Jump to a key chapter
What Are Python Arrays?
Python Arrays are a fundamental concept in Computer Science, particularly for those diving into the world of programming with Python. They play a crucial role in data management and operations. Through this exploration, you'll gain valuable insights into how arrays function in Python, starting from the basics to applying them in practical scenarios.
Understanding Python Arrays Definition
Python Array: A collection of items stored at contiguous memory locations. The idea is to store multiple items of the same type together. This makes the process of accessing and manipulating these items much more efficient.
In Python, arrays are handled by the array module. Unlike lists, arrays in Python can only store data of the same type, making them optimal for mathematical operations and more complex data structures like stacks and queues. Arrays come into play in scenarios where performance and memory efficiency are essential.
Knowing when to use arrays over lists can significantly optimise your Python code, especially in data-intensive applications.
Python Arrays Example to Get You Started
To understand how arrays function in Python, let's walk through a simple example of how to create and manipulate them.
import array as arr # Creating an array of integer type my_array = arr.array('i', [1, 2, 3, 4, 5]) # Accessing elements print(my_array[0]) # Output: 1 # Length of the array print(len(my_array)) # Output: 5 # Adding an element my_array.append(6) print(my_array) # Output: array('i', [1, 2, 3, 4, 5, 6]) # Removing an element my_array.remove(3) print(my_array) # Output: array('i', [1, 2, 4, 5, 6])
This example highlights several key operations you can perform with Python arrays, including creating an array, accessing elements, measuring the length of the array, and adding or removing elements. Python's array module provides a variety of methods for working with arrays, making them highly versatile for various programming tasks.
The Importance of Memory Efficiency: One of the reasons arrays are preferred over lists in certain scenarios is their memory efficiency. Arrays allocate a fixed size of memory, and because all elements must be of the same type, Python can optimise the storage and access of these elements, resulting in faster and more memory-efficient code.
Handling Python Arrays
Python arrays are crucial for managing sequences of data efficiently. In this section, you'll learn about merging, sorting, understanding essential methods, and slicing arrays to simplify data operations and make your Python code more efficient and readable.
Merging Arrays in Python: A Step-by-Step Guide
Merging arrays in Python is a common operation that combines two or more arrays into a single array. This operation is especially useful when dealing with large datasets or when you need to concatenate data from multiple sources.
import array as arr # First Array array1 = arr.array('i', [1, 2, 3]) # Second Array array2 = arr.array('i', [4, 5, 6]) # Merging array1 and array2 merged_array = arr.array('i') for element in array1: merged_array.append(element) for element in array2: merged_array.append(element) print(merged_array) # Output: array('i', [1, 2, 3, 4, 5, 6])
The merge operation illustrated above is straightforward but crucial for data processing and manipulation tasks. By appending elements from one array to another, you can easily combine datasets for further analysis or operations.
How to Sort Array Python Style
Sorting arrays is a fundamental operation in programming that organises elements in a specified order. Python offers a flexible approach to sorting arrays, making it simple to arrange data either in ascending or descending order.
import array as arr # Creating an array my_array = arr.array('i', [3, 1, 4, 1, 5, 9, 2]) # Sorting the array in ascending order sorted_array = sorted(my_array) print(sorted_array) # Output: [1, 1, 2, 3, 4, 5, 9] # For descending order my_array.reverse() print(my_array) # Output: array('i', [2, 9, 5, 1, 4, 1, 3])
Whether sorting data for analysis, display, or organisational purposes, Python provides intuitive and efficient means of accomplishing this task, highlighting its versatility in handling diverse data operations.
Essential Python Array Methods You Should Know
Understanding and applying Python array methods can significantly enhance your data manipulation capabilities. Below are some essential methods that are invaluable:
- .append(value) - Adds an element to the end of the array.
- .extend(array) - Adds multiple elements to the end of the array.
- .pop(index) - Removes an element at a specified index and returns it.
- .remove(value) - Finds and removes the first occurrence of an element.
- .reverse() - Reverses the order of the array elements in place.
Harnessing these methods can facilitate complex data operations, making your code more concise and your algorithms more efficient.
Python Array Slicing: Simplifying Your Data
Array slicing is a powerful feature in Python that allows you to access subsets of an array. This can be incredibly useful for data analysis and manipulation, offering a simple yet flexible way to work with large datasets.
import array as arr # Creating an array my_array = arr.array('i', [1, 2, 3, 4, 5]) # Slicing the array # Access elements from 1 to 3 slice_array = my_array[1:4] print(slice_array) # Output: array('i', [2, 3, 4])
Slicing arrays not only simplifies accessing data but also enhances readability and efficiency of your code by allowing you to work with only the relevant portions of your dataset, thus optimising resource utilisation.
Comparing Python Arrays and Lists
When you're diving into Python, you'll quickly come across two fundamental data structures: Python Arrays and Lists. While they seem similar at first glance, understanding their key differences is crucial for efficient coding and data management.Let's explore these differences in detail, helping you decide when to use each in your Python projects.
Key Differences Between Python Arrays and Lists
Arrays and Lists in Python serve the purpose of storing data, but they're designed for different types of use cases. The choice between them depends on the nature of your task and the requirements of your program.Here are the main points that set them apart:
- Type of Element: Arrays can only contain elements of the same data type, making them ideal for mathematical operations and data analysis tasks. On the other hand, Lists can hold elements of varying data types, providing greater flexibility for general-purpose programming.
- Performance: Because Arrays enforce a single data type, operations on them can be carried out more efficiently. This makes them faster for numerical computation and manipulation. Lists are generally slower because they need to store additional information about each element's data type.
- Functionality: The Python standard library provides the array module, which offers basic functionality for array manipulation. Lists, however, are a built-in Python data type and come with a wide variety of methods that make them more versatile for handling different types of operations.
- Usage: Arrays are used primarily for arithmetic computations and handling large amounts of similar data efficiently. Lists are the go-to data structure for general-purpose tasks, like collecting heterogeneous data and manipulating it in various ways.
Understanding the difference in memory allocation can provide further insight into why arrays can be more efficient in certain scenarios. When an array is created, Python allocates a block of memory that's optimised for storing items of the same type. This streamlined approach allows for faster access and manipulation of data stored in an array. Lists, by contrast, require a bit more memory to manage their flexibility, storing both the elements and the type of each element, which can lead to slightly slower performance.This doesn't make one better than the other universally; it's about choosing the right tool for the task at hand.
Use arrays when you're dealing with large quantities of data of the same type and computations where performance is critical. Choose lists when you need a simple, versatile data structure that can handle a variety of elements.
Exploring Python 2D Arrays
When you embark on your journey of understanding Python, one fascinating area you'll encounter is the concept of 2D arrays. A Python 2D array is essentially an array of arrays, enabling you to store data in a grid-like structure. This concept is not only pivotal in Python but in programming at large, as it closely mimics real-life data structures like spreadsheets.In this exploration, you'll gain foundational knowledge on Python 2D arrays, and through practical examples, you'll see them spring into action. This will arm you with the tools you need for effective data manipulation and analysis in Python.
Getting to Grips with Python 2D Arrays: Basics
Python 2D Array: A collection of items arranged in rows and columns, much like a matrix or table. In Python, 2D arrays are implemented by nesting lists (or arrays) within a list, effectively creating a 'list of lists'.
At the core of understanding 2D arrays is recognising that each element in the outer list represents a row, with each of these elements (or rows) being a list itself that represents the columns. This row-column paradigm makes 2D arrays incredibly useful for a multitude of applications, including but not limited to:
- Storing matrix data in scientific computing
- Representing games boards like chess or tic-tac-toe
- Handling multi-dimensional data in Data Analysis
Though Python itself does not have a built-in array type like some other programming languages, the list data structure is highly versatile and more than capable of handling 2D arrays efficiently. Moreover, for more specialized tasks, Python offers libraries like NumPy, which provide dedicated support for handling arrays of any dimensionality with ease.
Though Python lists are versatile, making use of libraries like NumPy for handling 2D arrays can significantly optimise performance, especially for complex calculations and large datasets.
Practical Examples of Python 2D Arrays in Action
To solidify your understanding, let's dive into some practical examples showcasing Python 2D arrays in action. These examples will help you grasp how to create, access, and manipulate 2D arrays.
# Creating a 2D array my_2d_array = [[1, 2, 3], [4, 5, 6], [7, 8, 9]] # Accessing the first row first_row = my_2d_array[0] print(first_row) # Output: [1, 2, 3] # Accessing the second element of the first row element = my_2d_array[0][1] print(element) # Output: 2 # Modifying an element my_2d_array[1][2] = 10 print(my_2d_array) # Output: [[1, 2, 3], [4, 5, 10], [7, 8, 9]]
This example demonstrates the intuitive nature of working with 2D arrays in Python. By simply nesting lists, you can create complex data structures that can be easily accessed and modified using indices.For tasks involving numerical computations or when working with large 2D arrays, leveraging specialised libraries like NumPy can further simplify operations, providing functions that enable efficient computations on arrays of any size.
When undertaking tasks that require manipulation of 2D arrays, such as matrix multiplication or image processing, Python's built-in lists might not suffice due to performance considerations. This is where libraries like NumPy shine, offering a broad range of functionalities specifically optimised for numerical computations.For example, NumPy arrays support vectorised operations, allowing for batch operations on array elements without the need for explicit loops. This not only makes the code more concise but significantly speeds up the computation, a crucial advantage when processing large datasets or real-time data.
Python Arrays - Key takeaways
- Python Arrays Definition: A collection of items stored at contiguous memory locations designed for efficient access and manipulation.
- Python Arrays vs Lists: Arrays hold items of the same type for efficiency in calculations, while lists hold items of any type and are more flexible.
- Merging Arrays in Python: Combining two or more arrays into a single array for large datasets or multiple data sources.
- Sort Array Python: Python allows sorting of arrays in ascending/descending order, enhancing data organisation.
- Python 2D Arrays: An array containing arrays, useful for storing data in grid-like structures like matrices or tables.
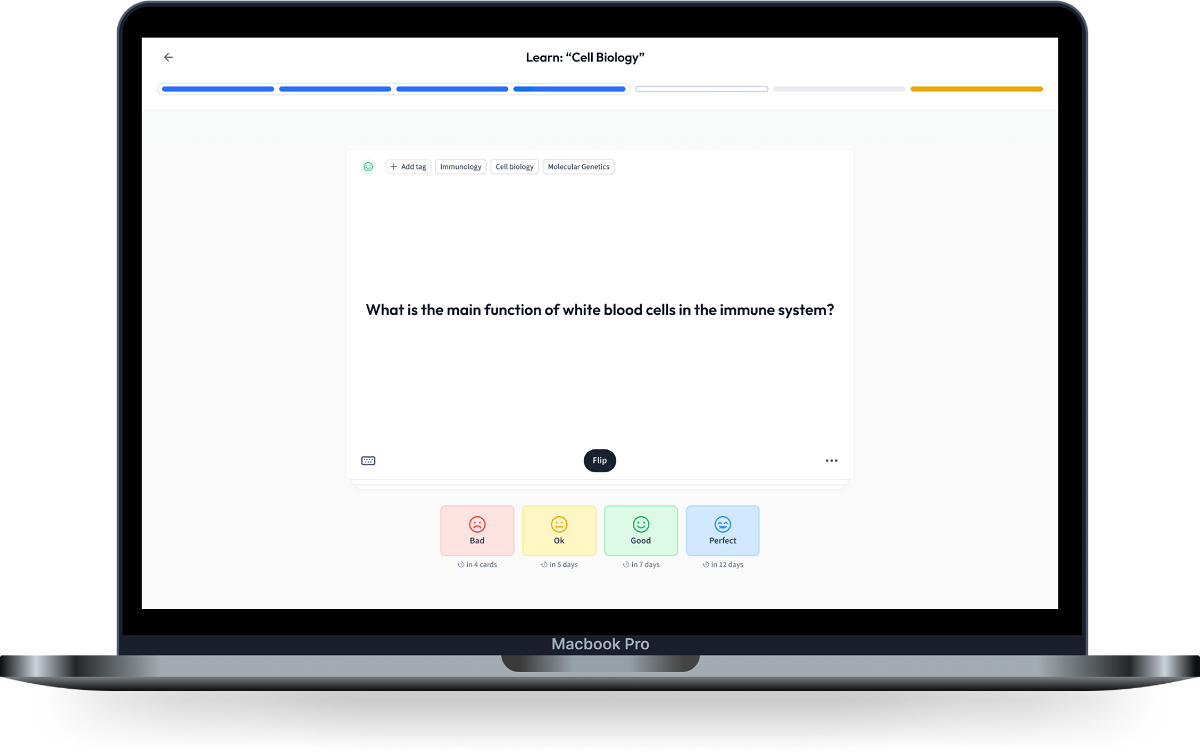
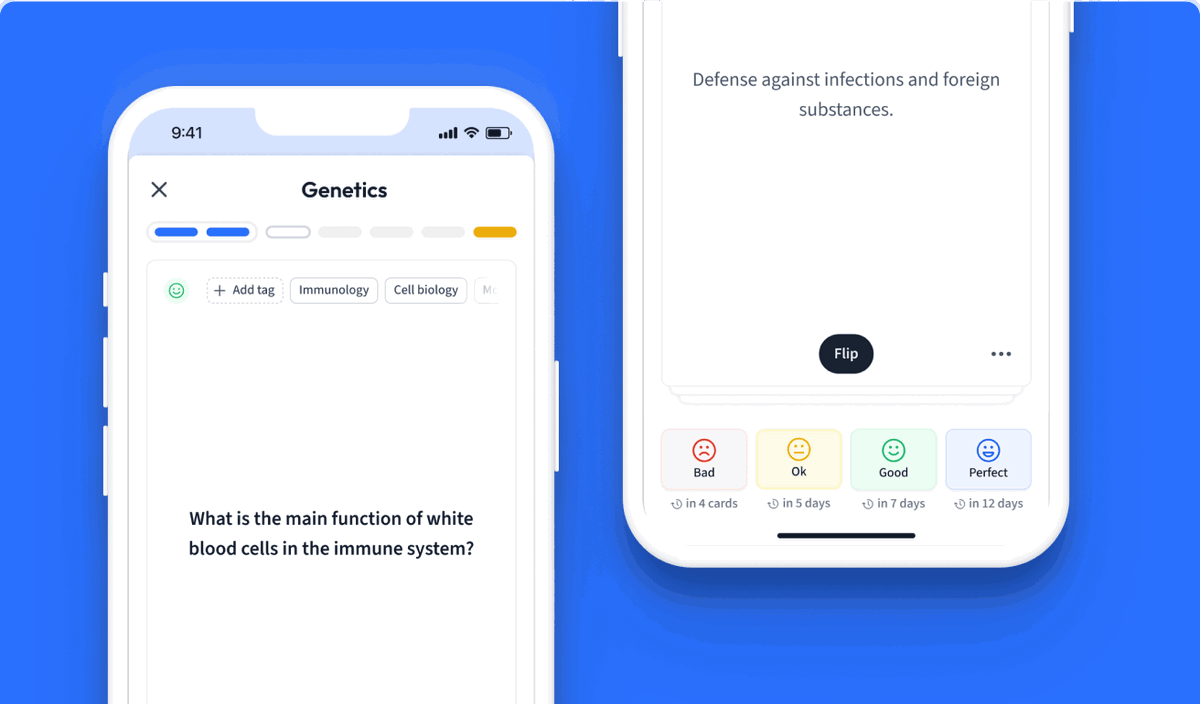
Learn with 42 Python Arrays flashcards in the free StudySmarter app
Already have an account? Log in
Frequently Asked Questions about Python Arrays
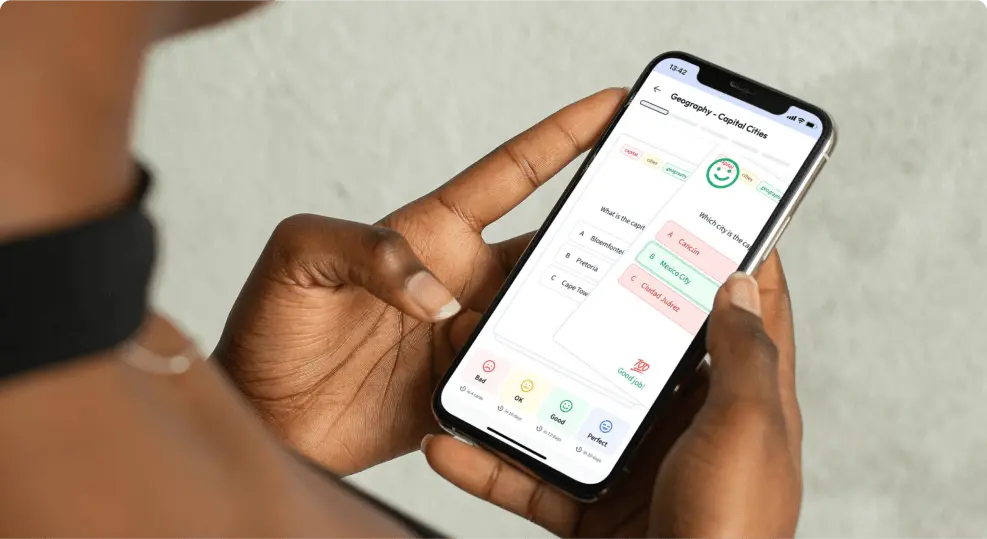
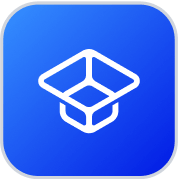
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more