Jump to a key chapter
The Basics of Python Assignment Operators
In the realm of computer programming, assignment operators hold a significant role when it comes to assigning values to variables. In Python, like in many other programming languages, assignment operators are used to manipulate and store data. To get a good grasp of the concept, let's dive into the basics of Python assignment operators.Assignment operators in Python are used to assign values to variables. They allow programmers to perform different types of operations and store the results in variables.
Different types of Python Assignment Operators
Python assignment operators can be grouped into two categories - basic assignment operators and compound assignment operators. Here's a list of commonly used Python assignment operators:- Basic Assignment Operator:
= Assigns a value to a variable - Compound Assignment Operators:
+= Addition assignment -= Subtraction assignment *= Multiplication assignment /= Division assignment %= Modulus assignment //= Floor division assignment **= Exponentiation assignment &= Bitwise AND assignment |= Bitwise OR assignment ^= Bitwise XOR assignment >>= Bitwise right shift assignment <<= Bitwise left shift assignment
How Python Assignment Operators work
Assignment operators work by performing an operation on the right-hand side operand and then assigning the result to the left-hand side operand. To clarify this concept, let's examine some examples.Addition assignment operator example: x = 10 # x is assigned the value 10 x += 5 # x is updated to x + 5, which is 15 print(x) # The output will be 15
Python assignment operators not only save time by making code shorter but also offer the advantage of improved performance. Compound assignment operators can lead to faster execution as the interpreted language like Python can optimize such operations more effectively than separate operations.
Python Assignment Operator List and Examples
As we delve deeper into the world of Python assignment operators, we can see that each of these operators serves a distinct purpose and offers unique advantages. To gain a comprehensive understanding of their functionality, let's explore some common Python assignment operators in great detail with the help of practical examples.
+=, -=, *=, and **= Assignment Operators in Python
These are some of the most widely used Python assignment operators:- += (Addition assignment): This operator is used to add the value on the right to the variable on the left and then update the value of the variable.
- -= (Subtraction assignment): This operator is used to subtract the right side value from the left side variable and then update the value of the variable.
- *= (Multiplication assignment): This operator is used to multiply the value on the right with the variable on the left and then update the value of the variable.
- **= (Exponentiation assignment): This operator is used to raise the variable on the left to the power of the value on the right and then update the value of the variable.
x = 10 # x is assigned the value 10 x += 5 # x is updated to x + 5, which is 15 x -= 3 # x is updated to x - 3, which is 12 x *= 2 # x is updated to x * 2, which is 24 x **= 2 # x is updated to x ** 2, which is 576 print(x) # The output will be 576
/=, //=, %=, and &= Assignment Operators in Python
Let's explore some more Python assignment operators and learn how to use them effectively:- /= (Division assignment): This operator is used to divide the variable on the left by the value on the right and then update the value of the variable.
- //= (Floor division assignment): This operator performs floor division on the left side variable by the right side value and then updates the value of the variable.
- %= (Modulus assignment): This operator calculates the modulus of the left side variable divided by the right side value and then updates the value of the variable.
- &= (Bitwise AND assignment): This operator performs a bitwise AND operation between the left side variable and the right side value, and then updates the value of the variable.
x = 50 # x is assigned the value 50 x /= 2 # x is updated to x / 2, which is 25 x //= 3 # x is updated to x // 3, which is 8 x %= 5 # x is updated to x % 5, which is 3 x &= 2 # x is updated to x & 2, which is 2 print(x) # The output will be 2
Overloading Python Assignment Operators
What is Python Assignment Operator Overloading?
Operator overloading is a programming concept that allows the same operator to perform various functions based on the types of operands interacted within an operation. In Python, we can overload assignment operators using special methods called 'magic methods' or 'dunder methods' (double underscore methods). These methods have specific names and functionalities that make operator overloading possible. By overloading assignment operators, you can extend their functionality in custom classes, providing a more convenient and readable programming style.Using Python Assignment Operator Overloading in Custom Classes
To overload assignment operators in custom classes, you need to implement the corresponding magic methods in your class definition. Let's look at the most commonly used magic methods for overloading assignment operators in custom classes:- __add__(): Corresponds to the '+=' operator
- __sub__(): Corresponds to the '-=' operator
- __mul__(): Corresponds to the '*=' operator
- __truediv__(): Corresponds to the '/=' operator
- __floordiv__(): Corresponds to the '//=' operator
- __mod__(): Corresponds to the '%=' operator
- __pow__(): Corresponds to the '**=' operator
- __and__(): Corresponds to the '&=' operator
- __or__(): Corresponds to the '|=' operator
- __xor__(): Corresponds to the '^=' operator
- __lshift__(): Corresponds to the '<<=' operator
- __rshift__(): Corresponds to the '>>=' operator
Advantages of Python Assignment Operator Overloading
Overloading assignment operators in Python offers various advantages, making it an essential feature for custom classes. These benefits include:- Improved readability: By overloading assignment operators, you can make your code more self-explanatory and easier to understand since the operators are more intuitive than function calls.
- Enhanced expressiveness: Operator overloading enables custom classes to provide a natural syntax that closely resembles the native data types, allowing programmers to write more concise and efficient code.
- Increased consistency: When operators are consistently represented across user-defined and built-in classes, the language semantics remain uniform, resulting in improved consistency.
- Greater abstraction: Overloading assignment operators allows you to hide the implementation details from the users, providing them with a straightforward and consistent interface for interacting with custom objects.
Python Assignment Operator Precedence
In any programming language, understanding how the language interprets and executes different operations is crucial, and Python is no exception. When working with Python assignment operators, one important aspect you need to keep in mind is operator precedence. Operator precedence determines the order in which operators are executed in an expression, which can significantly impact the final result. Grasping the concept of operator precedence allows you to write code that is both accurate and efficient.Understanding Operator Precedence in Python
Operator precedence in Python represents a set of rules the language follows to determine the execution priority of different operators in an expression. When an expression has two or more operators, precedence rules dictate which operators are evaluated first. Understanding operator precedence is essential for writing well-structured and error-free code, as it allows you to predict the outcome of an expression correctly by taking into account the order in which the various operators are executed.Precedence Rules for Python Assignment Operators
Python assignment operators have their own precedence levels, just like arithmetic and logical operators. However, assignment operators generally have the lowest precedence level, which means that they are performed last when multiple operations are involved. Here is a brief overview of the precedence rules for Python assignment operators:- Assignment operators (=, +=, -=, *=, etc.) are evaluated from right to left.
- Assignment operators have a lower precedence compared to all arithmetic, relational, and logical operators.
- When multiple assignment operators are present in an expression, Python evaluates the rightmost assignment operator first and proceeds from right to left.
To illustrate these precedence rules, consider the following example: ```python x = 10 + 5 * 2 ``` In this example, the multiplication (*) operator takes precedence over the addition (+) and assignment (=) operators. Therefore, the expression will be evaluated as follows: `x = 10 + (5 * 2)`. The final value of x will be 20.
Tips for Managing Python Assignment Operator Precedence
Knowing the existing precedence rules for Python assignment operators is a fundamental part of writing accurate and well-structured code. Here are some practical tips to help you manage Python assignment operator precedence more effectively:- Always use parentheses to group operations explicitly and avoid ambiguities. Using parentheses not only ensures that the expression is evaluated in the correct order but also improves the readability of your code.
- When evaluating complex expressions, break them down into smaller, simpler expressions and assign intermediate results to temporary variables. This approach not only makes your code more readable but also minimizes the chance of encountering precedence issues.
- If you are uncertain about the precedence levels of the operators involved in an expression, consult the Python documentation for clarification. Familiarizing yourself with the precedence rules will significantly enhance your ability to write accurate and reliable code.
- Always test your code thoroughly to ensure that the operator precedence does not introduce any unintended outcomes. Adequate testing will help you identify and correct potential errors and ambiguities caused by operator precedence.
Python Assignment Operator - Key takeaways
Python Assignment Operator - used to assign values to variables and perform operations while updating variable values.
Python assignment operator overload - using 'magic methods' to extend functionality of assignment operators for custom classes.
Python assignment operator list - Basic (=) and compound (+=, -=, *=, etc.) assignment operators available in Python for different types of operations.
What is the assignment operator in python? - '=' is the basic assignment operator used for assigning values to variables.
Python assignment operator precedence - Set of rules governing the order in which assignment operators are executed in an expression, assignment operators have the lowest precedence level.
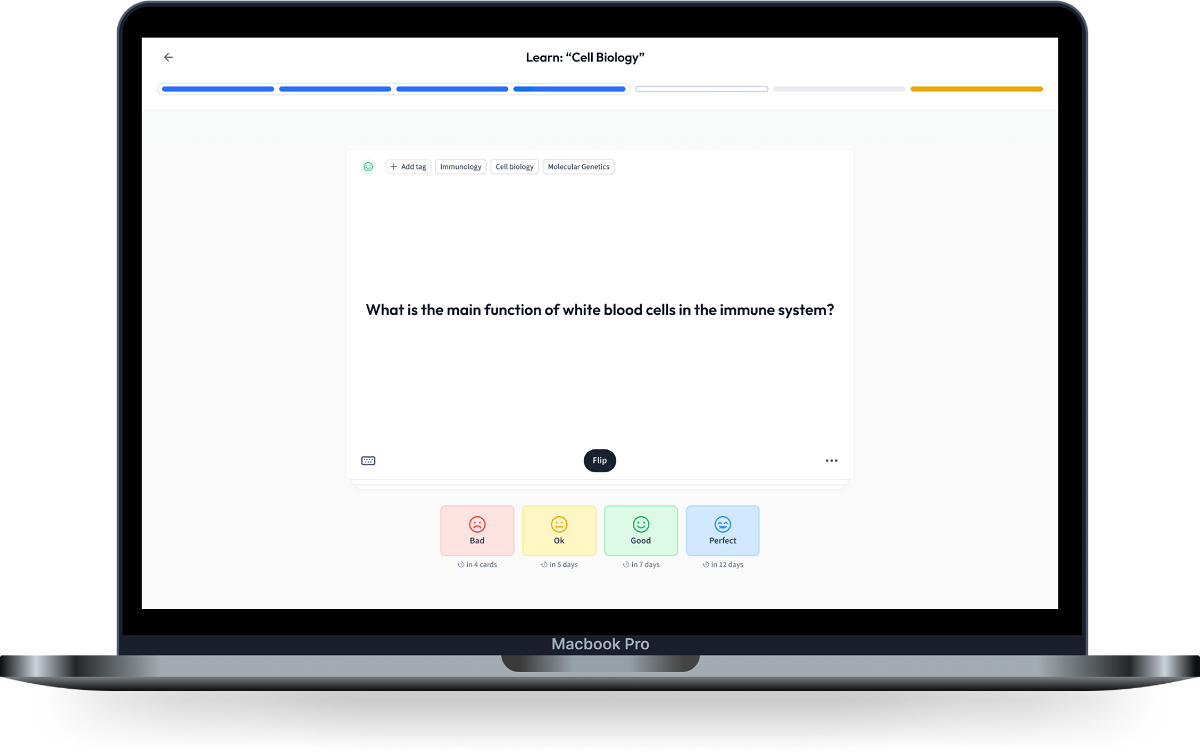
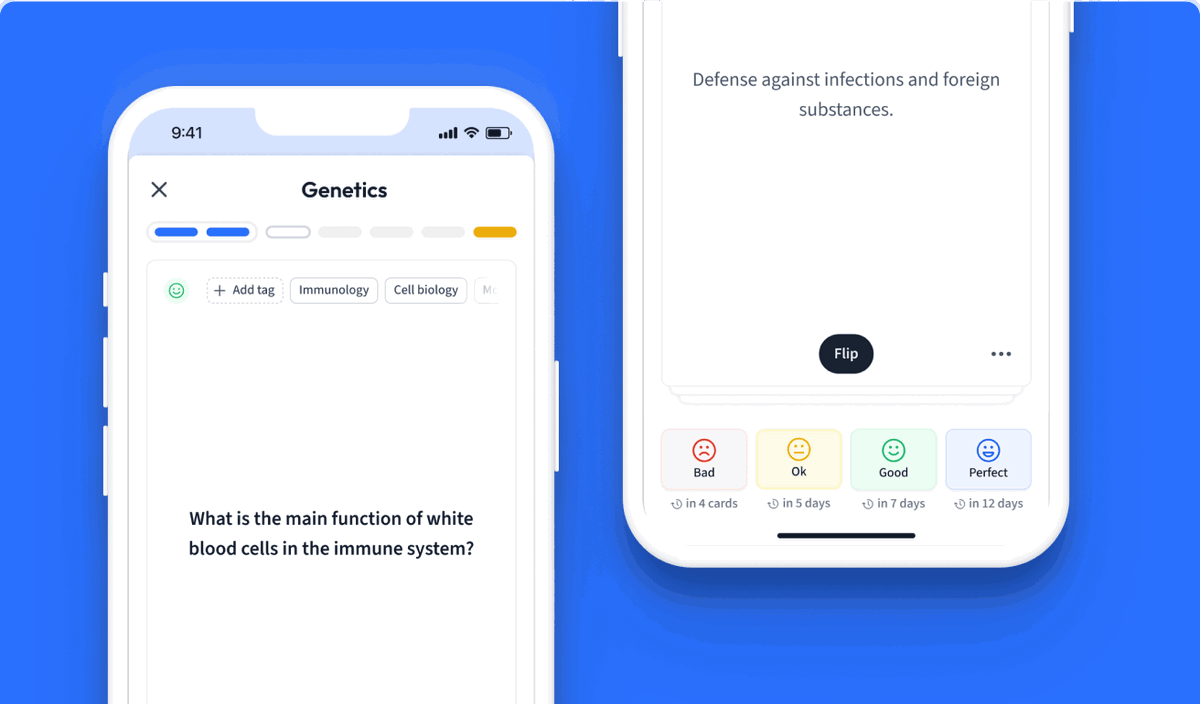
Learn with 31 Python Assignment Operator flashcards in the free StudySmarter app
Already have an account? Log in
Frequently Asked Questions about Python Assignment Operator
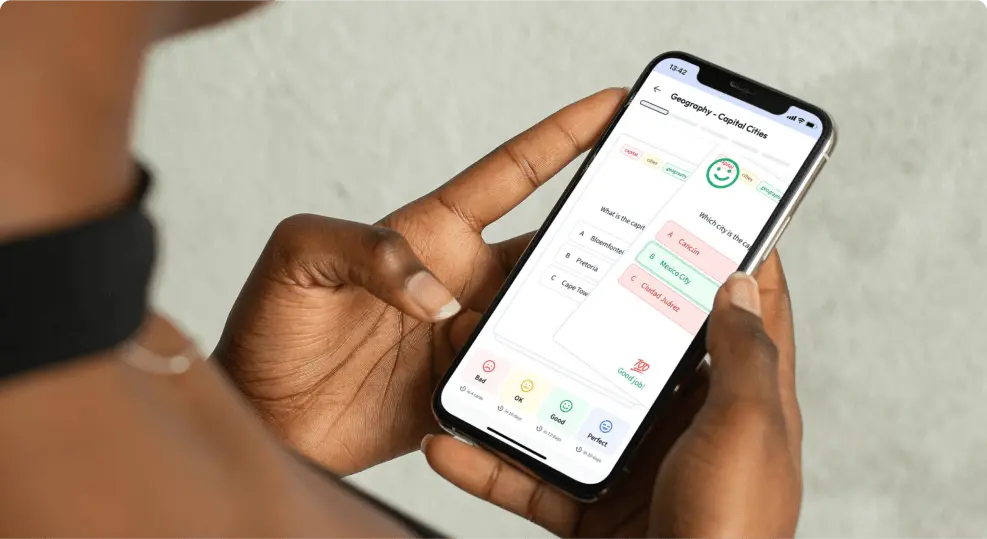
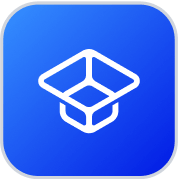
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more