Jump to a key chapter
Parameter Passing Definition in Computer Science
In computer science, Parameter Passing is a crucial concept. It refers to the way arguments are passed to functions or methods in programming languages. Parameters act as inputs to functions, allowing you to pass data and dictate how that data is utilized within a specific scope.
Understanding Parameter Passing in Programming
To comprehend parameter passing, you need to understand the main techniques used in programming:
- Pass by Value: A copy of the actual parameter is passed, so changes made to the parameter inside the function do not affect the original value.
- Pass by Reference: A reference to the actual parameter is passed, allowing changes made to the parameter inside the function to affect the original value.
These two methods can significantly impact how your program executes and its output. Each method has its advantages and particular use cases, playing a critical role in programming design strategies.
Parameter: A parameter is a variable used in a function or method definition, allowing the function to accept inputs when it is called.
An example in Python illustrates these two techniques:
# Pass by Value Example def add_ten(value): value += 10 return value number = 5 print(add_ten(number)) # Outputs 15 print(number) # Outputs 5 (original value unchanged) # Pass by Reference Example def append_to_list(lst): lst.append(10) my_list = [1, 2, 3] append_to_list(my_list) print(my_list) # Outputs [1, 2, 3, 10]
Some advanced programming languages support additional parameter passing techniques such as Pass by Name and Pass by Need. Pass by Name substitutes the expressions directly at runtime, which can result in more computationally expensive operations. Pass by Need, also known as lazy evaluation, delays the evaluation of an expression until its value is actually required, optimizing memory usage in certain cases.
Basic Concepts for Students
A few elementary points are vital for grasping parameter passing effectively:
- Understand the function signature, which includes the function name and its parameters.
- Distinguish between actual parameters (arguments provided to the function) and formal parameters (those described in the function definition).
- Learn the scope of a variable, as parameters might have a limited reach within the function body.
- Recognize the impact of parameter passing on performance and memory usage, especially in large-scale applications.
Always test different parameter passing methods to better understand their impact, particularly with large data structures or resource-intensive operations.
Parameter Passing Strategies Explained
Parameter Passing is a significant concept in programming that influences how functions receive inputs and execute tasks. Understanding different strategies will enhance your coding skills and optimize your program’s efficiency.
Call by Value and Call by Reference
When you pass parameters to a function, it can be done in several ways, with the most common being:
- Call by Value: The actual parameter's value is copied and passed to the function, ensuring that any changes made inside the function do not alter the original parameter values.
- Call by Reference: Instead of passing a copy, a reference to the actual parameter is passed, meaning changes inside the function affect the parameter's original value in the caller’s scope.
Call by Value: A method of parameter passing where a copy of the actual parameter is sent to the function, safeguarding the original data from modifications.
Call by Reference: A method where a reference to the actual parameter is sent, allowing direct modification of the original data by the function.
Beyond Call by Value and Call by Reference, certain languages offer advanced techniques like Call by Sharing, also known as Call by Object, mainly in languages like Python. Here, parameters act as references to the actual objects, and while the reference itself remains unchanged, the object's mutable aspects can be altered.
Practical Examples of Parameter Passing Techniques
Let’s explore examples to better understand how parameter passing works:
Consider these Python examples which illustrate the difference between the two strategies:
# Pass by Value-like behaviordef square_number(num): num = num ** 2 return numoriginal_value = 4result = square_number(original_value)print(result) # Outputs 16print(original_value) # Outputs 4, original value unchanged# Pass by Reference-like behaviordef modify_list(lst): lst.append(4)sample_list = [1, 2, 3]modify_list(sample_list)print(sample_list) # Outputs [1, 2, 3, 4], original list modified
Understanding these concepts is critical when dealing with complex data structures or optimizing your code performance. You often need to choose the appropriate method based on the problem requirements and expected behavior.
Choosing the right parameter passing strategy can prevent unexpected side effects and bugs in your program.
Importance of Parameter Passing
Parameter passing is a foundational concept in programming that plays a critical role in software development. It determines how arguments are handled when functions are called, affecting both execution and overall program performance. A clear understanding of parameter passing is crucial for writing efficient and effective code.
Role in Function Execution
Parameter passing influences how functions operate within a program:
- Data Transfer: Parameters allow functions to receive inputs, effectively transferring data to be processed within the function's scope.
- Encapsulation: By passing parameters, you can encapsulate the logic within functions, making programs more modular and easier to manage.
- Reusability: Functions can perform diverse tasks based on different input parameters, enhancing their reusability across various parts of a program.
- Abstraction: Parameters enable abstraction, allowing you to focus on the function's logic without concern for the specifics of how it will be executed with different arguments.
Consider how parameter passing styles vary between programming languages: Java uses call by value for objects, but the object references are what's actually passed, allowing for modifications to the object's contents. In contrast, languages like C++ offer explicit call by reference, using reference variables to directly manipulate input parameters.
Let's explore an example in C++ to see parameter passing at work:
// Call by Reference in C++void swap(int &a, int &b) { int temp = a; a = b; b = temp;}int main() { int x = 10; int y = 20; swap(x, y); // x becomes 20, y becomes 10}
How Parameter Passing Impacts Performance
Parameter passing can significantly affect a program's performance:
- Memory Usage: Passing large objects by value can increase memory usage due to copying, whereas passing by reference avoids this by using pointers or references.
- Execution Speed: Copying values can slow down function calls, especially with large datasets, affecting program efficiency.
- Side Effects: Call by reference can lead to unintended side effects if the function modifies the input parameter unexpectedly.
- Optimization: Choosing the right parameter passing method can optimize memory and processing resources, essential for high-performance applications.
In high-performance computing, using pointer-based parameter passing in languages like C minimizes overhead and promotes efficient resource usage, especially in algorithms with frequent function calls. Understanding the underlying memory allocation and data management is key to leveraging these techniques effectively.
When dealing with large datasets, consider passing references or pointers to minimize memory consumption and enhance execution speed.
Parameter Passing Methods Comparison
In programming, understanding the different parameter passing methods is vital for writing effective and efficient code. Various languages implement these methods in distinct ways, impacting both the operation and performance of programs.
Pros and Cons of Different Techniques
Let's explore the advantages and disadvantages of different parameter passing techniques:
- Pass by Value: Computers make a copy of the argument's value, which ensures the original data remains unchanged, offering data security. However, downsides include increased memory usage and slower execution when dealing with large data.
- Pass by Reference: This allows direct manipulation of the original data, which is more memory efficient and often faster for large datasets. The disadvantage lies in potential unintended side effects if the function alters the argument's value unexpectedly.
- Pass by Sharing: Used mainly in object-oriented languages, this method is similar to passing references. It benefits from not copying the entire object, making it efficient, but changes to the object's state may lead to unpredictable behavior.
Consider this JavaScript example showing Pass by Value vs. Pass by Reference:
// Pass by Valuefunction modifyPrimitive(num) { num = 10;}let originalNumber = 5;modifyPrimitive(originalNumber);console.log(originalNumber); // Outputs 5, unchanged// Pass by Referencefunction modifyObject(myObj) { myObj.value = 10;}let originalObject = {value: 5};modifyObject(originalObject);console.log(originalObject.value); // Outputs 10, modified
Choosing between value and reference passing depends greatly on the specific circumstances and requirements of the task at hand, especially when handling large data structures.
Choosing the Right Parameter Passing Method
When deciding on the appropriate parameter passing method, consider these factors:
- Data Size: For large objects or datasets, passing by reference is preferable to avoid unnecessary data duplication.
- Function Scope: If alterations to the original data are not desirable, pass by value to ensure data integrity.
- Performance: Consider the trade-offs; while passing by value may be safer, it can also be slower and more memory-intensive.
In functional programming languages like Haskell, techniques such as lazy evaluation come into play. Lazy evaluation, or delayed execution, doesn't compute parameter values until necessary, optimizing performance by avoiding needless calculations.
In concurrent applications, be cautious with pass by reference to prevent race conditions or data corruption.
Parameter Passing - Key takeaways
- Parameter Passing Definition: In computer science, parameter passing is the process of passing arguments to functions or methods, serving as inputs to facilitate data manipulation within a function's scope.
- Parameter Passing Strategies: Includes various methods such as Pass by Value (copying values) and Pass by Reference (referencing the original data), with advanced strategies like Pass by Name and Pass by Need.
- Importance of Parameter Passing: Affects software development by influencing function execution, data encapsulation, reusability, and abstraction.
- Practical Examples: In Python, Pass by Value involves copying the value, while Pass by Reference allows the function to modify the original parameter directly.
- Parameter Passing Techniques: Understanding scope, memory management, and potential side effects is crucial for efficient coding and performance optimization.
- Comparing Methods: Different methods, like Call by Value and Call by Reference, offer trade-offs in data security, memory efficiency, and execution speed, necessitating careful selection based on task requirements.
Learn faster with the 25 flashcards about Parameter Passing
Sign up for free to gain access to all our flashcards.
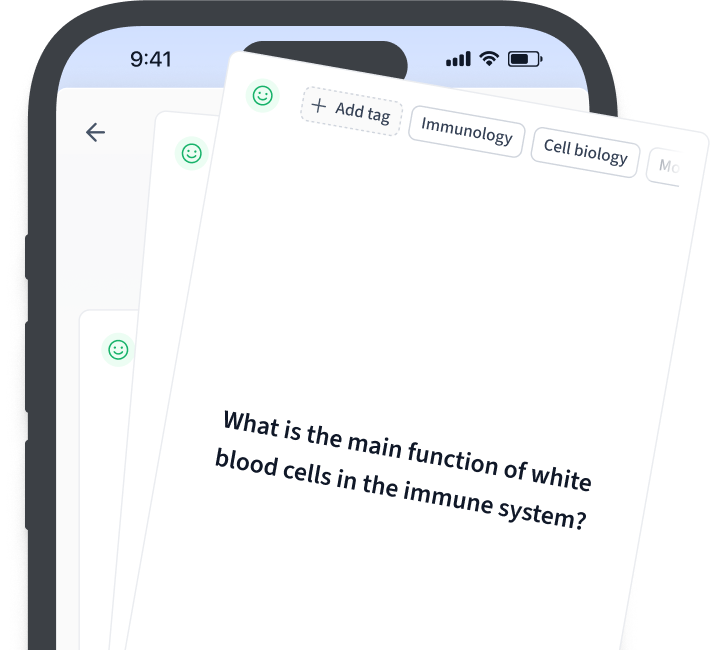
Frequently Asked Questions about Parameter Passing
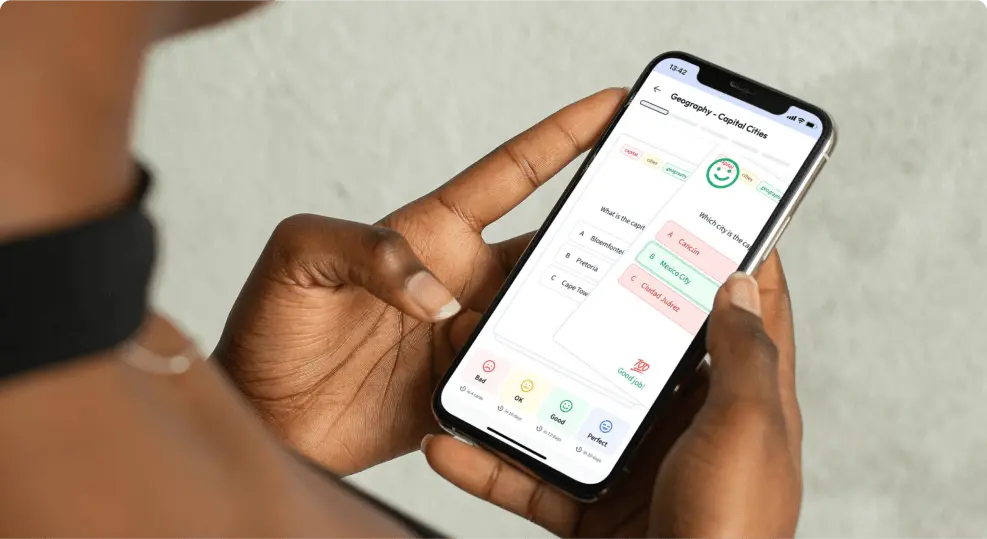
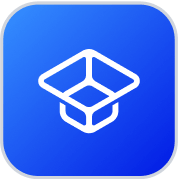
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more