Jump to a key chapter
Definition of One Dimensional Arrays in C
One Dimensional Arrays in C are a fundamental data structure that allow you to store a collection of elements of the same type in a contiguous block of memory. This essentially means that when you declare an array, you're creating a sequence of variables, which enables you to organize data more efficiently in your programs. Think of it as a long row of boxes, each box capable of storing a particular value, where the entire row represents the array.Using one-dimensional arrays can significantly enhance the versatility and efficiency of your code, especially when dealing with multiple data values that belong together, like test scores or temperature readings for a week.
Characteristics of One Dimensional Arrays
One-dimensional arrays have specific characteristics that make them unique and essential in programming:
- Fixed Size: Once an array is declared, its size cannot be changed. This means you need to specify the initial size based on your expected requirements.
- Indexing: Elements in an array are accessed using indices starting from 0, ranging up to n-1 where n is the size of the array.
- Homogeneous Elements: All elements within the array must be of the same data type, such as integers, floats, or characters.
- Contiguous Memory: Elements are stored in a sequence, allowing efficient access and manipulation.
One Dimensional Array: An array that allows you to store multiple items of the same data type in a contiguous memory block, accessed by a single index.
Consider the following example of a one-dimensional array in C, which stores the scores of five students:
int scores[5] = {85, 92, 78, 90, 88};This code snippet declares an array named scores, which can hold five integer values, representing student scores.
When accessing array elements, remember to always use valid indices to avoid undefined behavior which can lead to potential bugs.
Understanding One Dimensional Arrays in C
When learning C programming, mastering One Dimensional Arrays is crucial as they serve as a foundational component for managing and organizing data. A one-dimensional array in C allows you to store multiple items of the same type sequentially in memory. By doing so, it improves program efficiency and data management.
How to Declare and Initialize One Dimensional Arrays
Declaration: To declare an array, specify the data type, the array name, and the number of elements it will hold. This syntax is essential for creating arrays that serve your program's needs.
datatype arrayName[arraySize];Initialization: When initializing, you can assign values at the time of declaration, either specifying all elements, some elements, or leaving some for future assignment.
int numbers[5] = {1, 2, 3, 4, 5};You can also declare without initialization, which will fill the unassigned spots with garbage values:
int numbers[5];
One Dimensional Array Program in C
Creating a program that utilizes One Dimensional Arrays in C can significantly simplify your code when handling multiple data values of the same type. Such programs are widely used in computations, data storage tasks, or repetitive operations over a list of items.
Writing a Program Using One Dimensional Arrays
To start coding with one-dimensional arrays, it is essential to understand how to implement, access, and manipulate them within a C program. Let’s examine the basic steps:
- Declare and Initialize: Clearly define your array’s data type, provide it a name, and specify its size.
- Access Elements: Use the array indices to access and modify each element stored in the array.
- Iterate Through Elements: Utilize loops such as for loops to run through the array, enabling you to perform operations like summing all values or finding maximum values.
One Dimensional Array in C Example
In C programming, One Dimensional Arrays can be utilized to efficiently store and manage homogeneous data. Let's explore an example to see how you can declare, initialize, and manipulate arrays.
Here is a simple code example illustrating a one-dimensional array in C:
#includeThis simple program declares an integer array numbers with 5 elements, initializes it with specific values, and prints each element using a loop.int main() { int numbers[5] = {10, 20, 30, 40, 50}; for (int i = 0; i < 5; i++) { printf(%d, numbers[i]); } return 0; }
How to Pass One Dimensional Array to Function in C
To pass a one-dimensional array to a function in C, you must provide the array name and specify its size within the function signature.
- Function Declaration: When declaring the function that takes an array as an argument, specify the data type and use brackets to denote an array.
- Function Call: Simply pass the array name when calling the function, as the array's reference is sufficient.
- Function Definition: Within the function's body, the array parameter can be used just like any other array variable.
#includevoid display(int num[], int size); int main() { int numbers[3] = {5, 10, 15}; display(numbers, 3); return 0; } void display(int num[], int size) { for (int i = 0; i < size; i++) { printf(%d, num[i]); }}
When you pass an array to a function, you're really passing the address of the first element of the array. This implies that the function can alter the original array elements, a behavior known as 'passing by reference.' However, this does not mean passing the entire array needlessly increases memory use in functions, as only the reference is passed, ensuring efficiency.
Advantages of One Dimensional Arrays in C
Using one-dimensional arrays offers several benefits that amplify the efficacy of your C programs:
- Memory Efficiency: Arrays are designed to consume less memory due to their contiguous storage layout, which allows for rapid access and processing of elements.
- Data Management: Easily manage and organize similar data points, such as monthly sales numbers or daily weather readings.
- Efficient Algorithm Implementation: Use arrays to simplify the execution of various algorithms, such as sorting and searching techniques.
- Iterative and Recursive Operations: Implement loops and recursive functions more effectively using arrays, as they can easily iterate over indexed data.
One Dimensional Arrays in C - Key takeaways
- Definition of One Dimensional Arrays in C: An array that stores multiple items of the same data type in a contiguous memory block, accessed by a single index.
- Characteristics: Fixed size after declaration, zero-based indexing, homogeneous elements, contiguous memory storage.
- Declaration and Initialization: Use syntax
datatype arrayName[arraySize];
for declaration. Initialize withint numbers[5] = {1, 2, 3, 4, 5};
- Example:
int scores[5] = {85, 92, 78, 90, 88};
declares an array named scores for student scores. - Pass to Function: Pass array name and size in function signature. Example:
void display(int num[], int size);
- Advantages: Enhances memory efficiency, data management, algorithm implementation, and iterative operations.
Learn faster with the 23 flashcards about One Dimensional Arrays in C
Sign up for free to gain access to all our flashcards.
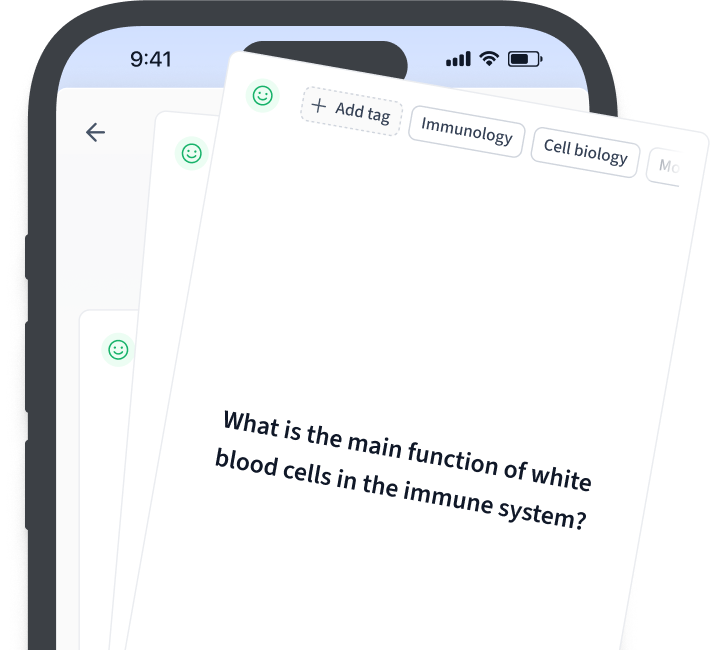
Frequently Asked Questions about One Dimensional Arrays in C
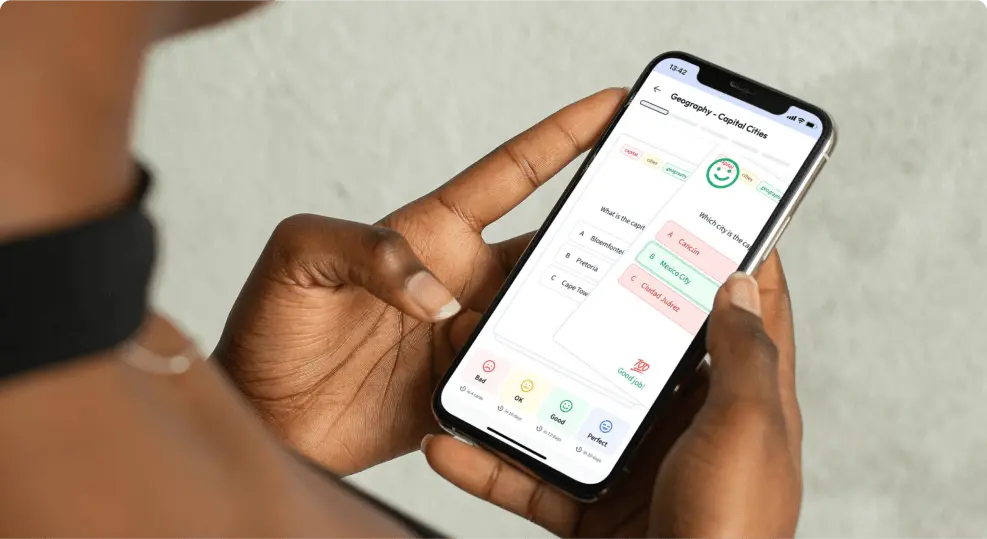
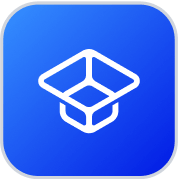
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more