Jump to a key chapter
What is Plotting in Python?
Plotting in Python refers to the creation of visual representations of data, making it easier to understand, interpret, and present. Python offers a wide range of libraries for plotting, such as Matplotlib, Seaborn, and Plotly, allowing for the creation of complex graphs and charts with relative ease.
Understanding the Basics of Plotting in Python
At the heart of plotting in Python is the concept of transforming numerical and categorical data into visual formats. These visuals range from simple line graphs to comprehensive 3D charts. Libraries like Matplotlib serve as a foundation for plotting, offering a flexible interface for creating a wide array of plots. For those venturing into data science or analytics, mastering plotting is key to effectively communicate findings.
Matplotlib: A plotting library for the Python programming language and its numerical mathematics extension, NumPy. It provides an object-oriented API for embedding plots into applications.
import matplotlib.pyplot as plt # Sample data x = [1, 2, 3, 4] y = [10, 20, 25, 30] plt.plot(x, y) plt.show()This basic example shows how to plot a line graph using Matplotlib. Here,
x
represents the horizontal axis and y
the vertical. The plt.plot()
function creates the plot, while plt.show()
displays it. Matplotlib is often the first plotting library learners encounter due to its simplicity and extensive documentation.
Key Benefits of Plotting in Python for Students
Plotting in Python is not just about creating visuals; it’s an essential skill that offers numerous benefits for students entering the field of computer science, especially those interested in data analysis and visualization.
- Enhanced Data Comprehension: Creating graphs and charts aids in better understanding complex datasets, highlighting trends and anomalies.
- Improved Presentation Skills: Being able to visually present data makes it more accessible to non-technical audiences, improving communication skills.
- Increased Efficiency in Data Analysis: Visual representations can quickly convey insights that might take longer to explain using text or numbers alone.
- Creative Visualisation Opportunities: With various libraries at disposal, students can experiment with different styles and aesthetics, encouraging creativity.
One fascinating aspect of plotting in Python is the ability to manipulate the aesthetics of plots extensively. For example, Matplotlib allows users to adjust colours, line styles, and markers, adding annotations, and even embedding plots within GUI applications for interactive data exploration. This flexibility makes Python an invaluable tool for both quick data analysis and the development of complex visualisation software.
Plotting in Python Techniques
Plotting in Python Techniques offer a dynamic way to visualise data, extending from simple 2D plots to intricate 3D visualisations. By harnessing libraries like Matplotlib, Plotly, and others, Python becomes a powerful tool for data analysis and presentation.
Introduction to Plotting in 3D Python
Plotting in 3D within Python entails generating three-dimensional graphs, offering a more in-depth perspective on data that cannot be captured as effectively in two dimensions. This technique is particularly useful in fields such as physics, mathematics, biology, and finance, where visualising multidimensional data sets can unveil patterns and correlations not evident in traditional 2D plots.
3D Plotting: A method of data visualisation that represents information in three axes (X, Y, and Z), enhancing the depiction of complex relationships within data.
from mpl_toolkits.mplot3d import Axes3D import matplotlib.pyplot as plt fig = plt.figure() ax = fig.add_subplot(111, projection='3d') # Sample data x = [1, 2, 3, 4, 5] y = [5, 6, 7, 8, 9] z = [2, 3, 4, 5, 6] ax.scatter(x, y, z) plt.show()This example demonstrates how to create a simple 3D scatter plot using Matplotlib. The
Axes3D
object from the mplot3d
toolkit enables 3D plotting, showcasing how Python can be used for advanced data visualisation. When beginning with 3D plotting, starting with simple scatter plots or wireframes can help in understanding how to manipulate data in three dimensions.
How to Create a Scatter Plot in Python
Scatter plots are a type of plot that display values for typically two variables for a set of data. The data are displayed as a collection of points, each having the value of one variable determining the position on the horizontal axis and the value of the other variable determining the position on the vertical axis. This type of plot is highly effective for visualizing the distribution of data points and observing any correlational patterns that exist between the two variables.
import matplotlib.pyplot as plt # Sample data x = [5, 20, 15, 25, 10] y = [8, 21, 32, 45, 6] plt.scatter(x, y) plt.title('Sample Scatter Plot') plt.xlabel('X Axis Label') plt.ylabel('Y Axis Label') plt.show()This simple example illustrates how to create a scatter plot in Python using Matplotlib. By calling
plt.scatter()
, points are plotted according to their x and y values, making patterns and trends within the data readily apparent. The Steps for Making a Bar Plot in Python
Bar plots, or bar graphs, are a straightforward way to present categorical data with rectangular bars, where the length of each bar is proportional to the value it represents. Bar plots are exceptionally useful for comparing different groups or tracking changes over time. In Python, creating bar plots can be done easily with libraries such as Matplotlib.
import matplotlib.pyplot as plt # Categories and their values labels = ['Category 1', 'Category 2', 'Category 3', 'Category 4'] values = [23, 45, 56, 78] plt.bar(labels, values) plt.title('Sample Bar Plot') plt.xlabel('Categories') plt.ylabel('Values') plt.show()This example demonstrates how to create a basic bar plot using Matplotlib. By specifying categories and their corresponding values, the
plt.bar()
function generates a bar plot that visually represents the data in an impactful way. Plotting in Python Libraries
When delving into the realm of data visualisation in Python, one is met with a plethora of libraries designed to simplify the process and enhance the aesthetics of plotting. From generating simple line charts to complex 3D visualisations, these libraries serve as powerful tools for data scientists, analysts, and enthusiasts alike.
Exploring Libraries for Plotting in Python
Python boasts a variety of libraries for data visualisation, each with its unique capabilities and applications. Among the most frequently used are Matplotlib, Seaborn, and Plotly. Matplotlib, known for its flexibility and simplicity, is akin to a Swiss Army knife for data plotting in Python. Seaborn builds on Matplotlib, offering more visually appealing and statistically sophisticated graphics. Plotly, on the other hand, brings interactivity to the table, enabling dynamic and intricate visualisations that can be embedded in web applications.Choosing the appropriate library depends on the specific needs of the project, including factors such as the complexity of the data, the required level of interactivity, and the intended audience for the data visualisation.
It's fascinating to note that whilst Matplotlib and Seaborn are primarily used for creating static plots, Plotly's capability for producing interactive plots makes it invaluable for web-based projects. Interactive plots allow viewers to engage with the data in a more meaningful way, such as zooming in on areas of interest or hovering over data points to display additional information. This level of interaction can significantly enhance the comprehension and retention of data insights.
For beginners in data visualisation, starting with Matplotlib is highly recommended due to its comprehensive documentation and wide range of use cases.
How Libraries Simplify Plotting in 3D Python
3D plotting in Python can appear daunting due to the complexity associated with representing multi-dimensional data visually. However, libraries such as Matplotlib's mplot3d
toolkit and Plotly simplify this process remarkably. They provide straightforward APIs that abstract away much of the complexity, making it easier to create stunning 3D visualisations.For instance, the mplot3d
toolkit in Matplotlib enables users to add a third dimension to their plots with minimal additional code. Plotly, known for its interactivity, allows users to create 3D plots that are not only visually appealing but also interactive, enabling users to rotate, zoom, and pan the view to explore the data from different angles.
import plotly.graph_objects as go fig = go.Figure(data=[go.Surface(z=[[1, 2, 3, 4], [5, 6, 7, 8], [9, 10, 11, 12]])]) fig.update_layout(title='3D Plot Example with Plotly', autosize=False, width=500, height=500, margin=dict(l=65, r=50, b=65, t=90)) fig.show()This example illustrates creating a simple 3D surface plot using Plotly. The ability to manipulate and explore the plot interactively in the browser showcases the power of Plotly for 3D data visualisation.
Selecting the Right Library for Your Plotting Needs
Choosing the right Python library for plotting requires a careful consideration of the project's requirements. Important factors include the type of plots required (2D vs 3D), the need for interactivity, the complexity of the data, and the overall aesthetics of the visualisation. While Matplotlib and Seaborn are exceptional for creating static 2D and simple 3D plots, Plotly shines when interactivity and advanced 3D visualisations are required.Additionally, it's worth considering the learning curve associated with each library. Matplotlib, with its extensive documentation and community support, is ideal for beginners. Seaborn, while similar, is best suited for those who need to incorporate statistical plots quickly and easily. Plotly, albeit more complex, offers extensive capabilities for interactive and high-quality 3D plots, making it a go-to option for projects requiring dynamic visualisations.
An intriguing aspect to consider when selecting a plotting library is its integration capabilities with other tools and frameworks. For instance, Plotly integrates seamlessly with Dash, a Python framework for building web applications, allowing for the creation of interactive, web-based data visualisation applications. This synergy between plotting libraries and web frameworks opens up vast possibilities for data presentation and exploration, making it an important consideration for projects aimed at a broader audience or requiring a high level of engagement.
Plotting in Python Examples
Plotting in Python allows you to turn raw data into meaningful insights through visual representations. Whether you're interested in revealing hidden patterns in your data, presenting complex information, or simply exploring the relationships between different sets of data, Python's plotting libraries like Matplotlib, Seaborn, and Plotly can help you achieve these goals with efficiency and sophistication.Through practical examples, you'll soon discover how plotting in Python can be both an engaging and indispensable part of your data analysis toolkit.
Visualising Data with Plotting in Python
Data visualisation is an integral part of data analysis and science. By converting numerical data into graphical representations, complex insights can be communicated effectively, making analyses accessible to a broad audience. Python, with its array of plotting libraries, stands as a beacon for those delving into data visualisation.From simple histograms and scatter plots to intricate heat maps and 3D plots, Python enables you to visualise data with precision and creativity, allowing for a deeper understanding of the underlying patterns and trends.
One of the remarkable features of data visualisation in Python is its ability to adapt to various kinds of data and analysis needs. For instance, time-series analysis benefits significantly from line plots, allowing viewers to track changes over time. On the other hand, for categorical data analyses, bar plots provide a clear comparison between groups. This versatility ensures that regardless of the data at hand, Python offers a plotting solution that can highlight the most relevant information in the most coherent way.
Pairing data visualisation with statistical analysis in Python can greatly enhance the depth of your insights. Libraries like Seaborn have built-in functions to display complex statistical patterns directly within plots.
Real-world Example of Scatter Plot in Python
Scatter plots are widely used in data analysis to explore the relationship between two numerical variables. Each point in the scatter plot represents an observation in the dataset with coordinates corresponding to two variable values. This type of plot is crucial for detecting correlations, trends, and potential outliers within data.A common real-world application of scatter plots is in the field of economics, where they can be used to visualise the relationship between countries' GDP and their population growth rate.
import matplotlib.pyplot as plt # GDP and Population Growth data gdp = [60000, 42000, 30000, 21000, 53000] pop_growth = [0.4, 0.9, 1.5, 2.0, 0.2] plt.scatter(gdp, pop_growth) plt.title('GDP vs. Population Growth') plt.xlabel('GDP in USD') plt.ylabel('Population Growth (%)') plt.grid(True) plt.show()This example demonstrates how a simple scatter plot can provide immediate visual clues about the relationship between a country's economic output and its population growth, serving as a basis for further statistical analyses.
Creating Compelling Visuals with Bar Plot in Python
Bar plots, or bar charts, represent data with rectangular bars where the length of each bar is proportional to the value it represents. They are exceptionally useful for comparing different categories of data or showing variations in data over time. For instance, in marketing, bar plots can effectively showcase the monthly sales totals for different product categories, offering clear insights into performance trends.Python's plotting libraries streamline the creation of bar plots, allowing for both horizontal and vertical chart orientations as well as a range of customisation options, from basic colour changes to complex annotations.
import matplotlib.pyplot as plt # Monthly Sales Data months = ['January', 'February', 'March', 'April'] sales = [230, 340, 560, 480] plt.bar(months, sales, color='blue') plt.title('Monthly Sales Data') plt.xlabel('Month') plt.ylabel('Sales') plt.xticks(rotation=45) plt.show()This example of bar plotting in Python illustrates how to visualise monthly sales data. By adding colour, rotating the x-axis labels, and other simple customisations, the bar plot becomes not just informative but also visually engaging.
Plotting In Python - Key takeaways
- Plotting in Python: The process of creating visual representations of data using libraries such as Matplotlib, Seaborn, and Plotly.
- Matplotlib: A foundational Python library used for creating a wide array of plots, with an object-oriented API that allows embedding into applications.
- Plotting in 3D Python: A technique for visualising data in three dimensions using libraries like Matplotlib's
mplot3d
toolkit, to enhance the depiction of complex relationships. - Scatter Plot in Python: A type of plot that displays values, typically for two variables, as a collection of points and is effective for identifying correlations in data.
- Bar Plot in Python: A method for presenting categorical data with rectangular bars, where the bar length is proportionate to the value represented, useful for comparisons and tracking changes over time.
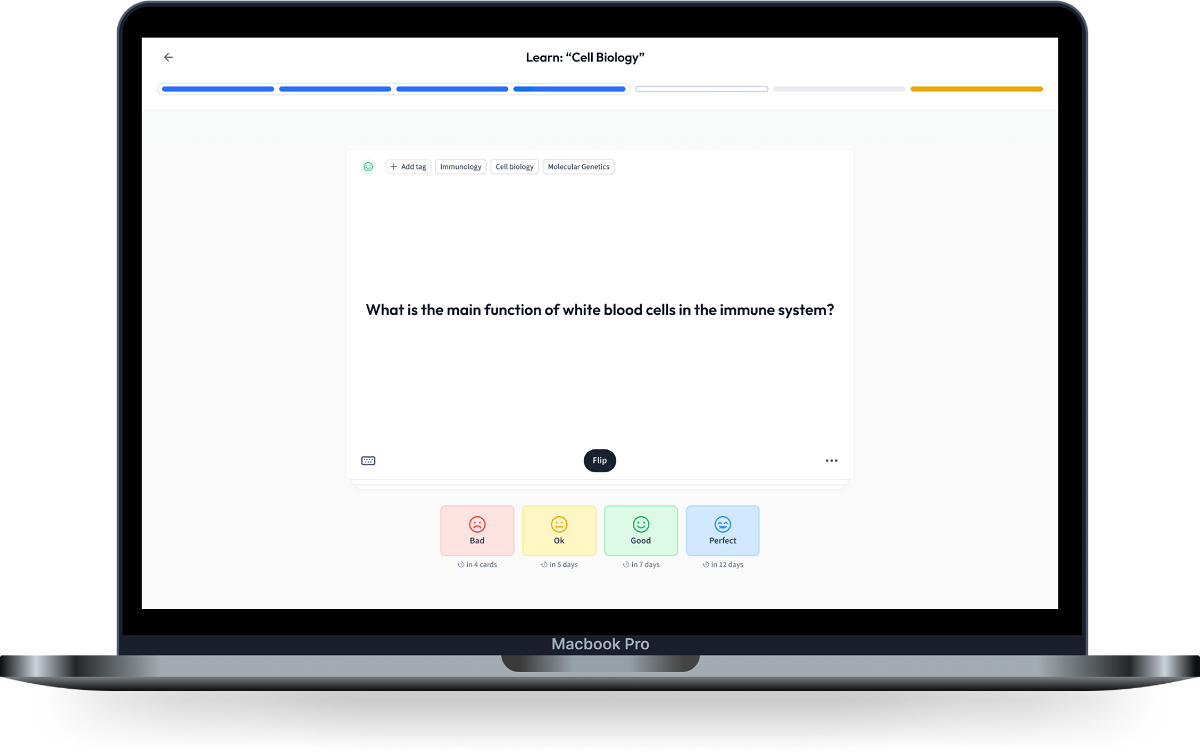
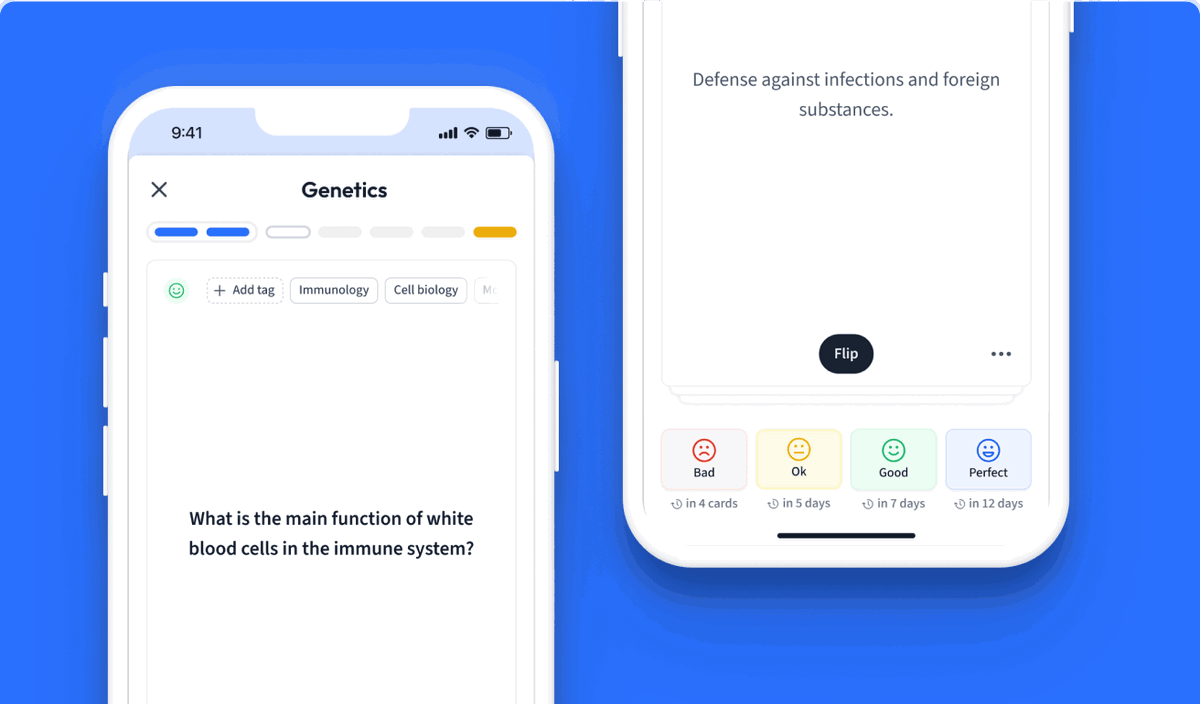
Learn with 41 Plotting In Python flashcards in the free StudySmarter app
Already have an account? Log in
Frequently Asked Questions about Plotting In Python
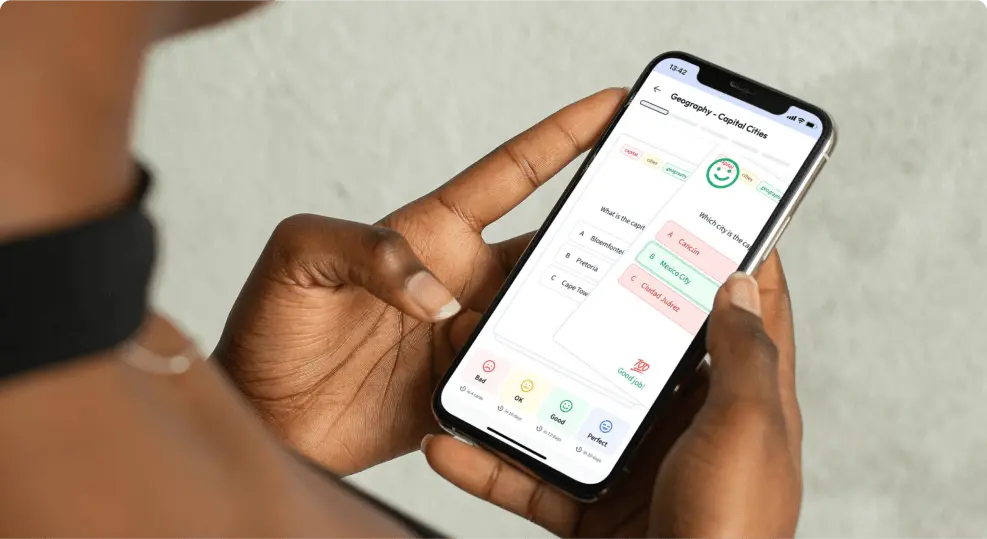
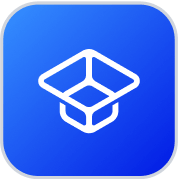
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more