Jump to a key chapter
Javascript Type Conversion
In JavaScript, converting a value from one type to another is a crucial concept. Understanding type conversion is essential as it allows you to handle different data types effectively.
Definition
Type Conversion refers to the process of converting one data type into another. In JavaScript, this can be done explicitly using functions or methods, or it can happen automatically (implicitly) when operations mix different data types.
Explicit Conversion: This occurs when you manually use functions to convert data types. For example, using Number()
to convert a string to a number.Implicit Conversion: This happens automatically when JavaScript needs to use a certain data type to perform an operation. For example, adding a number and a string will result in a string.
Explicit Type Conversion Examples
- Using the
Number()
function to convert a string to a number:
let str = '123';let num = Number(str); // num is now 123
- Using the
String()
function to convert a number to a string:
let num = 123;let str = String(num); // str is now '123'
Implicit Type Conversion Examples
- When a number and a string are added, resulting in a string:
let result = 'value: ' + 123; // result is 'value: 123'
- Boolean conversion during conditions:
let value = 0;if (value) { console.log('Truthy'); // This will not run} else { console.log('Falsy'); // This will run}
JavaScript treats an empty string ''
, null
, undefined
, NaN
, and 0
as falsy values.
Understanding Implicit Type Conversion (Coercion):JavaScript coercion can sometimes lead to unexpected results. When you use the +
operator with different data types, JavaScript may automatically convert the types to strings to perform concatenation. However, for subtraction, division, or multiplication, JavaScript tries to convert the values to numbers. Here are some nuanced examples to illustrate this:
'5' + 2
results in'52'
, a string. The number is coerced into a string.'5' - 2
results in3
, a number. The string is coerced into a number.'5' * 2
results in10
, a number. Again, the string is coerced into a number.
===
(strict equality) and explicit functions play important roles in controlling this behavior.Implicit Type Conversion in JavaScript
In JavaScript, implicit type conversion, also known as coercion, is a feature that automatically converts one data type to another during mathematical or logical operations. Understanding how this process works is key to effectively utilizing JavaScript in your programs.
Definition
Implicit Type Conversion occurs when JavaScript automatically converts types to match the operation being performed. For example, adding a string and a number results in the number being converted to a string.
How Implicit Conversion Works
Implicit conversion kicks in when an operation involves mixed data types. JavaScript attempts to make sense of the operation by converting the types automatically. Here are some typical scenarios you may encounter:
- When using the + operator, JavaScript converts numbers to strings if one of the operands is a string.
- For the -, *, and / operators, strings are converted to numbers if possible.
- Boolean context also triggers conversion, where non-boolean values are coerced to true or false.
- Example of automatic conversion in addition:
let result = '10' + 5; // result is '105', a string
- Example of automatic conversion in subtraction:
let result = '10' - 5; // result is 5, a number
Common Implicit Conversions
Here is how JavaScript typically handles implicit type conversions through various examples:
Expression | Result Type | Explanation |
'5' + 2 | string | The number 2 is converted to a string and concatenated. |
'5' - 2 | number | The string '5' is converted to a number, then subtracted. |
10 + true | number | The boolean true is converted to 1, therefore 11. |
10 + false | number | The boolean false is converted to 0, therefore 10. |
Remember, JavaScript considers undefined
, null
, NaN
, 0
, ''
(empty string), and false
as falsy.
JavaScript’s type coercion follows some intriguing rules that can surprise you if you're not careful. For loose comparisons (using ==), JavaScript will attempt to make types match, leading to unexpected results.For instance, comparing a number and a string:
5 == '5'
returnstrue
because the string is converted to a number.null == undefined
returnstrue
as they are considered equal in loose comparison.- However,
null === undefined
isfalse
because strict comparison (===) does not coerce types.
Javascript Type Conversion Methods
Handling different data types effectively involves understanding the methods available for JavaScript type conversion. It allows your code to work seamlessly with variables of various types.
Type Coercion in Javascript
Type coercion refers to the automatic or implicit conversion of values from one data type to another. This process is especially common in JavaScript, where it can occur in various scenarios:- Combining different data types using operators- Evaluating conditions in if statementsCoercion in JavaScript can sometimes lead to results you might not expect, making it essential to understand when and how it happens.
let val1 = '5';let val2 = 2;let sum = val1 + val2; // sum is '52'In this example, because
val1
is a string, val2
is coerced to a string, and they are concatenated. For precise operations involving type conversion, use explicit methods like parseInt()
for numbers.
Consider how JavaScript handles truthiness and falsiness. In logical contexts, JavaScript coerces values to boolean. Remember these key concepts:
undefined
,0
,null
,NaN
,''
(empty string), andfalse
evaluate to falsy.- All other values, such as objects, will be truthy.
Data Type Conversion in Javascript
While implicit conversion happens automatically, you can also manually convert data types using JavaScript's explicit type conversion methods. This practice ensures precision and clarity in your code:- Use Number()
, parseInt()
, or parseFloat()
to convert strings to numbers.- Use String()
to convert numbers to strings.
- Explicit conversion example using
Number()
:
let strNum = '123';let num = Number(strNum); // num is 123
Explicit conversion offers control and predictability, reducing errors from unintended type coercion. Here are basic functions used for type conversion in JavaScript:
Function | Usage |
Number() | Converts a string to a number |
String() | Converts any value to a string |
Boolean() | Converts any value to a boolean |
parseInt() | Parses a string and returns an integer |
parseFloat() | Parses a string and returns a floating-point number |
To avoid implicit conversion, always use strict equality ===
to compare values and types.
Javascript Type Conversion Examples
In JavaScript, handling and converting different data types is essential for writing robust and effective code. Knowing how type conversion works through examples will help you apply these concepts in your programming tasks.
Explicit Type Conversion
Example 1: Converting strings to numbers using Number()
:
let str = '42';let num = Number(str); // num is now the number 42console.log(typeof num); // Outputs: 'number'
For converting a string with leading zeros, parseInt()
is more reliable because it discards the zeros.
Example 2: Using parseInt()
and parseFloat()
:
let intValue = parseInt('101px'); // Parses '101' to the number 101let floatValue = parseFloat('3.14em'); // Parses '3.14' to the number 3.14These functions are handy when dealing with strings that contain units of measurement.
Implicit Type Conversion
Implicit or automatic type conversion occurs when JavaScript automatically converts data types during expressions involving different types. Here are some examples:
Example 1: String and number:
let sum = '5' + 5; // '5' is a string, result is: '55'JavaScript coerces the number 5 into a string and concatenates them.
Implicit conversion can sometimes lead to unexpected results due to how JavaScript handles mixed-type operands. Let's explore further:In arithmetic operations, when a string and a number are involved, JavaScript attempts to convert the string to a number for operations other than +
:
- Subtraction:
'10' - 5 // Returns: 5
the string '10' is converted to the number 10. - Multiplication:
'4' * 2 // Returns: 8
the string '4' is converted to the number 4.
'abc' / 2 // Returns: NaNIn this case, 'abc' cannot be converted to a number, resulting in NaN (Not a Number).
Always verify the data type you expect using typeof
to avoid unexpected behavior due to implicit conversion.
Javascript Type Conversion - Key takeaways
- Javascript Type Conversion Definition: The process of converting one data type into another, either explicitly with functions/methods or implicitly when operations involve different data types.
- Explicit Type Conversion: Manually using functions like
Number()
orString()
to change a data type. E.g.,Number('123')
gives123
. - Implicit Type Conversion in JavaScript: Automatic conversion when using mixed type operations. E.g., adding a string and a number yields a string.
- Type Coercion in JavaScript: Automatic conversion of data types, which can lead to unexpected results. E.g.,
'5' + 2
becomes'52'
. - JavaScript Type Conversion Methods: Functions like
parseInt()
,parseFloat()
,Number()
,String()
for explicit conversion. - Common Coercion Examples: JavaScript converts
'5' + 2
to'52'
, and'5' - 2
to3
. Use===
for strict equality to avoid unintentional coercion.
Learn faster with the 27 flashcards about Javascript Type Conversion
Sign up for free to gain access to all our flashcards.
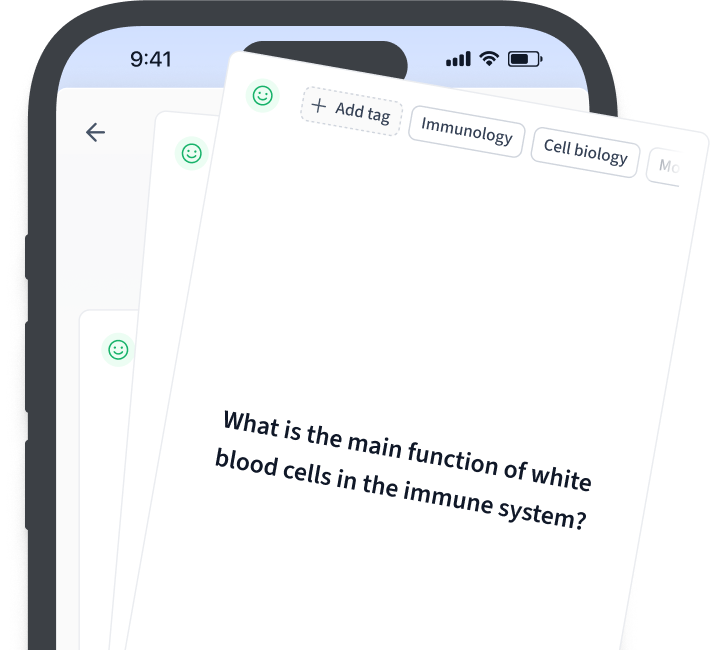
Frequently Asked Questions about Javascript Type Conversion
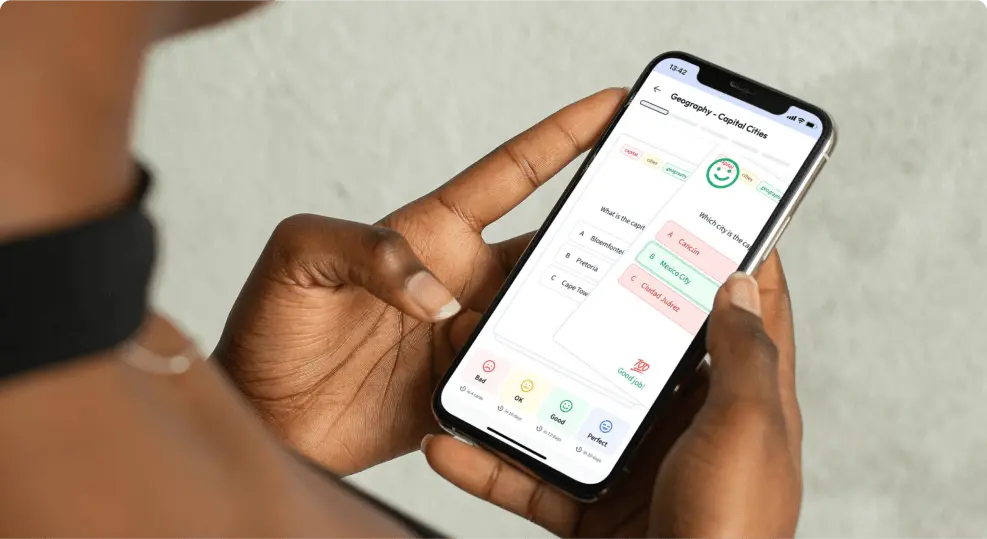
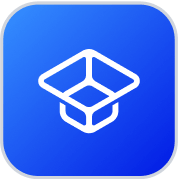
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more