Jump to a key chapter
Fundamentals of Matrix Operations in C
Matrix operations are a fundamental part of computer science, particularly when it comes to data manipulation and analysis. The C programming language is well-suited for performing matrix operations due to its powerful array capabilities and efficient memory management. In this section, we will discuss the basic concepts of matrix operations in C and provide examples to help you understand and implement these operations in your own programs.A matrix is a two-dimensional grid of numbers arranged in rows and columns. In C, matrices are represented as arrays of arrays, where each element of the array corresponds to an entry in the matrix.
Understanding Matrix Operations and Their Applications
Matrix operations are crucial in various fields, such as physics, engineering, computer graphics, and data science. These operations enable the manipulation of multidimensional data and the representation of transformations, among other things. Here are some real-world applications of matrix operations: - Solving simultaneous equations - Computer graphics transformations (scaling, rotation, projection) - Data manipulation, such as filtering and aggregationFor example, suppose you have a set of simultaneous equations that need to be solved. You can represent these equations as matrices and perform matrix operations to find the values of the variables. Similarly, in computer graphics, matrix multiplication is used to transform objects in three-dimensional space to manipulate their position, rotation, and scale.
Essential Concepts for Working with Matrices in C
To effectively perform matrix operations in C, it's essential to understand the following concepts: 1. Arrays: In C, an array is a collection of elements of the same data type, which can be accessed by their index in the collection. Arrays are used to represent matrices. 2. Pointers: Pointers hold the address of variables in memory. They can be used to dynamically allocate memory for matrices and access matrix elements efficiently. 3. Memory allocation: C allows dynamic memory allocation, meaning memory can be allocated at runtime. This flexibility is essential when working with matrices, whose dimensions might not be known during the compilation process. 4. Loop constructs: Loops are critical for performing matrix operations, as they enable traversing and manipulating the matrix elements. C provides various loop constructs such as 'for', 'while', and 'do-while'. Let's look at an example to demonstrate how these concepts can be used to perform a matrix operation, such as matrix addition. Suppose we have two matrices A and B, and we want to add them to get a new matrix C.#include int main() { int rows, columns, i, j; printf("Enter the number of rows and columns: "); scanf("%d %d", &rows, &columns); // Allocate memory for the matrices int A[rows][columns], B[rows][columns], C[rows][columns]; printf("Enter the elements of matrix A: \n"); for(i = 0; i < rows; i++) { for(j = 0; j < columns; j++) { scanf("%d", &A[i][j]); } } printf("Enter the elements of matrix B: \n"); for(i = 0; i < rows; i++) { for(j = 0; j < columns; j++) { scanf("%d", &B[i][j]); } } // Perform matrix addition for(i = 0; i < rows; i++) { for(j = 0; j < columns; j++) { C[i][j] = A[i][j] + B[i][j]; } } printf("The sum of matrices A and B is: \n"); for(i = 0; i < rows; i++) { for(j = 0; j < columns; j++) { printf("%d\t", C[i][j]); } printf("\n"); } return 0; }
Executing Matrix Operations in C using Functions
Matrix operations, such as addition, subtraction, and multiplication, can be implemented in C using functions. Functions modularise the code, enhance its readability, and allow for code reusability. When implementing matrix operation functions, consider the following design principles:
1. Pass matrices as function arguments.
2. Use pointers and dynamic memory allocation to handle matrices of varying dimensions.
3. Ensure proper function signatures to account for variable dimensions. Let's delve deeper into the implementation details for each matrix operation.
Matrix Addition & Subtraction: These operations are element-wise operations, i.e., each element in the resulting matrix is the sum or difference of corresponding elements in the input matrices. To implement functions for matrix addition and subtraction, follow these steps: - Define a function with a descriptive name, like `add_matrices` or `subtract_matrices`. - Pass the input matrices, their dimensions (i.e., rows and columns), and a pointer to the result matrix as function parameters. - Use nested loops to traverse the input matrices. - Perform element-wise addition or subtraction for each element in the matrices.
Matrix Multiplication:To multiply two matrices, the number of columns of the first matrix must equal the number of rows of the second matrix. The resulting matrix has the same number of rows as the first matrix and the same number of columns as the second matrix. To implement a function for matrix multiplication, follow these steps:
- Define a function with a suitable name, like `multiply_matrices`.
- Pass the input matrices, their dimensions (including the common dimension), and a pointer to the result matrix as function parameters. - Use three nested loops to traverse the input matrices and perform matrix multiplication.
- Calculate each element in the resulting matrix by summing the products of the corresponding elements of the input matrices.
Implementing functions for matrix operations in C: void add_matrices(int **A, int **B, int **result, int rows, int columns) { for(int i = 0; i < rows; i++) { for(int j = 0; j < columns; j++) { result[i][j] = A[i][j] + B[i][j]; } } } void subtract_matrices(int **A, int **B, int **result, int rows, int columns) { for(int i = 0; i < rows; i++) { for(int j = 0; j < columns; j++) { result[i][j] = A[i][j] - B[i][j]; } } } void multiply_matrices(int **A, int **B, int **result, int rowsA, int common, int columnsB) { for(int i = 0; i < rowsA; i++) { for(int j = 0; j < columnsB; j++) { result[i][j] = 0; for(int k = 0; k < common; k++) { result[i][j] += A[i][k] * B[k][j]; } } } }
Example Code for Matrix Operations Using Functions
This example demonstrates how to use the functions defined above to perform matrix operations, such as addition, subtraction, and multiplication in C.
#include
#include
void add_matrices(int **A, int **B, int **result, int rows, int columns);
void subtract_matrices(int **A, int **B, int **result, int rows, int columns);
void multiply_matrices(int **A, int **B, int **result, int rowsA, int common, int columnsB);
int main() { // Define matrix dimensions and allocate memory for matrices. int rowsA = 3, columnsA = 2, rowsB = 2, columnsB = 3; int **A, **B, **result; A = (int **)malloc(rowsA * sizeof(int *)); for(int i = 0; i < rowsA; i++) A[i] = (int *)malloc(columnsA * sizeof(int)); B = (int **)malloc(rowsB * sizeof(int *)); for(int i = 0; i < rowsB; i++) B[i] = (int *)malloc(columnsB * sizeof(int)); result = (int **)malloc(rowsA * sizeof(int *)); for(int i = 0; i < rowsA; i++) result[i] = (int *)malloc(columnsB * sizeof(int)); // Input values for matrices A and B. // ...
// Perform matrix operations using functions.
// For example: matrix multiplication multiply_matrices(A, B, result, rowsA, columnsA, columnsB);
// Output or further process the resulting matrix. // ...
return 0; }
Tips for Efficient and Accurate Function Implementation
When implementing matrix operation functions in C, follow these best practices to ensure efficient and accurate code:
1. Use meaningful function names to enhance code readability.
2. Clearly document the purpose and parameters of each function.
3. Always validate matrix dimensions before performing operations.
4. Use consistent array indexing and loop variable naming conventions.
5. Always handle memory allocation and deallocation properly to avoid memory leaks. 6. Write test cases to ensure function correctness and handle edge cases. By following these best practices and leveraging the power of functions in C, you can implement efficient and accurate matrix operation functions in your programs.
Matrix Operations in C++: A Comparison
Matrix operations in C++ can be implemented using classes, which offer an object-oriented and more structured approach compared to the procedural techniques in C. A class is a user-defined data type that bundles data members and member functions into a single unit. By designing a matrix class, you encapsulate all the matrix-related data and operations within a structured class hierarchy. When creating a matrix class, certain essential components should be included:
1. Class data members:
- Matrix data (stored as a two-dimensional vector or a dynamic array)
- Number of rows
- Number of columns
2. Class member functions:
- Constructors
- Destructor
- Overloaded operators (e.g. +, -, *, =)
- Accessor and mutator functions
- Additional utility functions (e.g. determinant, inverse, transpose)
Basic matrix class in C++ using vector: ```cpp #include
Advantages of Using Classes for Matrix Operations in C++
Using classes for matrix operations offers various advantages over the traditional procedural approach in C:
1. Encapsulation: Grouping matrix data and operations within a single class ensures proper data hiding and encapsulation.
2. Modularity: Separating matrix functionality into a dedicated class allows for better modularity in your code.
3. Code Reusability: Implemented matrix operations can be easily reused across different projects and applications.
4. Easier Maintenance: Object-oriented programming using classes simplifies code maintenance and debugging.
5. Abstraction: Using classes abstracts away low-level details of matrix operations, making the code more comprehensible.
6. Extensibility: When new matrix operations are needed, additional functions can be easily added to the existing matrix class.
Matrix Operations with Vectors in C++
C++ standard library offers `std::vector`, a dynamic array container that can be used for handling matrices. Vectors are more versatile and less error-prone compared to raw pointers and arrays in C. To implement matrix operations using C++ vectors, follow these guidelines:
1. Initialize the matrix as a two-dimensional vector (e.g., `std::vector<:vector>>`).
2. Use vector class functions (e.g., `push_back`) and syntax (e.g., `resize`) for memory allocation and resizing.
3. Use range-based 'for' loops or C++ algorithms (e.g., `std::transform`) for more elegant matrix traversal.
Example of initializing a matrix using C++ vectors: ```cpp #include
Differences in Approach Between C and C++ Vector Implementations
Comparing the use of C++ vectors against C arrays or pointers to handle matrices reveals key differences:
1. Memory Management: C++ vectors handle memory allocation and deallocation automatically, reducing the risk of memory leaks and errors.
2. Bounds Checking: Vectors provide optional bounds checking, increasing code safety and robustness.
3. Dynamic Resizing: Vectors offer dynamic resizing, simplifying matrix size modifications at runtime.
4. Improved Syntax: Vectors allow for cleaner and more consistent syntax than raw pointers and arrays in C.
5. Standard Algorithms: C++ offers standard algorithms, such as `std::transform`, that can be applied to vectors for more efficient matrix operations.
Matrix Operator Overloading in C++
C++ allows overloading operators, providing user-defined behavior for common arithmetic operations, such as +, -, *, and =. This feature can be used to simplify the syntax and enhance the readability of matrix operations. To implement matrix operator overloading, follow these steps:
1. Define a member function of the matrix class that corresponds to the desired operator, such as `operator+`, `operator-`, `operator*`, or `operator=`.
2. Implement the required matrix operation (addition, subtraction, multiplication, or assignment) within the operator function. 3. Return the result of the operation as an instance of the matrix class.
Example of matrix operator overloading for addition in C++: class Matrix { //... other class members public: Matrix operator+(const Matrix &other) { //... validate matrix dimensions Matrix result(rows, columns); for (int i = 0; i < rows; i++) { for (int j = 0; j < columns; j++) { result.data[i][j] = data[i][j] + other.data[i][j]; } } return result; } };
The Benefits of Overloading Matrix Operators
Implementing matrix operator overloading in C++ offers several advantages:
1. Intuitive Syntax: Operator overloading allows for cleaner and more intuitive syntax for matrix operations, resembling the mathematical notation.
2. Improved Readability: Operator overloading improves code readability by abstracting away the complexity of matrix operations from the user.
3. Efficiency: Overloaded operators can maintain performance similar to corresponding function calls, especially in the case of inline functions or compiler optimizations.
4. Consistency: Operator overloading promotes consistency with built-in C++ data types and operations.
5. Customizability: Users can define specific behavior for overloaded operators, tailoring them to their needs and requirements. With the numerous benefits of using C++ features such as classes, vectors, and operator overloading, implementing efficient and intuitive matrix operations becomes more manageable and streamlined.
Mastering Matrix Operations in C Explained
To master matrix operations in C, it is crucial to adhere to best practices. Following these guidelines will lead to efficient, reliable, and maintainable code.
Choosing the Right Data Structures and Algorithms: Best Practices
Selecting the most suitable data structures and algorithms for matrix operations will significantly impact your program's efficiency. Consider the following factors when making your choice: - Data Structure: Using the appropriate data structure will optimise memory usage and computational complexity. Common options include static arrays, dynamic arrays, and pointers to pointers. - Algorithm Complexity: Choose algorithms with low time and space complexity to ensure efficient matrix operations. Keep in mind the Big-O notation to analyse your chosen algorithm's performance. - Algorithm Scalability: Opt for algorithms that can handle various matrix sizes and remain efficient even with large matrices or complex operations.
When dealing with sparse matrices (matrices with a significant number of zero elements), consider implementing compressed row storage (CRS) or compressed column storage (CCS) to save memory and further optimise computational complexity.
Common Pitfalls and How to Avoid Them
Becoming proficient in matrix operations in C entails recognising and avoiding common pitfalls. Some frequent issues and their solutions include:
- Incorrect matrix dimensions: Always ensure that matrix dimensions are compatible before attempting operations (e.g., the number of columns in the first matrix should equal the number of rows in the second matrix for multiplication). Validate dimensions and handle errors accordingly.
- Memory leaks: Properly allocate and deallocate memory when using dynamic arrays or pointers to avoid memory leaks. Use the `malloc` and `free` functions consistently and consider utilising memory debugging tools.
- Array indexing errors: Be mindful of zero-based array indexing in C and avoid off-by-one errors. Use loop variables consistently and ensure your loop boundaries are correct. - Inefficient nested loops: Arrange nested loops optimally, especially when implementing matrix multiplication. Consider leveraging loop unrolling or parallelisation techniques to further boost performance.
Developing Your Skills in Matrix Operations in C
Enhancing your matrix operations skills in C involves understanding relevant theories, practising implementation, and learning from helpful resources.
Online Resources, Tutorials, and Exercises to Improve Your Understanding
Expanding your knowledge and skills in matrix operations in C requires dedication and persistence. Leverage these online resources, tutorials, and exercises to advance your understanding:
1. Documentation: Study the official C language documentation and relevant materials to gain a strong theoretical foundation in matrix operations, data structures, and algorithms.
2. Online Courses: Enrol in online courses, such as Coursera, edX, or Udacity, offering computer science and C programming classes. These courses often include lessons and assignments on matrix operations.
3. Video Tutorials: Watch video tutorials on YouTube or other platforms to gain insights from experienced programmers who explain and demonstrate how to perform matrix operations in C effectively.
4. Coding Challenges: Participate in coding challenges and competitions, such as LeetCode or HackerRank, for hands-on practice with matrix operations and other topics in C programming.
5. Books and Articles: Read books, articles, and blog posts focused on C programming, computer science, and matrix operations. Prominent titles include "C Programming Absolute Beginner's Guide" by Greg Perry and "Matrix Computations" by Gene H. Golub and Charles F. Van Loan.
6. Forums and Communities: Join programming forums, online communities, or social media groups where professionals and enthusiasts discuss matrix operations in C and share their knowledge and experiences to support your learning journey. Utilise these resources consistently and practice implementing matrix operations to hone your skills and become proficient in C programming.
Matrix Operations in C - Key takeaways
Matrix Operations in C - A crucial aspect in various fields like computer graphics, physics simulations, and numerical analysis.
Matrix operations in C++ - Advantage of using classes, vectors, and operator overloading for these operations, which improves code readability, maintainability, and efficiency.
Matrix Operations in C using function - Implement matrix addition, subtraction, and multiplication functions, which modularise the code and allow code to be reused across different projects and applications.
Matrix operations with vector in C++ - Using C++ standard library vectors, dynamic array containers can handle memory allocation and deallocation automatically, reducing the risk of memory leaks and errors.
Matrix overloading operator in C++ - Simplifies the syntax and enhances code readability for matrix operations through user-defined behavior for arithmetic operations.
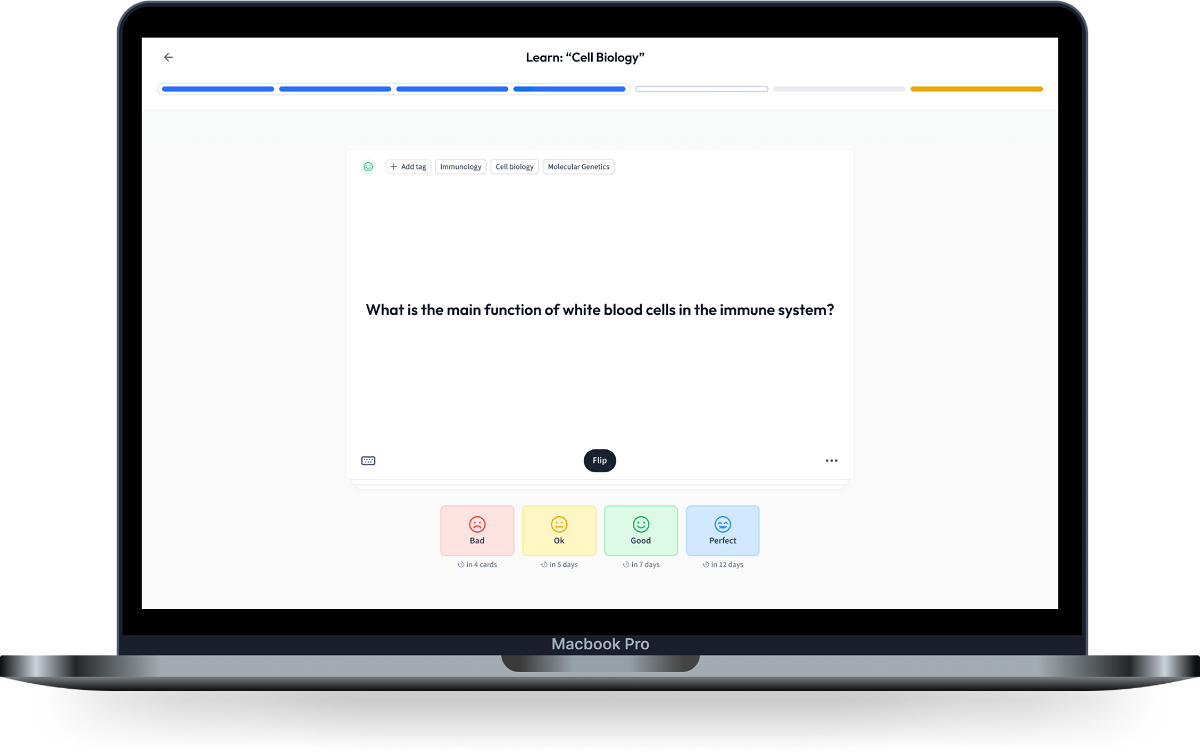
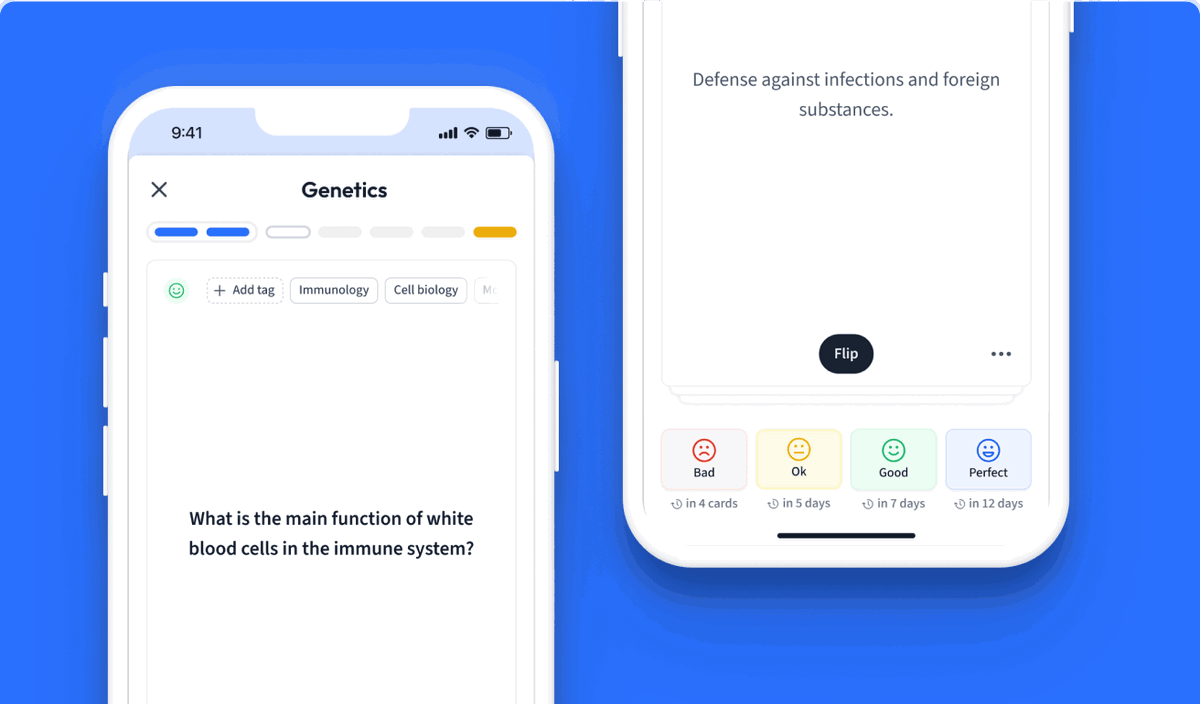
Learn with 13 Matrix Operations in C flashcards in the free StudySmarter app
Already have an account? Log in
Frequently Asked Questions about Matrix Operations in C
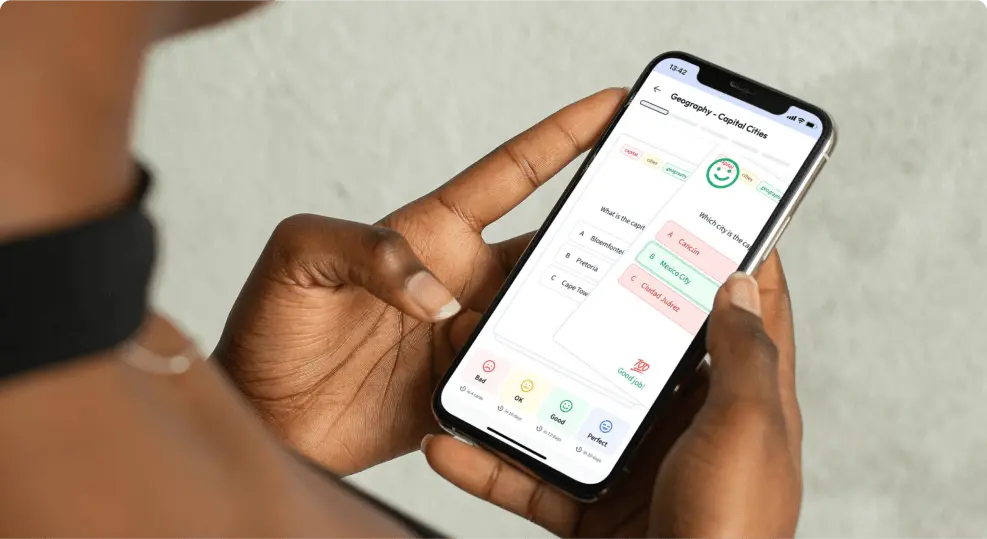
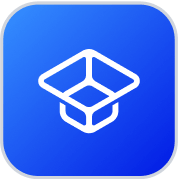
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more