Jump to a key chapter
Understanding Programming Tools in Computer Science
In the fascinating and ever-evolving world of computer science, programming tools play a central role. Speaking in simple terms, these are the key resources you utilise when creating computer programs, instructions, or software. Imagine trying to paint a picture without a brush, or build a house without a hammer - it's possible, but incredibly difficult and time-consuming. A similar principle applies here.Programming tools, often referred to as tools in the software industry, are sets of software applications used to write, test, debug and maintain source code. They provide foundations for compiling, interpreting and editing code in various programming languages.
What are Programming Tools? - Definition
Much like a carpenter relies on his tools to build a house, a computer programmer or developer relies on programming tools to create software and applications. But, what exactly are these tools? Let's dive in a little deeper.Compiler | An application that translates high-level programming language into machine code. |
Text editor | Used to write and edit code in various programming languages. |
Debugger | Detects and removes errors or bugs from source code. |
Assembler | Converts assembly language into machine code. |
Exploring Different Types of Programming Tools
When coding, you wouldn't be limited to only one type of programming tool. Various tools are available, each designed to assist a different part of your coding journey.- IDE (Integrated Development Environment)
- Code Editor
- Library
- API (Application Programming Interface)
- Database Management System
An example of an IDE is Eclipse, which is commonly used for Java development. Code editors, like Sublime Text or Atom, are perfect for making fast and simple edits to your code. Libraries are pre-written pieces of code that are reusable, thus saving you time. APIs provide a set of rules and protocols for building software. Lastly, Database Management Systems like MySQL help organise and manage databases.
The Role and Function of Programming Tools in Computer Science
Programming tools help computer scientists be more productive and efficient. Those tools not only help write and design programs but also help find and fix mistakes, optimise code, and run tests to ensure functionality. Understanding the role and function of these tools is crucial in learning how to code and develop software. Let's look at an example of how tools play an important role using Python. In Python, the primary tool used for writing code would be a text editor. For instance, Sublime Text is a commonly used editor that offers a clean, efficient interface for writing code. This tool can highlight syntax errors and automatically completes brackets and quotation marks, supporting speed and accuracy in writing code.Real-life Application of Programming Tools in Computer Science
In real-life contexts, programming tools vastly simplify the process of creating new software solutions. Additionally, they are the backbone of creating powerful, efficient, and robust coding solutions. Consider a software development company. In daily operations, these tools enable developers to manage large codebases, collaborate with team members, test and debug their code, and ultimately, produce high-quality software efficiently.In fact, it's not uncommon for companies to use a wide range of programming tools together to form an effective 'toolchain'. This allows each tool to focus on doing one thing well while collectively providing a comprehensive set of features and capabilities.
// A simplified representation of a toolchain in Javascript development // Step 1: Write code code = WriteCodeInTextEditor(); // Step 2: Use a compiler or transpiler compiledCode = Babel.transpile(code); // Step 3: Bundle assets bundle = Webpack.bundle(compiledCode); // Step 4: Test the code tests = RunTests(bundle); // Step 6: Deploy deployResult = DeployToServer(bundle);
The Evolution and History of Programming Tools
Understanding the history and evolution of programming tools is integral to understanding their current state and envisioning their future growth. These tools have witnessed transformations from the rudimentary punch-card systems to today's advanced, high-end software that supports seamless programming in a plethora of languages.Early Development Stages of Programming Tools
During the earliest phase of computer science development in the 1950's and 1960's, programming was a laborious and manual task. Machine language, precisely crafted sequences of \(0\)s and \(1\)s were the norm. Programmers used these **machine languages** to communicate directly with computers, making software creation an incredibly complex and challenging endeavour.Machine language is a low-level programming language which consists of binary or hexadecimal instructions directly executed by the computer's central processing unit (CPU).
// Example of how machine language looked: 1110 0010 1100 1011 0010 1010 1111 0001
The Shift from Traditional to Advanced Programming Tools
The shift from traditional to advanced programming tools commenced when **assembly languages** were introduced. These allowed programmers to use symbolic representations for machine operations and memory locations. Objects in programming have evolved throughout the time. Here is a brief comparison of an **Object** in the early-era vs the modern-era:Early Era Object | Punched Card with strings of 0s and 1s |
Modern Era Object | Digital Files of High-Level Programming languages (like .java, .py) |
Noteworthy Milestones in the History of Programming Tools
Over the years, revolutionary milestones have been reached that shaped the trajectory of programming tools. The emergence of structured programming in the 1960s broke programs into smaller, manageable sections, making them easier to troubleshoot and maintain. However, the next big leap came in the 1970s with the debut of **object-oriented programming** (OOP), marking a transformative advance in coding practices. OOP languages like C++ and Java encapsulated both data and the procedures applied to the data within the same unit, called an "object".Object-oriented Programming (OOP) is a programming paradigm that uses "objects" – data structures consisting of data fields and methods together with their interactions – to design applications and computer programs.
Evolution of Programming Tools - A Timeline
Let's take a tour of the timeline to understand the evolution of programming tools:- 1950s: Introduction of Assembly Languages
- 1957: Advent of FORTRAN, the first high-level language
- 1960s: Introduction of structured programming
- 1970s: Object-Oriented Programming comes into play
- 1990s: Popularisation of Integrated Development Environments (IDEs)
- 2000s-till date: Emergence of multiple high-level object-oriented languages like Python, Swift etc.
Delving into Programming Tools Techniques
Uncovering the varied techniques associated with programming tools can unlock new approaches and possibilities in programming. These can drastically improve efficiency, reduce errors in code, and open doors to more sophisticated and demanding applications.Utilising Programming Tools - Basic Techniques
Beginning with some basic techniques, a fundamental method is the use of the **text editor** of your choice. Whether it be Sublime Text, Atom, or Notepad++, understanding the functionality and shortcuts can speed up your coding process.// Switching between tabs in Sublime Text CTRL + TabNext, we introduce the usage of a compiler and how it can be utilised to detect errors in your code. A compiler takes in your source code and then translates it to machine-readable instructions.
A **Compiler** is a type of translator that transform an entire programme into machine language that is saved as an executable file.
// Compilation process in Java Java Compiler (javac) Source Code (.java) --> Bytecode (.class)Working with **libraries** is another essential technique. Libraries deliver pre-written code that you can utilise instead of writing everything from scratch. When programming in Python, libraries such as numpy and pandas can be imported to aid in calculations and data manipulation.
// Importing a library in Python import numpy as npA final basic technique is debuggers. Debuggers dissect your code and help find and correct any errors or bugs. This can be done by stepping through the code, setting breakpoints, or print debugging.
// Using print statements for debugging in Python print("Debugging statement")
Advanced Techniques for Navigating Programming Tools
Once the basic techniques are mastered, you can proceed towards more advanced techniques. One such approach is mastering keyboard shortcuts in your IDE (Integrated Development Environment). Speeding up navigation and tasks will save precious time and enable more efficient development.// Keyboard shortcuts in Visual Studio Code CTRL + P --> Open file CTRL + / --> Comment out codeInstallation and utilisation of **plugins** also falls under advanced techniques. Plugins extend your text editor or IDE further, increasing capabilities and providing unique features suited to your development needs.
IDE / Text Editor | Popular Plugin | Description |
Visual Studio Code | Live Server | Serves a local server with live reload feature for static & dynamic pages |
Sublime Text | Package Control | The Sublime Text package manager |
Common Problems and Their Solutions in Programming Tools Techniques
Every developer encounters challenges while using programming tools; it's part of the learning curve. One such issue might be difficulties with installations, where certain tools fail to install due to unsatisfied dependencies. Navigating this involves ensuring that your system meets the environment requirements for the tools.// Installing dependencies in Python pip installAnother common issue is errors or bugs within your code. Mastering the art of debugging becomes vital in overcoming this problem. Understanding how to read error messages can make a huge difference in how quickly you can find and fix a bug.
// Reading an error message in Python Traceback (most recent call last): File "", line 1, in print(5/0) ZeroDivisionError: division by zero
Keys to Mastering Programming Tools Techniques
Mastering programming tools techniques lies in practice and experience. Some key elements to this involve:- Understanding the tool's capabilities
- Maximising the usage of functionalities offered
- Persistently seeking ways to improve efficiency
- Staying updated with changes and updates in the tools
Grasping the Advantages of Programming Tools
Diving into the world of programming tools, it's clear that they offer a wealth of benefits, from making developers' lives easier to improving the efficiency and consistency of code generation. It's hard to imagine the programming world without these helpful tools.Efficiency and Speed: Top Advantages of Programming Tools
If you've ever written code, all the same, language, platform, or complexity level, you'll agree that efficiency and speed are paramount. Programming tools can significantly contribute to this. They are designed to automate and streamline aspects of writing code, facilitating rapid creation and modification of high-quality software. Tools like compilers, interpreters, debuggers, and modern integrated development environments (IDEs) make coding faster and more straightforward. They provide comprehensive environments that cover all steps of software development, from writing and editing code to debugging and deployment.An Integrated Development Environment (IDE) is a software suite that combines common developer tools into a single graphical user interface (GUI). It typically consists of a source code editor, compiler or interpreter, build automation tools, and a debugger.
- Automating repetitive tasks: Programming tools can Gautomate time-consuming, repetitive tasks. For example, build automation tools like Apache Maven or Gradle can compile a project's files into an executable program, reducing manual effort.
- Syntax highlighting and code formatting: These features allow you to easily distinguish language-specific keywords, objects, and structures, making code easier to read and write. The code formatting tool ensures that your code adheres to standard style conventions.
- Code suggestions: Some programming tools offer suggestions as you type, reducing the time you spend writing and inspecting code. This is particularly helpful when working with a language you're not entirely familiar with as it offers an on-the-go learning opportunity.
Exploring the Advantage of Simplified Debugging in Programming Tools
One of the significant benefits of programming tools is the simplified debugging process. Debugging refers to the process of identifying and rectifying errors or bugs within a program. Programming tools offer built-in debuggers that assist in this process, significantly reducing the amount of time and effort spent resolving these issues.A **Debugger** is a software tool that can help the software development process by identifying coding errors at various stages of the operating system or application development.
- Breakpoints: Debuggers allow you to set breakpoints in your code to pause program execution. This lets you examine the state of your program at that specific point, making it easier to pinpoint the origin of the bug.
- Stepping: The step feature lets you navigate through your code one line at a time. This gives you a detailed view of the program's flow and helps in tracking the bug's path.
- Variable examination: Debuggers also let you inspect the values of variables at a specific point in time. If a variable holds an unexpected value, it can be a clear sign of a bug.
// Using a breakpoint in Python debugging using pdb import pdb pdb.set_trace() // Sets a breakpoint
How Programming Tools Boost Productivity and Learning
Programming tools not only lead to efficient and fast coding but also significantly boost productivity. A streamlined development process and a reduced debugging period directly result in increased productivity. With less time spent managing your code structure or hunting down bugs, you can devote more time to building features and innovating. Programming tools also provide rich learning opportunities. Most come built-in with extensive documentation and vibrant user communities, offering resources to deepen your programming knowledge. They also offer practical exposure to best practice coding standards and styles. Additionally, modern programming tools provide solutions to speed up the learning curve of your chosen language or framework. They offer features like code completion, syntax highlighting, and semantic hints that help novice programmers get familiar with languages and code structures. Table below details few tools and their benefits:Tool | Productivity Boost | Learning Boost |
Visual Studio Code | Provides code completion, syntax highlighting and debugging capabilities optimizing productivity. | Extensions for learning a new language, and code snippets that contain examples of specific language syntax, help getting familiar with the language. |
PyCharm | Automates repetitive tasks, saving time and debugging capabilities, reducing-error resolution time. | Supports learning version control systems, and provides syntax error notifications helping to learn the language syntax. |
An Overview of Important Programming Tools
The world of programming comprises of a myriad of tools that aid in the development process. From text editors and Integrated Development Environments (IDEs) to version control systems and debuggers, these utilities are armed with features to create, test, debug, and deploy software applications effectively. In this section, you will explore some of these crucial tools, analysing their functionalities and significance.Essential Programming Tools Every Developer Should Know
As a budding developer, getting acquainted with essential programming tools should be one of your first steps. These tools, each varying in capacities and functionalities, can significantly enhance your programming experience, leading to more efficient and high-quality code. Following is a list of essential programming tools every developer should be familiar with:- Text Editors: Text editors like Sublime Text, Atom, or Notepad++ facilitate writing and editing code. They often feature syntax highlighting, auto-indentation, and other features that make code easier to read and write.
- Integrated Development Environments (IDEs): An IDE is a software application that provides a comprehensive environment for software development. IDEs like PyCharm, Eclipse, or NetBeans combine text editing with debugging, compiling, and other features for a streamlined development process.
- Version Control Systems (VCS): Tools like Git help you keep track of changes made to your code, facilitating collaboration and preventing data loss.
- Compilers: A compiler translates human-readable source code into machine code. Tools like GCC for C/C++ and javac for Java are examples of compilers.
- Debuggers: Debuggers, often integrated into IDEs, are tools used to test and debug software. They help developers identify and correct issues in their code.
Exploring Language-Specific Programming Tools
While some programming tools are language-agnostic, others are designed for use with specific languages. Using language-specific tools can enhance your programming experience, as they can offer features tailored to the language's unique characteristics and requirements. For instance, developers working with JavaScript might use the runtime environment Node.js or the task runner Grunt.js. For Python developers, tools like the web framework Django or the scientific library NumPy can come in handy.Here's an example using Pandas, a popular Python library for data analysis:
import pandas as pd df = pd.read_csv('data.csv') print(df.head())
Understanding the Function and Importance of Various Programming Tools
Getting hands-on with programming tools isn't only about knowing what they are. As a developer, you must understand their functions and why they're important. Let's explore the function and importance of different programming tools:- Text Editors and IDEs: These tools provide the environment for writing and editing code. Features like syntax highlighting, auto-completion, and code suggestions enhance readability and reduce coding time.
- Version Control Systems (VCS): These tools track changes made to your code and allow you to revert to earlier versions when needed. This is crucial for collaborating on projects and maintaining a coherent codebase.
- Compilers and Interpreters: They translate high-level code into machine-level code, enabling the computer to execute your programs. These tools also check for syntax errors and conduct preliminary debugging.
- Debugging tools: Debugging is a critical aspect of program development. Debugging tools can help identify and fix errors, reducing bug resolution time and improving software quality.
- Libraries and Frameworks: Libraries offer pre-written code snippets, and frameworks provide robust coding platforms. Both resources can save development time and facilitate the creation of powerful applications.
Evaluating the Best Programming Tools Based on Use Cases
Since there's a vast array of programming tools, you must identify the ones that best meet your coding requirements. Personal development preferences, use case, project complexity, team size, and chosen languages or platforms – all these factors can influence a tool's suitability. Below is a table that exemplifies the suitability of different tools for various use cases:Use Cases | Recommended Tools |
Web Development | VS Code, Atom, Git, Chrome Developer Tools, Bootstrap |
Data Science | Jupyter Notebook, Anaconda, Pandas, Scikit-learn |
Game Development | Unity, Unreal Engine, Blender, Visual Studio |
Mobile App Development | Android Studio, Xcode, React Native, Flutter, Kotlin |
Programming Tools - Key takeaways
- Machine language: A low-level programming language consisting of binary or hexadecimal instructions directly executed by the computer's central processing unit (CPU).
- Early programming tools: Physical equipments such as punched cards, switches, and paper tapes used for inputting machine code.
- Shift to advanced programming tools: Began with the introduction of assembly languages that used symbolic representations for machine operations and memory locations.
- FORTRAN: The first high-level programming language introduced in 1957. High-level languages are similar to human language, which made it easier for individuals to write and understand the code.
- Evolution of programming tools: Major milestones include the emergence of structured programming in the 1960s, debut of object-oriented programming (OOP) - a programming paradigm using "objects", in the 1970s, and popularity of dynamic and scripting languages, like Python and JavaScript in the past two decades.
- Programming tools techniques: Techniques associated with programming tools can unlock new approaches and possibilities in programming.
- Basic techniques: Include use of text editor, use of a compiler to detect errors in code, working with libraries to utilise pre-written code, and use of debuggers.
- Advanced techniques: Include mastering keyboard shortcuts in your IDE (Integrated Development Environment), and installation and utilisation of plugins.
- Common challenges: Difficulties with installations where certain tools fail due to unsatisfied dependencies and errors or bugs within your code.
- Mastering programming tools techniques: Involves practice, experience, understanding the tool's capabilities, maximising the usage of functionalities offered, persistently seeking ways to improve efficiency, and staying updated with changes and updates in the tools.
- Advantages of Programming Tools: Offer many benefits such as making developers' lives easier, improving the efficiency and consistency of code generation, enhancing speed, automating repetitive tasks, and simplifying debugging process.
- Integrated Development Environment (IDE): A software suite that combines common developer tools into a single graphical user interface (GUI).
- Debugger: A software tool that can assist the software development process by identifying coding errors at various stages of the operating system or application development.
- Programming tools boost productivity: They lead to efficient and fast coding, significantly enhancing productivity. They also provide rich learning opportunities.
- Learning benefits: Most programming tools come with extensive documentation and vibrant user communities, offering resources to deepen programming knowledge.
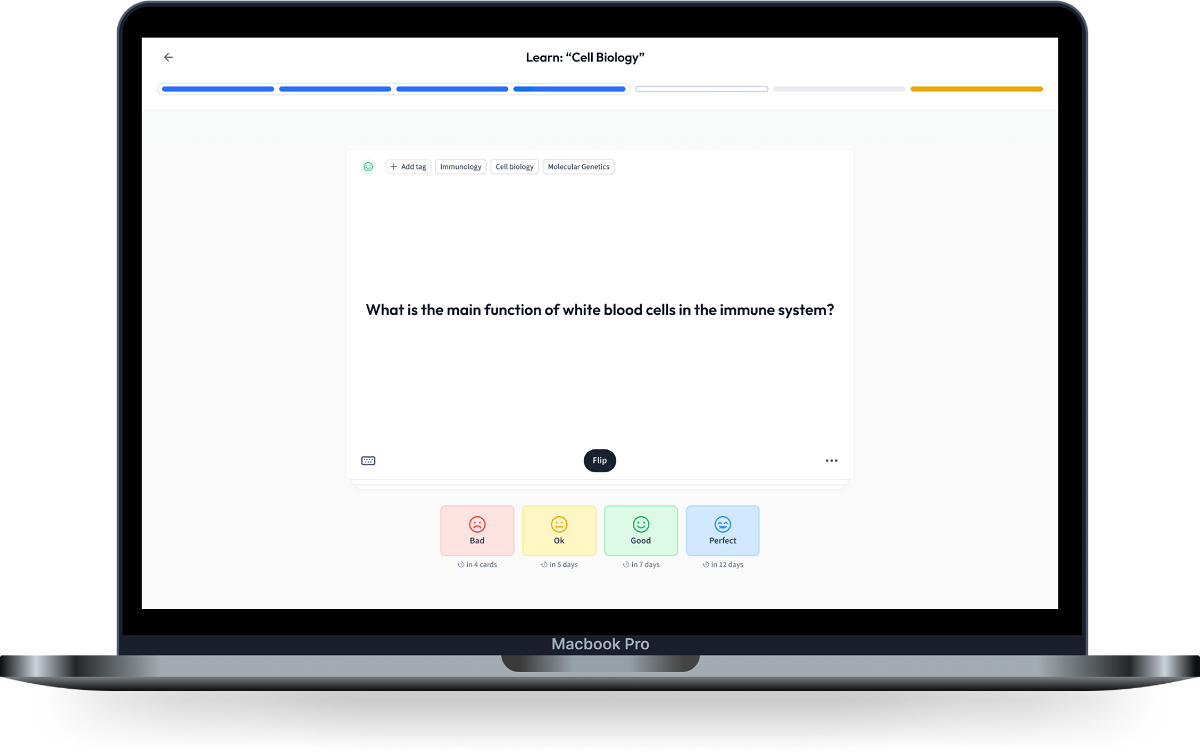
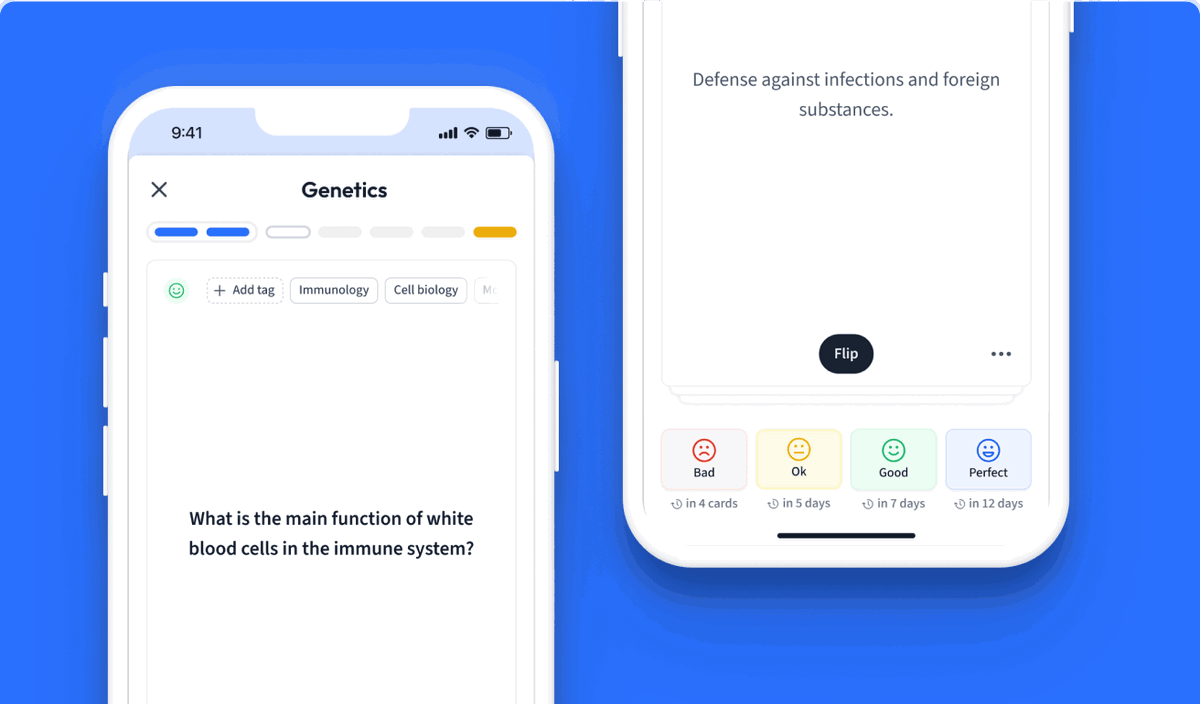
Learn with 15 Programming Tools flashcards in the free StudySmarter app
Already have an account? Log in
Frequently Asked Questions about Programming Tools
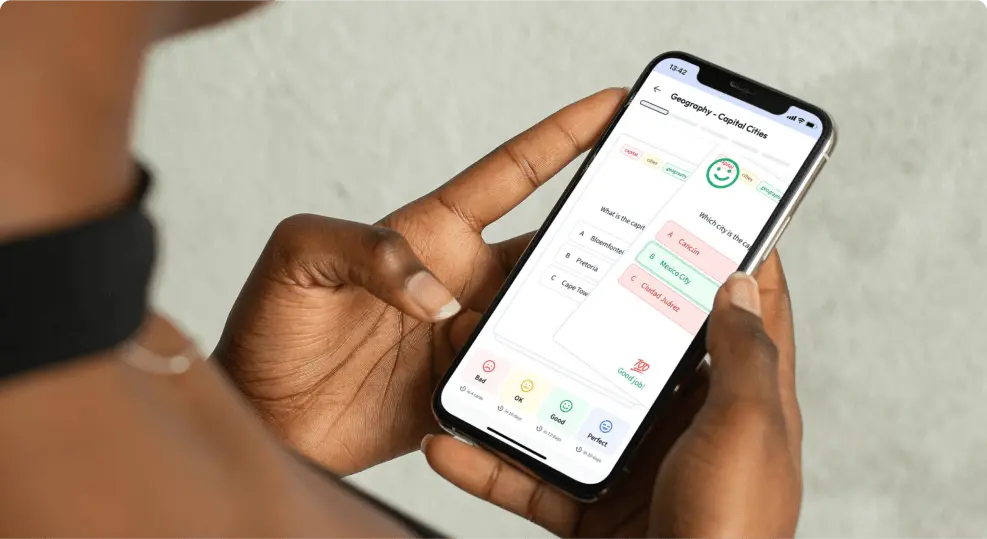
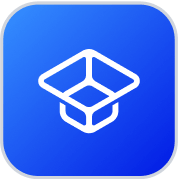
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more