Jump to a key chapter
Polymorphism Programming Basics
Polymorphism is a fundamental concept in object-oriented programming. It allows objects to be treated as instances of their parent class. This capability makes it possible to use a simpler interface while still harnessing the power of more complex classes.
Definition of Polymorphism in Computer Science
Polymorphism in computer science is a concept where a single function or operator behaves differently in various contexts. It promotes flexibility in the code, allowing developers to design systems that can handle a wide variety of tasks with the same method signature.
In simple terms, polymorphism allows for methods in different classes to have the same name, while catering to different implementations. It typically occurs in two forms: compile-time polymorphism (also known as static polymorphism) and run-time polymorphism (also known as dynamic polymorphism). The method used to call upon these capabilities distinguishes these two types.
Consider a scenario where you have a base class called Animal and two derived classes called Dog and Cat. Each derived class implements a method called makeSound.
class Animal { void makeSound() { // Default implementation } } class Dog extends Animal { void makeSound() { System.out.println('Woof'); } } class Cat extends Animal { void makeSound() { System.out.println('Meow'); } }In the example above, both the Dog and Cat classes implement the makeSound method, which behaves differently for each type of animal. This is an illustration of polymorphism, where the same method can exhibit different behaviors.
In polymorphism, method names can remain consistent while allowing different methods to execute based on the object type.
What is Polymorphism in Programming?
Polymorphism in programming allows developers to write efficient, maintainable, and reusable code. It is commonly applied through method overloading and method overriding.
To further grasp polymorphism, it's crucial to understand method overloading and method overriding:
- Method Overloading: This happens when two or more methods in the same class have the same name but different parameters. It's a compile-time polymorphism. The correct method execution is determined by the method signature known at compile time.
class Display { void show(int a) { System.out.println('Integer: ' + a); } void show(String b) { System.out.println('String: ' + b); } }
- Method Overriding: This occurs when a method in a child class has the same name and the exact same parameters as a method in the parent class. This is run-time polymorphism, where the method to be executed is resolved at run-time.
class Vehicle { void run() { System.out.println('Vehicle is running'); } } class Bike extends Vehicle { void run() { System.out.println('Bike is running safely'); } }
Polymorphism in Object Oriented Programming
In object-oriented programming, polymorphism plays a critical role by enabling classes to implement the same methods while maintaining different functionalities. This concept allows you to create scalable and reusable code, facilitating the process of software development.
Object Oriented Programming Polymorphism
Object-oriented programming (OOP) is a programming paradigm that uses 'objects' to design applications and computer programs. It makes comprehensive use of:
- Inheritance: Creating a new class using an existing one, inheriting features while adding unique characteristics.
- Encapsulation: Keeping the data (attributes) and the methods (functions) that manipulate the data into a single unit, or class.
- Abstraction: Hiding complex realities while exposing only the necessary parts.
- Polymorphism: Allowing methods to do different things based on the object it is acting upon.
Let's illustrate polymorphism with an example of a graphical system that supports different shapes. Consider a base class Shape, and two derived classes Circle and Rectangle.
class Shape { void draw() { System.out.println('Drawing a shape'); } } class Circle extends Shape { void draw() { System.out.println('Drawing a circle'); } } class Rectangle extends Shape { void draw() { System.out.println('Drawing a rectangle'); } }Using polymorphism, you can call the draw method on any shape, and the appropriate method for Circle or Rectangle will be invoked.
The draw method in each class demonstrates polymorphism by using the same method signature across different subclasses.
Understanding Polymorphism in Computer Science
Polymorphism is also understood as the ability of different objects to respond to the same operation in different ways. It offers the advantages of refactoring and code simplification, making it easier to manage and extend. Types of Polymorphism in computer science:
- Compile-time polymorphism: Known at the time of compilation, often achieved by method overloading or operator overloading.
- Run-time polymorphism: Known during program execution, typically achieved through method overriding.
To better understand compile-time vs run-time polymorphism, here is a detailed breakdown:
- Compile-time Polymorphism: Enables the function to take different forms at compile time. Method overloading is a way to achieve this. It supports different arguments in the method signature:
class OverloadExample { void display(int a) { System.out.println('Integer: ' + a); } void display(String b) { System.out.println('String: ' + b); } }
- Run-time Polymorphism: This form of polymorphism is resolved during run-time. It is usually achieved through method overriding where the base class's method is overridden in the derived class:
class Base { void show() { System.out.println('Base method'); } } class Derived extends Base { void show() { System.out.println('Derived method'); } }
Polymorphism Examples in Computer Programming
Polymorphism allows for the design of flexible systems by enabling objects to be treated as instances of their parent class. It's a key feature in object-oriented programming, facilitating code reusability and scalability.
Practical Examples of Polymorphism
Practical implementations of polymorphism are widespread in programming, allowing for effective and straightforward code structure. Two primary types of polymorphism used are:
- Compile-time polymorphism (method overloading)
- Run-time polymorphism (method overriding)
Here's how method overloading works in Java:
class Display { void show(int a) { System.out.println('Integer value: ' + a); } void show(String b) { System.out.println('String value: ' + b); } }In this example, two methods named show have different parameters. This is compile-time polymorphism, where the method is determined by the parameter list.
An illustration of method overriding:
class Animal { void sound() { System.out.println('Animal makes a sound'); } } class Dog extends Animal { void sound() { System.out.println('Dog barks'); } }Here, the method sound is overridden in the subclass Dog. This demonstrates run-time polymorphism, where the method that gets executed is known at run-time based on the object type.
Method overloading is a part of compile-time polymorphism, while method overriding pertains to run-time polymorphism.
In many practical applications, polymorphism is utilized extensively beyond the simple examples highlighted above. For instance, consider designing a user interface library where various UI components like buttons, sliders, and text fields derive from a base class called Component. This setup allows the library to process a collection of various components using the same method to render them, despite each component requiring a different drawing logic inside:
class Component { void display() { System.out.println('Displaying component'); } } class Button extends Component { void display() { System.out.println('Displaying button'); } } class Slider extends Component { void display() { System.out.println('Displaying slider'); } }Functionality such as this allows complex graphical applications to maintain a unified interface, while efficiently managing different behaviors tailored to each specific component type.
Real-World Scenarios of Polymorphism Programming
Polymorphism is a fundamental element in real-world software development scenarios, providing the dynamics needed to build adaptable applications. By allowing different techniques to execute under a unified interface, polymorphism is pivotal in ensuring flexibility and robustness.
Consider a payment processing system that handles diverse payment methods like creditCard, paypal, and bank transfer. Each payment method implements a common interface:
interface Payment { void pay(double amount); } class CreditCardPayment implements Payment { void pay(double amount) { System.out.println('Paid by credit card: ' + amount); } } class PaypalPayment implements Payment { void pay(double amount) { System.out.println('Paid via PayPal: ' + amount); } }Such a scenario showcases polymorphism by ensuring each payment type behaves according to its own requirements, while sharing the same pay method interface.
In real-world applications, polymorphism substantially minimizes code redundancy and complexity. Let's look at a vehicle tracking system that monitors different vehicle types like cars and trucks. Both vehicle types can inherit from a base class Vehicle, employing polymorphic methods to handle diverse operations such as moving or calculating fuel economy:
class Vehicle { void move() { System.out.println('Moving vehicle'); } } class Car extends Vehicle { void move() { System.out.println('Car is moving'); } } class Truck extends Vehicle { void move() { System.out.println('Truck is moving load'); } }This structured program design eases maintenance and future expansions, as new vehicle types can be integrated seamlessly by extending the existing Vehicle class, utilizing the polymorphic move function. As product requirements evolve, such structures empower a seamless embedding of new functionalities without significantly altering existing code. Polymorphism, fervently adopted across many domains, catalyzes a reduction in intricate interdependencies often seen in substantial, multifunctional software projects.
Benefits of Polymorphism in Programming
Polymorphism offers several advantages in programming by simplifying code maintenance and enhancing its flexibility. These benefits make it a key component of object-oriented programming and crucial for developing scalable applications.
Flexibility and Reusability in Code
One of the primary benefits of polymorphism is flexibility. It allows developers to write code that works with objects of different classes under a unified interface, thus reducing the need for multiple function implementations. This flexibility leads to the automatic extension of functionalities, promoting code reusability. For example, by using a base class reference to call methods from subclass objects, you can easily interchange components without altering the base class interface.
An illustration showcasing flexibility and reusability through polymorphism:
class Employee { void work() { System.out.println('Employee working'); } } class Developer extends Employee { void work() { System.out.println('Developer coding'); } } class Designer extends Employee { void work() { System.out.println('Designer designing'); } } void startWork(Employee e) { e.work(); } startWork(new Developer()); // Output: Developer coding startWork(new Designer()); // Output: Designer designingThis example demonstrates how various subclasses can dynamically implement the work method, providing flexibility in utilizing diverse object types.
Polymorphism allows the same interface or method to be implemented in various ways, depending upon the object type.
Delving deeper into the concept of reusability: Polymorphism streamlines expansions and future updates, empowering objects to be versatile across different codebases. Developers are encouraged to design applications with components that can be reused across multiple contexts, resulting in less code duplication and fewer errors. Consider a scenario involving different payment gateways (like credit cards, digital wallets) each implementing a Payment interface. Using polymorphism, you can easily integrate or swap new gateways without modifying existing application logic. This ensures consistency in how payment processes are conducted while facilitating quick transitions and updates, enhancing the overall modularity and adaptability of your software projects.
Simplified Code Management with Polymorphism
Polymorphism significantly simplifies code management by allowing you to manage objects with a single interface, regardless of their underlying class. This results in cleaner, more maintainable code. The complexity around dealing with numerous classes explicitly can be reduced, and the code becomes easier to navigate and modify.
Using an interface to illustrate code management:
interface Machine { void start(); } class Car implements Machine { void start() { System.out.println('Car is starting'); } } class Airplane implements Machine { void start() { System.out.println('Airplane is starting'); } } public void initiateMachine(Machine m) { m.start(); } initiateMachine(new Car()); // Output: Car is starting initiateMachine(new Airplane()); // Output: Airplane is startingThis example highlights how polymorphism efficiently manages diverse implementations under a common interface.
Using polymorphism, applications can maintain a consistent message-passing system, regardless of the specific class objects involved.
Exploring deeper into code management: Polymorphism supports the DRY (Don't Repeat Yourself) principle, minimizing code redundancy. It enables applications to abstract common behavior into base classes while allowing dynamic adaptations in derived classes. This methodology ensures that future developments or alterations do not require rewriting existing code bases. Additionally, developers can deploy more robust testing mechanisms by focusing on the base class functionalities while leveraging polymorphic behaviors. Consider a large-scale software system employing different communication modules like emails, messages, and notifications. Each module could be derived from a common class Communication. Using polymorphism, features like send messaing or logging could be centralized, aiding not only in simplified code execution but also in maintaining coherent operator interactions across different modules, which ultimately translates to reduced cognitive load on software management and enhances overall application efficiency.
Polymorphism programming - Key takeaways
- Polymorphism in programming allows objects to be treated as instances of their parent class, enabling a simplified interface while using complex functionality.
- In computer science, polymorphism is a concept where a single function or operator behaves differently in various contexts, promoting code flexibility and efficiency.
- Object-oriented programming leverages polymorphism to allow methods to perform differently based on the object type, achieved through method overloading and method overriding.
- Compile-time polymorphism (method overloading) occurs when methods have the same name but different parameters, determined at compile time.
- Run-time polymorphism (method overriding) resolves which method to execute at run-time, allowing methods in derived classes to override parent class methods.
- Polymorphism examples include designing systems where different objects (like `Animal`, `Dog`, `Cat`) implement the same methods differently, increasing code scalability and reusability.
Learn faster with the 23 flashcards about Polymorphism programming
Sign up for free to gain access to all our flashcards.
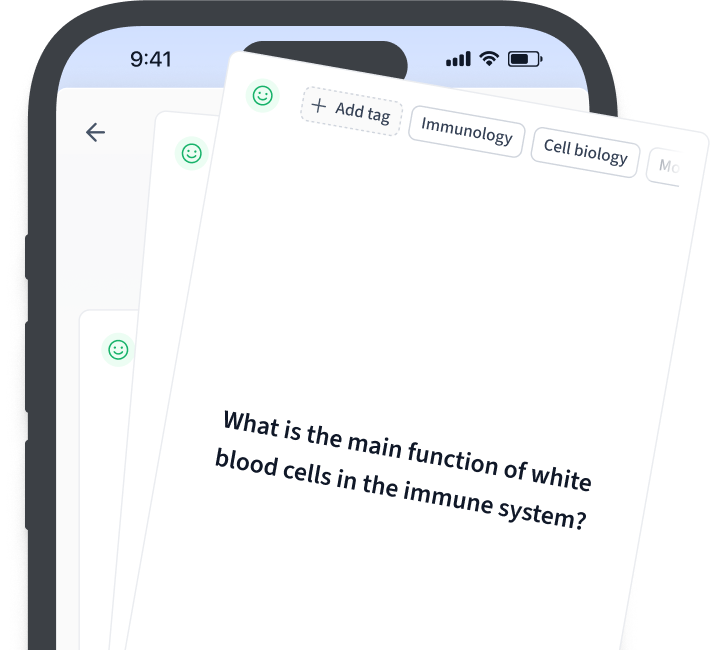
Frequently Asked Questions about Polymorphism programming
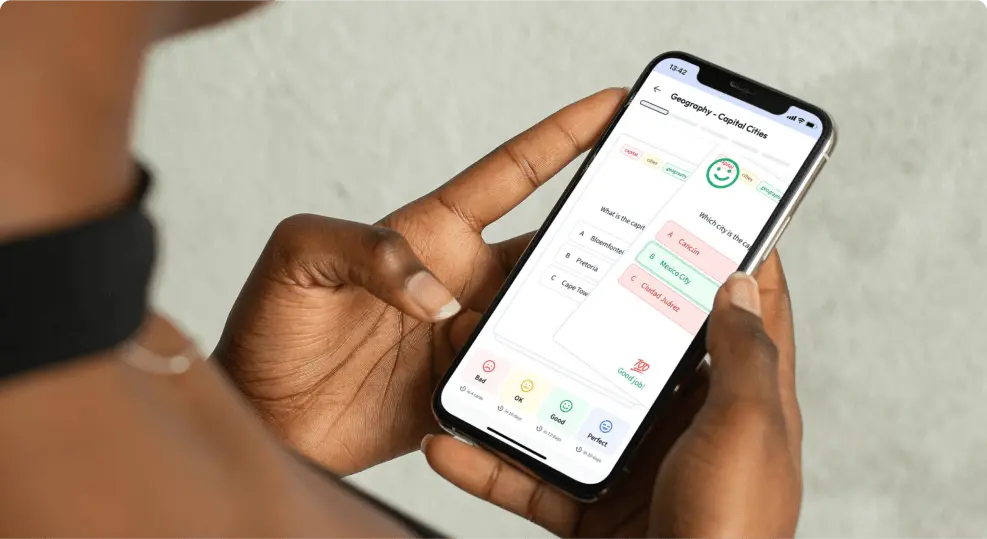
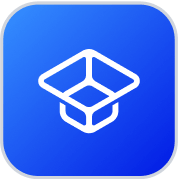
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more