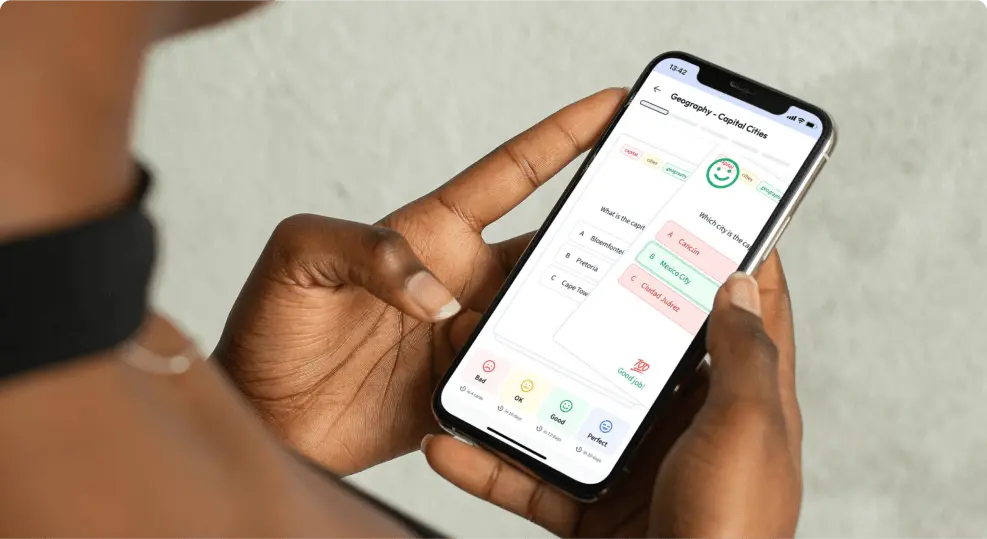
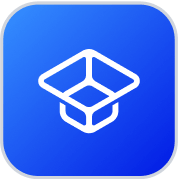
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more