Jump to a key chapter
Why Python is Essential for Computer Science Students
Python is an essential programming language for computer science students due to its simplicity, versatility, and numerous applications in the real world. With its user-friendly syntax and powerful capabilities, Python can be employed in various disciplines such as artificial intelligence, data science, web development, and more.Python is an interpreted, high-level, general-purpose programming language that is widely applicable across computing disciplines.
Python Code Basics and Syntax
Python is known for its readability and simplicity. Its code basics and syntax include:- Indentation: Python relies on indentation for defining code blocks rather than brackets or braces.
- Comments: Use the # character for single-line and triple quotes (''') for multiline comments.
- Variables: There is no need to explicitly declare a variable type in Python; the interpreter infers it based on the assigned value.
- Loops: Python supports both 'for' and 'while' loops with simple syntax.
- Functions: Functions are defined using the 'def' keyword, and you need to use parenthesis ( ) and a colon(:) to indicate the beginning of a function block.
- Classes: Python supports object-oriented programming with classes defined using the 'class' keyword.
Learn Python through Practical Examples
Working through practical examples is an effective way to gain understanding and experience when learning Python. Some examples that can help teach important Python concepts include:Text manipulation: Learn how to create, read, write, and manipulate text files using Python.
Web scraping: Practice extracting information from websites by scraping data using Python libraries like Beautiful Soup and Requests.
Data analysis: Gain experience with Python libraries like Pandas and NumPy to perform powerful data analysis and manipulation.
Python List and Array Fundamentals
Understanding lists and arrays is crucial, as these data structures play a significant role in various Python applications, such as data processing and mathematical operations.Understanding Python Lists
Python lists are versatile and easy-to-use data structures that can hold multiple items of varying data types. Lists can be modified, are ordered, and have many built-in methods for manipulation. Key features of Python lists include:- Creation: Lists can be created using square brackets ( [] ), enclosing the items separated by commas.
- Indexing: Items in lists can be accessed using positive or negative indices.
- Slicing: You can create a sublist from a list using a slice notation with a colon(:).
- Mutability: Lists allow item modification through assignment.
- List methods: Python lists come with built-in methods, such as append, extend, insert, remove, pop, and more.
Working with Python Arrays and NumPy
Python arrays are similar to lists but can only contain items of the same data type. They are most useful for mathematical operations and data analysis, as they consume less memory and offer better performance compared to lists. To work with Python arrays, it's often recommended to use NumPy, a powerful library for mathematical computing. NumPy array features include:- Creation: Use the numpy.array() function to create arrays.
- Indexing: Access elements in NumPy arrays using indices, similar to lists.
- Shape: Obtain the dimensions of a NumPy array using the 'shape' attribute.
- Reshape: Change the dimensions of a NumPy array with the 'reshape()' function.
- Arithmetic operations: Perform element-wise addition, subtraction, multiplication, and division on arrays. Also, perform linear algebra and statistical operations using specialized NumPy functions.
NumPy also offers advanced features such as broadcasting, which enables arithmetic operations on arrays of different shapes, and various functions for linear algebra, Fourier analysis, and more.
Programming Projects in Python: Examples
To truly understand Python as a programming language, working with real-world code examples and projects is essential. This not only helps you learn how to write efficient and scalable code, but also provides context for Python's various applications in different scenarios. Below are some examples of real-world projects that can offer valuable experience and insight.Building Web Applications using Flask
Flask is a lightweight web application framework in Python that allows you to create web applications easily. To get started with Flask, follow these steps:- Install Flask using pip:
pip install Flask
- Create a new file for your Flask application, such as
app.py
. - Import necessary libraries by adding
from flask import Flask, render_template, request
at the top of your file. - Instantiate the Flask app with
app = Flask(__name__)
. - Define routes for the application. Routes determine how your web app will respond to user requests and navigate between pages. An example of a route definition:
@app.route('/') def index(): return render_template('index.html')
- Create templates, which are HTML files with placeholders, for your web application using Jinja2, Flask's default templating language. Templates are stored in a 'templates' directory by default.
- Define how your Flask app will handle form submissions and user input by creating routes and functions.
- Start the Flask development server to run your web application locally using
if __name__ == '__main__': app.run(debug=True)
at the end of your file.
Data Analysis with Python and Pandas
Python's Pandas library is a powerful tool for data analysis and manipulation. Follow these steps for a beginner-friendly project centered around data analysis using Pandas:- Install Pandas using pip:
pip install pandas
. - Import Pandas in your Python script by adding the line
import pandas as pd
. - Load a dataset (in CSV format, for example) into a Pandas DataFrame using the syntax
data = pd.read_csv('dataset.csv')
. - Explore your DataFrame using methods such as
data.head()
anddata.info()
to get an understanding of the data structure and rows. - Clean your data by removing duplicates, filling missing data, and dealing with outliers.
- Manipulate your data using Pandas' built-in functions and operations, such as filtering, sorting, grouping, renaming, and more.
- Perform basic statistical analysis using Pandas, such as calculating mean, median, mode, standard deviation, correlations, and more.
- Create visualizations using a library like Matplotlib or Seaborn to better understand trends and patterns in your data.
- Draw insights from your data and present the results of your analysis.
Python for Game Development
Python can also be used for game development, thanks to various libraries and frameworks specifically designed for creating games. Below is an introduction to creating simple games using PyGame.Creating Simple Games Using PyGame
PyGame is a popular library for game development in Python. It allows for the creation of simple 2D games using the SDL library. To get started with PyGame, follow these steps:- Install PyGame using pip with the command
pip install pygame
. - Create a new Python script for your game, such as
game.py
. - Import the PyGame library by adding
import pygame
at the top of your file. - Initialize PyGame by calling
pygame.init()
. - Create a game window using
screen = pygame.display.set_mode((width, height))
, where width and height are the dimensions of your game window. - Create a game loop that will run continuously, polling for events, updating the game state, and rendering your game. An example:
running = True while running: for event in pygame.event.get(): if event.type == pygame.QUIT: running = False screen.fill((0, 0, 0)) # Clear the screen # Game logic and rendering go here pygame.display.flip() # Update the display pygame.quit()
- Load and display images using PyGame's built-in methods, such as
pygame.image.load()
andscreen.blit()
. - Handle user input using the
pygame.event
module and update the game state accordingly. - Add game elements, such as characters, enemies, and obstacles, and apply collision detection.
- Add sound effects and background music using PyGame's
pygame.mixer
module.
Enhancing Python Skills through Resources
To master Python and improve your skills, various educational resources are available, ranging from online tutorials and documentation to books and certified courses. By engaging with these resources, you can deepen your knowledge and understanding of Python, stay up-to-date with the latest developments in the language, and explore advanced topics.Online Learning Resources for Python
There are numerous online learning resources available for Python, catering to learners at different skill levels. These resources provide essential information, tips, and tricks that will help you become a proficient Python developer.Python Documentation and Tutorials
Python documentation and tutorials are critical resources for understanding the nuances of the Python language and its various features. Some of the key documentation and tutorials include:- Python Official Documentation: The official Python documentation covers the language reference, standard library, and various tutorials (available at docs.python.org). This is an indispensable resource for Python developers.
- Real Python: Real Python provides a wide range of tutorials and articles for learning Python. Topics range from basic Python features to advanced concepts such as web frameworks, machine learning, and more (available at realpython.com).
- GeeksforGeeks: This website contains numerous Python tutorials, example codes, and explanations covering various aspects of Python development (available at geeksforgeeks.org).
- Python.org's Beginner's Guide: This Python.org guide includes tutorials and examples aimed at helping beginners get started with Python (available at docs.python.org/3/tutorial/index.html).
Python-focused Forums and Communities
Engaging with Python-focused forums and communities enables you to learn from experienced Python developers and get assistance in resolving any issues you encounter. Some popular Python communities include:- Stack Overflow: With a very large and active community, Stack Overflow is a website where you can ask questions, find answers to existing questions, and collaborate with other Python developers (available at stackoverflow.com/questions/tagged/python).
- Reddit's /r/Python: This subreddit is dedicated to discussing Python and sharing Python projects, tips, and resources (available at reddit.com/r/Python).
- Python Community on Slack: Python developers can connect and discuss Python-related topics in various channels dedicated to specific Python categories (available at pythoncommunity.slack.com).
- Python Community on Discord: Another instant messaging platform where Python developers can join and engage with an active community, ask questions, and share resources (invitation link available at disboard.org/server/352097539998621697).
Python Books and Courses
Python books and courses are excellent ways to gain a deeper understanding of the language and learn from experienced instructors. Many books and courses cater to learners of various skill levels and cover a wide range of topics.Essential Python Books for Students
A well-written Python book can help you understand the language's foundations and provide valuable insights into programming techniques. Some essential Python books for computer science students include:- Python Crash Course by Eric Matthes: This book is a comprehensive guide for beginners that focuses on practical projects.
- Learn Python the Hard Way by Zed A. Shaw: This book is aimed at beginners, introducing Python concepts through hands-on exercises.
- Fluent Python by Luciano Ramalho: This advanced book explores Python's unique features and offers insights into Python functions, objects, and data structures.
- Effective Python by Brett Slatkin: This book presents concrete examples and practical advice for writing effective Python code.
- Python for Data Analysis by Wes McKinney: This book is essential for those who want to learn how to use Python for data analysis, leveraging powerful libraries such as Pandas and NumPy.
Python Online Courses and Certifications
Online courses and certifications offer structured Python learning, often with detailed explanations, examples, and assignments. Many courses also provide access to experienced instructors and interactive learning environments. Some notable Python online courses and certifications include:- Introduction to Python Programming by Udacity: This beginner-focused course covers Python basics, control structures, and data structures (available at udacity.com/course/introduction-to-python--ud1110).
- Python for Everybody by University of Michigan on Coursera: This popular specialization provides an introduction to Python programming. The course covers topics such as data structures, web access, databases, and data visualization (available at coursera.org/specializations/python).
- Google IT Automation with Python on Coursera: A hands-on certification program comprising six courses that focus on Python, Git, IT automation, and using Python to interact with operating systems (available at coursera.org/professional-certificates/google-it-automation).
- Python Core and Advanced by Udemy: This comprehensive course covers basic to advanced Python topics, including file handling, object-oriented programming, and web development with Django (available at udemy.com/course/python-core-and-advanced).
- Data Science and Machine Learning Bootcamp with R and Python by Udemy: This course teaches Python and R programming, data manipulation, machine learning, and other relevant skills for data science and machine learning (available at udemy.com/course/data-science-and-machine-learning-bootcamp-with-r).
Python - Key takeaways
Python: An interpreted, high-level, general-purpose programming language with various applications in fields like artificial intelligence, data science, web development, and more.
Python code basics: Include indentation, comments, variables, loops, functions, and classes.
Python lists: Versatile and easy-to-use data structures that can hold multiple items of varying data types, allowing modification and offering built-in methods for manipulation.
Python arrays: Similar to lists but can only contain items of the same data type, useful for mathematical operations and data analysis with the help of the powerful NumPy library.
Learn Python: Enhance Python skills through resources such as online tutorials, forums, essential books, and certified courses.
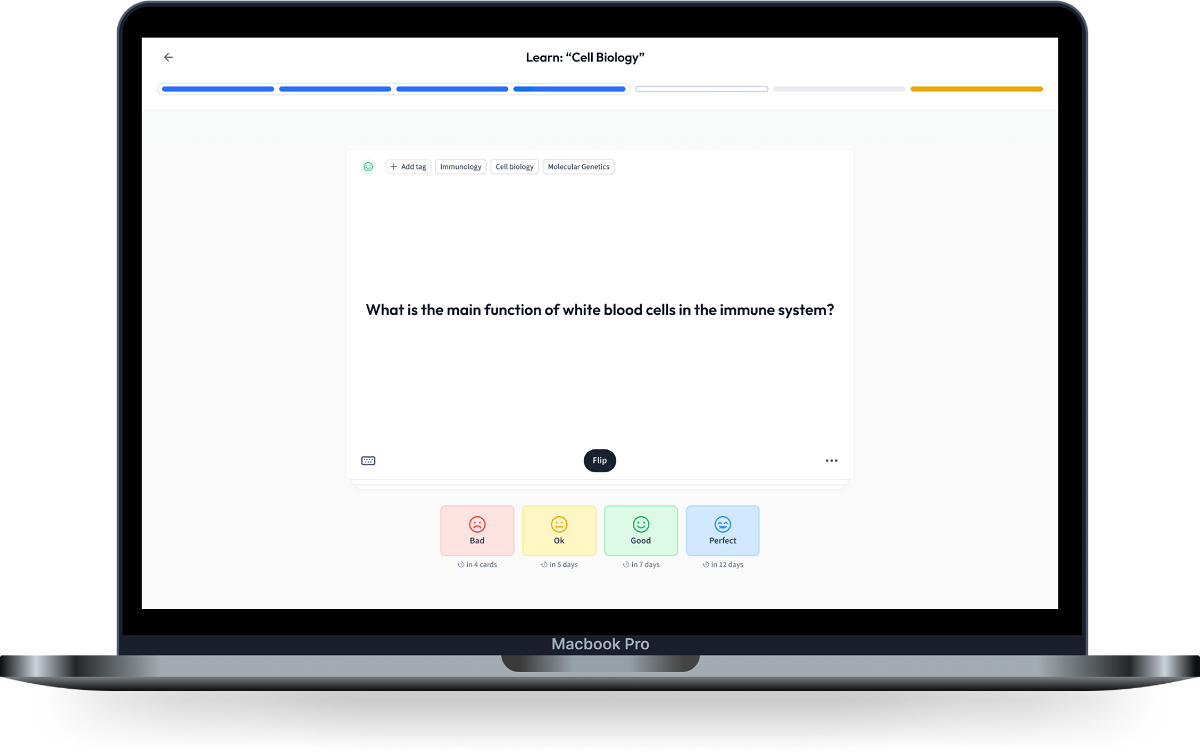
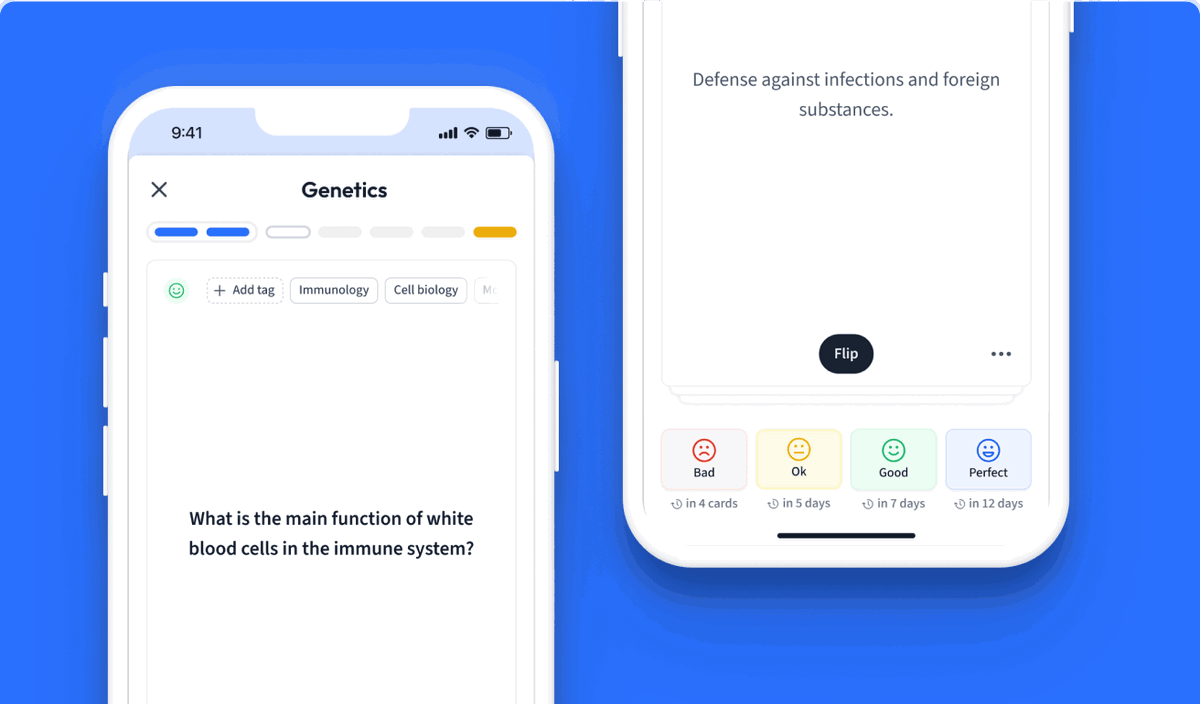
Learn with 27 Python flashcards in the free StudySmarter app
Already have an account? Log in
Frequently Asked Questions about Python
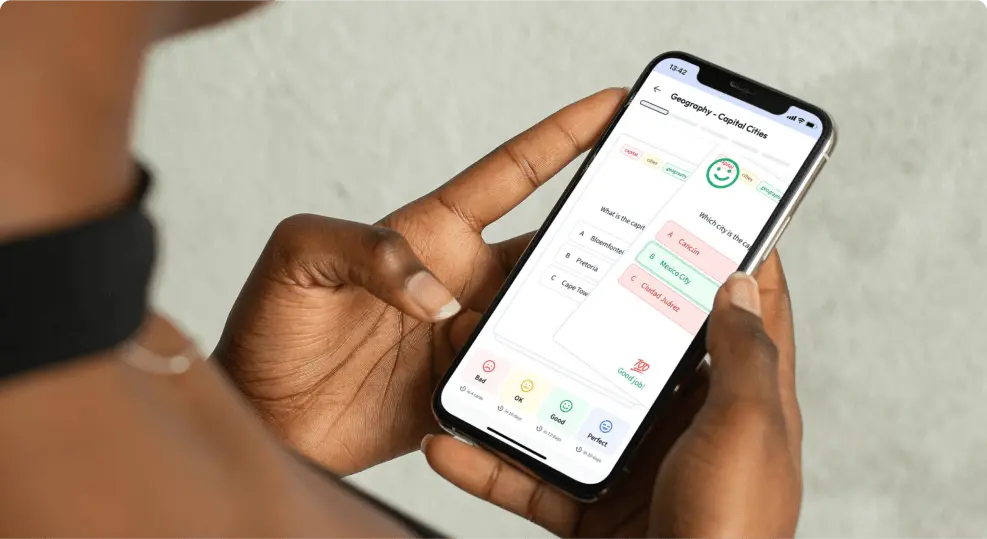
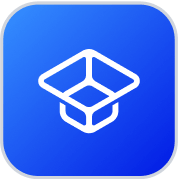
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more