Jump to a key chapter
Introduction to Logical Operators in C
Logical Operators in C are essential for making decisions based on multiple conditions. They allow us to combine and test these conditions to decide the flow of a program. Understanding the types of logical operators and how they function will enhance your ability to write efficient and effective code in the C programming language.
Understanding the Types of Logical Operators in C
There are three main types of logical operators in C: AND, OR, and NOT. These operators allow you to compare expressions and make decisions based on their results. Each operator has its own specific function and behaviour when working with conditions. To understand them in detail, let's delve into each one individually:
AND, OR, and NOT Operators
The AND, OR, and NOT operators are also known as Boolean operators. They work on boolean expressions (true or false) and return boolean results. Here's a quick explanation of each:
AND Operator (&&): This operator returns true if and only if both conditions being evaluated are true. If either of the conditions is false, the result will be false. It's used in the syntax "expression1 && expression2".
OR Operator (||): This operator returns true if at least one of the conditions being evaluated is true. If both conditions are false, the result will be false. It's used in the syntax "expression1 || expression2".
NOT Operator (!): This operator returns true if the condition being evaluated is false, and false if the condition is true. It's essentially used to negate a given expression. It's used in the syntax "!expression".
Examples of AND, OR, and NOT operators in C:
int a = 10;
int b = 20;
if (a == 10 && b == 20) {
printf("Both conditions are true.\n");
}
if (a == 10 || b == 30) {
printf("At least one condition is true.\n");
}
if (!(a == 20)) {
printf("The condition is false.\n");
}
Bitwise and Logical Operators in C
It's important to differentiate between bitwise and logical operators in C, as they may appear similar but perform different functions. While logical operators work on boolean expressions, bitwise operators work directly on the bits of integer values.
Bitwise operators include:
- Bitwise AND (&)
- Bitwise OR (|)
- Bitwise XOR (^)
- Bitwise NOT (~)
- Left Shift (<
- Right Shift (>>)
Here's an example to distinguish between the logical AND and the bitwise AND:
Logical AND Operator (&&) | Bitwise AND Operator (&) |
Operates on boolean expressions | Operates on the bits of integer values |
Returns a boolean result | Returns an integer value |
Example: (a == 10 && b == 20) | Example: (a & b) |
In conclusion, understanding Logical Operators in C is crucial for making decisions based on multiple conditions in your programs. Take the time to explore and practice with AND, OR, and NOT operators, as well as distinguishing between bitwise and logical operators. This knowledge will help you create more efficient and effective code and ultimately improve your overall programming skills in the C language.
Logical Operators in C Example
Examples are imperative to fully understanding logical operators in C and their practical applications. By examining the use of logical operators in simple programs, exploring their precedence and how they incorporate into conditional statements, you will gain a comprehensive understanding of their functionality.
Using Logical Operators in a Simple Program
Creating a simple program with logical operators enables you to see how they work in practice. Consider the following scenario: You are developing a program to determine if a given number is both positive and even. To accomplish this, you need to use logical operators in an if-statement to evaluate multiple conditions.
The task requires the use of the AND operator in C to ensure both conditions are true simultaneously. Here's a simple C program to achieve this:
Simple program using logical operators:
#include
int main() {
int num = 8;
if (num > 0 && num % 2 == 0) {
printf("The number is positive and even.\n");
} else {
printf("The number is not positive and even.\n");
}
return 0;
}
In the above example, the program checks if the number is positive (num > 0) and if it's even (num % 2 == 0) using the AND operator (&&). If both conditions are true, it prints "The number is positive and even". Otherwise, it prints "The number is not positive and even".
Precedence of Logical Operators in C
It's essential to understand the precedence of logical operators in C to ensure the correctness of your expressions. Operator precedence determines the order in which operators are evaluated, which can affect your code's overall functionality. The order of precedence for logical operators in C is as follows:
- NOT operator (!)
- AND operator (&&)
- OR operator (||)
It's worth noting that when using logical operators with other operators, such as arithmetic or relational operators, the order of precedence may differ. As a general rule, arithmetic and relational operators have higher precedence than logical operators.
Example of precedence in complex expressions:
int a = 10;
int b = 20;
int c = 30;
if (a + b * c > 30 || !(a == 5) && b / a == 2) {
printf("The complex expression is true.\n");
} else {
printf("The complex expression is false.\n");
}
In the given example, arithmetic operations are performed before the logical operations due to their higher precedence. Furthermore, according to precedence rules, the NOT operator (!) is evaluated first, the AND operator (&&) is evaluated next, and finally, the OR operator (||) is evaluated.
Conditional Statements in C Logical Operators
Conditional statements in C allow you to instruct your program to execute specific statements depending on whether the given conditions are true or false. These statements include if, if-else, and else-if. Logical operators are commonly used in conjunction with conditional statements for evaluating multiple conditions and determining the flow of your program.
Here's an example demonstrating the use of logical operators within various conditional statements:
Conditional statements with logical operators:
#include
int main() {
int age = 25;
int height = 180;
if (age >= 18 && height >= 170) {
printf("Eligible for registration.\n");
} else if (age >= 18 && height < 170) {
printf("Not eligible due to height constraint.\n");
} else if (age < 18 && height >= 170) {
printf("Not eligible due to age constraint.\n");
} else {
printf("Not eligible due to both age and height constraints.\n");
}
return 0;
}
In this example, logical operators are used in conjunction with if, else-if, and else statements to evaluate multiple conditions and make decisions based on the values of age and height. Depending on the combination of conditions, a different message is printed to reflect eligibility.
Logical Operators in C Explained
Logical operators in C are the building blocks for evaluating and processing multiple conditions in a program. They play a crucial role in various practical applications such as combining conditional statements, performing error checking, and validating user inputs. By understanding the versatility of logical operators and their use cases, programmers can develop more efficient and effective programs in C.
Practical Applications of Logical Operators
Logical operators offer a wide range of applications in different programming scenarios, especially in combining conditional statements, error checking, and input validation. Let's delve into each area in detail to enhance our understanding of these practical applications.
Combining Conditional Statements
Conditional statements, such as if, if-else, and switch, are vital for implementing logic and controlling the flow of a program. In many cases, you need to evaluate multiple conditions simultaneously or check for the satisfaction of at least one condition among several. Logical operators play an indispensable role in these situations by enabling you to combine and evaluate these conditions within the same conditional statement.
Here are some example use cases:
- Ensuring a student has met both the exam grade and attendance requirements to pass a course.
- Confirming a bank account has sufficient funds and accessibility for funds withdrawal.
- Performing an action when certain conditions are not met (e.g., displaying an error message or re-prompting user input).
Combining logical operators with conditional statements allows programmers to implement complex decision-making logic and improve code efficiency by reducing redundancy and manual comparisons.
Error Checking and Validation with Logical Operators
Error checking and input validation are essential to creating robust and secure software, and logical operators in C contribute significantly to these processes. By using logical operators to verify input data against predefined constraints, programmers can prevent incorrect or malicious data from entering the system and causing errors or vulnerabilities.
Here are some practical applications of logical operators in error checking and validation:
- Validating user inputs in a registration form using logical operators to ensure all required fields are filled and conform to the allowed formats, such as checking for proper email formatting or password strength.
- Detecting invalid data in files or databases by comparing either individual data points or sets of data using logical operators, thus preventing data inconsistencies and incorrect calculations.
- Performing real-time data validation on sensor readings or live data streams in IoT devices, utilizing logical operators to confirm the data is within the expected range and maintaining the system's stability and security.
By incorporating logical operators in error checking and validation processes, developers can increase the reliability, stability, and security of their software, ensuring a better user experience and reducing the likelihood of catastrophic failures or breaches.
Logical Operators in C - Key takeaways
Main types of Logical Operators in C: AND (&&), OR (||), and NOT (!) operators, which are also known as Boolean operators and work on boolean expressions.
Bitwise and Logical Operators distinction: Bitwise operators work directly on the bits of integer values, while logical operators work on boolean expressions.
Precedence order of Logical Operators in C: NOT (!), AND (&&), and OR (||). Arithmetic and relational operators have higher precedence than logical operators.
Logical Operators in C can be used with Conditional Statements like if, if-else, and else-if, allowing evaluation of multiple conditions and determining the program's flow.
Practical applications of Logical Operators in C include combining conditional statements, error checking, and input validation for creating efficient and robust software.
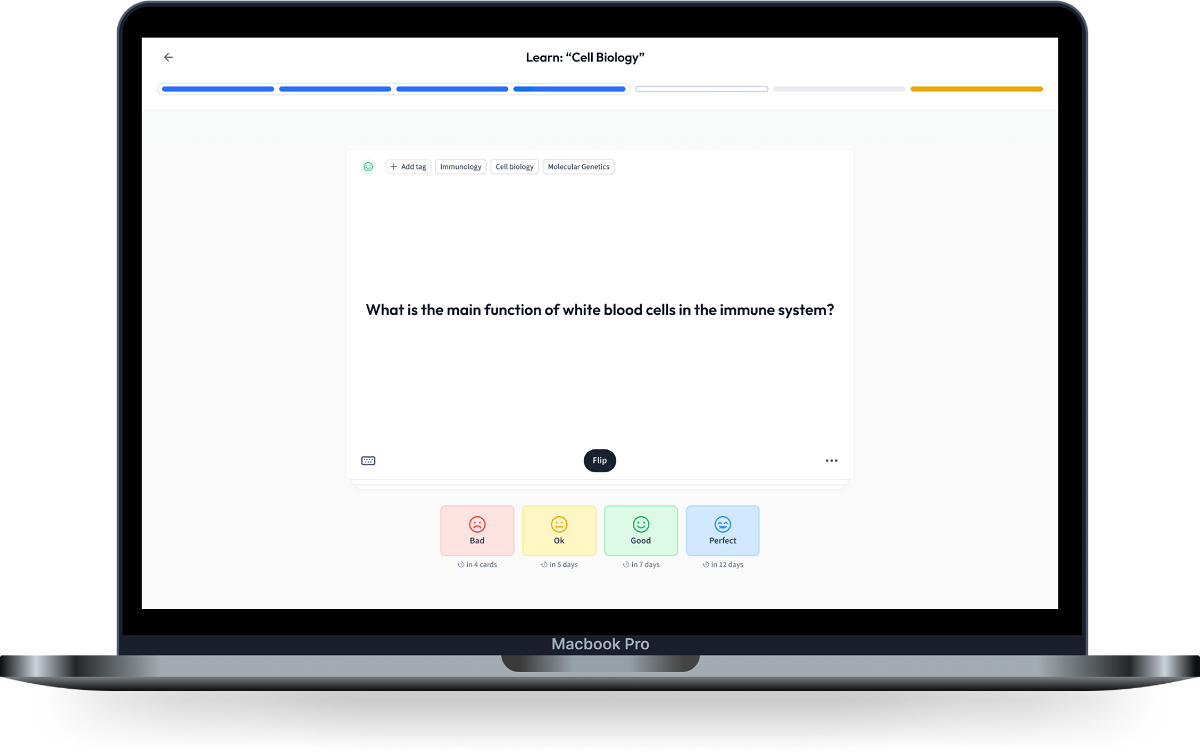
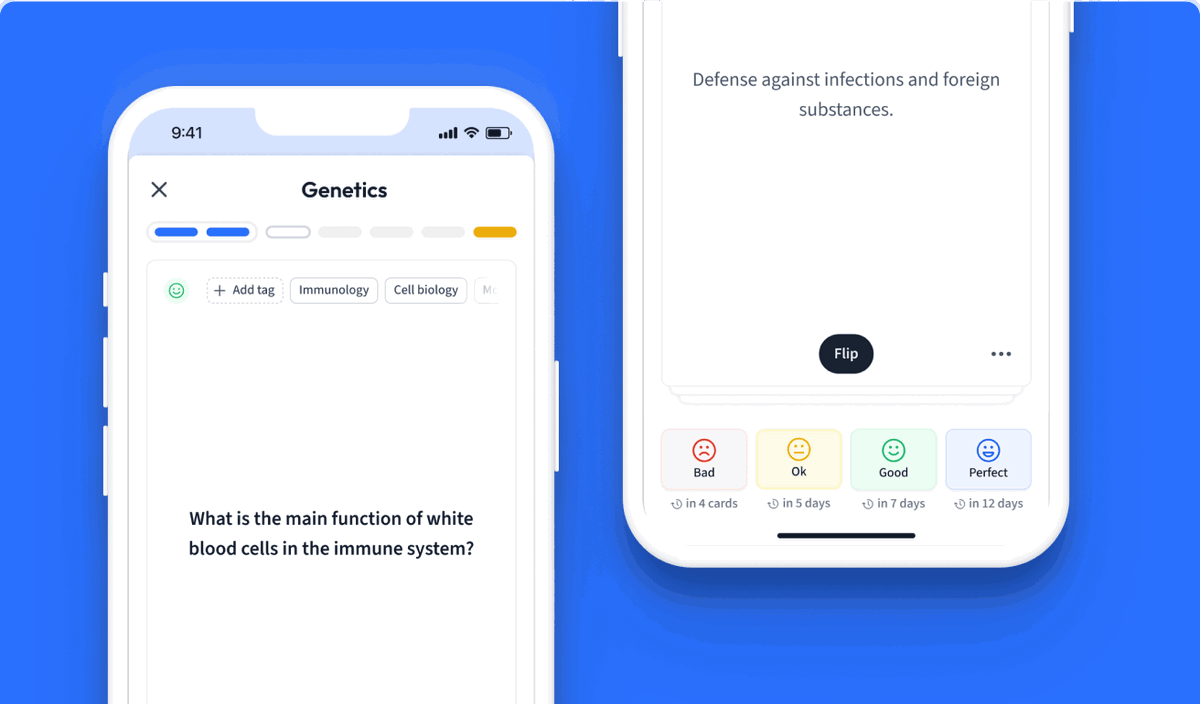
Learn with 11 Logical Operators in C flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Logical Operators in C
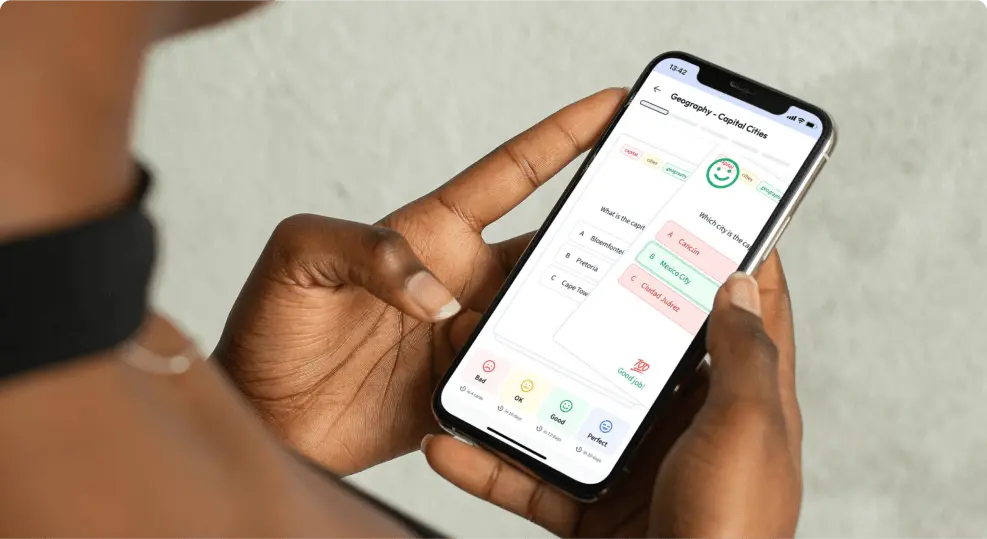
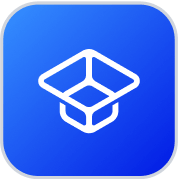
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more