Jump to a key chapter
Introduction to Python Bar Chart
Bar charts are widely used in data visualization to represent categorical data in a simple and informative way. In Python, bar charts can be easily created with the help of several libraries and tools. In this article, we will guide you through the basics of creating bar charts in Python, discuss their advantages in data visualization, and provide some tips and examples on how to make your charts effective and engaging.
Basics of Bar Charts in Python
A Python bar chart is a graphical representation of data using rectangular bars of various lengths, where the length of each bar depends on the value it represents. Bar charts can be used to visualize different types of categorical data, such as comparing the values of categories in a single dataset or comparing the values of multiple datasets.
A categorical variable is a variable that can take a limited number of distinct values (categories), such as colours, car brands, or countries.
There are several popular Python libraries for creating bar charts, including:
- Matplotlib
- Seaborn
- Plotly
To get started with creating bar charts in Python, it's essential to have a basic understanding of the syntax and functionalities of these libraries. Most of the time, you'll be able to achieve the desired results just by tweaking a few lines of code.
You can install these libraries via pip. For example, to install Matplotlib, run: `pip install matplotlib`.
Let's have a look at some of the primary components of a bar chart:
- Bars: The main elements of a bar chart, representing the values of the categories.
- Axis: The horizontal and vertical lines that define the reference frame for the chart.
- Ticks and Labels: Marks along the axes that indicate the categories and their corresponding values.
- Title and Legends: Descriptive text that provides information about the chart and its data.
Advantages of Bar Charts in Data Visualization
Bar charts provide various benefits in data visualization. These advantages make them a popular choice among analysts and professionals working with data. Some of the main advantages include:
- Easy to create and understand: Bar charts are simple to design and interpret, making them an excellent choice for data visualization, especially for beginners.
- Comparing categories: Bar charts enable quick and clear comparison between different categories or groups within a dataset.
- Multidimensional analysis: Bar charts can accommodate multiple categorical variables in one chart, allowing for more complex data analysis.
- Customizability: Bar charts offer flexibility in terms of design and layout, which enables presenting the data more effectively and aesthetically appealing.
- Wide application: Bar charts are versatile and can be used in various domains, including business, scientific research, or social studies.
For instance, imagine you want to compare the sales of different fruit types during a specific month in a grocery store. A bar chart allows you to quickly see which fruit types were more popular (had higher sales) and helps you identify trends or outliers. You could also expand the chart by incorporating additional categorical data, such as the sales trends across multiple stores.
Overall, Python bar charts are a powerful tool in data visualization. They provide a simple yet effective way to represent and analyze categorical data, allowing for meaningful insights and easy communication of your findings. By understanding the basics of bar charts and their advantages in data visualization, you can create informative and engaging charts to enhance your data analysis tasks.
Creating a Python Stacked Bar Chart
Stacked bar charts are an extension of standard bar charts and are useful for visualising categorical variables with multiple subcategories. The bars are divided into segments, each representing a subcategory, and the length or height of the segments is determined by their respective values. Stacked bar charts offer a comprehensive view of the data, enabling comparison not only between the categories but also between the subcategories within them.
Step-by-Step Guide to Creating Stacked Bar Charts in Python
Creating a stacked bar chart in Python is a relatively straightforward process that involves the use of popular libraries like Matplotlib or Seaborn. Let's explore the steps required to create a stacked bar chart using Matplotlib:
- Import libraries: First, import the necessary libraries. Matplotlib and Pandas are the primary libraries required to create stacked bar charts.
import matplotlib.pyplot as plt
import pandas as pd
- Prepare the data: Organise the data into a Pandas DataFrame. The data should include the categories, subcategories, and their respective values. Additionally, make sure the subcategory values are aggregated in a way that allows stacking.
data = {'Category': ['A', 'B', 'C'],
'Subcategory1': [10, 20, 30],
'Subcategory2': [15, 25, 35],
'Subcategory3': [20, 30, 40]}
df = pd.DataFrame(data)
- Create the chart: Use the `plot` function from the imported `matplotlib.pyplot` library, specifying the kind of chart as 'bar', setting the 'stacked' parameter to 'True', and customising other relevant options.
ax = df.plot(x='Category', kind='bar', stacked=True)
plt.show()
These steps will result in a basic stacked bar chart. To achieve a more sophisticated chart, further customisation and additional subcategories can be included.
Customisation Options for Stacked Bar Charts
Customisation improves the readability and visual appeal of the chart. Some common options for enhancing stacked bar charts in Python are:
- Colours: Modify the colours of the bars, segments, and borders according to your preference or to match a specific theme.
- Axis labels and ticks: Use descriptive labels for the axes, adjust their font size, style, and colour. Modify the tick marks for clarity and readability.
- Chart title and legends: Add informative titles and legends to your chart, aiding in the interpretation of the displayed data. Customise the position, size, and style of these elements as required.
- Bar width and spacing: Adjust the width of the bars and the spacing between them to create a visually appealing chart.
- Gridlines: Include gridlines to make it easier to compare values across categories and subcategories.
- Annotations: Add data labels, values or custom annotations to the chart to enrich the information provided and enhance the audience's understanding of the data.
For example, here's how you can customise some elements of a stacked bar chart:
# Customise colours
colors = ['#FFA07A', '#7CFC00', '#1E90FF']
ax = df.plot(x='Category', kind='bar', stacked=True, color=colors)
# Add axis labels
plt.xlabel('Category')
plt.ylabel('Values')
# Add chart title
plt.title('Stacked Bar Chart Example')
# Add legend
plt.legend(title='Subcategories', loc='upper right')
# Display chart
plt.show()
By employing these customisation options, you can create more visually appealing and informative stacked bar charts in Python, which will ultimately help present the data more effectively and make your analysis more accessible to various audiences.
Plotting a 3D Bar Chart in Python
3D bar charts are an alternative to 2D bar charts and can be used to represent more complex and multidimensional datasets. These charts display the data in a three-dimensional space, enabling easier comparison among multiple variables. In this section, we'll dive into the details of creating and analysing 3D bar charts in Python, as well as comparing them to their 2D counterparts for data interpretation.
How to Create and Analyse 3D Bar Charts in Python
Creating a 3D bar chart in Python involves using Matplotlib, a popular data visualisation library. Before diving into the steps, it's important to note that 3D bar charts may not provide the same level of clarity as a 2D bar chart due to the complexity of the visual representation. It's crucial to ensure the use of 3D bar charts aligns with your data and purpose. Here are the steps for creating a 3D bar chart:
- Import libraries: Import the required libraries, including 'Axes3D' from the 'mpl_toolkits.mplot3d' module.
import matplotlib.pyplot as plt
import numpy as np
from mpl_toolkits.mplot3d import Axes3D
- Prepare the data: Organise the data into a suitable format, typically a NumPy array or a Pandas DataFrame. Ensure the dataset includes the variables you wish to display on the X, Y, and Z axes.
x = np.array([1, 2, 3])
y = np.array([1, 2, 3])
z = np.array([10, 15, 20])
- Create the chart: Initialise a figure and 3D plot axis, then use the 'bar' function from the 'Axes3D' module to create the 3D bar chart. Customise the appearance and labels as needed.
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
ax.bar(x, y, z, zdir='y', color='#FFA07A')
ax.set_xlabel('X Axis Label')
ax.set_ylabel('Y Axis Label')
ax.set_zlabel('Z Axis Label')
plt.show()
Analysing a 3D bar chart relies on the same principles as interpreting a 2D bar chart. Comparing the heights of the bars allows for an understanding of the underlying data. However, it's essential to be cautious when analysing 3D bar charts, as perspective distortion might interfere with accurate comparisons.
Comparison of 2D and 3D Bar Charts for Data Interpretation
Both 2D and 3D bar charts serve the purpose of visualising categorical data. However, there are differences in their complexity, clarity, and application. Understanding these differences can help you decide which type of bar chart is best suited for your data interpretation needs.
Some key differences between 2D and 3D bar charts include:
- Complexity: 3D bar charts are more complex due to the additional dimension, making them harder to design, interpret, and understand than 2D bar charts.
- Clarity: 3D bar charts can sometimes lead to distorted perspectives, adding difficulty to accurate data interpretation. 2D bar charts, on the other hand, provide a clearer and straightforward representation of categorical data.
- Data representation: While 2D bar charts are suitable for displaying data with a single dependent variable, 3D bar charts can enhance the display of multidimensional datasets that have more than one dependent variable.
- Scope of application: The choice between 2D and 3D bar charts depends on the intended outcome and dataset complexity. 2D bar charts generally work well for simpler datasets and processes, whereas 3D bar charts may be better suited for more complicated scenarios.
In conclusion, 3D bar charts can be a powerful tool for visualising more complex datasets but should be used with caution due to the increased difficulty in interpretation. It's essential to consider the purpose and the target audience when selecting a chart type, as well as the important differences between 2D and 3D bar charts when presenting your data.
Understanding Clustered Bar Charts in Python
Clustered bar charts, also known as grouped bar charts, are an extension of standard bar charts that can be used to represent multiple datasets or categorical variables in a single chart. They enable easy comparison among different categories as well as among groups within each category. In this section, we will delve into the concept of clustered bar charts in Python and their applications in data visualization.
Implementing Clustered Bar Charts using Python Libraries
Creating a clustered bar chart in Python is relatively straightforward, thanks to libraries like Matplotlib, Seaborn, and Plotly. Let's explore the process of creating clustered bar charts in Python using the popular library Matplotlib.
- Import libraries: Start by importing the necessary libraries, including the Matplotlib library and the Pandas library for managing data structures.
import pandas as pd
import matplotlib.pyplot as plt
- Prepare the data: Create a Pandas DataFrame consisting of categories and their corresponding values for different groups. Make sure the data is organised so that comparisons can be made across groups and categories.
data = {'Category': ['A', 'B', 'C'],
'Group1': [10, 15, 20],
'Group2': [15, 25, 35],
'Group3': [20, 30, 40]}
df = pd.DataFrame(data)
- Create the chart: Utilise the `plot` function from Matplotlib library, specifying the chart type as 'bar' and adjusting the positions of the bars for proper clustering. You can also customise other chart parameters as needed.
ax = df.plot(x='Category', y=['Group1', 'Group2', 'Group3'], kind='bar')
plt.show()
These steps will result in a basic clustered bar chart. To achieve a more advanced chart, further customisation and additional groups or categories can be incorporated.
In addition to Matplotlib, other Python libraries such as Seaborn and Plotly also support creating clustered bar charts with similar functionalities, providing more options for users constrained by specific data visualisation requirements.
Applications and Use Cases of Clustered Bar Charts in Computer Programming
Clustered bar charts find applications in various domains including business, scientific research, social studies, and computer programming. Their ability to present complex data relationships in a visually appealing and graspable format makes them invaluable in these contexts. Here are some typical use cases of clustered bar charts in computer programming:
- Performance evaluation: Clustered bar charts can be used to compare the performance of different algorithms or systems when operating under various conditions or with diverse input data.
- Error analysis: They can help visualise the distribution and impact of different types of errors across various categories, making it easier to identify trends and prioritise improvements.
- Resource usage: Clustered bar charts can display how multiple resources, such as memory, processing time, and network bandwidth, are being used across different components of an application or system.
- User behaviour: In web and application development, clustered bar charts can be employed to analyse user behaviour across different web pages or app features, facilitating design and functionality optimisation decisions.
- Feature importance: In machine learning, clustered bar charts can help to evaluate the importance of various features or predictors contributing to the overall model performance, aiding in feature selection and model tuning.
Overall, clustered bar charts provide a potent tool for data visualization, especially when dealing with multiple datasets or categorical variables. Their numerous applications in computer programming enable users to extract relevant insights and improve their decision-making processes effectively.
Techniques to Create a Bar Chart in Python
In Python, there are several techniques to create effective and visually appealing bar charts. These techniques primarily involve using different libraries and functions tailored to specific requirements. The most popular libraries for creating bar charts in Python include Matplotlib, Seaborn, and Plotly, each offering various features and customisations to cater to diverse data visualisation needs.
Comparing Different Python Libraries for Creating Bar Charts
When it comes to creating bar charts in Python, selecting the appropriate library plays a crucial role. Each library offers unique functionalities, customisation options, and ease of use. Let's dive deep into the details of the most commonly used Python libraries for creating bar charts:
- Matplotlib: Matplotlib is a widely-used data visualisation library for Python that allows for creating static, interactive, and animated charts. It provides a versatile and low-level interface for drawing complex plots, including bar charts, with full customisation capabilities. However, the extensive customisation sometimes leads to longer and more complex code.
- Seaborn: Built on top of Matplotlib, Seaborn offers a high-level interface for creating aesthetic and informative bar charts with minimal effort. It includes advanced statistical capabilities, allowing users to generate complex visualisations with fewer lines of code. Seaborn also integrates well with Pandas DataFrames and automatically deduces the desired information from the data when possible. On the other hand, it may lack some low-level customisations offered by Matplotlib.
- Plotly: Plotly is a powerful data visualisation library that supports creating interactive and responsive graphics, including bar charts, in web browsers. It is compatible with multiple platforms, such as Python, R, and JavaScript. Plotly offers extensive customisation options and the ability to embed charts in web applications, making it a suitable choice for those seeking interactivity and sharability. The main drawback of Plotly is its reliance on web connectivity, as some plot features require an internet connection to function properly.
Tips for Choosing the Right Library for Your Bar Chart Project
Selecting the appropriate library for your bar chart project depends on your specific requirements, preferences, and the complexity of your dataset. Here are some tips to help you choose the right library:
- Evaluate the data: Examine your dataset's complexity and attributes to determine the level of customisation and functionality required in your visualisations. Consider choosing libraries with built-in statistical capabilities for more complex datasets.
- Customisation needs: Assess your customisation requirements, such as colours, axis labels, and annotations. Some libraries, like Matplotlib, offer extensive customisations, while others, like Seaborn, provide more limited options.
- Interactive vs static charts: Determine whether you need interactive or static charts for your project. If interactivity and web-based graphics are essential, choose libraries like Plotly that support these features.
- Learning curve: Consider the ease of use and available documentation for each library. Some libraries, like Seaborn, are beginner-friendly, whereas libraries like Matplotlib might have a steeper learning curve due to their extensive customisation options.
- Integration with other libraries: Evaluate the compatibility of the library with other relevant libraries and tools you may be using in your project, such as Pandas DataFrames, NumPy arrays, and web development frameworks.
- Performance and scalability: Ensure that the chosen library can handle your data's size and scale without compromising performance, especially when dealing with large datasets or multiple complex plots.
Ultimately, the choice of library for your bar chart project depends on your specific needs and priorities. By carefully considering the factors mentioned above, you can make an informed decision and select a library that best suits your data visualisation goals.
Python Bar Chart - Key takeaways
Python Bar Chart: Graphical representation of data using bars of various lengths, where the length represents the value of a categorical variable.
Stacked Bar Chart: An extension of standard bar charts for visualising categorical variables with multiple subcategories, where bars are divided into segments representing different subcategories.
3D Bar Chart: Displays data in a three-dimensional space, enabling easier comparison among multiple variables.
Clustered Bar Chart: Represents multiple datasets or categorical variables in a single chart, allowing for comparisons among different categories and groups within each category.
Create a Bar Chart in Python: Techniques include using libraries such as Matplotlib, Seaborn, and Plotly, each offering specific features and customisations catered to diverse data visualisation needs.
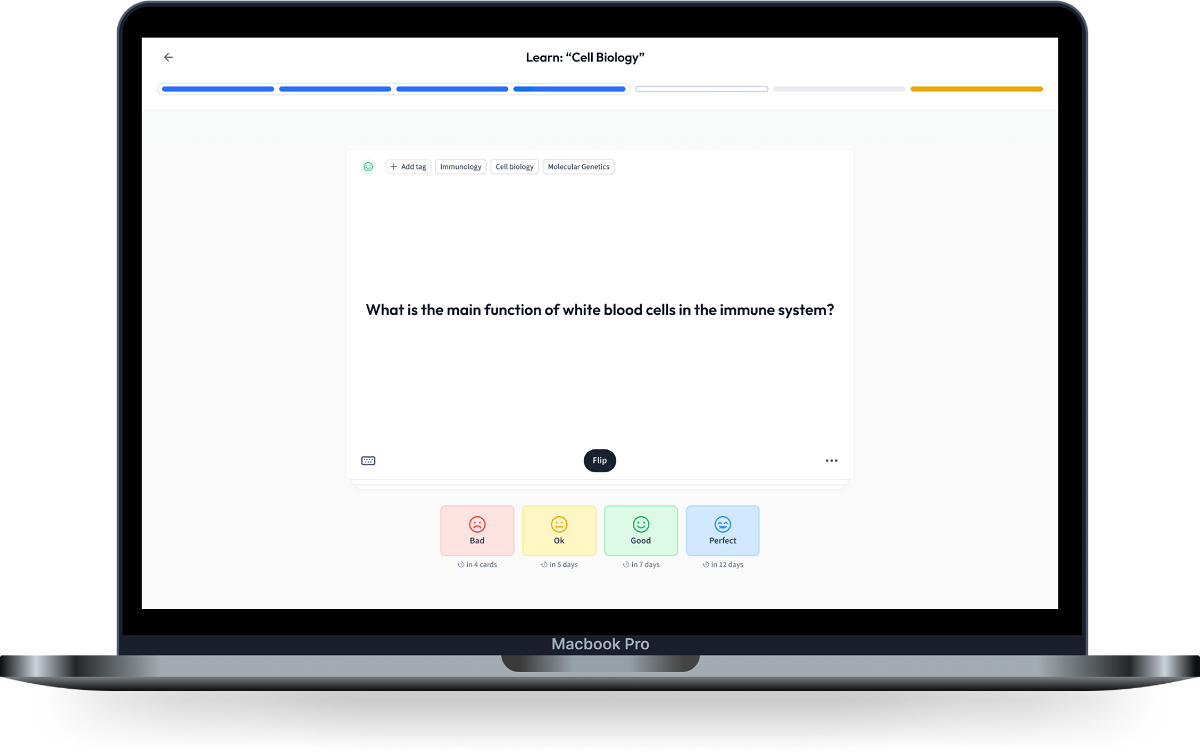
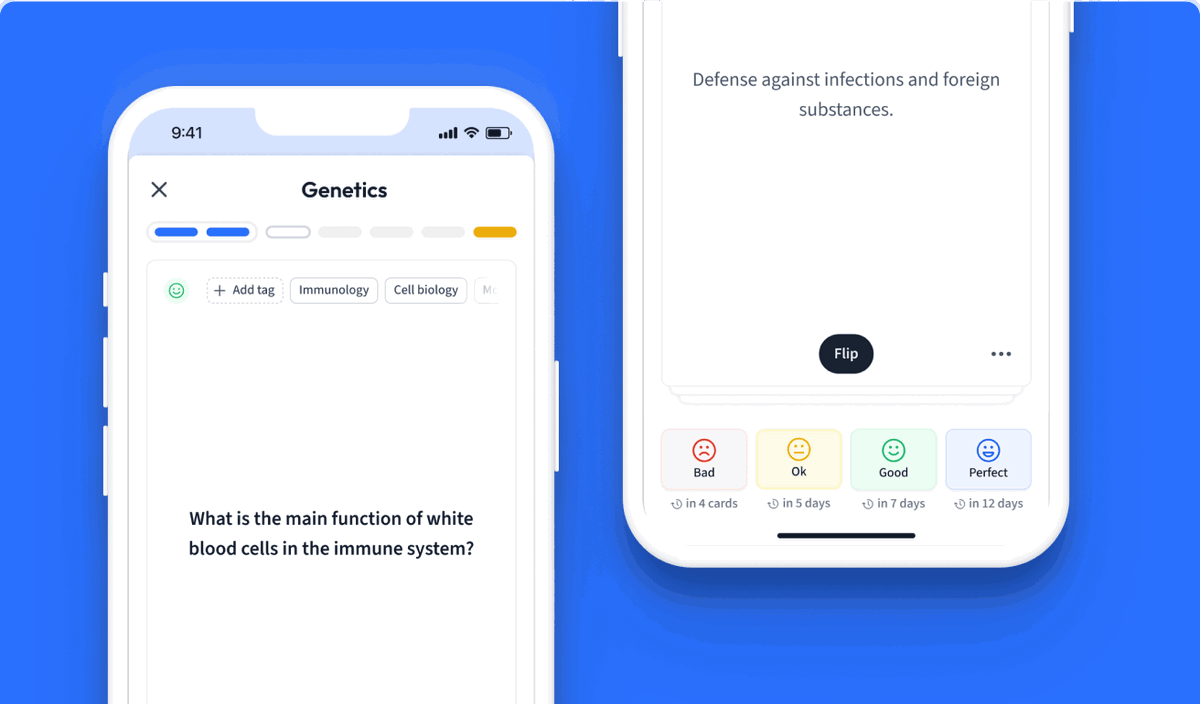
Learn with 29 Python Bar Chart flashcards in the free StudySmarter app
Already have an account? Log in
Frequently Asked Questions about Python Bar Chart
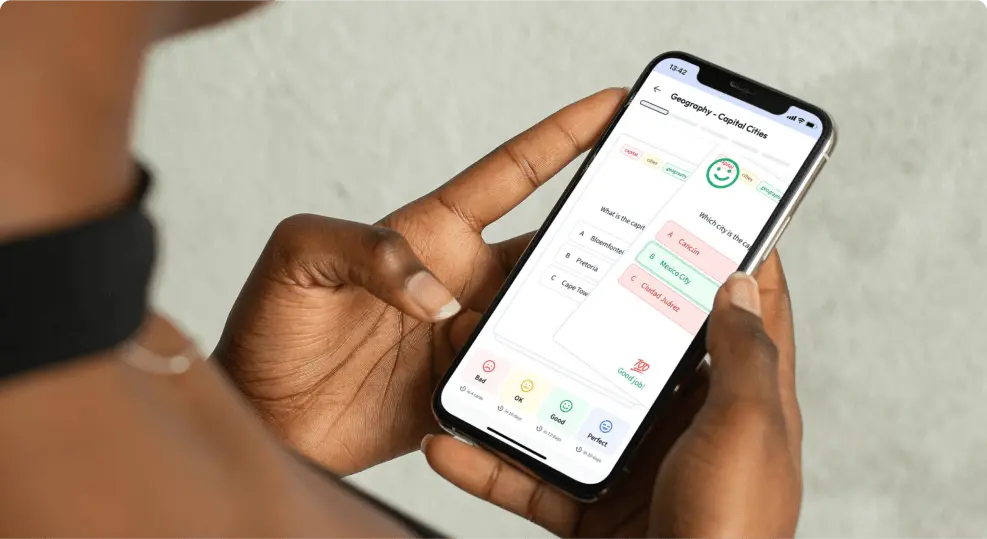
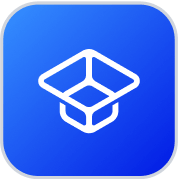
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more